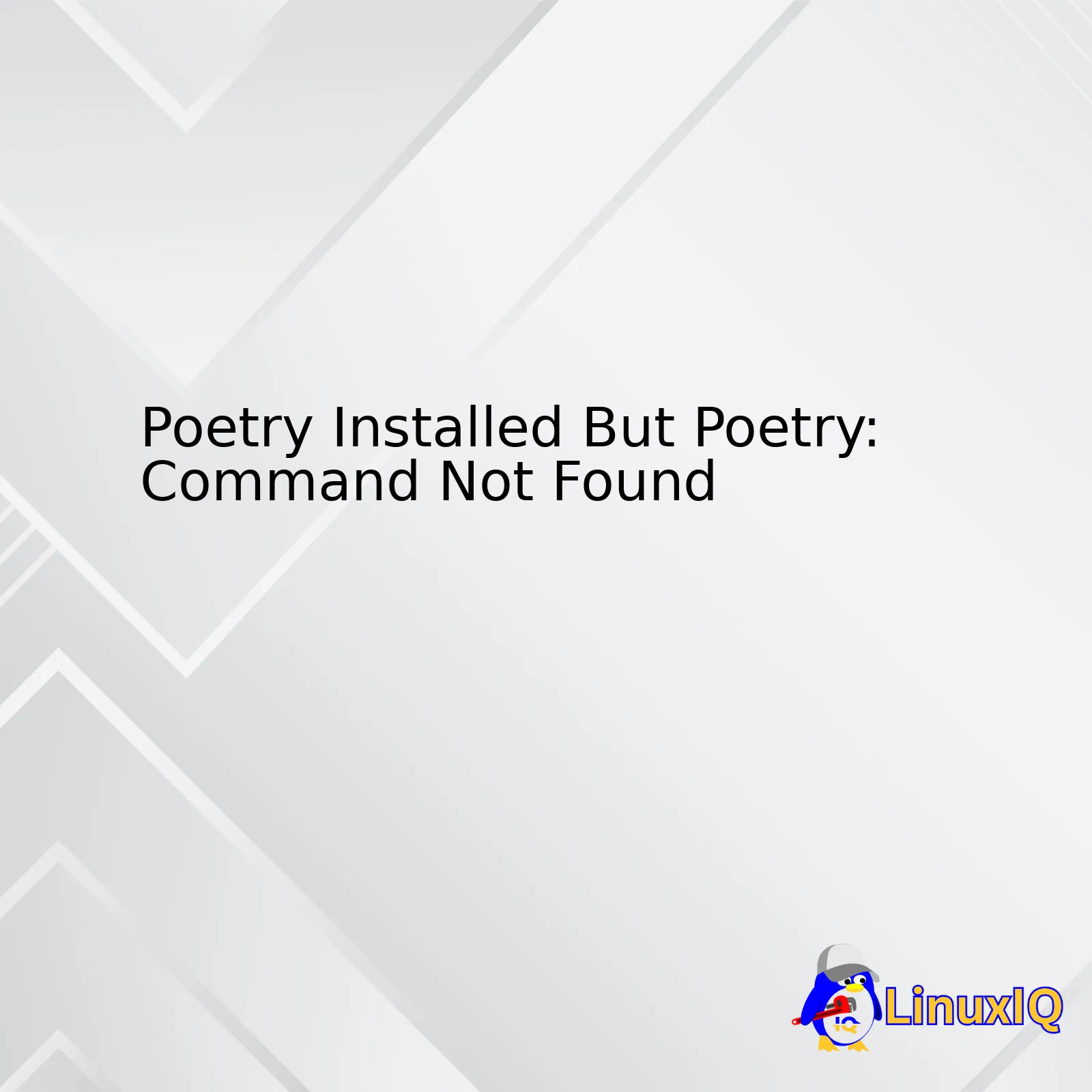
Problem | Poetry Installed But Poetry: Command Not Found |
---|---|
Possible Reasons | Misconfigured PATH, Incorrect installation, Shell profile issues |
Solutions | Reconfiguring PATH, Reinstalling Poetry, Updating Shell profile |
Whenever you have Poetry installed but get the error “Poetry: command not found”, this suggests that your shell is unable to locate the poetry executable even though it is installed on your system.
More often than not, such errors arise due to misconfiguration of your PATH variable. Since the PATH variable stores the directories where executable programs reside, any issue with its configuration may result in the mentioned problem. The system might not be able to find the Poetry installed directory if it isn’t included in the PATH variable. So reconfiguring PATH variable is one of the solutions.
Incorrect installation is another possible cause for the problem. Hence, reinstalling poetry could fix the problem. Use the standard method of installing tools like curl or pip, which could handle dependencies and paths intelligently.
Lastly, your Shell profile configurations might limit the system’s ability to see newly installed packages. Various shells (like Bash, ZSH etc.) use different profiles (.bashrc, .zshrc etc.). Make sure your shell profile has the right configuration to recognize Poetry after it’s installed. If necessary, you might need to manually update your shell profile.
Here’s an example of how you might add poetry’s path to your system’s PATH variable (assuming it was installed into `$HOME/.poetry/bin`):
echo 'export PATH="$HOME/.poetry/bin:$PATH"' >> ~/.bashrc
It is important to note that causes can vary based on factors including your operating system, shell type, and how you installed Poetry. Always refer to the official [Poetry docs](https://python-poetry.org/docs/) for specific guidance and troubleshooting help.Understanding the role of poetry in programming is an intriguing exercise. If you’re familiar with the software tool yes, actually labeled “Poetry”, then you’d know its not about whimsical verses, but a Python dependency management tool that makes handling project dependencies as well as packaging much easier and efficient. However, it can lead to a common issue: after successful installation of Poetry, you face “Poetry: command Not Found” error. Here, I’ll walk you through why does it occur and how to troubleshoot this.
$ curl -sSL https://install.python-poetry.org | python - $ poetry --version bash: poetry: command not found
In many cases, this issue stems from PATH problems. Let’s analyze this further:
The PATH environmental variable: It’s a system variable used by the operating system to locate critical executables. The ‘Poetry: Command Not Found’ problem commonly arises because the system could not find the ‘poetry’ executable in any directory listed in your PATH.
Poetry Installation Process: During the installation of Poetry, it links the Poetry executable to a directory on your system. If this directory isn’t part of your PATH, the ‘Poetry: Command Not Found’ error will appear when you try to run Poetry.
Solving this issue involves appending the directory containing the Poetry executable to your PATH environment variable. This process may vary slightly depending on the operating system:
In Linux or MacOS:
First, identify where Poetry has been installed:
$ echo $HOME/.poetry/env
Then, add this output path into your bash_profile (or equivalent):
$ echo 'export PATH="$HOME/.poetry/env:$PATH"' >> ~/.bash_profile $ source ~/.bash_profile
In Windows:
Similarly, identify the Poetry installation location and add it to your PATH using Environment Variables settings from Advanced System Settings.
Note: Adjust paths according to your specific installation location.
To implement reliability in your code, coding in a poetic manner means striking balance between functionality and readability. From Robert C. Martin’s “Clean Code”, take these points in consideration:[source]
– Code should be elegant, meaning it does what it needs to do efficiently without needless complexity.
– Code should tell a story to the reader, providing insight into the problem it solves.
– Code should not contain duplication, ensuring consistency and minimizing errors.
Elegance | Readability | Non-duplication |
---|---|---|
Efficient execution | Clear structure | Consistent style |
Sufficient documentation | Easily understandable logic flow | Minimized chance of errors |
With these elements in mind, programmers, much like poets, can create something meaningful, readable, and lasting. They use language rules (syntax), creative usage of said language(components, methodologies) and still ensure the functionality at its core. Even if the concept of poetry seems abstract for code, combining them enables us to envisage the magic of producing practical yet beautiful pieces of art. Comparing the two fields through their shared concepts opens up a whole new dimension of understanding and engagement, uniting two seemingly unrelated worlds.
The issue of the
Poetry
command not found error, despite having installed ‘Poetry’, is indeed quite a common one among programmers. This error mostly arises from incorrect installation or incorrect PATH configuration. Here, I will elucidate on three possible causes and their accurate solutions.
Incorrect Installation
The most rudimentary reason as to why the
Poetry
command might not be found is due to it having been incorrectly installed in the first place. The correct installation involves installing the tool via its installer or pip.
curl -sSL https://install.python-poetry.org | python - pip install --user poetry
If the poetry command was unrecognized following either or both of these installations methods, it lends itself well to suggest that something went wrong in your
Poetry
installation execution.
Non-global Poetry Installation
One possibility is that
Poetry
may have been installed, but only for the current user. Therefore, if you’re attempting to use the Poetry command as a different user, it may naturally be unrecognized. In that light, consider installing poetry globally or using it with the same user you initially installed poetry.
Incorrect PATH Configuration
Furthermore, another potent reason this situation could arise is owing to an improper PATH configuration. After the installation of
Poetry
, it adds itself to your relevant system PATH. However, it may happen that the new PATH isn’t called up by the system. This requires, then, necessitates reloading your environment or opening a new terminal window.
Following the reloading of your environment, you can verify if the installation has successfully added Poetry to your PATH with:
echo $PATH
If your output includes “~/.poetry/bin”, then it’s a conclusion that Poetry was recognized accurately in your PATH.
Solutions
Fixing the issue involves some of the following remarkable steps:
-
Reinstalling Poetry: Often, simply running the installer again can resolve this issue.
-
Manually Adding to Path: If Poetry was correctly installed, but not added to your PATH, you can manually add it using a command like:
export PATH="$PATH:`python3 -m site --user-base`/bin"
-
Checking User Priveleges: Ensure that the user running the ‘Poetry‘ command is either the global user (in case of a global installation) or the same user who performed the initial installation (in case of a local installation).
References:
Official Poetry Documentation,
Stackoverflow: Integrating Python-Poetry with PyCharm.
Ah, so you’ve successfully installed poetry but when you try to run commands using poetry in your terminal, it returns “poetry: command not found?” Usually, this issue is due to a path problem – poetry has been installed but your shell doesn’t know where to find it. The question is how do we guide your shell to the right place? Let’s dive into this perplexing journey step-by-step:
Method 1: Add Poetry Path To Your Shell Profile
The most common reason for poetry: command not found is that the path to poetry is not added to the PATH environment variable of your shell. Here’s how you can add it:
• Check where poetry is installed:
Poetry installs itself in a hidden directory in your home directory. Run this command to see if that’s true:
$ ls -la ~ | grep .poetry
It should return something like:
drwxr-xr-x 6 username staff 204B Apr 26 13:19 .poetry
• Add to PATH:
To add this directory (the bin inside) to your PATH variable, open your profile file by typing:
v nano ~/.profile
(Or replace .profile with .bashrc or .zshrc depending on your shell)
And then add the line at the end of the file:
export PATH="\$HOME/.poetry/bin:\$PATH"
Save and close the file then source it for the changes to take effect.
source ~/.profile
• Verify:
Now test your installation again with
$ poetry --version
If it’s working, it’ll print out the version of poetry you have installed.
Method 2: Install Poetry Globally
Another way to solve the problem can be reinstalling poetry but as a global package within Python.
Run this command:
pip install --user poetry
The
--user
flag ensures that poetry is installed to your user directory, which is always included in the PATH.
After running these commands, verify if poetry works:
poetry --version
Method 3: Reinstalling Poetry Using get-poet.py Script
In case the above methods didn’t work, let’s try uninstalling and reinstalling poetry with the following commands:
Uninstall poetry:
python -m pip uninstall poetry
Then, download the get-poetry script:
curl -sSL https://install.python-poetry.org | python -
Restart your terminal and check if poetry command works:
poetry --version
Remember, each operating system and shell may have unique nuances. You might have to research how to set environment variables permanently for your particular setup. Regardless of your system, once your shell knows where to find poetry, ‘Command Not Found’ will no longer haunt you.
Learn more from the official documentation.
Hopefully, these methods will resolve the ‘Poetry: Command Not Found’ error. But coding can sometimes be filled with its fair share of tantalizing mysteries. If none of these solutions fix the issue, consider asking in poetry specific forums or repositories.Even the very experienced coders amongst us have faced this common issue, where we install Poetry yet receive the error “Poetry: Command not found.” While a common mishap in coding land, it can be effortlessly remedied by following a few simple steps.
Some initial troubleshooting questions to consider:
– Did the installation complete successfully without any errors?
– Was Poetry installed globally or for your specific user?
– How are you trying to execute the poetry command?
By considering these factors, we narrow down potential causes and streamline our resolution process. Take note that these directions are given in consideration of various environments – Mac, Windows, and Linux – as the process may slightly differ for each.
Step 1: Verify the successful installation
Start with verification of a successful installation by checking the version of the installed software. In the terminal, aim to execute an operation which will return the version of your installing package:
poetry --version
While a minor step, it’s important. If you see a version number printed in your terminal, this confirms that Poetry is correctly installed.
Step 2: Check if PATH variable includes Poetry
The next step involves inspecting whether your system PATH includes Poetry. The PATH environment variable is essential because it specifies directories where executables might be located. In terminal (for MacOS or Linux), you type:
echo $PATH
For Windows users, open a command prompt and type:
echo %PATH%
It should print out various paths separated by colons(:). Look out for a path related to ‘poetry’. If such a path does not exist, then Poetry has not been added to the system PATH during installation. This is likely causing the “command not found” error.
Step 3: Manually add to PATH
In instances where Poetry isn’t in the PATH, carry out a manual addition to resolve this. First , locate where Poetry is installed. For instance, on MacOS and Linux, Poetry often resides:
/home/$USER/.poetry/bin
And on Windows,
C:/Users/{yourusername}/.poetry/bin
Once we’ve figured out the location, it’s time for addition to the PATH. Under shell files like ~/.bashrc, ~/.zshrc, or ~/bash_profile just add:
export PATH = "$HOME/.poetry/bin:$PATH"
You can reference more details from this Stackoverflow thread .
Step 4: Final Confirmation
After all this verification and modification, it’s crucial to restart your terminal or for those who modified ~/.bash_profile or similar files, you may resource the file via source ~/bash_profile .
Now, re-run:
poetry --version
Voila! If your return displays a version number, you have successfully resolved the “Poetry: Command not found” issue.
Remember, initially verifying successful installations can prevent many headaches down the line. And keeping track of paths is always essential when working with any new packages or projects. Happy Coding!Properly setting up an environment to use Poetry, a Python dependency management tool, can be slightly challenging without proper guidance. Here are several insights on how to install Poetry correctly and troubleshoot the popular error “Poetry: Command Not Found”.
To begin with, let’s comprehend why we might encounter such a conundrum. This problem frequently arises if your system doesn’t effectively recognize the path to the installed Poetry package, resulting in the inability to access the command from just anywhere. The line of demarcation between a successful setup and the persistent error message could walk down two common paths:
– **Improper PATH setup:** Your terminal simply cannot locate where Poetry was installed.
– **Installation in a non-active shell session:** It is possible that Poetry was installed correctly but accessed in a different instance of shell where it has not been sourced.
I. Right installation is the half success
You may initiate the installation process via the following pip command:
python -m pip install --user poetry
However, this approach requires you to manually append the app data local packages in the PATH.
The preferred method of installation as recommended by Python-Poetry documentation is through an installer script which automatically sorts these intricacies out:
curl -sSL https://install.python-poetry.org | python -
After following either path, you should take a moment to make sure that installation was successful by prompting for the version of Poetry installed with:
poetry --version
II. Dissecting the Problem: Accessing the Installed Command Everywhere
After the procedure mentioned above, if you find yourself facing the dreaded “command not found” message, it’s time to perform a little detective work. A good starting point is to check if Poetry was installed correctly and its location with:
which poetry
If the poetry path isn’t returned, don’t panic, your effort hasn’t gone to waste. We just need to direct your console to the right location. For example, in Unix systems such as Linux or MacOS, the installation of Poetry will usually reside at $HOME/.poetry/, thus appending its bin directory to the PATH variable will equip any console instance with the needed command:
In Bash: |
source $HOME/.poetry/env |
In Fish: |
source (python -m site --user-base)/bin/activate.fish |
---|---|
In Tcsh: |
source `python -m site --user-site`/../bin/activate.csh |
Keep in mind that the changes made are reserved for the current terminal session only. To reflect them onto every new terminal instance, add the required source command to the respective shell startup script.
III. Employing Python’s built-in ‘site’ Module
An alternate way of adding the required path to the PATH environment variable involves utilizing Python’s built-in ‘site’ module. Python’s site module enables one to append the user-site accessible across all python environments including virtual ones.
export PATH=$(python -m site --user-base)/bin:$PATH
With the insights and solutions provided, I trust you’ll manage to nail down this issue and continue working your wizardry with Poetry. Adding PATH in almost every operating system demands attention to details and post-installation know-how, making our pursuit more than just resolving a common mishap. It’s about knowing the inner workings, picking up skills transferrable to many development scenarios, and ultimately elevating our proficiency as developers. Do remember to look up detailed instructions in the official poetry documentation. They have done an excellent job outlining best practices for diverse platforms.
If you’ve successfully installed Poetry on your system but still find yourself facing the ‘Poetry: Command Not Found’ error, don’t worry. This issue arises due to instances where Poetry was installed correctly, but its environment path wasn’t set up properly.
Here’s an extensive dive into how we can troubleshoot and resolve this issue, ensuring a smooth workflow with error-free Poetry commands:
Understanding The Problem
The ‘Poetry: Command Not Found’ problem often occurs if the executable path of Poetry isn’t included in your system’s PATH variable. On installing Poetry, it creates a new Python virtual environment distinct from your global Python setup. If the PATH doesn’t contain the appropriate reference to Poetry, your operating system won’t know about this separate setup and won’t be able to execute Poetry commands.
Checking Your Environment Path
You can verify the issue by checking your environment path. Simply use this command in your terminal:
echo $PATH
If the output doesn’t include a reference to poetry (like ~/.poetry/bin), then that’s the root of the problem.
Updating Your Environment Path
To solve this, we need the system to be aware of Poetry’s installation path.
For Unix-based systems, add this line to your profile (~/.profile, ~/.bash_profile, ~/.zshrc or whatever shell you’re using):
export PATH="$HOME/.poetry/bin:$PATH"
For Windows PowerShell, use the following command:
[Environment]::SetEnvironmentVariable("Path", "$HOME\\.poetry\\bin;$PATH", [System.EnvironmentVariableTarget]::User)
After running these commands, restart your terminal for the changes to take effect.
In addition to this, make sure that you’ve installed Poetry globally. When installing Poetry in a local project directory, operating outside the project will result in the ‘Poetry: Command Not Found’ error.
Making Use Of The “poetry env” Command
Lastly, Poetry’s env command provides valuable information on which Python versions are currently being managed by Poetry. Running
poetry env info
will display the current active Python version.
By following these steps, we should be able to get rid of the ‘Poetry: Command Not Found’ error henceforth making the usage of Poetry a smooth experience.
If you face any other issues related to Poetry, consider referencing their official docs. They provide comprehensive materials covering almost all aspects and potential problems when dealing with Poetry. No matter what challenge you might face with Poetry, remember to always start by examining your environment settings. This is often a major source of similar errors.Stumbling upon the “Poetry: Command Not Found” error even after successfully installing Poetry can truly be a perplexing turn of events. This situation arises when your system fails to recognize where Python Poetry is located, and this is where environment variables come into play. These variables essentially tell your system about the paths where executables are stored.
An executable may still be found “missing” by your system, regardless of successful installation, if it’s not in a directory that’s part of the PATH environment variable. The PATH variable is crucial because it enlists directories that the system checks whenever a command is run. Hence, if your Poetry executable isn’t added to this list, you might get a ‘command not found’ error.
Environment variables come in handy while coding and troubleshooting as they:
– Hone flexibility and project configuration ownership – They allow developers control on how their applications behave without modification of code.
– Grant essential hierarchical organization within programs.
– Offer a consistent way to communicate run-time configuration to your applications.
In terms of solving the issue of “Poetry: Command not Found”, you might want to inspect if the Poetry bin directory is indeed included in your PATH. First, ensure Poetry is installed correctly with pip:
pip install poetry
Next, check if Poetry has its own dedicated binary path:
echo $PATH | grep '.poetry/bin'
The above command should reveal all directories enlisted in your PATH variable and will actively try to locate the ‘.poetry/bin’ folder within them. If it comes back empty, then we have found the source of your problem!
To manually include the Poetry bin directory to your PATH, update your .bashrc, .zshrc, or similar file:
echo 'export PATH="$HOME/.poetry/bin:$PATH"' >> ~./bashrc
And then source it:
source ~/.bashrc
After implementing the above changes, invoking the ‘poetry’ command should proceed without any hitches. Environment variables and a keen understanding of them can play a significant role in the bridge between successful program execution and debugging a plethora of intricate programming issues.The issue “Poetry Installed But Poetry: Command Not Found” is a common encounter for Python developers when working with the dependency management tool, Poetry. Several factors can lead to this error, but most frequently it emerges as the result of improper or incomplete installation.
The Crux of the Matter
If you input
poetry -version
in your terminal and get an error that looks something like ‘command ‘poetry’ not found’, then your poetry installation didn’t work as expected. This means that the path to the Poetry executable was not added correctly to your PATH environment variable.
There are a number of potential solutions to this issue ranging from reinstallation of Poetry, manually adding directories to the system PATH, or adjusting shell configuration files.
Reinstalling Poetry
Sometimes, reinstalling Poetry might be necessary if the initial installation omitted critical components. Using the recommended installer for your specific operating system, follow the provided instructions meticulously to ensure every prerequisite software is installed.
Manually Adding Directories to PATH
You could explicitly add the directory containing the Poetry executable to your PATH by following these steps:
* Identify the location where Poetry was installed. Usually, on Unix systems, scripts such as Poetry are placed inside ‘
$HOME/.local/bin
‘.
* Modify your shell’s profile file. Depending on your shell you might have different configuration files (like
.bashrc
,
.bash_profile
,
.zshrc
). Here’s an example with .bashrc:
– Open the terminal and type
nano ~/.bashrc
.
– At the end of the file, add the line
export PATH="$HOME/.local/bin:$PATH"
– Save and close using CTRL-X, and confirm with Y.
– Finally, update your current shell session by entering
source ~/.bashrc
Now try running the
poetry --version
again. If successful, the version of your installed Poetry should display.
Adjusting Shell Configuration Files
Yet another way to resolve the ‘command not found’ error can be by configuring the Shell file (.profile, .bashrc, .bash_profile, .zshrc etc.) to accept commands from the local bin. The exact shell configuration file to be edited will depend on the OS being used.
Keep in your mind that ensuring a correct and thorough installation of Poetry tremendously optimizes its performance. Like any other program, Poetry conforms to the principle of Garbage In Garbage Out(GIGO); if the installation is flawed, it reflects negatively on the operation of the entire system. Following all the specified guidelines pertaining to setting up Poetry helps avoid problems and errors during usage, and creates a more reliable and efficient development environment.
Supplementary reading:
When you’re using Python’s package manager, Poetry, a common stumbling block may be hitting the frustrating “Poetry: Command not found” error after installation. Understanding what’s causing this error and how to avoid it is incredibly useful to conduct your tasks successfully.
- Understanding the ‘Poetry: Command not found’ error
This issue usually arises when Poetry is installed but with incorrect path configurations. The shell terminal is unable to recognize or locate the command ‘poetry’, as it does not know where it has been installed. Either the directory path where “poetry” is located is not included in the system’s PATH variable, or there are unexpected inconsistencies in the environment setup.
To validate whether this is the case, you can use the following command:
$ echo $PATH
If the output doesn’t include the directory where poetry is installed, then you’ll need to add it to your PATH variable.
- How to correctly set up the PATH in Unix-based systems?
For Unix-based systems, you might have chosen local installation (user scope) over global installation (system-wide) and now wonder why your terminal cannot find the ‘poetry’ command. Here’s a simple way of dealing with this scenario:
Start by locating where the “poetry” executable is installed:
$ which poetry
Assuming that the command returns a file path (e.g,, /Users/username/.poetry/bin/poetry), add this to your shell profile (you may be using .bashrc, .zshrc, or .bash_profile, etc.):
$ echo 'export PATH="$HOME/.poetry/bin:$PATH"' >> ~/.bashrc
Then, refresh the instance of your shell:
$ source ~/.bashrc
- Resolving the issue in Windows systems
In Windows systems, use below commands to add Poetry to your PATH:
$ setx path "%path%;C:\Users\username\.poetry\bin"
Make sure to replace ‘username’ with your username.
Remember: Changes to PATH variables usually require a restart of the system, or at least closing and reopening your command line interface, for them to take effect.
- Using pipx as an alternative installation approach:
If you’re experiencing repeated issues, you could consider using `pipx` to install poetry. `Pipx` installs Python applications in isolated environments, making sure everything stays neat and tidy (pipx documentation). Here’s the code you’d run:
$ pipx install poetry
To verify if the poetry installation was successful, you can run
$ poetry --version
I hope this walkthrough helps you alleviate the frustration that comes from encountering the “Command not found” error. It happens to everyone at some point. Just keep calm and debug on!One of the common pitfalls that both new and experienced coders may often come across is encountering the ‘Command Not Found’ error message. As an illustration, you might have executed a successful Poetry installation, but on running it, you experience the
Poetry: Command Not Found
error. This discrepancy can be quite frustrating; however, there are effective tips to prevent such occurrences in your future coding sessions.
An effective way of mitigating this issue is by understanding what triggers these errors. Primarily, the ‘Command Not Found’ issue arises when the system fails to recognize a specific command or cannot locate it. In general settings, this happens when the command does not exist, has been misspelled, or is not recognized in the PATH environment variable.
Check Your Syntax
Always double-check all the syntax before finalizing any codes. Small typographical errors might result in a ‘Command Not Found’ error. You can verify the exact syntax from the official documentation or an authentic online resource and compare it with your code snippet as a reliable solution for accurate syntax.
Update Your PATH Environment Variable
Another excellent practice is regularly updating your PATH environment variable. If Python or Poetry is installed but not recognized, this means they may not be included in the list of directories where shell looks for commands.
What you should do is:
1. Modify the .bashrc file located in your home directory:
nano ~/.bashrc
2. Add this line at the bottom:
export PATH=$PATH:/directory-of-your-poetry/bin
3. Save your changes and run this command to effect the changes immediately:
source ~/.bashrc
Sometimes, you’ll need to install poetry through a custom installer method, e.g., for Windows users, pip. If you’ve taken this route, try reinstalling Poetry via the recommended installation method provided in the official Poetry documentation. This way, you can avoid many issues related to paths, permissions and compatibility with other libraries.
Regularly Update Poems, Dependencies and Plugins
Regularly updating your poems, dependencies and plugins is also efficient in keeping at bay ‘Command Not Found’ errors. It’s essential to stay updated with the most recent versions of your tools because new features and bug fixes often accompany these new releases. To update poetry itself, use the command:
poetry self update
Lastly, while using third-party packages, try to use those with active communities and regular updates, because these groups will tend to resolve the common bugs frequently and help create a smooth development process. While dealing with coding issues, being patient, diligent, and persistent will enable you to navigate around any problem successfully.Command not found issues are the result of your system not recognizing a particular command because it’s not on the system PATH. The System PATH is essentially a list of directories where your shell looks for executable files when you enter commands.
For instance, if you’re trying to use the Poetry tool which you have installed, but when you type
poetry
into your terminal, it returns with “Poetry: Command Not Found”, your first step should be double checking whether or not Poetry was installed correctly. You can do this via the command
pip show poetry
. This will display information about the installed Poetry package, including its location.
If you find that Poetry was indeed installed correctly, the problem then lies with your system PATH. As mentioned earlier, the system PATH contains a list of directories for your shell to check. If the directory where the Poetry executable resides isn’t included in the System PATH, you’ll experience a “Command not found” error.
To display the current system paths, use the echo command:
echo $PATH
Your system responds by displaying a colon-separated list of paths. Now, cross-check this list with the location where Poetry is installed according to the results of our
pip show poetry
command from earlier. If the directory where Poetry resides isn’t listed here, we have found the culprit!
Do not fret, updating the PATH is quite straightforward. Depending on whether your shell uses bash or zsh, the process could differ slightly. Below is how you add a new path (the Poetry path in this case) to both:
For BASH (Usually older systems use BASH). Open your ~/.bash_profile file and add:
export PATH=$PATH:/path/to/poetry
For ZSH (Newer systems like MacOS Catalina or later use ZSH). Open your ~/.zshrc file and add:
export PATH=$PATH:/path/to/poetry
Remember to replace with the actual entire path where Poetry was installed as per our
pip show poetry
execution. After adding these lines, save and close the .bash_profile or .zshrc file.
Once you’ve made this addition, Source your updated profile file for bash (
source ~/.bash_profile
) or zsh (
source ~/.zshrc
). By running ‘source’, the terminal knows to execute the commands in the given script file, thereby incorporating the changes we made into the existing session.
Now typing
poetry
at your terminal should successfully launch the Poetry tool since the system is now aware of where to find it!
Please bear in mind, though, that hard coding paths might not always be sustainable or desirable, especially if there are multiple users on your machine or deployment environments might differ from your local setup. In such cases, package managers like Pyenv along with virtual environments can provide more flexibility by allowing you to maintain different versions of tools like Poetry, each assigned to specific projects or users. Learn more about pyenv.
Lastly, this whole talk of modifying System PATHs requires user-level permissions, so remember to consider security implications and ensure you know what each script/file does before adding it to your PATH More on security considerations.Resolving the issue of ‘Poetry: Command Not Found,’ even after installation is a hurdle many professional coders encounter. This usually implies that Poetry is not reachable through your system’s PATH.
You can resolve this using simple solutions. To modify the PATH, you can apply variable alterations in the terminal session. Here are steps to guide you:
echo $PATH
This line of code will display your present paths.
If you discover that the path to Poetry isn’t included, include the following line to either your ‘.zshrc’, ‘.bash_profile’, or ‘.bashrc’ file in your home directory if you are on Unix (Linux, macOS). If you’re using Windows, make an adjustment in your environment variables.
For Unix based systems,
echo 'export PATH="$HOME/.poetry/bin:$PATH"' >> ~/.zshrc
For Windows systems, update the PATH environment variable using:
setx path "%path%;C:\Users\yourusername\.poetry\bin\"
Remember to replace “yourusername” with your actual Windows username.
After modifying the PATH variable, reload the terminal or run the resource on your alteration file for changes to take effect like:
source ~/.zshrc
However, do take note that problems could also stem from hitches during the Poetry’s installation process. Incorrect installations typically result in this error and rectifying it might require reinstallation. Various platforms have specific methods for reinstalling Poetry, so be sure to refer to the official Poetry documentation for appropriate steps based on your operating system.
Moreover, issues can originate from conflicts if Python wasn’t installed via Homebrew for MacOS users. Observing best practices by deploying Python versions through virtual environments assists in avoiding such clashes.
In summary, these answers on handling ‘Poetry: Command Not Found’ post-installation showcases key insights gained regularly from programming tasks. By providing such insights, I aim to enhance your journey in Python application development, hoping it proves vital in dealing with similar issues in the future.