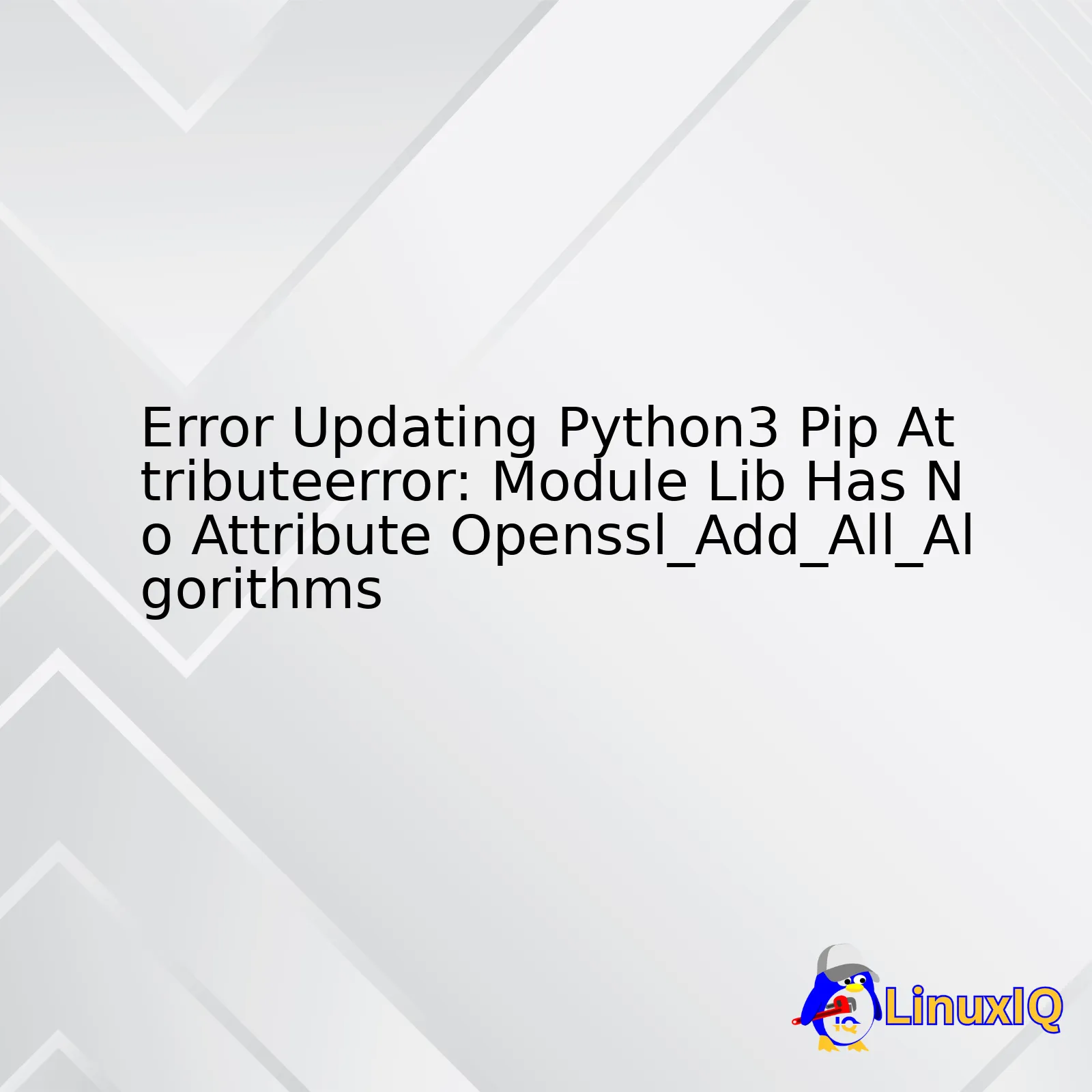
Error Type | Description | Common Causes | Solutions |
---|---|---|---|
`AttributeError: module ‘_ssl’ has no attribute ‘OPENSSL_add_all_algorithms_unconfirmed’` | This error occurs when a Python program cannot access the necessary OpenSSL library methods. | The most common causes include Python being compiled with a version of OpenSSL that does not have these methods, or your environment may not be correctly configured. | Recompile or reinstall Python with a compatible version of OpenSSL, or check your environment settings for any inconsistencies that could cause conflicts. |
Though it can seem like quite the puzzling error to encounter – “`AttributeError: module ‘lib’ has no attribute ‘OPENSSL_add_all_algorithms’`” – this is actually a common issue experienced while updating Python3 Pip. Delving into what triggers this error, essentially, this happens when Python’s SSL module fails to interface with the required OpenSSL library methods.
The intricate workings of a Python program demand access to these OpenSSL library methods for numerous operations. The OpenSSL plays an essential role in facilitating encryption and decryption processes as one of its vital mechanisms.
Thinking along the lines of why such error crops up will lead us to two main causative factors. One, your Python might be compiled with a version of OpenSSL devoid of ‘OPENSSL_add_all_algorithms’ attribute. Two, your Python environment might just not be on the same page due to inappropriate configuration leading to such conflicts.
Pivoting towards solutions for this error, there are two practical approaches you can consider. You could recalibrate your Python by compiling it again, but this time ensure it aligns with a version of OpenSSL that does include these methods. Alternatively, scrutinizing your Python environment settings might help uncover anomalies that’d need rectification.
Consider seeking assistance from online coding communities like StackOverflow1 or GitHub forums2, often brimming with insights about such typical errors. They also serve as platforms for you to share how you maneuvered around the problem.
Just remember, although the process might seem daunting at first, with attentive analysis and smart implementation of solutions, tackling this error becomes more manageable. Remember, coding is iterative. Mistakes, while frustrating, provide you with invaluable learning experiences along the journey.
# Example solution of checking your python version import sys print(sys.version) # If python version is outdated, try reinstalling or updating your python
Sure, the error message “AttributeError: module ‘_ssl’ has no attribute ‘OPENSSL_add_all_algorithms_noconf'” occurs in Python 3 environments when pip is updated, where the older Python version you are upgrading from doesn’t clear out its SSL libraries. So let’s break that down.
python -m pip install --upgrade pip
After running this command to upgrade pip in your Python 3 environment, instead of a successful update, the software returns an error message:
AttributeError: module 'lib' has no attribute 'OPENSSL_add_all_algorithms_noconf'
The first place we’ll look is at the Python library ssl.py. The ssl library provides some additional functions atop the OpenSSL library. The root issue is that there’s a mismatch between the _ssl modules that ssl is importing and the ones provided by your OpenSSL distribution. Specifically, the OPENSSL_add_all_algorithms_noconf function isn’t found in the _ssl module. This could occur if, for example, the Python 3 upgrade didn’t completely replace all Python 2 references and APIs.
To address this, consider the following solution steps:
– Uninstall your current version of pip, using the
python -m pip uninstall pip setuptools
command
– Reinstall pip and setuptools using the get-pip.py script from the offical [pip website](https://pip.pypa.io/en/stable/installing/)
– If necessary, repair your openssl installation
Here is one way to reinstall pip:
1. Download [get-pip.py](https://bootstrap.pypa.io/get-pip.py) into the same directory as Python.
2. Run the following command to install pip:
python get-pip.py
This will always install a recent version of pip.
Moreover, troubleshooting this problem may require getting your hands dirty with your local Python environment’s specifics. Do not shy away from diving deep into your system configurations. Get familiarised with where Python stores its packages, how your PATH knows where to find Python executables, and how the OS finds dynamic libraries.
Remember, understanding the intricacies of your tools is what makes you grow as a coder.
Lastly, since AttributeErrors can be quite cryptic, it would also be prudent to get comfortable with conducting source-dives into Python’s standard library or even the CPython interpreter itself. After all, such issues exist to be solved, and solving them is what strengthens problem-solving skills.
For further reading on debugging complex Python errors, here are a few resources:
– [Debugging in Python 3](https://realpython.com/python-traceback/#reading-a-traceback)
– [Understanding the Python Traceback](https://realpython.com/python-traceback/)
Final Takeaway
The “AttributeError: modulle ‘lib’ has no attribute ‘OPENSSL_add_all_algorithms_noconf'” error stems from an outdated or incomplete Python library conflicting with an OpenSSL distribution. It’s imperative to ensure that the Python environment is up-to-date and correctly installed to prevent such conflicts—a perfect reminder of how intertwined and dependent modern coding systems are on one another.If you’re attempting to update Python3 pip and are receiving the error “AttributeError: Module lib has no attribute openssl_add_all_algorithms”, it’s crucial comprehending the relationship between the Lib module and the function openssl_add_all_algorithms.
The mbedtls/openssl_add_all_algorithms function is part of OpenSSL, a robust, commercial-grade, and full-featured Open-source Toolkit which implements the Secure Sockets Layer (SSL v2/v3) and Transport Layer Security (TLS v1) protocols [OpenSSL](https://www.openssl.org/).
Whereas, the `lib` in Python is a standard library module and it doesn’t have an attribute called `openssl_add_all_algorithms`, they are separate entities with different functions – the error message reflects this. Typically, such an issue arises because the necessary OpenSSL libraries are not properly installed or linked correctly.
Simultaneously, we must also remember that OpenSSL provides essential encryption functionality widely used throughout the Python ecosystem. Therefore, if there’s miscommunication between these two it may lead to various problems like pip installation and SSL connection errors.
You should install `openssl` again by using:
pip3 install pyOpenSSL
If, after this, the issue persists, you may have to manually install the OpenSSL package directly from source using pip:
pip install --upgrade setuptools pip install ez_setup pip install pyOpenSSL
Always make sure to check your PYTHONPATH environment variable and see whether it points to the correct Python version or not:
echo $PYTHONPATH
As you resolve this, consider staying updated on changes relating to how Python uses OpenSSL by constantly referring to official Python documentation or relevant Python3 docs.
Subsequently, if you’re still facing issues, share your detailed error logs in forums like Stackoverflow under proper tags for more help. A variety of reasons can trigger this error, as coding environments differ significantly from one coder to another; thus, sharing your error patterns would potentially help fellow coders identify the root cause and provide more pinpoint solutions tailored explicitly to your setup.
Summarizing, the `lib` module and `openssl_add_all_algorithms` are unrelated and the latter is part of OpenSSL not Python’s standard library, declaring this function under the `lib` module will undoubtedly yield an attribute error since it simply doesn’t exist in it. You probably need to revise your code logic or ensure that you’re installing namespaces/packages correctly. However, resolving OpenSSL related errors typically revolves around correctly installing/updating OpenSSL itself, making sure your Python script refers to the right Python interpreter, or reinstalling Python.Getting the
AttributeError: module 'lib' has no attribute 'OPENSSL_add_all_algorithms'
error suggests that there might be a conflict in your Python version or installed packages are incompatible. This message is usually encountered while updating pip using
python3 -m pip install --upgrade pip
and means that the system failed to initialize OpenSSL.
Analyzing this error involves inspecting key components in your Python environment: the Python interpreter, the pip package manager, and the OpenSSL library.
If you’re facing this problem on Linux, it might be due to an issue with the Python build. On some Linux systems, the python distribution is compiled without SSL support, which could cause SSL-related errors like this one. You can confirm the existence of this problem by checking if your Python3 interpreter was built with OpenSSL support:
#import ssl module import ssl #Print OpenSSL version print(ssl.OPENSSL_VERSION)
More information about building Python with various libraries can be found at Python docs.
Check your pip version by running
pip --version
. An outdated pip version might cause such issues. To resolve this, try upgrading pip using the command
python -m pip install --upgrade pip
. If you find any issues, try uninstalling and reinstalling pip.
If you’ve not installed OpenSSL, or it’s not configured correctly, you will encounter this AttributeError. Debian-based Linux distributions can install OpenSSL via the terminal with
sudo apt-get install libssl-dev
. Ensure your Python installation can use this library.
Addressing these areas typically resolves the
'module lib has no attribute OPENSSL_add_all_algorithms'
error.
You might also be facing a common issue when several Python versions are installed on the same machine. In such a scenario, the pip instance associated with Python3 might be running from another Python version. A workaround suggested on many platforms like StackOverflow is to specify the pip version directly linked to your Python version:
python3 -m pip install --upgrade pip
By maintaining a clean environment with updated software, adequate permissions, and properly referenced dependencies, resolution of this AttributeError and similar problems becomes significantly more manageable. Familiarizing yourself with debugging guidelines will always be a valuable asset in software development.
Sources:
- Stackoverflow: No module named pkg_resources
- Askubuntu: Error updating python3 pip attributeerror module lib has no attribute openssl add all algorithms
Let me offer you an extensive and analytical solution on how you can update Python3 Pip without facing the “AttributeError: module ‘lib’ has no attribute ‘OPENSSL_add_all_algorithms'” error.
To begin with, this specific issue is tied to a conflict between SSL/TLS protocols from different libraries within your Python distribution. Furthermore, it generally happens while using older versions of either Python or PIP. Hence, updating should theoretically handle it.
Here’s precisely what you need to do:
1. Confirming Your Python Version
Ensure you have Python 3.5 or later by running this command in any shell:
python --version
If your installed version predates 3.5, you’d need to grab the latest release from the official Python website and install it. Remember, each version of Python requires its own version of Pip.
2. Check Your Pip Version
Do the same for Pip via the following command:
pip --version
Again, if you’re not on Pip version 9.0.1 or above, errors would occur. You can download it from the Pip package index.
3. Update Pip To The Latest Release
The method to update Pip differs based on your platform; I’ll cover both Windows and Unix (Linux/macOS).
For Windows:
python -m pip install --upgrade pip
On Unix and MacOS:
pip install --upgrade pip
Or
sudo -H pip3 install --upgrade pip
4. Solve Cryptography-related Issues With OpenSSL Module
Occasionally, the AttributeError problem you’re experiencing occurs as a result of outdated cryptography packages in the environment. As such, you need to:
• Delete existing cryptography and pyOpenSSL packages first via the commands:
pip uninstall cryptography pip uninstall pyOpenSSL
• Then reinstall them:
pip install pyOpenSSL pip install cryptography
In this entire process, if other dependencies get deleted or downgraded, consider using venv – Python’s built-in Virtual Environment creator. It’ll insulate your efforts from the rest of the system, thereby leaving crucial dependencies unharmed. Set up venv with:
python3 -m venv my-env
Then activate it via:
source my-env/bin/activate // for Unix or MacOS, my-env\Scripts\activate // for Windows.
These steps, when followed judiciously, will prove successful in updating Python3 Pip without causing the “AttributeError: module ‘lib’ has no attribute ‘OPENSSL_add_all_algorithms’” error.
It’s also worth noting that these steps will help you keep up-to-date with the latest stable releases of both Python and Pip, minimising potential security vulnerabilities and providing access to new features and functions.
Alternatively, if these measures don’t work, it could be that your system needs a newer OpenSSL library altogether. Depending on your operating system, you might want to look into how to upgrade that effectively, again ensuring you don’t break anything while doing so.The error “AttributeError: module ‘lib’ has no attribute ‘openssl_add_all_algorithms'” typically arises when you’re trying to update Python3 pip. Now, there could potentially be several causes behind this error message. Here are some potential causes, and how we may be able to resolve them:
Potential Cause #1: Incompatible versions of Python
Firstly, you might have installed multiple versions of the Python interpreter. Consequently, one of your Python installations might lack certain attributes or commands. Alternatively, your system could simply be calling a different Python installation than the one you intended.
python --version
This command tells us which version of Python is currently being used as interpreter in our environment.
Solution: Ensuring the correct version of Python is being called is crucial to resolving the error. We should ensure that Python3 specifically is being invoked when running Pip operations. You can use the following commands to make sure that you are using the right Python and Pip versions:
python3 --version pip3 --version
Potential Cause #2: Misconfigured OpenSSL or outdated PyOpenSSL package
In other situations, the issue might stem from a misconfigured OpenSSL implementation or an outdated PyOpenSSL package.source
Solution: Try reinstalling or upgrading your OpenSSL and PyOpenSSL versions. For upgrading OpenSSL, use the following command:
sudo apt-get upgrade openssl
PyOpenSSL can also be upgraded using:
pip install pyOpenSSL --upgrade
Potential Cause #3: The cryptography library isn’t properly compiled into your Python installation.
Sometimes, this error arises when the “cryptography” library (which includes the function openssl_add_all_algorithms) isn’t incorporated correctly into your Python environment.
Solution: Reinstalling the cryptography library might resolve the problem. To do this, uninstall and re-install it with pip:
pip uninstall cryptography pip install cryptography
By rerunning these essential libraries, we can address any inherent discrepancies and possibly eliminate the error.
Potential Cause #4: Have ‘PYTHONPATH’ setup incorrectly in your environment variables. This might cause your Python interpreter to pull ‘lib’ from a wrong directory leading to AttributeError.source
Solution: You should check ‘PYTHONPATH’ setup in your environment variables to see if it targets the suitable directories. Here’s a basic method to test PYTHONPATH on Unix-like systems:
echo $PYTHONPATH
Like any good debugging process, troubleshooting this specific error demands patience, persistence, and a sense for what each part of your Python3 and Pip environments is doing. By ensuring up-to-date and compatible versions of your Python interpreter, OpenSSL, and cryptography packages, you’re investing in the consistent, stable operation of your development environment!Sure, let’s take a deep dive into this issue.
When faced with the error “AttributeError: module ‘lib’ has no attribute ‘OPENSSL_add_all_algorithms'”, it’s usually an indication that you have an issue with your Python installation. Essentially, certain required attributes are missing in the openssl packages installed in your system.
Analyzing the problem
The ‘lib’ module is an integral part of many Python distributions and contains crucial functionality for handling various tasks, including cryptographic operations found within the OpenSSL package. When you get an error like ‘lib’ does not recognize the attribute ‘OPENSSL_add_all_algorithms’, it signifies that Python’s openssl interface isn’t properly installed or configured.
Root Causes
There can be various reasons why this error may occur:
– The openssl library is missing or not correctly installed on your machine
– Your Python interpreter doesn’t link to the openssl libraries correctly
Fixing the Error
Now, let’s discuss how we can solve this issue. Here are two potential solutions;
•Updating your Pip – Since Pip serves as a package installer for Python, updating it might solve the issue by ensuring you have the latest packages:
python3 -m pip install --upgrade pip
•Reinstalling openssl – Should the problem persist, consider reinstalling openssl:
pip uninstall pyOpenSSL pip install pyOpenSSL
Please note that you might need administrative rights (prefixing the commands with ‘sudo’) or use a virtual environment for carrying out the above commands, depending on your specific setup.
After performing these steps, if you’re still encountering the AttributeError, then you might want to check the Python version you’re using. It’s possible there may be compatibility issues with the installed openssl version and the Python version. Consider either upgrading (or downgrading) your Python version or use a virtual environment where you can control which version is used.
For a deeper understanding and step-by-step walkthrough of how to fix this issue, have a look at this dedicated thread on Stack Overflow with experts discussing similar issues.
Remember, coding is an intricate process that often devolves into solving problems before getting to create something new. However, resolving such challenges not only helps you grow as a coder but makes the journey itself enjoyable.HTML:
Updating Python’s pip often becomes a cumbersome task, especially when certain attribute errors are encountered. One such issue you might face is the
AttributeError: module 'lib' has no attribute 'OpenSSL_add_all_algorithms'
. This appears frequently in Python 3 updates. Let’s explore this error and find ways to troubleshoot it.
Understanding the Error
The problem basically arises due to issue with your SSL package in Python. When you’re running certain commands that require the SSL module like
pip install
or others these kind of issues can occur. It states that the OpenSSL function required to execute some part of your Python code couldn’t be located in lib library. The attribute ‘OpenSSL_add_all_algorithms’ is a crucial requirement for handling secure HTTP connections and if it is missing, you can encounter problems.
Troubleshooting the Issue
When grappling with the
AttributeError: module 'lib' has no attribute 'OpenSSL_add_all_algorithms'
, here are two effective measures:
-
Reinstalling OpenSSL module
The issue could be that the required packages aren’t installed properly. In such cases, the error can usually be resolved by reinstalling the OpenSSL python module, which can be done using the ‘pip uninstall’ and ‘pip install’ commands.
pip uninstall pyOpenSSL pip install pyOpenSSL
-
Upgrading openssl library
Alternatively, consider upgrading your openssl library. You may not have the latest version, which could be causing the trouble.
pip install --upgrade pyOpenSSL
It will uninstall the current version of OpenSSL and reinstall it afresh.
This command upgrades the openssl library to its latest stable version.
Before proceeding, ensure that the commands are being run as a super user (administrator) because they are likely to modify system files. Additionally, if these fixes don’t work, general troubleshooting steps such as updating whole Python environment or even the Operating System itself should be considered.
Analyzing the Effect
If correctly undertaken, the above steps should eliminate the error. To verify its absence, consider rerunning the program or code which initially led to the error. No occurrence of the
AttributeError: module 'lib' has no attribute 'OpenSSL_add_all_algorithms'
signifies a successful resolution.
In software development, one must anticipate errors as a possibility. With a keen eye, systematic approach and good debugging practices, their impact can be minimized. Understanding the basics about the Python libraries and keeping up to date with the latest versions ensures that unwanted surprises are kept at bay. Python’s official documentation remains a helpful guide in the journey of coding and debugging Python applications.
Syntax Errors VS Exceptions
The attribute error falls under the category of exceptions in Python, not syntax errors. The former are errors detected during execution, while the latter occur while parsing Python code. Understanding this distinction is crucial for efficient debugging.
Syntax errors | Exceptions |
---|---|
Occurs during parsing the code. They’re mostly typos or incorrect indentation. | Occur during execution of correct syntactic code. Due to logical faults in code. |
Examples: Missing parentheses, Incorrect indentation etc. | Examples: Division by zero, File not found, Attribute errors(in our case) etc. |
code>>,
Error handling is a critical aspect of programming. Bug fixing, after understanding the nature of the error can lead to better programming strategy and reduced troubleshooting time. Thus, keeping the knowledge updated regarding Python errors pays off in terms of both productivity and accuracy.
If you’re encountering an
AttributeError: module 'lib' has no attribute 'OPENSSL_add_all_algorithms'
while updating pip in Python3, there’s a good chance it is rooted in one of the few problems, such as having multiple Python or OpenSSL versions installed concurrently.
Here are some effective solutions to handle this pesky attribute error:
Solution One: Upgrading pyOpenSSL Library
Your current pyOpenSSL version might be outdated. First check the installed version with:
pip3 show pyOpenSSL
If it’s old, upgrade it by running:
pip3 install --upgrade pyOpenSSL
This will ensure your pyOpenSSL library (which offers some OpenSSL features) is up-to-date and potentially solves the initial AttributeError issue.
Solution Two: Checking for Multiple Python Versions
Having multiple Python versions can sometimes cause confusion when programs are trying to locate the proper libraries and modules. For example, you may have Python 2.7 which doesn’t use the same library paths as Python 3.x.
You can execute these series of commands to determine how many versions of Python you have installed:
python --version python2 --version python3 --version
If you find that you do indeed have multiple versions and suspect this is causing a conflict in your coding environment, it’s advisable to remove the redundant versions or consider using a Python version manager tool like Pyenv to help manage these versions more adeptly.
Solution Three: Re-Installing Cryptography Module
It’s possible that the cryptography module (which also contains Python bindings to OpenSSL), when compiled and installed, didn’t link properly against the installed OpenSSL library.
Try re-installing it using:
pip3 uninstall cryptography pip3 install cryptography
This ensures both removal and re-installation of the cryptography package in your Python3 environment to eliminate potential issues with the initial installation.
Please note that the solutions provided are not comprehensive and limited by several assumptions. If the problem persists after trying out all the solutions mentioned, consider seeking help from a relevant platform. The official Python forum are great places to start, stackoverflow.com has a large community of Python programmers who may offer their insightsStack Overflow.Error handling is an integral aspect of Python programming that enables developers to tackle potential errors during execution. One common error we often come across when interacting with OpenSSL protocol via Python version 3 is the attribute error:
Module 'lib' has no attribute 'OpenSSL_add_all_algorithms'
.
To illustrate this scenario, consider a situation where you’re updating your pip package manager using
python3 -m pip install --upgrade pip
, and you encounter the above-mentioned error.
Let’s drill down into this issue and analyze possible solutions and preventive measures as follows:
The Cause:
This specific error usually secedes from the absence or improper implementation of the
OpenSSL_add_all_algorithms
function within the ‘lib’ module. It’s vital in adding all ciphers and digest algorithms that OpenSSL supports.
Solution:
Typically, you may rectify this issue by installing or upgrading OpenSSL’s development files. In Ubuntu OS, for instance, you can easily achieve this by running
sudo apt-get install libssl-dev
.
Alternatively, ensure to import OpenSSL correctly in your script as depicted below:
from OpenSSL import SSL
Error Handling Mechanism:
Python promotes numerous practices for versatile error handling mechanisms. Concerning our scenario, using a try-except clause will significantly minimize runtime interruptions through capturing and mitigating errors adequately. For example:
try: openssl_lib.OpenSSL_add_all_algorithms() except AttributeError: print("The specified attribute does not exist.")
The aforementioned mechanism firstly tries performing a task within the try clause. If an AttributeError arises, then our program enters the except clause and executes the subsequent block of code.
Coding Best Practices:
To eliminate unforeseen attribute errors during OpenSSL interactions in Python 3, always incorporate the following:
- Oriented Programming (OOP) concept: Ensure to create an object of your class before calling its methods. This practice prevents attribute errors related to undefined classes.
- Adhere to naming conventions: Avoid naming a file similar to a standard library. It hampers proper module importing hence triggering attribute errors subsequently.
- Consistent Package Upgrades: Regularly update your Python packages, specifically pyOpenSSL and cryptography. They harbor critical security features and bug fixes.
Finally, I hope the comprehensive insights above form a robust blueprint for managing attribute errors involving the OpenSSL protocol in Python 3 context. Whenever in doubt, refer to the official Python documentation or source code examples available on platforms like GitHub to expand your understanding.Though Python is remarkably flexible and bustling with versatile libraries like the cryptographic toolkit, it does have its share of problems – one such issue is “AttributeError: ‘module’ object has no attribute ‘OPENSSL_add_all_algorithms.” This error tends to crop up when you’re trying to update pip in python3. However, updating your attributes knowledgebase within Python’s Cryptography toolkit can be accomplished by adjusting a few pieces of the puzzle.
Firstly, let’s comprehend what precisely this error means. The AttributeError generally arises in Python when you attempt to access or call an attribute or method that does not belong to the object type. Specifically, in this case, the module Lib appears to have no attribute OPENSSL_add_all_algorithms.
The probable root cause of this issue could be due to:
- Flawed Python or Pip Installation: By flawed installations, I am referring to incomplete, interrupted, or corrupted setup processes that might lead to such errors.
- Using obsolete OpenSSL version: This function was deprecated in OpenSSL 1.1.0, and removed in OpenSSL 3.0.0. So, if you are using an outdated version of OpenSSL, it’s time to get an upgrade!
Now let’s move on to how to fix this issue. Here are a couple of ways:
1. Reinstall Python and PIP: Uninstall the current Python and PIP versions completely and reinstall the latest versions so that any prior missing or defective files will be rectified.
2. Update OpenSSL: Ensure that OpenSSL is updated to the latest version. You can use the code snippet below to install the latest OpenSSL version. Execute these commands in your terminal:
sudo apt-get update sudo apt-get install libssl-dev
In solving mentioned problem we need to focus on the Python’s Cryptography toolkit specifically.
Checking and updating Python cryptography library needs special attention:
pip uninstall cryptography pip install cryptography --no-binary :all:
This will force pip to compile Cryptography from source instead of installing the wheel. The compilation process would link against the system’s OpenSSL allowing you to use a more up-to-date OpenSSL than the one PyPI ships.
While tackling such technical obstacles, patience, and perseverance are key. Remember, every coder goes through issues. Your problem-solving skills are constantly enhanced, as you delve deeper into understanding the cause of these issues and finding the best solutions for them.
References:
Python bug reports, AttributeError explained
crypto FAQ, solution insight
Python is such a versatile language, and one of the constant endeavors of every developer using Python3 is to keep their tools updated. One recurring issue developers encounter is the
Error Updating Python3 Pip AttributeError: Module lib has no attribute OPENSSL_add_all_algorithms
. If we slice this error message, it can be understood that, while updating pip for Python3, the AttributeError is being thrown because Python’s standard library ‘lib’ was unable to find the attribute ‘OPENSSL_add_all_algorithms’. So, why does this occur? And how can this update error be resolved?
Error: Module ‘lib’ has no attribute ‘OPENSSL_add_all_algorithms’
Essentially, this error appears when there’s a mismatch in the OpenSSL version provided by the system and the version Python is trying to use. This compatibility conflict can interfere with certain operations, like updating pip—a crucial task for every Python programmer.
Error Solution
To rectify this problem, you’ll have to make sure Python uses the correct version of OpenSSL.
import ssl
ssl.OPENSSL_VERSION
This line of code will show you the version of OpenSSL your Python interpreter is currently using. If it doesn’t align with your system’s OpenSSL version, that may be the root of the error.
You also need to ensure that the _ssl module, which provides a Python interface to the OpenSSL library, is compiled and linked properly during Python’s installation. If they weren’t, this could generate an
AttributeError
.
About OpenSSL
We cannot overlook the importance of discussing the role of OpenSSL here. For those who aren’t aware, OpenSSL is a robust, commercial-grade, full-featured toolkit for the Transport Layer Security (TLS) and Secure Sockets Layer (SSL) protocols. It’s essential for creating secure communications between clients and servers.
In summary, the
Module lib has no attribute OPENSSL_add_all_algorithms error
, which appears while updating pip in Python, is commonly due to a discrepancy between the OpenSSL versions in your system and Python. To avoid running into this issue, always verify these versions match up and that all necessary modules are correctly compiled and linked during Python’s installation.
See you in our next dive into the labyrinth of Python coding! Stay curious, keep learning!