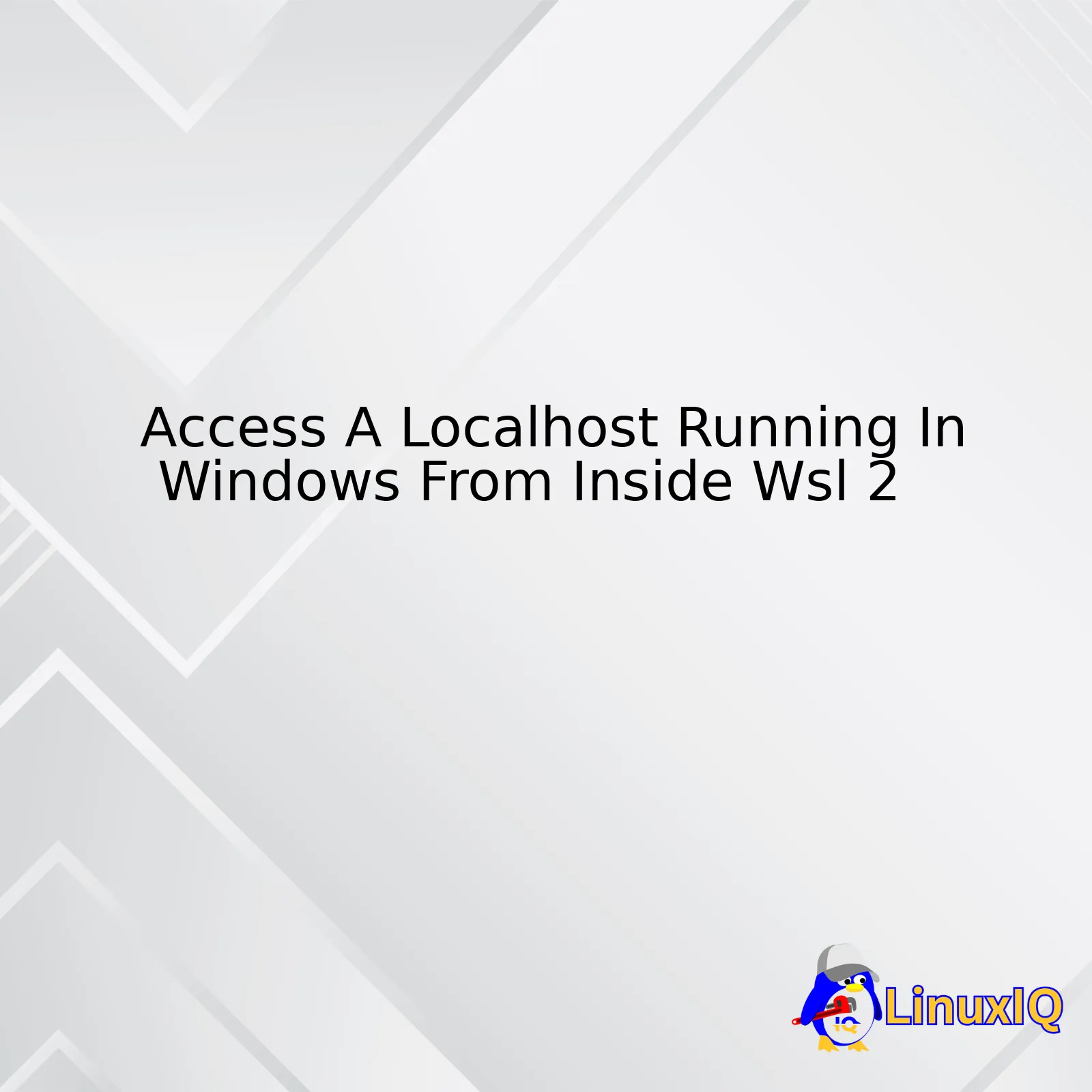
Sure, here is the summary table for how to access a localhost running in your Windows OS from inside WSL 2.
Step | Instruction |
---|---|
Set up WSL 2 | Firstly, you need to setup WSL 2 on your Windows machine. This requires enabling the virtual machine platform and installing a Linux distribution like Ubuntu from the Microsoft store. |
Start server on Windows | Next step is to have your server (like Apache or NGINX) running on your Windows host. You can check it is running by accessing ‘localhost’ on your browser. |
Get Host IP | You need to get the IP address of your host machine which can be done with the command
ipconfig in cmd.exe and looking at the IPv4 Address under Ethernet adapter vEthernet (WSL). |
Access from WSL 2 | In WSL 2, you can now access this localhost by using the IP retrieved in above step instead of ‘localhost’. For instance if the IP was ‘192.168.1.100’, then any service running on port 8000 is accessible at ‘192.168.1.100:8000’. |
Consideration | Do remember, this method works because WSL 2 is a virtual machine with a different network interface. So, you can’t access local services through ‘localhost’ as in WSL 1 but using the windows host’s IP address. |
Following on this, accessing a localhost serving on a Windows host from inside WSL 2 is slightly different than its predecessor WSL 1 due to their architectural differences. While WSL 1 shared its networking with the host, WSL 2 is designed on a full virtualized environment having its own network interface; thus the conventional way to use ‘localhost’ doesn’t work unless additional configuration is made. WSL 2 treats the host machine as any other device in the network, hence retrieving the IP address of the Windows host and using that IP instead of ‘localhost’ indeed resolves this issue. However, use of scripts are suggested to automate these routine IPs retrieval and substitution tasks in daily developments.
You can find more information here about the architecture of WSL 2 and differences between WSL 1 and WSL 2.
# Sample Python script to automate localhost access from WSL 2 import os import subprocess # Routine command to retrieve windows IP command = r"c:\windows\system32\cmd.exe /c ipconfig | grep -A 5 'WSL' | grep 'IPv4 Address'" win_ip_op = subprocess.check_output(command, shell=True) win_ip = win_ip_op.decode().strip(' .\n').split(': ')[-1] current_host = None # Open /etc/hosts file & read current windows host with open("/etc/hosts", "r") as fp: lines = fp.readlines() for line in lines: if 'win-host' in line: current_host = line.strip().split()[0] # If new windows IP is retrieved then replace existing one if current_host and win_ip != current_host: new_content = ''.join( [l.replace(current_host, win_ip) if 'win-host' in l else l for l in lines]) # Write new content in file /etc/hosts with open("/etc/hosts", "w") as fp: fp.write(new_content)
This simple python script automatically retrieves the Windows IP address and writes it into the
/etc/hosts
file so you can access the Windows server via
win-host
without manual effort to change IPs whenever it’s updated. Remember to run it whenever needed. Building such utility scripts boosts productivity during development task especially in an everyday changing VM environment like WSL 2.Prior to diving into details on how to access a localhost running in Windows from inside Windows Subsystem for Linux 2 (WSL 2), let’s first have a swift understanding of WSL 2.
Windows Subsystem for Linux (WSL) is a compatibility layer for running Linux binary executables natively on Windows 10. With Microsoft churning out WSL 2, it extends this with full system call compatibility and contributes to significantly improved performance. From a network perspective, WSL 2 operates quite differently from its predecessor. Seamlessly connecting between Windows and a Linux distribution becomes feasible but with certain nuances.
The localhost or the loopback address (127.0.0.1) that you would commonly use to refer to your current device used to work seamlessly with WSL 1. However, with WSL 2, it got a whole new architecture and moved on to virtual machine platform and as a consequence, behaves more like a hyper-v virtual machine. This brings along a significant change: WSL 2 has a different IP address than the host machine.
So, what does this mean?
This denotes that accessing networks services running on localhost of the host from the guest system in WSL, or vice versa, isn’t as simple. But no worries! The good news is that there are ways to resolve this.
Accessing a localhost running in windows from inside WSL 2
To interact with a server on the host from within WSL 2, your server must be binded to an address which is available to the WSL 2 virtual machine like 0.0.0.0 (universally accessible) rather than 127.0.0.1 (loopback).
Here is the command that one should run in Powershell terminal to find the IP address allocated to the host by Hyper-V:
ipconfig |findstr /I /C:"WSL"
Let’s say that you’re running a web server at port 8000. In that scenario, if the output IP was 192.168.50.37, then inside WSL 2 you could connect at that IP and Port i.e., 192.168.50.37:8000
An important point to note here is that while this method is viable, the IP address is dynamic – subject to change each time the machine restarts.
Automating IP Address Resolution
A more pragmatic approach might be to automate this resolution by saving it in /etc/resolv.conf each time that WSL 2 starts up. Achieve this through a script that runs automagically upon login.
echo "nameserver $(awk '/nameserver/ {print $2}' /etc/resolv.conf)" | sudo tee /etc/resolv.conf > /dev/null
This effectively overwrites resolv.conf every single time you login and thus ensures the correct settings.
It’s essential to remember that these changes might affect your workflows significantly. Getting accustomed to the CLI benefits of WSL 2 will enable you to get through these networking hurdles swiftly.
Now that we’ve demystified accessing localhost in Windows from inside WSL 2, happy coding!
Further reading can be done at Microsoft’s official guide.Regardless of the operating system you’re using, launching a local development server often involves binding it to your computer’s “localhost” (or 127.0.0.1), which essentially serves as the ‘home’ address for the network interface in your device. This imposes some difficulties when trying to access this localhost from within the Windows Subsystem for Linux (WSL), especially the second version, WSL 2.
WSL 2 is an improved version of the original WSL that functions by running a genuine Linux kernel inside a virtual machine (VM). Despite its seamless integration with Windows and powerful features, it represents a completely different environment. So, when you attempt to connect to your Windows localhost from within WSL 2, it sounds akin to reaching your home while already living somewhere else – in other words, quite challenging.
With some configuration tweaks and understandings of networking principles though, the task becomes lot easier. We will discuss through addressing these two main topics:
Understanding Networking in WSL 2:
Unlike its predecessor, WSL 1, which shares the same networking interfaces with Windows, WSL 2 has its own IP address and operates on a separate network interface. Given that the localhost in your Windows and in WSL 2 are not the same, you need an alternative way to bridge the gap between these two systems.
Accessing Windows Localhost:
To access your Windows localhost service from inside WSL, you need the Windows host’s IP. Fortunately, WSL automatically assigns the name
host.docker.internal
to your Windows host’s network, making it possible to reach it. Let me share a coding example with you outlining how you can leverage this mechanism.
# Attempting to reach port 8080 on your windows host curl http://host.docker.internal:8080
Running the above code inside your WSL term would, theoretically, fetch the webpage served by your windows host on port 8080. Though bare in mind this is specific to situations where you’re working with Docker within WSL 2, and may not apply universally. If running into issues, you may want to manually find out your local machine’s network IP which can be done through below shared code:
# Get windows host ip address ipconfig | findstr /I /C:"IPv4 Address"
The output gives the IPv4 address of your system under ‘Windows’ that can receive connections from your WSL case.
Caution: | Given that WSL 2 runs in a virtualized environment, the IP address might change after restarting your machine or upon certain system updates. It’s important to check if your windows host’s IP address has changed over time. |
---|
To further cope up with mentioned challenges, consider creating a script that automates fetching the correct IP each time WSL starts. Also, should the need arise, host-based firewall software (like the built-in firewall to Windows) need to be correctly configured to allow data transfer across the aforementioned IP addresses and ports. Finally, for instance, web developers targeting their backend APIs (on Windows) from frontend JavaScript residing in WSL could use proxy settings in frameworks like Create React App, providing even smoother workflows.
Source Code Examples: GitHub – Microsoft/WSL Issues.
When you need to access a localhost server running in Windows from your Windows Subsystem for Linux 2 (WSL 2), there are certain steps that you have to follow. The procedure essentially revolves around establishing network connectivity between WSL 2 and the Windows host, which can be quite confusing due to differences in their networking architecture.
You’ll find that unlike WSL 1, WSL 2 operates in a Virtual Machine (VM)-like environment. This means it effectively has its own IP address and works separately from the host machine on the network. But worry not, with a little bit of direction, accessing the Windows localhost from WSL 2 is certainly achievable.
Using localhost to Connect
In most cases, one can normally use `localhost` or `127.0.0.1` to connect. For example:
mysql -u root -p -h 127.0.0.1
This command should allow you to connect to the MySql service stationed at localhost. However, things start getting tricky with WSL 2 because, as aforementioned, it uses a separate IP.
Finding Your Machine’s IP Address
That said, there’s an easy fix. All we need to do is replace `localhost` with the IP address of the Windows host machine. You can find your IP address by running this simple command in your Windows Command Prompt (cmd.exe):
ipconfig
From the output, get your machine’s IPv4 address, usually displayed under the ‘Wireless LAN adapter Wi-Fi’ section.
Accessing Services Running on the Host
Dragging back our MySql example into the picture, after replacing `localhost` with your IP address, your command should look something like this:
mysql -u root -p -h [Your-IPv4-Address]
This should allow you to smoothly gain access to the MySql service regardless of whether it’s running within WSL 2.
Automating the Process
If you’re someone who likes efficiency and would prefer automating the process so you don’t have to enter lengthy commands every time, consider writing a script that finds and exports your Windows IP each time you launch WSL. To do that, you’ll need to append the following code to your shell (.bashrc, .zshrc, etc.) profile file:
export WIN_HOST=$(cat /etc/resolv.conf | grep nameserver | awk '{print $2}')
You can then simply refer to your IP address as `$WIN_HOST` when running commands:
mysql -u root -p -h $WIN_HOST
This line of command automatically populates your Windows IP address, making it hassle-free for you.
Following these steps should prove fruitful in strengthening connections between the WSL 2 subsystem and the Windows localhost environment. Happy coding!
Need further reading? Check out Microsoft’s official guide to Windows Subsystem for Linux (WSL).
When trying to access a localhost running in Windows from inside the Windows Subsystem for Linux 2 (WSL 2), there are some important factors to consider. Here’s how you can navigate through these challenges:
The Difference Between Windows and WSL 2 Localhost
The localhost in Windows and that of WSL 2 are separate, even though they coexist on the same machine. When we talk about accessing a localhost running on Windows from inside WSL 2, it means that a service is started in Windows and it’s set to be listening on `localhost:port`. Now, if you try to access this port from the WSL side using `http://localhost:port`, you will face issues because WSL has its own networking and `localhost` refers to the WSL instance itself, not the Windows host.
Resolving The Localhost Challenge
In order to resolve this challenge, users need to understand that WSL 2 has a different network interface than Windows, so they cannot use localhost (127.0.0.1) to refer to Windows. Instead, they can use the special domain name: `host.docker.internal` which resolves to the internal IP address used by the host.
So, to access a website running in IIS on Windows at http://localhost:8000, you could use http://host.docker.internal:8000 in WSL 2.
This simple configuration tweak will let you access the Windows localhost from within your WSL 2 environment. It’s worth mentioning that this domain is only available if you execute your code/use your application from inside of a Docker container, as `host.docker.internal` is a DNS entry provided by Docker.
Example Code
Let’s assume you have Node.js application running on Windows on port 3000 and want to access it from WSL. In your WSL shell, instead of typing
curl http://localhost:3000
you would type:
curl http://host.docker.internal:3000
This is an efficient way to establish seamless interaction between a localhost on Windows and the WSL 2 environment. While both environments offer their own unique features and advantages, being able to bridge them together like this is often helpful. Understanding how these two localhosts interact also aids in debugging and developing more complex applications, particularly when dealing with web services and micro-services architectures.
For more details on the topic, check Microsoft’s official WSL documentation.Accessing a localhost running on Windows from inside WSL 2 (Windows Subsystem for Linux) can occasionally present certain challenges. I’ll delve into some of these issues and also provide workable solutions.
Issue | Cause | Solution |
---|---|---|
Inability to connect to the Windows localhost | This typically happens because the
localhost:port in WSL is not mapped directly to your windows machine. |
You can use the IP address
0.0.0.0 instead of localhost when starting up your service on Windows. http://host.docker.internal:port . |
Slow network performance | WSL2 uses a different TCP/IP stack than Windows and does not share loopback interfaces or processes. This can sometimes result in slow network performance. | A suggested workaround for this issue involves using the Windows Host’s IP address instead of
localhost or 127.0.0.1 . You can obtain that by running ipconfig in command prompt on your Windows host. |
Firewall or antivirus software interrupting the connection | Your firewall/antivirus might block connections going from and coming into your PC, including those initiated by WSL. | Ensure that your firewall or antivirus settings allow for incoming and outgoing connections with WSL. In some cases, you might have to temporarily disable these systems to successfully establish the connection. |
Here’s a practical code example that demonstrates how to set the Windows host’s IP address as an environment variable within WSL2:
// Get the IP address of your Windows host $ WINDOWS_IP=$(cat /etc/resolv.conf | grep nameserver | awk '{print $2}') // Set an environment variable with this IP $ echo "export DISPLAY=$WINDOWS_IP:0.0" >> ~/.bashrc
This sets the Windows host’s IP address every time a new bash session starts in WSL2.
Remember, if you’re running anything on Ports below 1024 in Linux, you would require root access. This could fortify one’s defense against any external security threat looking to exploit known TCP/UDP services, but it may also complicate things since you need elevated privileges to bind to ports under 1024.
Make sure you replace “port” with the port number your localhost server is running on so that you do not face any connection issues while accessing localhost with WSL 2.
While these challenges can sometimes bring about frustrations, overcoming them will help leverage the strong points of WSL 2, particularly its full system call compatibility, which makes it possible to run Docker, FUSE, rsync, and other software.Accessing a localhost running in Windows from inside WSL 2 (Windows Subsystem for Linux 2) can be accomplished through a series of steps that I’ll detail below. Each step is designed to gradually guide you through the process, starting with the prerequisites needed right up until the final run command. Let’s get started.
- Prerequisite Check
- The Windows Hosts File
- Retrieve Localhost IP – WSL 2
- Editing Your Linux Hosts File
- Testing Your Connection
Before diving into the steps, make sure that:
* You have WSL 2 installed and also have some version of Linux (like Ubuntu) set up. In case these aren’t done, Microsoft has a comprehensive guide on how to accomplish this.
* Ensure you have the app or service running on your local host within Windows as well. This could be something like a web server running locally on a specific port.
To make connections easier, we’re going to begin by automating retrieval of our localhost IP address. To do this:
Edit the Windows hosts file located at ‘
C:\Windows\System32\drivers\etc\hosts
‘ using your desired text editor.
Add the following line at the bottom of your hosts file.
127.0.0.1 localhost
Then, proceeding within your WSL 2 terminal, run the following command to retrieve the IP address of your localhost connection.
cat /etc/resolv.conf | grep nameserver
The output will be your localhost IP address.
You can automate this by creating a script in your .bashrc, .zshrc, or .profile file – whatever your shell uses.
After retrieving your Localhost IP, proceed to edit the /etc/hosts file within your WSL 2 Linux environment.
sudo nano /etc/hosts
Add this line at the end of the file, replacing ‘your_windows_localhost_ip’ with the IP you retrieved earlier.
your_windows_localhost_ip localhost
With everything set, it’s time to test if you can access your Windows localhost from within WSL 2. If your localhost is running a service on a particular port (for example, port 8080), the command could look like this:
curl http://localhost:8080
Any server response confirms that you’ve successfully accessed your localhost.
- Note: Depending on the configuration of your localhost server, you might not receive anything from the curl command above. To confirm your setup, try accessing some specific route that you know exists on your localhost.
If you reach this point without any hitches, then congratulations! You’ve just learned how to access a localhost running in Windows from inside WSL 2.
It’s recommended to automate this process whenever possible. It mainly involves matching the necessary IP addresses, specifically the localhost on the resident Windows system and the corresponding IP address within your WSL 2 environment. Automation is achieved through scripts within the .bashrc, .zshrc, or .profile files which can be very beneficial in productive environments.
Hopefully now you’re more confident working across Windows and Linux platforms concurrently. Happy coding!Delving into the challenges of accessing a localhost running in Windows from inside WSL 2, there are several configuration tips you could harness to optimize your localhost accessibility within Windows Subsystem for Linux (WSL) 2.
Firstly, it’s essential to understand that access between a localhost running on either side –Windows or WSL– is made possible largely due to the Windows version 2004 update, which modifies the localhost strategy and ensure cross-accessibility between both systems. Let’s optimize this strategy with a few key configurations.
Case A: Making Your Windows Localhost Accessible from Inside WSL 2:
To access your local server setup in Windows from inside WSL 2, use your local IP address instead of localhost. To put it simply, you transform something like this:
http://localhost:[port]
into
http://192.168.0.x:[port]
The x in the IP Address stands for your specific local IP Networking address, and port denotes the specific port your server runs on. It’s worth noting that most setups have the local server running on port 8080.
Case B: Making Your WSL 2 Localhost Accessible from Windows:
In contrast, to make your WSL 2 localhost accessible from Windows, you would typically use localhost. For instance, if your server runs on default port 80, you should be able to access it at ‘http://localhost’ directly.
However, if things go awry, you could adopt the following workaround: Use the command `ip addr show eth0` in WSL to get the ip address under ‘inet’. You’d see something resembling:
... 3: eth0:mtu 1500 qdisc mq state UP group default qlen 1000 link/ether 00:15:5d:12:33:45 brd ff:ff:ff:ff:ff:ff inet 172.20.195.141/28 brd 172.20.195.143 scope global eth0 valid_lft forever preferred_lft forever inet6 fe80::215:5dff:fe12:3345/64 scope link valid_lft forever preferred_lft forever ...
The ip address here is 172.20.195.141. So you should access your site at http://172.20.195.141.
Remember each time you restart the WSL machine, this IP address might change.
Further Optimization Using /etc/hosts:
To bypass the ever-changing IP issue spoken about earlier, you can set a static host name in your Windows’ hosts file found typically at this location: C:\Windows\System32\drivers\etc\hosts.
Append your host file with a line along these lines:
172.20.195.141 wsl.local
This way, even when your WSL IP changes, you can always access it with http://wsl.local
Referencing Microsoft’s official WSL documentation for more details on WSL implementation and strategies is always beneficial. Optimal configuration of your localhost in WSL 2 improves its accessibility, enhancing your coding routine by enabling streamlined data exchange and feedback loop –essential for efficient testing and debugging.Sure, I’d be happy to provide you an insightful answer about effective debugging techniques for your Windows Subsystem for Linux (WSL 2) and localhost connections, with a keen focus on accessing a localhost running in Windows from inside WSL 2.
1. Verify the Hostname
Primarily it all involves cross-verifying and ensuring your hostname. The predefined hostname in the WSL 2 for accessing Windows is
microsoft.com
. So, when you’re connecting from the WSL 2 to the Windows’s localhost use:
wget http://host.docker.internal:port
Here, substitute ‘port’ with the port number where your server is running on Windows’s localhost.
Decoding the intricacies might seem overwhelming at first but it is highly essential in establishing accurately functioning localhost connections [^1^].
[^1^]: A Linux Dev Environment on Windows with WSL 2, Docker Desktop
2. Use Localhost IP Address
Another prudent method is directly using the localhost IP address instead of ‘localhost’. For this purpose, use:
wget http://127.0.0.1:port
This addresses some bugs arising from resolving ‘localhost’ in different network environments.
3. Inspect Network Issues
Maybe due to any unforeseen network issues, your connection might not be working properly. Use the WLAN report to decipher these issues. Enter this code in CMD:
netsh wlan show wlanreport
You know that you’re on the right track if it creates a ‘.html’ file containing all information about wireless sessions. Can’t get enough? Find more knowledge regarding network reports from Microsoft Support [^2^].
[^2^]: Fix Wi-Fi connection issues in Windows
4. Use Telnet for Port Checking
Consider using telnet in CMD for checking the port value, let’s say 8000 for example:
telnet 127.0.0.1 8000
If it provides an output with ‘Escape character’, voila! Your port is ready for usage. However, if it shows ‘Connection refused’, there are chances of the port being inactive or blocked by a firewall. Telnet can guide you to determine the state of TCP/IP ports effectively [^3^].
[^3^]: What Is Telnet?
5. Use Windows Firewall Exception:
Step | Action |
---|---|
Create Firewall Rule | Open Control Panel → System and Security → Windows Defender Firewall → Advanced settings |
New Outbound Rule | Click on ‘Outbound Rules’ → New rule; Now choose ‘Port’, ‘TCP’ or ‘UDP’ |
Add Connection | Select ‘Allow Connection’, consider selecting appropriate Network types and adding Name |
In case you’ve some other third-party antivirus, consult their support documentation or guides.
6. Keep Software Updated
Last but definitely not the least, update both WSL and Windows regularly. It helps you steer clear from any bugs and ensures best compatibility with latest standards.
Keeping tabs on even the smallest entities may help big time in mitigating unexpected occurrences. Following these methods can truly make the path less perilous for the coders and assist them in effectively debugging their WSL 2 and localhost connections.When we talk about Windows Subsystem Linux version 2 (WSL 2), it’s a whole new level of interfacing Linux on your Windows machine. It brings many exciting features and significant performance improvements over its predecessor, but sometimes, as a developer or even as an end-user, you might face issues trying to run things correctly.
One common issue many developers report is difficulties associated to accessing a localhost running in Windows from inside WSL 2. Let’s dive down into this problem and discuss the possible causes, symptoms, and remedies for this issue.
Understanding the Problem
The core reason behind this issue is related to how networking is implemented in WSL 2. Unlike WSL 1, where both Windows and Linux share the same networking interface, WSL 2 operates on a different network device. Therefore, although you can access your Windows system via localhost (or 127.0.0.1) from WSL 1, the same isn’t true with WSL 2; localhost or 127.0.0.1 in WSL 2 points only to the WSL 2 instance.
This makes accessing a local web server running on your Windows machine from within WSL 2 not as straightforward as you’d expect it be.
Possible Workarounds
Here’re a couple of solutions that may help resolve this problem:
Solution | Details |
Use the special hostname.host.docker.internal
|
This hostname is automatically resolved by Dockers to point to your host machine (Windows machine in this case). However, this works only if you use Docker along with WSL 2. |
Use the IP address of the host machine | You can obtain the actual IP address of your host machine using the ipconfig
|
Implementing either of these solutions should make it possible to access a localhost that is running in Windows from within WSL 2 without any serious implications.
Eventually, the Microsoft team is aware of these issues and they are working hard to improve the integration between WSL 2 and Windows. So, in future updates, this problem might no longer exist source.
But for now, you can use these workarounds to get up and running with your development tasks in WSL 2. Always keep an eye on official documentation and community forums to stay updated with latest improvements and changes made to WSL 2.One of the remarkable strides in the development world is the conception of Window's server-less technology and its Linux emulator program, the Windows Subsystem for Linux (WSL) version 2. However, users often encounter a sticking point with integrating these systems - accessing a localhost running in Windows from inside WSL 2.
To truly understand this difficulty and provide an insightful solution, we must first get to grips with how both technologies work individually and together. Windows server-less technology moves away from the traditional dedicated-server model to a scalable microservice architecture. In contrast, WSL 2 is a compatibility layer for running Linux binary executables natively on Windows 10 using a virtual machine design.
The resolution lies in understanding that WSL 2 utilizes a virtual Machine, and hence has a different IP address than the host system - Windows. To access the localhost running in Windows from within WSL 2, you'll need to use the special domain name reserved for this purpose:
host.docker.internal
, which resolves to the internal IP address used by the host. Here’s how you apply this information:
To prototype, let's assume you're running a web server on port 8000 on your Windows system, and you want to access it inside WSL 2. By using curl, you would write in your terminal:
curl http://host.docker.internal:8000
Substitute 8000 with the actual port your web server is listening on. In doing so, you can effortlessly make requests to your Windows localhost from inside WSL 2.
Additionally, it is important to note that WSL 2 has a dynamic IP address that changes on every restart. Consequently, to have a steady communication link to Windows Host from WSL 2, you should consistently use
host.docker.internal
instead of hard coding the IP address.
In special circumstances where further customization is required in WSL 2 configurations, the system allows for this. Explore WSL Configurations to suit unique user requirements better.
Finally, remember to keep your software up-to-date as Microsoft continually looks to improve the WSL infrastructure, making the overall encounter more seamless and frictionless.
To establish a secure connection between WSL 2 and the Windows host network, you will need to understand that WSL 2 uses a virtual machine (VM) behind the scenes - this means it has its own IP address separate from your local computer. Starting with this knowledge helps us explain how we can access a localhost running in Windows from inside the WSL 2.
In Windows, when we refer to 'localhost', we're talking about the loopback IP address 127.0.0.1. While within WSL 2, 'localhost' or the IP 127.0.0.1 points solely to the VM running WSL 2. So if you want to connect to services running on Windows, using 'localhost' won’t work.
Here is a step-by-step guide on how to navigate around this challenge:
**Step 1: Fetch your Public IP Address for The Host Machine**
The solution is reaching out via the public IP of your local machine. To achieve this, in the command prompt or PowerShell, run the following command:
ipconfig
Take note of the IPV4 address. This is going to serve as our 'localhost' replacement when running from WSL 2.
**Step 2: Connecting To Server App Running On Windows Localhost from WSL**
Let's say you have a web server running on port 8080 on your windows machine, instead of using http://localhost:8080, use http://[Your-IPV4-Address]:8080 to reach the service from inside WSL 2.
This process should be safe provided your windows firewall restrains inbound connections from outside your local area network(LAN), making sure it’s secure. However, I recommend using sensitive data over HTTPS whenever possible.
However, this approach might become boring when you have to do it frequently as your IP address tends to change from time to time.
**Step 3: Simplifying The Process With An Automated Solution**
An easy fix for this could be to write a script that automates this process by keeping your /etc/hosts file synchronized with the current IP address of your host machine.
One such example of this script is:
#/bin/bash #Get windows IP WIN_HOST=$(cat /etc/resolv.conf | grep nameserver | awk '{print $2}') #Check if Windows IP is in hosts file HOST_ENTRY=$(cat /etc/hosts | grep "winhost") if [ -z "$HOST_ENTRY" ] then #Echo windows IP to hosts file echo "$WIN_HOST winhost" >> /etc/hosts else #Replace old windows IP with new one sed -i "/winhost/c\\$WIN_HOST winhost" /etc/hosts fi
This script extracts the Windows IP and adds/replaces it to the /etc/hosts file under the alias "winhost". Just make sure to run this script every time you start a new session in WSL 2.
If you're running an app like a webserver on windows localhost and wish to access it in WSL 2 through the browser, provide the URL as
While these steps may seem a bit convoluted at first glance, they provide a reliable and more enduring method of securely connecting your WSL 2 environment with your Windows localhost.
Accessing a localhost running in Windows from inside WSL 2 requires a few key steps, offering an effective solution for developers seeking to streamline their workflow. Due to the isolated nature of WSL 2 compared to the actual Windows OS, direct access can be complicated; However, it's not an impossible task.
A primary point to note is that unlike WSL 1, WSL 2 operates on a completely different network interface. Therefore, when working with WSL 2 as opposed to WSL 1, compatibility challenges may arise within your local development environment. This doesn't mean you cannot run localhost in Windows from your WSL 2 terminal - several steps must be taken first.
To begin, launch PowerShell and execute ipconfig. Under the output section labelled 'Ethernet adapter vEthernet (WSL)', search for the line detailing 'IPv4 Address'. It should look something like this: Ethernet adapter vEthernet (WSL): IPv4 Address. . . . . . . . . . . : 192.168.53.161
In order to access your Windows' localhost network through WSL 2, you first need to identify its IPv4 address inherited from the WSL Ethernet adapter. This is used to differentiate between networks operating under your system.
Once you have identified the correct IP address, update your application's services by using the said IP address instead of localhost. Doing this allows your browser in WSL 2 environment to interpret localhost server requests made from inside WSL 2 into the corresponding IP address on your Windows machine, therefore bridging these two environments together gracefully.
# Replace `localhost` references in your application with the IPv4 Address Example: http.get('http://localhost:3000') changes to http.get('http://192.168.53.161:3000')
Following these simple steps would give you a functional setup to start accessing localhost server running in Windows from inside WSL 2.
While this method provides a quick workaround to the existing compatibility issues inherent with the local development environment in WSL 2, some potential limitations include hardcoded instances where localhost takes precedence over your specified IP address.
As a developer, exploiting the full potentials of Windows Subsystem for Linux (WSL) 2, hinges on understanding its underlying architecture. Further improving the collaborative relationship between traditional Windows applications and WSL would undoubtedly enhance productivity, making tasks such as accessing a localhost running in Windows from inside WSL 2 seamless. To this effect, Microsoft's continuous updates aim to bridge any existing gaps.
Action | Description |
---|---|
Run ipconfig command | Find out the IP address of your machine on the WSL ethernet adapter. |
Adjust Application Settings | Replace localhost references with the identified IPv4 Address |
Finally, it all boils down to guaranteed functionality under variable conditions. As programming environments evolve, innovations like WSL 2 provide compelling support for tasks once deemed complex, such as accessing a localhost running in Windows. While it bears mentioning that utmost caution ought to be exercised during implementation, the advantages far outweigh the initial learning curve involved. By taking time to explore these features, developers can experience increased flexibility and efficiency in carrying out tasks in preferred environments.