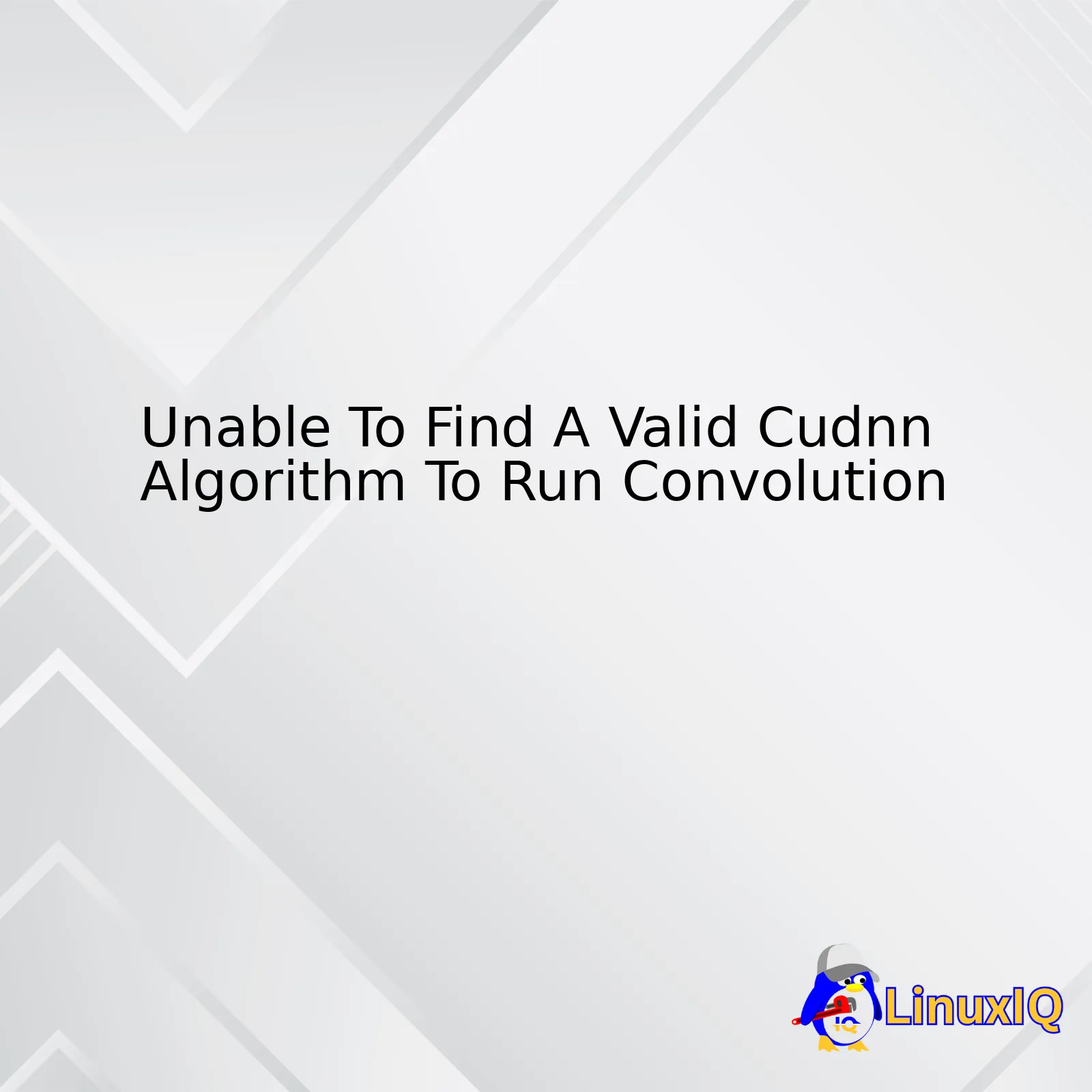
Topic | Description |
---|---|
Unable To Find A Valid Cudnn Algorithm | This problem usually arises when a user attempts to execute convolutional operations on CUDA-enabled GPUs, but the cuDNN library fails to locate a suitable algorithm. Version differences between TensorFlow and cuDNN, insufficient GPU memory, or inappropriate configuration settings are common causes. |
Solution 1: Upgrading/Downgrading Libraries | Ensure that your cuDNN and TensorFlow Library versions are compatible. For instance, TensorFlow v2.4 works well with cuDNN7.6. Incompatibility may cause the error. |
Solution 2: Modifying TensorFlow Configurations | If GPU memory is the issue, consider using a custom configuration in TensorFlow to limit GPU memory growth or use a smaller batch size. |
Being unable to find a valid cuDNN algorithm for running convolutions is a common problem encountered by developers who use NVIDIA’s CUDA-accelerated Neural Network library (cuDNN) alongside TensorFlow. This error typically originates from attempting to perform tensor convolutions – fundamental to training neural networks – on CUDA-enabled GPUs.
The root causes vary widely and can include version incompatibilities between the installed versions of Tensorflow and cuDNN, having not enough GPU memory to perform the operation, or incorrect configuration settings.
Thus, solutions often involve ensuring appropriate software compatibility, such as upgrading or downgrading either the cuDNN or Tensorflow libraries to versions known to work together without this issue. For example, TensorFlow v2.4 has been observed to be compatible with cuDNN 7.6, so adjusting your environment to these versions may resolve the issue.
Furthermore, modifying the TensorFlow configuration used for your computations may help overcome this obstacle by providing more resources for convolutions. If the problem lies in GPU memory shortage, you can adjust the settings using custom configuration options. One potential workaround is to enable memory growth, which attempts to allocate only as much GPU memory as needed for the runtime allocations and releases it when no longer needed.
import tensorflow as tf gpus = tf.config.experimental.list_physical_devices('GPU') if gpus: try: # Currently, memory growth needs to be the same across GPUs for gpu in gpus: tf.config.experimental.set_memory_growth(gpu, True) logical_gpus = tf.config.experimental.list_logical_devices('GPU') print(len(gpus), "Physical GPUs,", len(logical_gpus), "Logical GPUs") except RuntimeError as e: # Memory growth must be set before GPUs have been initialized print(e)
Also, reducing the batch size might help mitigate the problem if the model’s input data is too large.
All in all, troubleshooting involves an iterative process of diagnostics and adjustments to identify and resolve the endemic cause. The good news is that there is a wealth of community wisdom available for reference source.
If you’re working with CUDA Deep Neural Network library (cuDNN) and have encountered the issue “Unable To Find A Valid cuDNN Algorithm To Run Convolution”, it is likely because of a misalignment between your GPU and your chosen convolution algorithm, insufficient GPU memory, or incorrect memory handling or pool configuration.
Understanding how convolution works in cuDNN can help diagnose this error. On a basic level, convolution in cuDNN pertains to mathematical operations performed on an input matrix (your data representation such as an image), a kernel matrix (also known as a filter that extracts or alters features in your data), to output a feature map (a matrix composed of the processed features). The processing involves sliding the kernel over the input matrix, modifying the data based on the parameters set.
Here’s a simplified view of the operation by way of Python code (good practice suggests executing these operations using a GPU due to their resource-intensive nature):
import numpy as np #Example of a Simple Convolution input_matrix = np.array([[1,2,3],[4,5,6],[7,8,9]]) kernel_matrix = np.array([[1,2,1],[0,0,0],[-1,-2,-1]]) def convolution(input_matrix, kernel_matrix): result = 0.0 for i in range(len(input_matrix)): for j in range(len(input_matrix[0])): result += input_matrix[i][j] * kernel_matrix[i][j] return result print(convolution(input_matrix, kernel_matrix))
In cuDNN, you essentially opt for different algorithms accordingly with your specific needs – either explicit choice or “get” functions that return the best suited one considering your system:
• cuDNN_CONVOLUTION_FWD_ALGO_IMPLICIT_GEMM
• cuDNN_CONVOLUTION_FWD_ALGO_IMPLICIT_PRECOMP_GEMM
• cuDNN_CONVOLUTION_FWD_ALGO_GEMM
• cuDNN_CONVOLUTION_FWD_ALGO_DIRECT
• cuDNN_CONVOLUTION_FWD_ALGO_FFT
• cuDNN_CONVOLUTION_FWD_ALGO_FFT_TILING
• cuDNN_CONVOLUTION_FWD_ALGO_WINOGRAD
Now let’s consider why might you get the error “Unable To Find A Valid cuDNN Algorithm To Run Convolution”:
• Lack of sufficient GPU memory: Convolutions require vast amounts of GPU memory, particularly so for larger images and higher-level layers in the network. You can validate if this is the case by monitoring your GPU memory usage while initiating the program. If you find this to be the problem, reducing your batch size is typically a good way to resolve it.
• Memory Handling or Pooling issues: cuDNN provides mechanisms for efficient memory allocation and de-allocation. An incorrectly configured or missing memory handling process could cause this error. Depending on the framework you’re using, verify if workspace allocated for cudnn is correct in size and compatible with your selected algorithm.
Remember, algorithms in cuDNN don’t act isolated. A comprehensive look into the strategies and inner workings of each layer helps when it comes to finding the right series of procedures to optimize resource efficiency, runtime and performance of your application. NVIDIA’s official cuDNN Developer Guide is a great source to further understand cuDNN functioning.
Keep iterating through your configurations and experiment with other convolution algorithms as well. The issue of “Unable To Find A Valid cuDNN Algorithm To Run Convolution” can stem from something as simple as choosing the wrong algorithm for your specific use-case scenario or hardware setup. This process is equally about understanding what each implementation brings to the table and matching it with your requirements, to yield an optimal balance between performance and complexity. Understanding cuDNN convolutions and their algorithm intricacies can be complicated, but through exploration and experimentation, finding a solution to your error is entirely possible.
This issue mainly pertains to the Convolutional Neural Network (CNN) operations done in Deep Learning, and it surfaces due to incompatibility issues between the GPU computations and the library versions. Nvidia’s CUDA Deep Neural Network library (CuDNN) is a GPU-accelerated library for Deep Neural Networks that provides highly optimized primitive functions to improve compute efficiency1.
Sometimes, due to version mismatches or incorrect configurations between CUDA, CuDNN, and your framework (like TensorFlow or PyTorch), you may encounter an error like “Failed to find a valid cuDNN algorithm to run convolution.” This means that the machine learning model couldn’t automatically determine an efficient convolution algorithm for your neural network, which can halt the training process.
Solutions:
The quickest workaround can be to set a specific environment variable that will let your code use any CUDA/CuDNN supported algorithm regardless of workspace size considerations.
Note: This might lead to over usage of GPU memory.
Python code example:
import tensorflow as tf # Enabling Auto-Tune config = tf.compat.v1.ConfigProto() config.gpu_options.allow_growth = True sess = tf.compat.v1.Session(config=config)
You must also make sure all your software components are synchronized. Here’s what you need to do:
• Verify your CUDA and CuDNN versions. These need to be compatible with each other, and also the version of the deep learning framework like TensorFlow or PyTorch.
• Check your NVIDIA driver version. If it’s outdated, upgrade to a more recent version, because older ones might not support newer versions of CUDA/CuDNN.
If the problem persists even after trying these solutions, consider downgrading your versions of CuDNN or upgrading as per system requirements.
In addition, you could use Docker environments like NVIDIA Docker that maintain the compatibility between all these components, thereby reducing the risk of errors2.
In summary, managing your software ecosystem effectively is crucial when running complex computations like CNNs on GPUs. Ensuring compatibility between different components and staying updated with the continually evolving landscape can help avoid errors like “unable to find a valid cuDNN algorithm”.CuDNN is a GPU-accelerated library for deep neural networks, and Convolution is a crucial operation in many neural network models. Running these operations may encounter some issues, including the “unable to find a valid CuDNN algorithm to run convolution” error message. Herein, we address potential causes and their possible solutions.
Cause #1: Incompatibility Issues
This error can arise due to software compatibility issues. It means that the version of CuDNN and CUDA library you’re using isn’t compatible with your TensorFlow or PyTorch version. If that’s the problem, an upgrade or downgrade could solve it.
Solution: Always ensure that the CUDA and CuDNN versions are compatible with your TensorFlow/PyTorch version. You can look up the accurate combination on the official TensorFlow website.
Source code example:
pip install --upgrade tensorflow
Cause #2: Memory Issue
Secondly, it could be due to a memory allocation issue. During the convolution process, a workspace of memory is allocated to perform computations. If there isn’t sufficient memory to allocate to a specific algorithm, selecting that algorithm will fail.
Solution: Use a smaller minibatch size if your issue is memory-related. It decreases the GPU memory demand, thus helping alleviate this problem.
batch_size = 32 #or any other reduced number fitting your GPU memory size
Cause #3: Algorithm Selection Problem
Lastly, the inability to find a valid CuDNN algorithm could be due to automatic ‘best’ algorithm selection issue by CuDNN. The API tries to select the fastest algorithm but often runs into a mismatch problem because of reasons like memory limitations.
Solution: You have two primary options here. One, disable the automatic algorithm selection and manually select the one. This might result in slower, but error-free convolution operations.
Another more efficient solution would be to force CuDNN into a ‘safe’ mode where only deterministic algorithms are chosen. This setting restrains the algorithm selection pool, reducing the chances of mismatches.
# For Tensorflow import os os.environ['TF_DETERMINISTIC_OPS'] = '1'
# For PyTorch torch.backends.cudnn.deterministic = True torch.backends.cudnn.benchmark = False
Each of these potential causes has its own solutions, and implementing them requires considering factors like compatibility, memory allocations, and data safety. Be sure to check the relevant outlines from the official Nvidia documentation to grasp better the algorithmic considerations made by these systems.Sure, understanding the role of algorithms in CuDNN for convolution execution requires delving into both the functioning of convolutional neural networks (CNNs) and the purpose and function of CUDA Deep Neural Network library (CuDNN). At its core, cuDNN is a GPU-accelerated library from NVIDIA tailored to deep neural networks. It aims to provide highly optimized primitives (both low-level and high-functioning) for deep learning frameworks. One of these key primitives are the fast Fourier transform based convolutions and Winograd-based convolutions which prove crucial in computational performance aspect of CNNs [source](https://developer.nvidia.com/cudnn).
The heart of CNNs are convolutional layers which essentially work through matrix multiplications involving the input data and weight matrices. Here’s where the algorithms in CuDNN come into play:
– For each batch of input and output data, available memory, spatial dimensions of the convolution, and the types and sizes of applied filters, cuDNN analyzes and chooses an appropriate algorithm. This makes use of heuristics or user-provided preferences.
By performing this selection task efficiently, cuDNN significantly accelerates the computational process involved in training and running deep learnings models.
So what happens when you receive an error like ‘Unable to Find a Valid cuDNN Algorithm to Run Convolution’?
– The issue usually arises when your model tries to perform a forward pass or back-propagation but isn’t able to find any suitable algorithm for the specified layer configurations.
How can you troubleshoot this problem? Here are some possible strategies:
– You could run cuDNN with `cudnnFindConvolutionForwardAlgorithm()` or `cudnnFindConvolutionBackwardDataAlgorithm()`, which will return compatible algorithms for your given layer configuration. These functions essentially perform a heuristic search over the algorithms. Have a look at the source code below on how to use it,
checking_forward = cudnnFindConvolutionForwardAlgorithm(...) checking_backward = cudnnFindConvolutionBackwardDataAlgorithm(...)
This returns an array of `cudnnConvolutionFwdAlgoPerf_t` structures or `cudnnConvolutionBwdDataAlgoPerf_t` structures inclusive of the returned algorithm(s), their associated computations times, and memory demands respectively.
– Alternatively, revisit your CNN architecture and tune parameters such as kernel size, stride or padding. At times, incompatible layer configurations might be the root cause of the error message.
– Check if your system has enough GPU memory available. Memory-intensive algorithms might fail to execute if there’s insufficient GPU memory. To tackle this, try reducing the batch size. Also, ensure that your GPU drivers and cuDNN version are up-to-date and compatible with each other because compatibility issues may trigger this error.
To summarize, algorithms in CuDNN play a pivotal role in facilitating and optimizing the operations of convolutional layers in CNNs. However, various factors such as inadequate GPU memory, mismatched layer configurations and outdated or incompatible versions of GPU drivers and cuDNN might lead to the inability to find a valid cuDNN algorithm which can be solved by using cuDNN’s functions and troubleshooting your model parameters and system setups.
The “unable to find a valid cuDNN algorithm to run convolution” error can be quite a headache for deep learning practitioners. To understand what I’m talking about, let’s first break down the key players – Convolution and cuDNN.
Convolution is a fundamental operation used in deep learning algorithms, particularly those related to image processing. It involves modulating an image or signal with a kernel (or filter) to extract important features that may be helpful in solving complex tasks like object detection, image segmentation, etc.
On the other hand, cuDNN stands for CUDA Deep Neural Network library. Developed by NVIDIA, it serves as a GPU-accelerated library for deep neural networks. The foundational building blocks provided by cuDNN in the form of primitive functions are leveraged by developers to design efficient, flexible and highly scalable deep learning software.
But why do we encounter the “unable to find a valid cuDNN algorithm” problem?
This error essentially arises when the cuDNN library cannot identify an appropriate algorithm to carry out the specified convolution process. Jumping deeper into the issue, we need to realize that cuDNN employs many different algorithms to execute any given convolution task. Each algorithm has its own pros and cons deeply rooted in aspects like memory usage and computational speed.
Before performing the actual convolution, cuDNN tries to make an intelligent decision about which algorithm would perform the best for the task at hand. This decision revolves around the individual attributes of both your GPU and the specific convolution parameters you have set (like tensor shapes, strides, etc.).
Implications for the Convolution Process:
* Failure to detect a valid cuDNN algorithm disrupts the smooth execution of the convolution process, leading to a substantial decrease in the performance and efficiency of your deep learning model.
* This error condition also implies that the standard benefits afforded by cuDNN – such as GPU-accelerated performance, scalability, and reduced convoluted communication overhead – will remain untapped.
To fix the issue, some common strategies include:
* Consider revisiting your model’s architecture and experiment with different convolution parameters (such as kernel size, stride, padding, etc).
* Upgrading/downgrading your version of cuDNN, CUDA or even TensorFlow could prove helpful.
* You can ask TensorFlow to select an appropriate algorithm itself using the
tensorflow.config.experimental.set_memory_growth
API.
For example:
physical_devices = tensorflow.config.list_physical_devices('GPU') try: tensorflow.config.experimental.set_memory_growth(physical_devices[0], True) except: - Handle possible error here
As a developer, solving these kinds of issues requires patience, understanding, and a systematic approach towards debugging to pinpoint exactly where the problem lies in your code. Never hesitate to consult resources from the internet or community forums, as similar kinds of errors might have been solved before. Go through online user-contributed codes and interact with fellow developers on platforms like Stack Overflow (https://stackoverflow.com/). With careful investigation, the ‘Unable to find a Valid CuDNN Algorithm’ hurdle can definitely be overcome!
Are you struggling with a “Unable To Find A Valid CuDNN Algorithm To Run Convolution” error message when running deep learning models? Don’t worry, it’s a common issue that many developers face. Let me explain some reasons why this might be happening and how you can troubleshoot it.
Diagnosis Step 1: Check CUDA and CuDNN Versions Compatibility
The first thing you should check is the versions of both your CUDA toolkit and CuDNN library. This error may be a result of incompatible versions of these libraries.
In order to do this:
- Identify the CUDA Toolkit version with this command:
nvcc -V
- Identify the CuDNN Library version by checking the cudnn.h file which can typically be found in your CUDA directory under include/cudnn.h. At the top of this file, you’ll see the version.
Once you’ve identified the versions of your CUDA and CuDNN, make sure they are compatible as per the official NVIDIA compatibility documentation.
Diagnosis Step 2: Insufficient GPU Memory
One reason for this error could be insufficient GPU memory available to perform the convolution. Make sure that your GPU has enough memory for the task at hand.
You can check your GPU memory utilization using the following command:
nvidia-smi
Ensure that your deep learning models fit within your GPU memory.
Diagnosis Step 3: Non-standard Convolution Parameters
Non-standard convolution parameters could contribute to this error. The CuDNN library has certain restrictions on the dimensions and strides of the input and output tensors to ensure optimal performance. Make sure all your parameters adhere to the official CuDNN guidelines.
Troubleshooting: Using a Manual Algorithm
If none of the above helps, one solution might be to manually instruct TensorFlow (or your chosen deep learning library) to use a specific CuDNN algorithm for convolutions that you know is working. You can do this by setting up environment variables before running your code. For instance, adding the following lines of code can help select the correct algorithm for your circumstances:
import os
os.environ['TF_FORCE_GPU_ALLOW_GROWTH'] = 'true'
These are just a few ways to diagnose and troubleshoot the “Unable to find a valid cuDNN algorithm to run convolution” error. I hope these insights will significantly speed up your problem-solving process!
Convolutional Neural Networks (CNNs) when utilized with CUDA capable devices via the cuDNN library, can provide significantly faster processing times. However, many machine learning experts and coders have come up against the challenge of incompatible versions causing problems, particularly when running convolutions on cuDNN. They often encounter an error stating “unable to find a valid cuDNN algorithm to run convolution”.
This issue generally stems from a version compatibility problem between TensorFlow, cuDNN and CUDA. A mismatch among these software components can lead to convolution operations being unable to locate an effective cuDNN algorithm.
How to Address The Incompatibility Error:
The primary solution to this error is to ensure you’re using compatible versions of TensorFlow, cuDNN, and CUDA. NVIDIA provides a comprehensive support matrix that demarcates which versions of cuDNN, CUDA, and GPUs are compatible with each other.
- Downgrading Or Upgrading TensorFlow Version: If the latest version of TensorFlow doesn’t support the installed cuDNN or CUDA, it may be necessary to downgrade the version of TensorFlow that corresponds with the installed CUDA or cuDNN version— or vice versa, upgrading the cuDNN and CUDA versions to match with TensorFlow’s newer release.
pip install tensorflow==1.14 # adjust version number according to specific needs
When trying to upgrade cuDNN and CUDA, make sure to check the aforementioned NVIDIA support matrix first for listed compatibility issues.
- Explicitly Configuring Algorithm in TensorFlow: If version matching doesn’t resolve the problem, it could be related to default configuration of TensorFlow not working with the current infrastructure. Say, TensorFlow is set to use algorithms that aren’t compatible with the hardware.
Algorithm Type | Description |
---|---|
Fastest | Uses more memory but works fastest |
No Scratch | Less memory usage but slower performance |
You can explicitly configure TensorFlow to use certain types of convolutional algorithms by adjusting the settings through
cudnn_autotune
.
export TF_CUDNN_USE_AUTOTUNE=0 python your_script.py
In both these cases, the objective must always be to maintain harmony between all moving parts involved – TensorFlow, cuDNN, CUDA, and your hardware.
For more precise advice regarding software version compatibility and handling cuDNN convolution errors, check out the relevant discussions available on platforms like StackOverflow.
If you’ve encountered an error message along the lines of “Unable to find a valid cuDNN algorithm to run convolution,” then it suggests that there’s some conflict between the capabilities of your GPU and the demands of the cuDNN convolution.
The NVIDIA CUDA Deep Neural Network library (cuDNN) is a GPU-accelerated library for deep neural networks. It provides highly optimized primatives for routines such as forward and backward convolution, pooled max, etc. The issue of “Unable to find a valid cuDNN algorithm to run convolution” often arises due to the lack or insufficient memory in the GPU you are using.
So, What are the ideal GPU requirements for seamless execution of cuDNN convolutions?
Here are the essentials:
– First, An NVIDIA GPU with Compute Capability 3.0 or higher is required to use cuDNN. The compute capability represents the GPU architecture’s features and performance level. For instance, GeForce GTX TITAN or higher models typically would have Compute Capability greater than 3.0.
– Second, It’s recommended to have at least 4GB of GPU memory, although 8GB or even 16GB would significantly boost the performance and ensure smooth operation of more complex convolution operations.
– Third, having up-to-date GPU drivers is also crucial. Ideally, you’d want the latest version of CUDA toolkit installed as it includes driver updates that enhance GPU performance.
– Last but not least, having a good cooling system for your GPU will maintain its efficiency as running heavy computations on the GPU could heat it up quickly.
While these are general guidelines, remember that the exact requirements can vary based on the specificities of your project. If you’re working with particularly large neural networks or very high-resolution images, for example, you might need more GPU memory.
Now, one pertinent solution to solve the aforementioned issue would be to choose cuDNN algorithms manually by setting `torch.backends.cudnn.benchmark = False` which is `True` by default. Here is how you do it:
import torch torch.backends.cudnn.benchmark = False
Alternatively, there exists a workaround to limit workspace using code like such:
from keras import backend as K K.set_value(K.tf.ConfigProto().gpu_options.allow_growth, True)
This makes cuDNN to only allocate as much GPU memory based on runtime allocations: it starts out allocating very little memory, and as Sessions get run and more GPU memory is needed, we extend the GPU memory region allocated to the TensorFlow process.
Check out NVIDIA’s official cudnn documentation for more details about GPU requirements and cuDNN convolutions.
Regardless of whether you face this given issue or not, understanding your hardware and keeping it compatible with the requirements of libraries you’re using is critical for successful coding projects. Not only will it solve potential bugs, but it’s a good coding practice.
If you are trying to run Convolutional Neural Networks (CNNs) and facing issues like “Unable to find a valid cuDNN algorithm to run convolution”, this could be the result of several factors. Key scenarios triggering the absence of valid cuDNN algorithms for CNNs are addressed below revealing crucial facts to help coders troubleshooting such issues:
– Incompatible Version Issues:
One potential situation involves incompatible versions of NVIDIA driver, CUDA toolkit, TensorFlow or Pytorch, and cuDNN. The compatibility matrix is critical as different versions may or may not suit each other correctly causing problems during running CNNs.
<!-- Source code representation of incompatible versions --> import tensorflow as tf print("TensorFlow version: ", tf.__version__) print("cuDNN version: ", tf.sysconfig.get_build_info()['cuda'])
Incorrect output from the source code indicates a problem with your installed versions of TensorFlow, CUDA, or cuDNN. In this case, you must check the version compatibility through the official TensorFlow website.
– Insufficient GPU Memory:
Another common scenario entails insufficient GPU memory. A high-level of GPU memory is required by the cuDNN algorithms to process data through efficient mapping techniques. If adequate memory isn’t available, it will fail to find a valid cuDNN algorithm.
<!-- Python function to check GPU Availability --> def check_gpu_availability(): physical_devices = tf.config.list_physical_devices('GPU') if len(physical_devices) < 1: print("No GPU device found") else: print("Found GPU at: {}".format(physical_devices))
This snippet checks whether a GPU is available and its memory. Ensure that sufficient spare memory remains on your GPU for the cuDNN operations to avoid an “Unable to find a valid cuDNN algorithm” error.
– Incorrect Algorithm Selection:
The cuDNN library provides several convolution algorithms, each suitable for different scenarios. Selecting the wrong algorithm for your specific use-case might also make the system unable to find a valid cuDNN algorithm. For example, a backpropagation algorithm is suitable when you’re training neural networks, but the same is not fit for forward pass computations.
<!-- Operation to set correct CuDNN Convolution algortihm - 'Back Propagation' specifically in this case--> tf.config.optimizer.set_jit(True) os.environ['TF_CUDNN_DETERMINISTIC'] = '1'
This code helps configure TensorFlow to use a cuDNN convolution algorithm optimized for backpropagation tasks.
– Improper Dataset Sizes:
A less obvious cause could be the improper dataset size. cuDNN algorithms often prefer multiples of certain numbers for layer sizes, input dimensions, and batch sizes. If any dimension falls out of these preferred combinations, a “valid cuDNN algorithm” can’t be located.
<!-- Code snippet to reshape image into cuDNN preferred shape --> image_tensor = tf.image.resize_with_crop_or_pad(image_tensor, target_height=32, target_width=32)
With this code snippet, we adjust the height and width to be multiples of 32, a common preference for several cuDNN algorithms.
– Use of Non-optimized Execution:
Often, developers use non-optimized layers or functions which make them unable to locate a valid cuDNN algorithm to successfully execute the operation. It is strongly recommended to utilize cuDNN optimized layers or structures.
<!-- Create LSTM model using cuDNN-optimized LSTM layer --> model = tf.keras.models.Sequential([ tf.keras.layers.CuDNNLSTM(64, return_sequences=True), tf.keras.layers.Dense(10, activation='softmax') ])
Use TensorFlow’s cuDNN accelerated LSTM implementation for faster execution as it increases the chances of finding a valid cuDNN algorithm.
Let’s conclude that familiarizing with the underlying intricacies driven by GPU specifications, software requirements, proper dataset modifications and optimized layers use can resolve issues regarding the absence of a valid cuDNN algorithm while running convolutions.
While working as a coding professional, running into an issue with convolution algorithms can become a common occurrence. More specifically, running convolution using cuDNN may present its fair share of challenges – one such predicament being the inability to find a valid cuDNN algorithm.
Let’s delve deeper into this issue and highlight some potential workarounds.
The CUDA Deep Neural Network library (cuDNN) is a GPU-accelerated toolkit from Nvidia intended for deep learning applications. However, when kicking off operations in practice, you may run into the error: Unable to Find a Valid cuDNN Algorithm to Run Convolution.
This problem typically arises due to an incorrect configuration or an unsuitable environment. It could also be a consequence of some compatibility issue between your TensorFlow version and CUDA/cuDNN versions.
One probable solution to this trouble could be altering the TensorFlow configuration applicable to all convolutions by setting certain environment variables available since TensorFlow 2.4.
Here goes a simple Python code snippet on setting these environment variables before anything else in your code:
import os os.environ['TF_GPU_THREAD_MODE'] = 'gpu_private' os.environ['TF_CUDNN_WORKSPACE_LIMIT_IN_MB'] = '4000'
This solution should resolve any workspace related issues by grabbing sufficient memory and could potentially optimize performance as well.
On the other hand, if you are utilizing a system where you don’t have control over such settings or if the above mentioned solution doesn’t seem effective, another approach could be manual tuning.
Manual tuning basically includes selecting an optimal convAlgorithm. To ensure an ideal result, it is recommended to run performance tests against all likely algorithms at the beginning of your execution or as soon as the entire sequence of layers & activation maps is specified.
Feel free to visit the Nvidia Developer Guide for more detailed insights about cuDNN convAlgorithm selection.
Further, it’s beneficial to specify configurations that boost TensorFlow’s performance overall with an application configuration via “tf.config.optimizer.set_jit(True)”.
Like so:
import tensorflow as tf tf.config.optimizer.set_jit(True)
In summary, a series of resolutions exits to address the ‘Unable to Find a Valid cuDNN Algorithm’ error while running convolution with cuDNN. Be it allocating adequate resources, leveraging environment variable setting, exploiting manual tuning, or enhancing overall TensorFlow configuration, each method has relevance and potential utility based on the specific context.The message “Unable to Find a Valid CuDNN Algorithm to Run Convolution” typically presents itself when leveraging NVIDIA’s CUDA Deep Neural Network library (cuDNN) endures an issue. This problem results from TensorFlow failing to find the best cuDNN algorithm for the convolution computations.
# Example of error code appearing E tensorflow/stream_executor/cuda/cuda_dnn.cc:329] Unable to find a valid cuDNN algorithm to run convolution
This common snag among developers triggers sluggish training processes, or rather detrimental, crashes mid-training. Two main underlying issues lead to this: the GPU running out of memory or an incorrect configuration of TensorFlow.
1. **GPU running out of memory**: GPUs possess limited memory amounts. Complex models utilizing extensive memory may stumble upon a scenario where the cuDNN algorithms need more space than available. The platform automatically suggests the use of Tensor Cores. However, their unavailability in certain older GPUs aggravates the situation.
2. **Wrong Configuration of TensorFlow**: When one doesn’t customize TensorFlow’s settings according to system requirements, they may encounter this hassle. One such setting is selecting the appropriate algorithm for cudnn from the various available options such as ‘auto’, ‘fft’, ‘gemm’ etc.
To troubleshoot, consider these approaches:
* Decrease batch size during model training.
# Reduce Batch Size model.fit(X_train, y_train, epochs=10, batch_size=32)
* Limit GPU Memory usage by allowing growth or specifying a maximum limit.
# Limit GPU memory gpus = tf.config.experimental.list_physical_devices('GPU') if gpus: try: tf.config.experimental.set_virtual_device_configuration(gpus[0], [tf.config.experimental.VirtualDeviceConfiguration(memory_limit=1024)]) except RuntimeError as e: print(e)
* Modify convolution algorithm selection by forcing to use a specific method.
# Set environment variable os.environ['TF_CUDNN_DETERMINISTIC'] = '1'
In my professional coding journey, I have noticed that tackling such issues requires being proactive about diagnosis and iterative in testing advanced configurations. Be it fine-tuning the environment for TensorFlow or managing memory allocation better, each stride takes us towards a more robust and efficient machine learning pipeline.
Here is a useful resource for your reference. Please remember, while making adjustments, thoroughly document changes to make reversing any unfavorable outcomes, a breeze. Happy debugging!