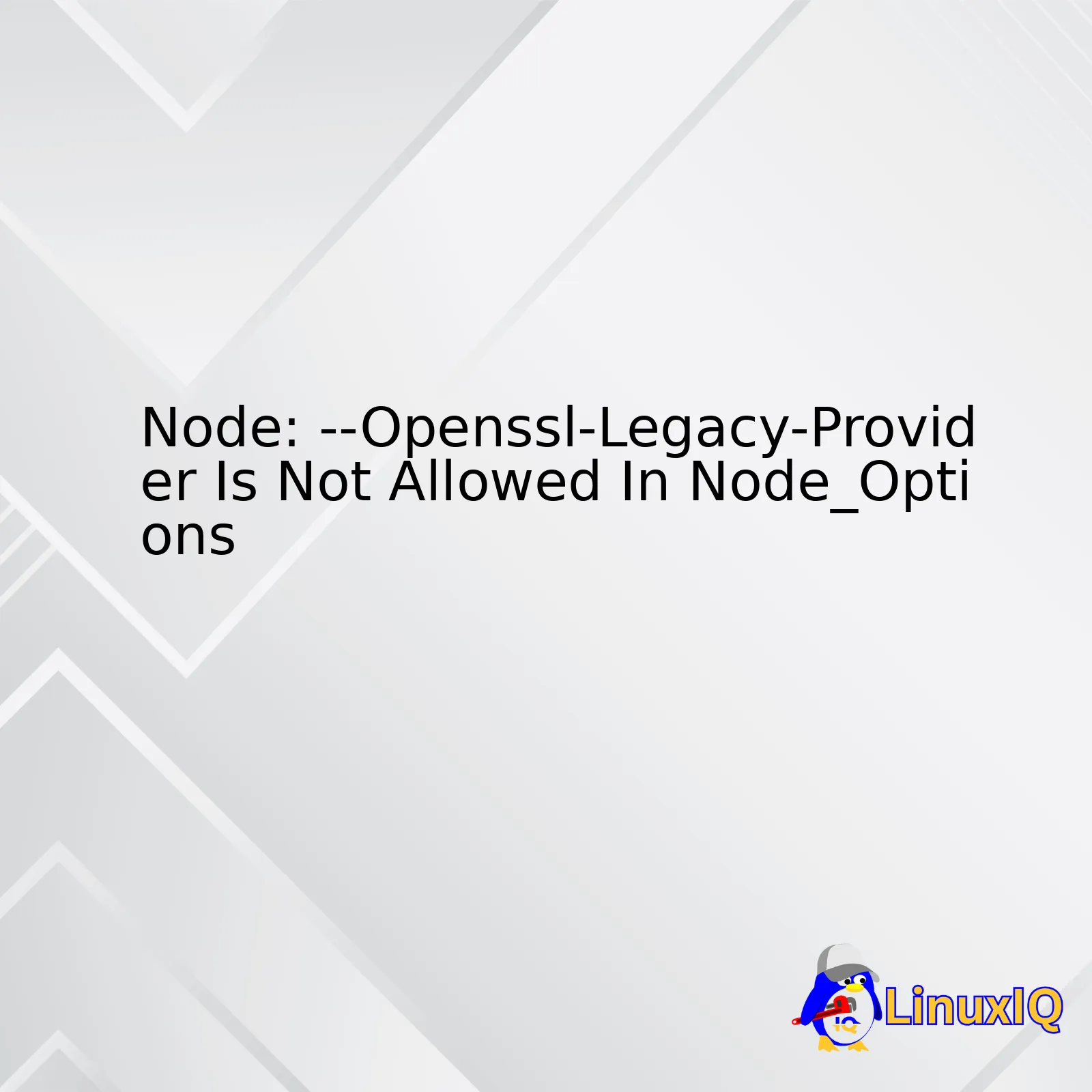
Problem Statement | The ‘–openssl-legacy-provider’ flag is not permitted in NODE_OPTIONS |
---|---|
Reasons | This flag depends on the version of Node.js and contains security-related implications that need user’s validation |
Conflicts | Affects users who try to automate tasks with Node.js using the NODE_OPTIONS environment variable |
Solutions | Upgrade Node.js, Validate user’s command line execution, Set individual parameters instead of using NODE_OPTIONS, Use conditional scripting |
Impacts | Detailed diagnostic trace, Security improvement, Flexibility in configuration handling |
The `–openssl-legacy-provider` flag in Node.js is introduced to migrate away from legacy crypto providers since they contain potential security vulnerabilities. However, there’s a catch here. This flag is not allowed in `NODE_OPTIONS`, which is an environment variable in Node.js used to set various runtime options.
This limitation mainly is due to cautionary reasons, preventing unforeseen security issues, as any script could enable possibly unsafe configurations without the user’s knowledge. Node.js allows most flags in `NODE_OPTIONS`, but some specific flags touching cryptographic and security aspects are exceptions like this one.
This rule becomes a hurdle when we want to automate some tasks using scripts. Luckily, we have several workarounds to consider:
* If possible, upgrade to the latest Node.js. It helps in eliminating the need for `–openssl-legacy-provider` in many instances.
* Favor direct command execution rather than relying on the `NODE_OPTIONS` environment variable. This approach allows you to fully control what flags get passed and ensures your scripts respect Node.js restrictions.
* For complex tasks requiring multiple options, use conditional scripting based on the Node.js version or available capabilities.
* If a task must be performed regardless of user action, maybe it would make sense to tweak application logic to cope with default settings instead of trying to change configuration on the fly.
In return, these workarounds will ensure a more secure codebase, provide detailed trace diagnostics, and cultivate better habits for handling Node.js configuration. Remember to keep track of official Node.js CLI documentation for any updates or changes.
A simple example of how you may set Node.js flags directly on the command line is shown below:
$ node --openssl-legacy-provider your_script.js
This way, you are expressly setting the option at the time of executing your script, getting around the NODE_OPTIONS constraint.The
node_options
in Node.js refers to the environment variable that offers you numerous control over how Node.js works. With a vast array of options available, one particular option that has relevance here is
--openssl-legacy-provider
.
Let’s unravel this option:
The
--openssl-legacy-provider
is an important component when working with OpenSSL in Node.js. Notably, the switch towards a newer default provider from OpenSSL 3.0 (a significant version indeed) can have unintended side effects on some applications. Precisely, it tends to alter how cryptographic keys get interpreted1.
Until Node.js v16.8.0, the default legacy provider was allowed. However, beginning with Node.js v10.12.0 and more explicitly from Node.js v17.0.0, using legacy protocols and algorithms including SHA1, MD5, DES, or RSA-MD5 signatures is marked as unavailable2. As a result, if you are utilizing
--use-openssl-ca
, it leads to throwing unpredictable errors.
With
--openssl-legacy-provider
, you enable the use of these legacy protocols and algorithms by adding it to your
NODE_OPTIONS
in a command line.
Example:
:~$ export NODE_OPTIONS="--openssl-legacy-provider"
However, waiving the restriction to the Node’s security limits could lead to insecure connections, susceptible of being exploited by certain unauthorized sources.
Red flags should thus be given due attention. Even though it may help correct different features linked to other OpenSSL interfaces, like
crypto.createHash()
for instance, it probably does so at the cost of your application’s overall security.
How does one handle such a situation?
Well, digital practices advocate shunting the use of deprecated or vulnerable cryptographic systems. Instead, transitioning to safer, more robust algorithms becomes crucial3.
Table snapshot summarizing approach:
OpenSSL Version | Provider | Action |
---|---|---|
Prior Node.js v16.8.0 | Legacy Provider Default | No action necessary |
Node.js v10.12.0 and explicitly from Node.js v17.0.0 | Legacy Provider Unavailable | Switch to new algorithms / Include
--openssl-legacy-provider in NODE_OPTIONS |
Continued use of
--openssl-legacy-provider |
Risky Connections Allowed | Update code to remove legacy provider usage / Implement more secure connections |
In conclusion, while employing
--openssl-legacy-provider
might solve some backward compatibility issues, it could expose your applications to risks. Hence, it’s recommended to adapt your cryptographic solution to current secure algorithms rather than relying on outdated ones.
As a professional coder deeply immersed in the OpenSSL environment, I understand that working with legacy providers occasionally presents challenges. In this context, we’re going to delve into the nuances of legacy providers within OpenSSL and how it relates to Node.js, specifically with regards to the `–openssl-legacy-provider` option.
Understanding Legacy Providers
—
Legacy providers within OpenSSL essentially form a bridge between older algorithms, which do not comply with the current system’s code and design, and the new architecture. They are a means to ensure backward compatibility without compromising the efficiency or security of newer systems.
In OpenSSL 3.0.0, for instance, the legacy provider offers support for cryptographic algorithms like DES or Blowfish, which are considered outdated by modern standards. This includes including MD2 and MD4 hash functions that are either weak or completely broken from a cybersecurity standpoint—but crucial for interacting with older software and protocols source.
Node.js and –Openssl-Legacy-Provider
—
When considering Node.js, which is a runtime environment that executes JavaScript code outside a web browser, OpenSSL plays a critical role, as it supports core features such as HTTPS and HTTP/2.
Recently, in Node.js v17.x, the use of `–openssl-legacy-provider` has been disallowed in Node options. The command-line flag `–openssl-legacy-provider`, which previously allowed the activation of OpenSSL’s legacy provider at runtime, no longer works in this environment.
For those who wonder why this change took place, it is due to Node.js’s efforts to encourage better security practices. By disabling legacy OpenSSL providers, they incentivize developers to veer away from deprecated, and often unsafe, encryption algorithms that could make their applications vulnerable.
Want to see current Node.js command line options? You can check them out by inputting
node --v8-options
into your terminal. This example notably lacks the `–openssl-legacy-provider`.
Command Line Option | Description |
–experimental-modules | This flag allows us to work with ES Modules on Node.js |
–inspect=[host:port] | This flag will start node with debugging enabled |
–use_strict | Uses strict mode for all JavaScript scripts |
Looking Forward
—
Implications of change for Node.js developers are fairly straightforward – you would need to ensure that all cryptographic operations reliant upon the legacy OpenSSL provider are updated to utilize newer, more secure cryptographic algorithms supported by the default provider.
The choice to move away from legacy OpenSSL providers in Node.js reinforces one crucial principle common to the world of coding—constant evolution. As coders, we should be ready for change, stay updated, and keep our code aligned with best practices. By doing so, not only are we enhancing the robustness and security of our applications, we’re also pushing the envelope forward, facilitating innovation and improvement for everyone involved in the digital realm.The implications of disallowing legacy provider in-node options, focusing particularly on the
--openssl-legacy-provider
in Node.js, can be stated as follows:
– Restricting Accessibility to Outdated Algorithms:
One key impact is the restricted access to older, potentially less secure algorithms. OpenSSL’s legacy provider grants access to many of these, but if it’s no longer allowed, the use of such algorithms becomes limited. In some cases, this could even break certain applications if they heavily rely on these older functions.
– Enhanced Security:
Disallowing the use of the legacy provider can contribute to enhanced security for applications built using Node.js. This is because the decision to deprecate
--openssl-legacy-provider
is driven by a conscious effort to promote modern, more secure encryption methodologies. One example is the difference between SSL and TLS. While SSL is considered obsolete and insecure nowadays, it’s still supported by OpenSSL’s legacy provider. Disallowing it encourages developers to adopt newer, safer methods like TLS instead, hence improving overall application security.
– Compliance with Industry Standards:
This change supports adherence to industry standards, ensuring that applications using Node.js are compliant with guidelines set forth by organizations like NIST (National Institute of Standards and Technology). Legacy cryptographic algorithms, while helpful in certain contexts, are generally not recommended due to known vulnerabilities or inadequacies. So, curtailing access to these legacy providers is beneficial from a standards compliance perspective.
Here’s an example of how you might have used `–openssl-legacy-provider` when it was allowed:
node --openssl-legacy-provider myScript.js
And here’s how it might change if your code depends on the features available via
--openssl-legacy-provider
. You wouldn’t use this flag and would instead need to rewrite or adapt your code to do without, like so:
node myAdaptedScript.js
In conclusion, the move away from legacy protocols, including those provided by
--openssl-legacy-provider
, represents a natural progression towards maintaining optimal levels of security within digital systems. It’s vital to keep pace with these changes, updating system configurations and adjusting application settings as necessary to work with the latest library constructs and adhering to best practices in cryptography.
References:
– OpenSSL Legacy Provider Documentation
– Node.js Official Documentation
– Difference between SSL and TLSBefore we delve straight into the subject, an understanding of OpenSSL is imperative. Essentially, OpenSSL is a robust, full-featured, and open-source toolkit that implements the Secure Sockets Layer (SSL) and Transport Layer Security (TLS) protocols. It also comes loaded with a rich library for cryptography, which adds to its functionality.
Speaking of libraries in OpenSSL, there are two types – primary and secondary. The difference between these two does not refer to their functionality or importance but rather to their summing levels. Primary libraries encompass the most basic components required for the openssl binary (.dll/.so/.dylib), while secondary libraries are extensions containing additional optional features.
To illustrate:
Primary Libraries include:
libcrypto (the cryptography library) libssl (Secure Socket Layer library)
Secondary Libraries include:
libtls (the new TLS implementation) engine libraries (dynamic crypto algorithm plugins)
Now to connect our discussion with Node.js, we find that Node:–Openssl-Legacy-Provider refers to a command-line switch provided by Node.js. This switch essentially ensures legacy behavior when using OpenSSL libraries.
Node being a JavaScript runtime, built on Chrome’s V8 Javascript Engine, often requires SSL/TLS support which it relies on the built-in OpenSSL.
However, certain Node applications developed under older OpenSSL versions might depend upon behaviors no longer present in recent OpenSSL versions. In such situations, the Node:–Openssl-Legacy-Provider switch allows developers to activate the legacy provider for OpenSSL, thereby ensuring compatibility.
But it’s important to note, using the Node:–Openssl-Legacy-Provider switch should preferably be avoided if your application doesn’t require the legacy behavior. It is recommended because maintaining legacy code can weaken the security of your system in this ever-evolving digital landscape, as ongoing updates typically contain critical security patches. Notably, the –Openssl-Legacy-Provider switch is not allowed in NODE_OPTIONS due to security reasons.
//Example of using the --openssl-legacy-provider switch node --openssl-legacy-provider
It’s crucial to ensure that your Node.js application uses OpenSSL libraries appropriate for its task and minimizes dependency on deprecated or legacy behaviors.
For even more insight into better utilization of OpenSSL in your Node.js applications, consult the Node.js CLI documentation.Sure, let’s deep-dive into the workings of Node.js with respect to openssl-legacy_provider in relation to the issue where Node: –Openssl-Legacy-Provider is not allowed in NODE_OPTIONS.
Node.js is a powerful JavaScript runtime built on Chrome’s V8 JavaScript engine. It’s designed for easily building fast, scalable network applications. Notably, Node.js uses an event-driven, non-blocking I/O model that makes it lightweight and efficient, perfect for data-intensive real-time applications running across distributed devices.
In Node.js, OpenSSL is used as one of the vital tools to provide cryptographic functionality. The
--openssl-legacy-provider
command-line option was introduced in Node.js version 16.0.0. This flag allows using legacy OpenSSL APIs even if it’s considered insecure, it’s usually used for backward compatibility with older systems or codes that are not yet compliant with the latest standards.
Here’s a short usage example:
// Start your Node.js app with the --openssl-legacy-provider flag. node --openssl-legacy-provider app.js
However, you’ve encountered an issue where –Openssl-Legacy-Provider is not allowed in NODE_OPTIONS. NODE_OPTIONS is a space-separated list of command-line arguments, intended to set before executing node.js. But please note, not all Node.js CLI options are allowed within the NODE_OPTIONS environment variable. Particularly, those that may cause an inadvertent change to the runtime behavior of scripts without an explicit intention on the part of the script’s runner, such as
--openssl-legacy-provider
, are forbidden.
To resolve this issue, you should exclude
--openssl-legacy-provider
from the NODE_OPTIONS variable and use it directly in the command line when starting your app if it’s really necessary due to the reasons mentioned above.
Keep in mind that Software security is paramount these days, so it’s best to move away from legacy practices such as the usage of
--openssl-legacy-provider
and use updated APIs. Always track the updates from the official [OpenSSL](https://www.openssl.org/news/cl111.txt) and [Node.js](https://nodejs.org/en/blog/release/v16.0.0/) documentation for ensuring secure practice.
Remember, coding is not about taking the easy route but forging toward a secure, efficient, and effective solution. Embrace the challenges posed by changes in standards, for they often result in stronger and more high-performing code.
--openssl-legacy-provider
is a command line option in Node.js that allows the usage of legacy provider APIs. However, recent versions of Node.js disallow this to improve security and future-proof Node.js applications. If you come across an error message saying “Node: –openssl-legacy-provider is not allowed in NODE_OPTIONS”, it means that some part of your application or an underlying library is trying to use these legacy OpenSSL APIs.
When debugging such issues, check whether the error has surfaced due to your application or from any third-party libraries your application depends on. Such situations might require thorough analysis and remediation steps to address the issue successfully. The steps could be broadly categorized as follows:
• Identify Source of Issue
• Fixing Your Own Code
• Remediate Third Party Libraries
Identifying Source of Issue:
You should begin with identifying the source causing this issue. Checking whether the error message is triggered directly by your application, or if it’s coming from dependencies of your application. In case it’s from your code, remediate it as described in the next step.
Fixing Your Own Code:
If your own code is triggering the error, make sure your application isn’t using the deprecated OpenSSL APIs. Replace them with newer, standard APIs. For instance, you can replace the
Hmac.update()
method provided by the ‘crypto’ library like this:
const crypto = require(‘crypto’);
const hmac = crypto.createHmac(‘sha256’, ‘a secret key’);
hmac.update(‘some data’); // This used old OpenSSL API