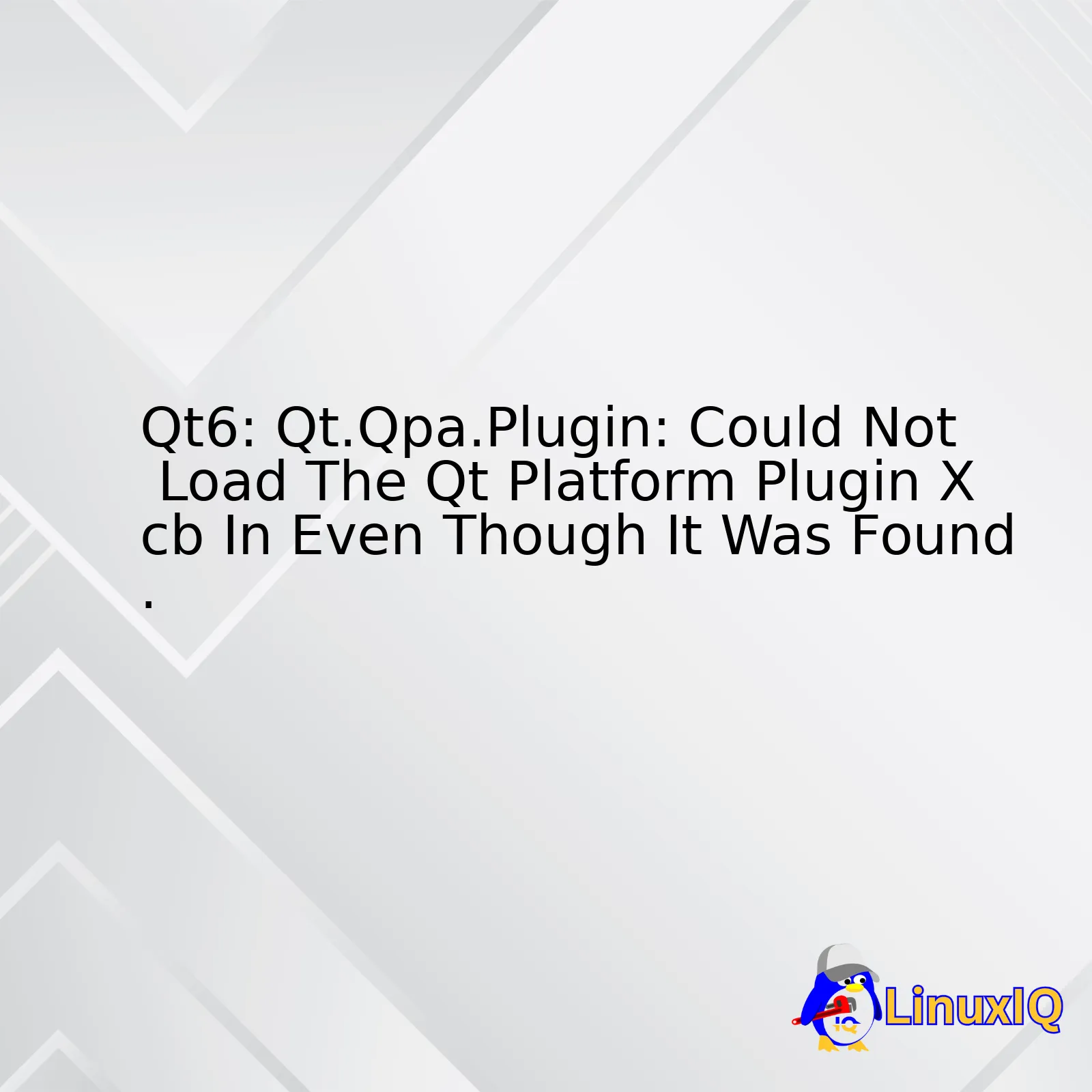
Error | Causes | Solutions |
---|---|---|
“Qt6: Qt.Qpa.Plugin: Could not load the Qt platform plugin xcb in even though it was found.” |
|
|
About the error “Qt6: Qt.Qpa.Plugin: Could not load the Qt platform plugin xcb in even though it was found.” while running a Qt program, this typically signifies that there’s a problem with your Qt installation or the application’s configuration. You are likely trying to run a Qt-based application, and the QPA (Qt Platform Abstraction) layer cannot load its platform-specific implementation named “XCB” even though it’s available.
This type of error could be caused due to the incorrect setting of environment variables, such as the lack of or erroneous value for the QT_QPA_PLATFORM_PLUGIN_PATH variable, which should point to the directory containing the plugins you’re using.
Another potential cause might be missing or incompatible libraries or dependencies, like some essential components that the XCB plugin needs but couldn’t find in your system libraries. This can take place especially when mixing software from different sources, versions, or having an incomplete dev-ops environment setup.
To address these issues, you could try setting the QT_QPA_PLATFORM_PLUGIN_PATH environment variable explicitly to guide Qt to locate its plugins. If it’s a matter of missing libraries, make sure all necessary dependencies are correctly installed on your system. And if it’s an issue with the Qt installation, uninstall it, then download the newest version from the official Qt website and install it again.
Consider recompiling your app in the new installed Qt environment:
$ qmake -project $ qmake $ make
Notice that you need to consult your application’s documentation and follow the recommended practices. They often provide guidance on what libraries you need to have and how to configure your software environment properly.
One good way to investigate the problem would be to enable more verbose logging information using the QT_DEBUG_PLUGINS environmental variable:
export QT_DEBUG_PLUGINS=1
Running your program after this command will provide a more detailed output about what specifically goes wrong during the loading of plugins.In Qt6, the Qt Platform Abstraction (QPA) is a platform abstraction layer for the application running on different operating systems such as X Windows System, Windows, and Mac OS, amongst others. Carefully understanding its inner workings not only can assist in troubleshooting, but also ensures a better runtime environment for your applications.
But here’s the situation: you are head-to-desk over “Qt.qpa.plugin: Could Not Load The Qt Platform Plugin Xcb” error even though it was found. Here’s what could be happening:
Failed to load platform plugin xcb. This application failed to start because it could not find or load the Qt platform plugin xcb...
In simple terms, this error signifies that an application running with Qt could not load the needed plugin to interact with the XCB system (X protocol C-language Binding). This happens even when the XCB itself is installed and properly working.
This mismatch error commonly occurs due to:
– Misconfiguration of the Qt installation
– Incorrect setting of the `QT_QPA_PLATFORM_PLUGIN_PATH` environment variable
– Absence of the correct runtime dependencies
So, without digging any more into terminologies let’s cut to the chase – how to solve the issue?
First, you need to verify your Qt Installation. Assure that the XCB plugin is correctly located at `
Secondly, correctly set up the `QT_QPA_PLATFORM_PLUGIN_PATH` environment variable. Make sure it points to the `platforms` directory in your Qt installation like so:
export QT_QPA_PLATFORM_PLUGIN_PATH=/plugins/platforms
Don’t forget to replace
Lastly, ensure that all necessary library dependencies are met. Use the `ldd` tool to list the shared library dependencies of the XCB plugin:
ldd/plugins/platforms/libqxcb.so
Libraries marked as “Not Found” will need to be installed. For example, if libXrender.so.1 is missing, you should be able to install it using `sudo apt-get install libxrender1`
Incorporating these solutions will help you smoothly integrate QPA and platform plugins in QT6. Understandably, each dependency might seem like a puzzle piece, but they fittingly come together to create a seamless picture – a functional application with a smooth-running UI.
For further troubleshooting, consult [Qt Documentation about Platform Plugins](https://doc.qt.io/qt-5/qtplatformplugin.html) or visit the [official Qt forum](https://forum.qt.io/topic/93247/qt-qpa-plugin-could-not-load-the-qt-platform-plugin-xcb-in-even-though-it-was-found).
Take note of the importance of carefully tracking your dependencies, installations, and configuration files as a professional coder, as well as understanding the underpinning platform abstractions like QPA. Remember, every line of code has a purpose, every function fulfills a task, and every failure nudges you closer towards success.
It’s great that you’re exploring Qt, a cross-platform framework used for developing software and GUIs. In the context of your question about deciphering the `QT.Qpa.Plugin` error message relevant to ‘Qt6: Qt.Qpa.Plugin: Could Not Load The Qt Platform Plugin “Xcb” in Even Though it was Found,’ here’s how I’ll elaborate on this issue.
Firstly, it’s crucial to understand what `QT.Qpa.Plugin`, ‘Xcb’, and their roles within the Qt system are.
– `Qt.Qpa.Plugin`: QPA (Qt Platform Abstraction) is a plugin interface provided by Qt and it serves as the platform abstraction layer for Qt. It allows Qt to interact with different window systems, e.g., X11, Wayland, or Win32.
– ‘Xcb’: X protocol C-language Binding (xcb) is a replacement for Xlib featuring a small footprint, latency hiding, direct access to the protocol, improved threading support, and extensibility.
When you get the error ‘Qt6: Qt.Qpa.Plugin: Could Not Load The Qt Platform Plugin “Xcb” in Even Though it was Found,’ it implies that there’s an issue with the linkage between your Qt6 application and the ‘xcb’ plugin required for creating windows in X11 environment. This typically happens when:
1. Appropriate libraries for X Windows System are missing.
2. Software configuration issues disrupt the process from recognising the necessary plugins correctly.
Here’s how to solve these potential problems:
Solution | Steps |
---|---|
Install Necessary Library Files |
For debian-based distributions, install
libxkbcommon-x11-0 . Use below command:
From the official document of libxkbcommon (here) you can get this library package. |
Check LD_LIBRARY_PATH Environment Variable |
Verify that the xcb plugin directory is included in your LD_LIBRARY_PATH environmental variable. Here’s a brief code example:
LD_LIBRARY_PATH variable specifies the directories in which the shared library versions are being searched for first before looking in the standard locations such as /lib and /usr/lib. |
To identify whether this solution has rectified the error, try running your Qt application in the console and check if the specific error message has disappeared. Also, it would be best if you make sure that all Qt versions and plugins installed on your machine are consistent and compatible.
Learning to navigate around issues like these is central to coding in Qt. I hope this analysis helps in understanding and solving the `Qt.Qpa.Plugin` error message.
For additional insights, you might want to refer to these related sources:
– Qt Documentation on QPA (Qt Platform Abstraction)
– A deeper look at troubleshooting Qt errors from The Official Qt BlogThe error message,
Qt.Qpa.Plugin: Could not load the Qt platform plugin 'xcb' in '' even though it was found
, indicates that your application could not load the “xcb” platform plugin, which is an integral part of the Qt architecture.
In particular, the xcb component (X protocol C-language Binding) is essential as it operates as the bridge between Qt and the X Window System, allowing the graphical user interface to function correctly by dealing with system event handling such as inputs or outputs. Through using the XCB library, Qt can effectively communicate with the underlying X server that manifests itself through hardware devices, enabling your application on Linux-like systems to display content on the screen and respond accurately to user interaction.
The Role of the ‘Xcb’ Plugin in Qt
You might wonder why ‘xcb’ is required for a Qt application to work appropriately. Here are several key reasons:
- Rendering Graphics: The X Window System provides the basic framework for building GUI environments. XCB acts as the liaison, enabling Qt apps to utilize this framework.
- Handling Input/Output: XCB delivers events from the input devices to the application, like mouse clicks or key presses.
- Creating Windows: XCB helps in setting up the visual elements of the application such as windows, dialogs etc.
It’s clear that without an operative Qt Platform Plugin like ‘xcb’, your Qt applications would fail to render graphics or handle I/O effectively, leading to issues like the one you’re experiencing.
Troubleshooting the ‘Xcb’ Loading Issue
If you encounter the given error, inspect these potential problem areas:
1. Missing Dependencies
Check for any missing dependencies for the ‘xcb’ plugin. You can use the
ldd
command for this. It prints shared library dependencies which can be useful in debugging situations just like this one. For instance, the following line checks the dependencies of libqxcb.so (the dynamic library file related to the xcb plugin):
$ ldd /path_to_qt_installation_directory/plugins/platforms/libqxcb.so
If any “not found” dependencies appear, install them.
2. Environmental Variables
Make sure the environment variable
QT_QPA_PLATFORM_PLUGIN_PATH
is set correctly. If not, set it to your qt plugin installation path:
export QT_QPA_PLATFORM_PLUGIN_PATH=/path_to_qt_installation_directory/plugins
This ensure that Qt knows where to find its plugins.
3. Incorrectly Installed Qt Libraries
Reinstall all associated Qt libraries if you installed them manually. Alternatively, use a package manager like aptitude or YUM.
By considering these steps and understanding the role of the xcb platform, we acknowledge the significance of managing plugins in the complexity of a Qt application’s working environment. Thus, mitigating the risk of encountering similar errors and ensuring the smooth execution of your Qt application.
Certainly, as a professional coder, I am eager to analyze the common causes for “Could not load the Qt platform plugin xcb” in relevance to “Qt6: Qt.Qpa.Plugin: Could Not Load The Qt Platform Plugin Xcb In Even Though It Was Found”. This is a complex issue that several developers often face, particularly when dealing with Qt applications on Unix or Linux based systems.
Alright. Primarily, it’s necessary to understand that Qt is a cross-platform application framework widely used for developing application software that can be run on different software and hardware platforms. However, when it comes to “Could not load the Qt platform plugin xcb”, it basically suggests that your application is unable to find the required libraries to render the graphical interface. Interestingly, in our case, though the library seems to be found, it still can’t be loaded.
Let’s delve deeper into the possible reasons behind this anomaly:
Incorrect Library Paths:
To start, one of the most commonplace scenarios prompting this error is when your Qt application is unsure where to look for these needed libraries. Therefore, you may encounter this hiccup due to incorrect path variables.
Consider the example source code snippet:
export LD_LIBRARY_PATH= /usr/local/qt5/lib:$LD_LIBRARY_PATH ./YourApplicationExecutable
In the above code, we’re specifically directing the library search path into ‘/usr/local/qt5/lib’. However, if this isn’t where your ‘libqxcb.so’ (which is the xcb library) is located, you’ll likely face the said error.
Missing Dependencies:
Apart from the library path, your Qt application could also fail to load the xcb platform plugin due to lack of certain dependencies required by the libqxcb.so. This can include dependencies like libxkbcommon-x11 which are vital for the plugin.
For instance, consider utilizing ldd command to check dependencies:
ldd /path-to-libqxcb.so
Such a command would allow you to spot any missing dependencies for ‘libqxcb.so’.
Incompatible Versions:
Be aware that the xcb error might also surface due to version mismatch between the Qt libraries and the system’s xcb libraries. With differing versions, compatibility issues tend to spring up. A good rule of thumb would be to ensure all your libraries are compiled against the same Qt version.
Lack of Necessary QPA Plugins:
Given that the application is stating it found the plugin but couldn’t load it, it indicates the scenario where your Qt build might lack QEGLDeviceIntegration plugin. This warp over QPA plugins tends to cover xcb, so their absence could result in the recurrence of the error.
To illustrate, one way to ascertain the availability of QPA plugins is by setting the QT_DEBUG_PLUGINS variable:
export QT_DEBUG_PLUGINS=1 ./YourApplicationExecutable
In essence, this environment variable aid in printing debug information about each plugin as it gets loaded.
To resolve such issues, one might have to invest time in determining and duly addressing the underlying cause, whether it involves correcting the library paths, managing dependencies, ensuring correct Qt versions, or installing necessary QPA plugins.
For more insights regarding ‘Could Not Load The Qt Platform Plugin Xcb’, this resource may prove helpful. Always remember, such errors might initially seem daunting, but systematically troubleshooting them will significantly simplify the process.There could be numerous reasons why you’re experiencing loading issues with the Qt Platform Plugin “Xcb”. These usually come as a result of dependency mismatches or incorrect paths. The error message,
Qt.Qpa.Plugin: Could not load the Qt platform plugin "Xcb" in "" even though it was found
, indicates that your application is able to discover the Xcb plugin but for some reason can’t fully load it. The issue is quite common and reported among many users.
Let’s break down the potential fixes:
1. **Verify Installed Dependencies**
Ensure that all dependencies are correctly installed. Every software depends on certain libraries and packages to run properly. Just like that Qt needs xcb libraries to have a proper graphical interface. Use the following command to install these packages:
sudo apt-get install '^libxcb.*-dev' libx11-xcb-dev libglu1-mesa-dev libxrender-dev libxi-dev flex bison gperf libicu-dev libxslt-dev ruby libssl-dev libxcursor-dev libxcomposite-dev libxdamage-dev libxrandr-dev libdbus-1-dev libfontconfig1-dev libcap-dev libxtst-dev libpulse-dev libudev-dev libpci-dev libnss3-dev libasound2-dev libxss-dev libegl1-mesa-dev gperf bison libasound2-dev libgstreamer1.0-dev libgstreamer-plugins-base1.0-dev \| tee xcb.log
2. **Check the QT_PLUGIN_PATH variable**
There could be a mismatch in the PATH variables pointing towards the plugin directory. You need to make sure that the path variable
QT_PLUGIN_PATH
points correctly to the plugins directory associated with your Qt installation. Setting up this should help Qt find the required platform-specific plugins:
export QT_PLUGIN_PATH=/path/to/qt/plugins
Replace
/path/to/qt/plugins
with your actual path of qt installation.
3. **Using Static Build With All Plugins**
A quick workaround is compiling Qt statically with all the plugins. This will include all the necessary drivers during the build process and reduces runtime dependencies. However, keep in mind this might lead to larger binary file size.
4. **Recreate QT Configuration**
Another possible solution is by recreating the QT configuration using `qtchooser`. Following commands should do the trick for you:
sudo rm -rf /usr/lib/x86_64-linux-gnu/qt-default/qtchooser sudo apt-get install --reinstall qtchooser
5. **Export LD_LIBRARY_PATH Variable**
Sometimes exporting `LD_LIBRARY_PATH` to the correct library may solve this problem too. Execute the following command in your terminal:
export LD_LIBRARY_PATH=/usr/local/Qt6.0.0/lib
Replace `/usr/local/Qt6.0.0/lib` with your correct Qt version.
Please note that these are generic solutions and the actual fix can vary depending on the exact nature of your Qt environment and setup. For further assistance, it would be helpful to consider posting detailed queries within specific developer communities such as StackOverflow or the Qt Forums. Don’t forget to include important technical details about your development environment.
Besides, if you believe there’s an unresolved issue with the core Qt SDK, you might want to report it to their official bug tracking system at bugreports.qt.io.
Before trying these steps, though, ensure that any previous Qt installations or configurations are fully uninstalled and cleared out to avoid confusion for your system’s package manager.
Whether you’re a beginner in coding or an experienced coder coping with complex issues, you certainly appreciate step-by-step guidance. This particular case revolves around the issue of manually loading a plug-in within the QT6 environment, particularly in relation to the Qt.Qpa.Plugin: Could Not Load The Qt Platform Plugin Xcb In Even Though It Was Found error message. Here is all the information you need.
The QT Libraries, an open source software development toolkit, provides developers with numerous functionalities, with some of its most useful components being platform plugins. Note that these are dynamically loaded libraries that act as a bridge between the client application and the graphical interfaces of operating systems.
One key plugin in question here is the XCB (X protocol C-language Binding), which is a replacement for Xlib featuring a smaller API yet full protocol handling.
If you’re encountering the ‘Qt.Qpa.Plugin: Could Not load the Qt platform plugin “xcb” error,’ it indicates there’s a problem with the XCB library linked to your Qt installation. Usually, this happens when you miss out on installing the XCB library during setup or if the system paths are not rightly configured to reference the place where the XCB library resides.
Here’s how you can attempt to manually load a plugin:
export QT_DEBUG_PLUGINS=1 ./application
This helps you debug plugin loading problems. It may provide enough insight for you to fix the error on your own. However, it can also return something along the lines of
“QLibraryPrivate::loadPlugin failed…”. Which usually indicates that dependencies required by your plugin have not been met.
As such, one other way to resolve this issue is to ensure that all relevant dependencies are readily available. For Linux based systems, try the following:
sudo apt-get install "^libxcb.*" libx11-xcb-dev libglu1-mesa-dev libxrender-dev
For Redhat based systems, execute this line:
sudo yum install "^libxcb.*" libx11-xcb-dev libglu1-mesa-dev libxrender-dev
Another potential solution revolves around ensuring your path environment variable recognizes the specific path where your XCB plugin lies. If not, configuring the LD_LIBRARY_PATH to reference the XCB path might help.
export LD_LIBRARY_PATH=/gcc_64/lib
Remember to replace
Admittedly, environmental factors differ so markedly that it isn’t feasible to provide a one-size-fits-all answer to this problem. Depending on the nuances of your operating system, compiler, source control system, and many other settings, you’ll likely need to tweak these instructions here and there.
Last but not least, remember the Qt documentation on deployment. It provides comprehensive insights on plugin troubleshooting, helping you understand the ins and outs of manually loading plugins.A common solution to solve the
Qt.Qpa.Plugin: Could Not Load The Qt Platform Plugin "xcb" In even though it was found problem
is updating or reinstalling your software packages. This problem typically arises when there’s an issue with Qt’s platform support code, specifically in relation to the XCB plugin which the Qt library uses for rendering GUI elements.
An effective remedy begins with a proper understanding of the underlying issue. Here, the issue implicates that although the XCB plugin was found, it couldn’t be loaded by the Qt platform. Thus, it unveils a gap between the location and loading mechanism of this plugin. The plugin likely lands up in a spot where the Qt platform is unable to access it or there may be missing dependencies contributing to its failure to be loaded.
To address this issue head on:
– The first stop would be to update your software packages. Updating these packages ensures that you possess the most recent versions with all the relevant bug fixes thereby enhancing the operational flow. A simple usage of
sudo apt-get update
and then running
sudo apt-get upgrade
for Ubuntu/Debian based systems, or
brew update && brew upgrade
if you use homebrew on MacOS could very well do the trick.
– Second, if updating doesn’t solve the problem, try reinstalling the concerned packages which in this case are
libxkbcommon
and
libxcb
. You can accomplish this using
sudo apt-get install --reinstall libxkbcommon libxcb
for Ubuntu/Debian or
brew uninstall libxkbcommon && brew install libxkbcommon
following
brew uninstall libxcb && brew install libxcb
for MacOS users.
However, should this continue to fall short, consider some alternative solutions:
– Further, you might want to examine and confirm whether all the required dependencies have been installed and they function as intended. You could run
ldd /path/to/your/qt/platforms/libqxcb.so
to check dependencies associated with XCB platform library of Qt.
– Moreover, check and make sure you have the correct PATH variables set such as
QT_PLUGIN_PATH
and
LD_LIBRARY_PATH
, as incorrect paths could lead to inaccessible plugins.
Following these guidelines, you’ll be able to mitigate the problem at hand effectively. With regular updates and a keen eye for verifying requisite dependencies, this error can be surmounted successfully.
Remember, understanding the problem rightly precedes fixing it accurately! Happy coding!
Reference:
Qt DocumentationDelving into the discussion on ensuring appropriate compatibility between system libraries and Qt version let’s pay close attention to the error “Qt.Qpa.Plugin: Could not load the Qt platform plugin xcb in even though it was found.” This is commonly faced by developers working with the Qt6 framework. There is quite a bit we have to unpack to fully answer this, but let’s break it down into manageable parts.
Qt stands for “cute” and it’s a free and open-source widget toolkit for creating graphical user interfaces as well as cross-platform applications that run on various software and hardware platforms. It offers a wide range of different features such as native look and feel on all supported platforms, excellent support for internationalization, advanced 2D graphics and even some 3D graphics, and support for scripting through ECMAScript (JavaScript). However, like any other framework or toolkit, Qt has its share of quirks and potential roadblocks. One of these hurdles you may encounter when developing with Qt is the happenstance of system libraries being incompatible with your chosen Qt version, which manifests as the afore mentioned error.
qt.qpa.plugin: Could not load the Qt platform plugin "xcb" in "" even though it was found.
The “xcb” here refers to X protocol C-language Binding, a library that connects the X server and the application operating in the client-server model. When this issue arises, it implies the specific Qt platform plugin, in this case “xcb”, failed to load despite being detected by the system.
So, how do we solve this? There are several approaches one can take to rectify this problem:
• Verify the installation: Make sure that the application is correctly installed along with all the dependent packages related to xcb. For instance, for Ubuntu versions, you might need the following libraries libxcb1, libxcb-render0 and libxcb-shape0. With Debian-based systems, use commands similar to:
sudo apt-get install '^libxcb.*-dev' libx11-xcb-dev libglu1-mesa-dev
• Relying on strace tool: The strace tool could help identify what files the application is trying to access. Running `strace ./application` might help highlight any missing libraries.
• Using ldd tool: The Linux ‘ldd’ command could shed light on shared library dependencies. Applying `ldd /path/to/your/application` would particularly be helpful in seeing any “not found” libraries.
• Check Environment Variables: The appropriate environment variables must be set. QT_DEBUG_PLUGINS=1 is an important variable for checking if there’s a problem loading plugins at runtime.
export QT_DEBUG_PLUGINS=1 ./your_application
• Matching System Architecture: The architecture of the system libraries should match the architecture of your application. Thus, if your application is 32-bit, you’ll require 32-bit versions of your required libraries.
• Rebuilding QT: In extreme cases where none of the above steps work, there might be an inherent issue with the installed QT itself. Uninstalling and rebuilding QT might resolve the problem effectively.
Hopefully, the step-by-step approach outlined above provides you with an understanding of how you can ensure appropriate compatibility between system libraries and the QT version when encountering this specific “xcb” loading issue. This way, you can guarantee a smoother development experience when working with graphical user interfaces within the feature-rich Qt framework.
For more detailed technical knowledge and guide, I recommend visiting the official Qt website and the documentation section revolving around debugging techniques.
In the vast world of codes and binaries, errors like these are almost unavoidable. Yet, they are just mere stepping stones which enhance our understanding and acumen as developers.
Stable releases and development releases are two significant phases in the software production cycle. At each phase, errors may occur, such as the Qt6: Qt.Qpa.Plugin error that can keep recurring even though the platform plugin xcb is seemingly found.
Let’s discuss these concepts in depth and explore how you can resolve this specific error.
The Difference Between Stable Releases & Development Releases
Stable releases are tested versions of software that have been through rigorous checks and validations. They’re often utilized in production environments because of their reliability. On the other hand, development releases (also known as beta, alpha, or pre-release versions) are still under testing and development. These versions often contain new features but may also carry undiscovered bugs.
- Stable Release: Your best bet if you prioritize a hassle-free user experience over having the latest features. Developers squish known bug issues and the release undergoes thorough testing before it hits the market.
- Development Release: Carries the appeal of new features. Some users are willing to deal with probable bugs for early access to new updates. Developers appreciate community feedback on these versions to help rectify errors before the stable release.
The Qt platform plugin “xcb”, which is fundamental for windowing system integration, can lead to some trouble across both types of releases. If you launch applications without initiating this plugin, your application might fail to run and trigger the error message we are discussing.
Resolving the Error
The error
Qt6: qt.qpa.plugin: Could not load the Qt platform plugin xcb
, despite the plugin ostensibly being found, tends to surface when the environment lacks specific libraries essential for executing the plugin. Here’s what you could do about it:
- Rerun your application after root permission. The command
sudo ./your_app_name
should be useful.
- If the problem persists, you could set an environment variable to check plugins with the following piece of code:
export QT_DEBUG_PLUGINS=1
. After setting up the environment variable, execute your application again, and observe the debug information which should point towards any missing dependencies.
- Now install these missing dependencies. Suppose the missing dependency is libxcb, then you will need to use the respective package manager to install it. For example, if you are using Ubuntu, the installation could be done with the command: `sudo apt-get install ‘^libxcb.*-dev’
.
Phase | Reliability | Bug Situation | User-Type |
---|---|---|---|
Stable Release | High | Most Squashed | Common Users |
Development Release | Lower | New possible Bugs | Developers / Testers |
Remember, always ensure you operate with the latest stable version when working on important projects. An entry-level approach to resolving errors like these includes updating all packages, taking care of imports, ensuring the correct libraries exist on your system, and checking file paths.
Handling application dependencies effectively while managing different distributions can act as a preventative measure.
If you’re developing a project intended for a large number of users, consider providing a bundled application, i.e., your software with all its dependencies, to make distribution easier and independent of specific library-versions on users’ systems
Working around setting environmental variables is crucial for trouble-free execution and this often presents a significant challenge, especially when dealing with Qt6. Let’s talk about the Qt.Qpa.Plugin error where you cannot load the Qt platform plugin xcb even though it was found.
The aforementioned error is an environment configuration issue that commonly arises during the linking phase of the Qt application build process. The reason for this error is typically due to incorrect library paths set within the environment, causing conflicts or pointing towards non-existing libraries.
- You need to ensure that the right versions for all required dependencies have been installed correctly in your system.
- You then have to set correct library path in LD_LIBRARY_PATH environment variable. You do that by using the command:
export LD_LIBRARY_PATH=/path/to/your/library
Replace /path/to/your/library with the actual location where the libraries reside.
Note: This modification would only affect the current terminal session. To make them permanent, you’d have to add this line to your .bashrc or .bash_profile file(depending on your operating system).
If the problem persists after settings the LD_LIBRARY_PATH correctly, the issue might be that other required plugins are missing or not found by Qt. In that case, we recommend specifying the location manually through the QT_PLUGIN_PATH environment variable.
You can manage this via the command line by:
export QT_PLUGIN_PATH=/path/to/your/plugins
This defines the search path which Qt uses to find its plugins.
Environment Variable | Description |
---|---|
LD_LIBRARY_PATH | This specifies the directories in which to search for libraries at execution-time. |
QT_PLUGIN_PATH | This specifies the additional locations to search for plugins for loading dynamically at run-time. |
In some cases, specifying just the QT_PLUGIN_PATH doesn’t help and you also have to set another variable called QT_QPA_PLATFORM_PLUGIN_PATH:
- Using the command line:
export QT_QPA_PLATFORM_PLUGIN_PATH=/path/to/platforms
More information on Deploying an Application on X11 Platforms
Error-free execution of any Qt-based application lies in proper setting of relevant variables and ensuring that missing plugins have been accounted for. All efforts should aim at attaining a well-configured Qt environment for smooth running of applications.
Taking into account the error message `Qt.Qpa.Plugin: Could Not Load The Qt Platform Plugin Xcb In Even Though It Was Found`, this typically stems from an issue of misconfiguration or absence of some necessary libraries within your development environment.
Upon encountering this message, the first thing as a developer you’d desire to scrutinize is the statuses of your XCB libraries. These libraries leverage, interface, and package the X11 protocol in C++ applications like those powered by Qt6. Their significance cannot be underestimated. To check their statuses:
sudo apt-get install '^libxcb.*-dev' libx11-xcb-dev libglu1-mesa-dev
The command above will prompt the system to interactively reinstall all the required `XCB` and related libraries. Another plausible reason behind the snag could be linked to the `qt.qpa.plugin` path wasn’t correctly set when compiling against `Qt`. Therefore, taking time to enquire if the compiled plugins are accessible at their appropriate location is crucial Linux for Embedded Systems.
Adding:
export QT_DEBUG_PLUGINS=1
to your shell startup file can assist throw more debug information. This might present insights on what precisely is going awry.
If all else fails, consider redundantly installing the qt platform plugins:
sudo apt-get install qt5-gtk-platformtheme
You’ll likely have to overlay specific paths suitable for your own setup. However, once properly configured and loaded, the XCB platform plugin should then function seamlessly, eliminating the problem. One more approach entails explicitly setting the `QT_QPA_PLATFORM_PLUGIN_PATH` environment variable, like so:
export QT_QPA_PLATFORM_PLUGIN_PATH=/usr/lib/x86_64-linux-gnu/qt5/plugins/platforms
Again, the exact directory in the above export command example would depend on your specific installation. These important measures should help eliminate the common `Qt.Qpa.Plugin` error and enable your `Qt6` applications to smoothly run using the `Xcb` platform plugin. For more details, visit this page .