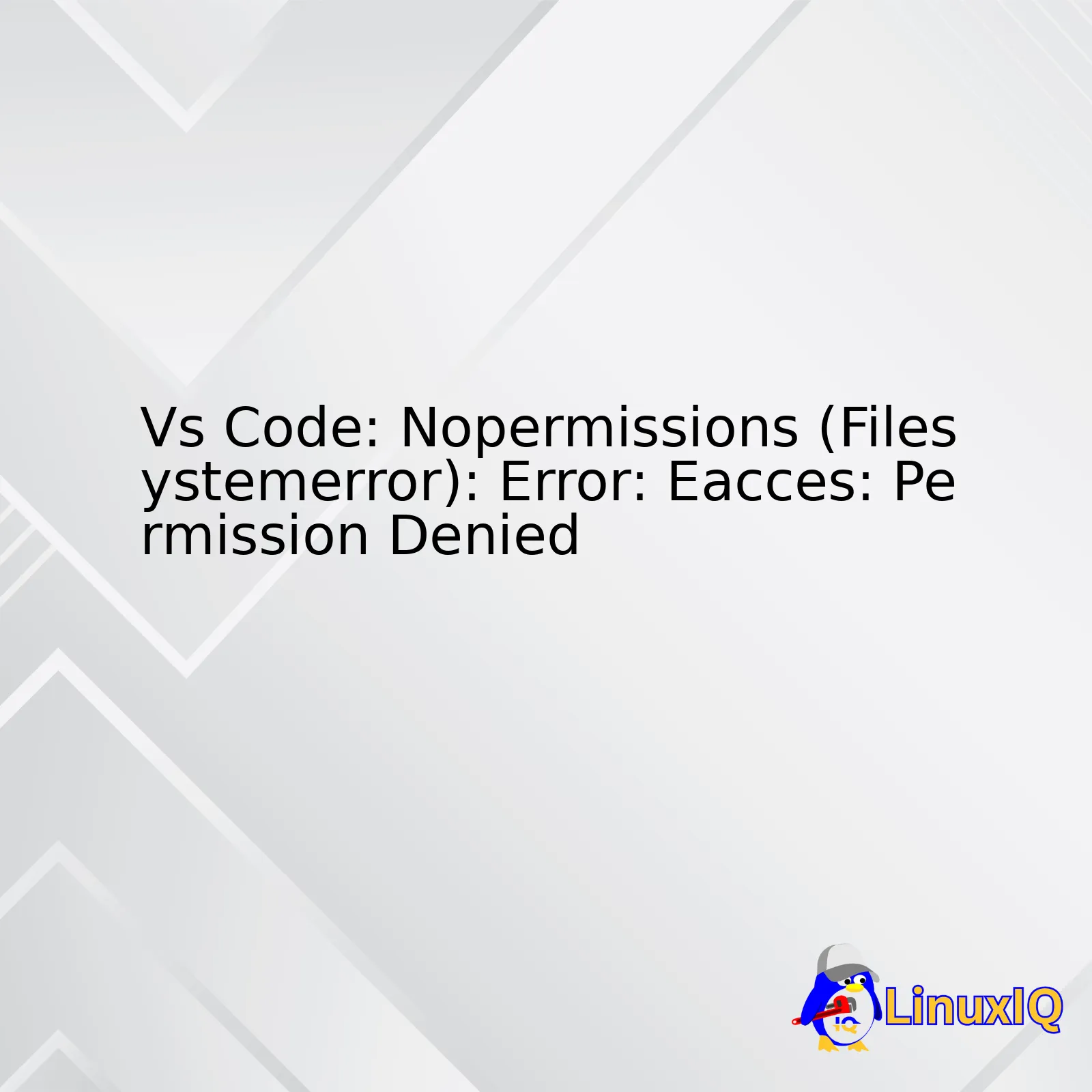
Error Type | Description | Possible Causes | Solutions |
---|---|---|---|
Nopermissions (FilesystemError): Error: EACCES: Permission Denied | A system-level error indicating failed access requests on a certain file or directory. | 1. VS Code does not have necessary permissions. 2. A system-level process may have changed permissions. 3. Operation performed beyond current user’s authority. |
1. Alter permissions using chmod command. 2. Run VS code with super-user (sudo) privileges. 3. Verify/install latest VS Code version. |
In terms of relevance to nopremissions filesystemerror in VS Code, it’s easy to forget how many complex operations we delegate to our coding environments these days. With ease comes complexity, and with that complexity sometimes come errors like these.
When VS Code throws an EACCESS error, it’s saying “I tried to do something (like open, read, or write to a file), but the operating system didn’t let me”. It could be due to restricted user rights, or running an operation that is outside the scope of what the current user is authorized to do, among other reasons.
One typical solution is changing the permissions of the file or directory VS Code is trying to access. By switching to the terminal in VS Code, you can navigate to the problematic file or folder and change its permissions using the chmod command, which modifies file access rights. While exact syntax can vary, a typical command might look like:
$ chmod +rw /path/to/your/file
Running this command will grant read-write permissions to all users. Note that ‘chmod’ commands should be used judiciously – ensuring they aren’t providing unnecessary access can help maintain your system’s security.
Alternatively, you could potentially solve the issue by running VS Code with superuser (admin) privileges. On a Unix-like platform, that would involve launching VS Code via the terminal using the sudo command. However, use sudo with caution, as it gives VS Code unrestricted access to your system files.
Lastly, always ensure that you’re running the latest version of VS Code. Updates are regularly released to handle bugs and improve functionality, and these include patches related to issues like access permissions. Visit VS Code download page to get the latest version.
These strategies should provide a road map to dissecting and addressing most instances of the nopremissions (FilesystemError): Error: EACCES: Permission Denied issue in VS Code.Ah, the dreaded
Error: EACCEs: Permission Denied
. This error typically springs up when you are trying to open a file or directory and you do not have proper permission to do so. Every file and folder on your computer system has an associated set of permissions that determine who can read, write, execute, or delete that file. If you’re not the owner of the file, or part of a group with suitable permissions, you could face this roadblock.
Let’s dive deeper into understanding what’s causing this, and how it can be remedied. One of the primary causes is inappropriate file permission levels. In Unix-like systems, three types of permissions exist – Read (r), Write (w) and Execute(x). These can be assigned for:
* The file owner.
* The group owners.
* Other users.
To set these permissions effectively, we use the
chmod
command, followed by a three-digit number representing each user’s access level in binary format. A handy reference table is as follows:
Numerical value | Permission |
---|---|
0 | No Permission |
1 | Execute |
2 | Write |
4 | Read |
7 | Read, Write and Execute |
Now let’s say you are facing the EACCES problem. Here’s an example that illustrates the appropriate course of action:
You navigate over to your VS Code terminal and enter:
ls -l /path/to/your/folder/or/fileThe console returns something like:
-rw------- 6 username staff XYZ ABC .07 10:50 fileName
This means that only the ‘username’ currently has read and write permissions for ‘fileName’. No other group or user has any permissions. Hence, if another user tries to run VS Code to modify this file, they will run into our notorious friend – the
Error: EACCES
.
You can resolve this issue simply by changing the file permissions. You might adjust permissions like so:
sudo chmod 664 /path/to/your/folder/or/file
Now when you recheck file permissions with
ls -l
, you should see:
-rw-rw-r-- 6 username staff XYZ ABC .07 10:50 fileName
This signifies both the owner and the group now have read and write privileges. Other users can read the file but cannot alter it.
If concerns about security prevent you from extending file permissions, consider changing the ownership of the file using the chown command:
sudo chown $USER:$GROUP /path/to/your/file
$USER and $GROUP need to be replaced with the specific usernames and groups that should take control of the file respectively.
Implement these straightforward solutions, and the annoying EACCES error message will cease to haunt your coding hours! Remember, to handle file permissions and ownership smartly, keeping the spectrum of potential threats in mind. Refer to official Linux manuals for detailed understanding and intricate control over files and directories.The Permission Denied or Eacces: Permission denied error in Visual Studio Code (VS Code) typically arises due to issues with file permissions. Here are a few common causes and their solutions.
Running VS Code as a regular user trying to access a root-owned file:
Suppose you’re running VS Code in an average user mode. Restricted file system permissions can occur when the current user doesn’t have enough privileges to access a particular directory or file. This is quite often when you open a root user-created (owned) file with your regular user in VS Code.
To resolve this issue, one solution is to change the file ownership from root to your user. On UNIX-like operating systems, this can be done using the chown command. It’s a command-line utility for changing the owner of a file or directory. Below is an example where USERNAME is the username, and FILENAME is the name of the file:
sudo chown [USERNAME] [FILENAME]
Note that misuse of chown can lead to serious system malfunction. So, ensure you understand the consequences before using this command. For more on file permissions, you can also refer to the Wikipedia article on this topic.
Execution Permissions:
If you see ‘Permission Denied Error’, it’s also possible your script lacks execution permissions. Unix-like os assign different permission types for each file: read, write, and execute permissions.
You can add execute permissions to your files using the chmod utility by entering the following line in the terminal:
chmod +x [FILENAME]
This command grants the execute permission to the file, allowing it to be executed.
Parent Directory Issue:
Another typical cause pertains to the parent directory’s permissions. If any parent directory of the file you’re trying to access has restricted permissions, you might face this error.
You’ll need to update the directory permissions to solve this. Here is a standard command to modify directory permissions:
chmod 755 /path/to/directory
This command sets read and execute permissions for everyone and also writes and execute permission for the owner of the file.
Remember, VS Code is a powerful tool used by developers worldwide. However, it must be given the correct permissions to access and manipulate files on your system effectively. Always verify that you have the necessary permissions to access specific directories or files when working with VS Code, as most of these ‘Permission Denied’ errors come down to simple authorization issues.
Finally, remember that changing permissions can impact system security, always be aware of potential risks before modifying file and directory permissions. Consider consulting documentation such as the official Linux man page for chmod to understand how these changes may affect your system.Certainly, addressing VS Code FileSystemError can be quite challenging, especially in situations relevant to “Vs Code: No permissions (FileSystemError): Error: EACCES: permission denied.”
Manually modifying the permissions of the applicable directories or files is the most immediate approach. This involves using
chmod
and
chown
commands, primarily utilized for altering file permissions. Here’s an example of how one can use them:
To modify user-level permissions:
sudo chown -R $USER <path/to/the/directory>
For group-level permissions modification, one might execute:
sudo chmod -R 777 <path/to/the/directory>
Running vscode as root is another effective solution. You only need to preface your command with sudo like this:
sudo code --user-data-dir="~/.vscode-root"
The first solution caters more to permissions at the user level and functions by changing the ownership rights to your user account. The second directs its attention to file level permissions by assigning read, write, and execute abilities to everyone. Consequently, both eliminate the EACCES error (as long as you’ve correctly specified the file pathway in each situation).
However, these solutions are recommended cautiously. Assigning permissions in Linux is a sensitive task. In many cases, overuse of ‘777’ permissions or administering applications as root may paint a large red target for potential security threats.
If manual editing doesn’t feel right, you could utilize an external extension to overcome the issue. There is a range of third-party extensions available which cater specifically to this problem. This tool, for instance, offers fine-tuned permission management controls, making it easier to handle folder and file permissions without having to step out of the comfort of your IDE.
Every so often, FileSystemError roots itself from problems unrelated to permissions. Remember that your troubleshooting ought to involve verifying whether Visual Studio Code is updated to the latest version. You’ll come across occurrences where File System errors were resolved after installing the newest update.
Aside from updates, Always be sure to clear your workspace data regularly. It’s easy to end up with a clogged-up workspace that’s bubbling with FileSystemErrors underneath the surface. Navigate to
File > Close Folder
and then proceed to open it again. Doing so refreshes your workspace directory data and cleans things up a bit.
The main crux here is not to become daunted when you encounter the FileSystemError message. There’s almost always a logical explanation —and a reasonable fix— breathing behind the curtain of those intimidating error messages. So, keep calm and code on!
Rest assured, you’re not alone in this quest of yours. A whole community of fellow developers is standing by, ready to offer help and share their insights. Joining discussion forums such as Stackoverflow, subscribing to developer blogs, or participating in the Github Community are excellent ways of staying on top of possible FileSystemError encounters and their fixes, not just for VSCode, but any similar IDEs you might work with in the future.When working on a project using Visual Studio Code (VS Code), it’s highly likely that you’ve encountered an error like this:
VS Code: NoPermissions (FileSystemError): Error: EACCESS: Permission denied
This issue is usually rooted in not having appropriate permissions to read or write over files/directories and is referred to as an “EACCESS” error message. To put it simple, the EACCESS error is sent by the operating system to alert programmes whenever an operation being performed doesn’t have the necessary permission.
Digging into the technicalities of the EACCES error
The main causes for the EACCESS permission denied error are:
- The user is attempting to open a file/directory/write data, but they don’t have the needed access rights.
- The code is running inside a directory where the user has limited access rights.
- An attempt was made to open a shared file in write mode whereas it can be only opened in read mode
These scenarios typically arise when VS Code processes run with inappropriate user permissions. Here’s an example to illustrate this:
Suppose users A and B are working on the same machine. User A creates a folder, making them its owner who possesses all the required permissions. If User B tries to modify anything inside that folder through VS Code, that’s when the EACCES error is prompted.
Solutions
One common go-to solution is altering folder permissions using the chmod command. The following code snippet gives permissions (read, write, execute) to all users for the target folder:
sudo chmod -R 777 {path_to_folder}
Nevertheless, remember that this approach might impact your data security. You’re virtually giving every user on your system full access to this folder, which is ill-advised in production environments.
Hence, an optimal way to deal with this is to change the ownership of these folders/files to the user running the VS Code application. Use the below command:
sudo chown -R $USER:$USER {your_directory_path}
In most cases, using sudo while running programs fixes the EACCESS error because it elevates your permissions to root. However, constantly using sudo isn’t recommended in production for security reasons.
Wrap-up – Remember, understanding how different operations require different kinds of access is crucial in troubleshooting such errors. You should grant minimal permissions adequate enough for performing the operations without exposing any security risks.
Relevant resources worth more insights on permission issues include:
Change Folder Permissions and OwnershipWhen it comes to working with local IDE (Integrated Development Environment) like Visual Studio Code, or VS Code for short, there’s an occasional likelihood you might encounters a
EACCES: permission denied
error. This kind of error is tied to something called ‘User Permissions’, which are a fundamental part of how computers restrict access to specific resources.
Think of ‘User Permissions’ as the digital equivalent of keys that let you access rooms in a building. Some keys allow you to open multiple doors (admin rights), while others would only allow access to one room (regular user rights). In a similar way, your operating system assigns different permissions to files and directories so only authorized users can perform operations like reading, writing, and executing.
In UNIX systems, permissions are divided into three classes:
- User (u): The primary owner of the file/directory.
- Group (g): The users who are members of the file’s group.
- Others (o): The users who are not the part of user’s group.
Each class has three type of permissions:
- Read (r): Provides the read access
- Write (w): Provides the write access
- Execute (x): Provides execute access
Now, coming back to the error at hand with VS Code –
nopermissions (filesystemerror): Error: Eacces: Permission Denied
. It’s essentially saying that you, as the current user, don’t have appropriate permissions required to perform the operation.
Let’s assume as an example we’re trying to create a new directory using VS Code in a location where our user doesn’t have write permission. When we attempt to create this folder, VS Code triggers an action that directs the system to create a new folder. But since our user lacks the right permission, the system declines and sends back an error message that reads ‘
nopermissions (filesystemerror): Error: Eacces: Permission Denied
‘.
A potential resolution to this is assigning correct permissions using the `chmod` command in terminal. If you’re facing this issue due to lack of execute permission on a certain script, you can grant it by typing in the terminal:
chmod u+x /path/to/your/script.sh
This gives the user (u) execute (x) privilege for the specified script.
Do remember though, understanding and altering User Permissions must be performed cautiously and accurately. Since they are designed to protect system assets, inappropriate changes may expose vulnerabilities in your system.
If you ever find yourself constantly needing root privileges to perform regular development tasks, it may be worth considering a change in your project’s directory structure or maybe utilizing a containerization platform like Docker to provide an isolated environment that mitigates permission-related conflicts.
For further reading, you might find this article titled “Linux File Permissions Explained : chmod :/ rwx” useful. It provides a comprehensive guide about user-class permissions on UNIX-based systems.Indeed, the ‘Vs Code: No permissions (FileSystemError): Error: Eaccess: permission denied’ issue is something many coders encounter. Let me dive deep into this topic and explain how Permission Protocol plays a pivotal role in either contributing to or resolving this frequent Denied Access Issue.
Vs Code: No permissions (FileSystemError): Error: Eaccess: permission denied
This error message typically appears when you’re attempting to open, edit, or execute a file that does not have enough permissions set for your user account in the UNIX/Linux operating system. Fundamentally, it’s about how the permission protocol of the underlying file system interacts with Visual Studio Code (VS Code).
The Permission Protocol is a strategy used by operating systems to secure data. In Unix-like operating systems, each file and directory is assigned three types of permissions:
- Read (r)
- Write (w)
- Execute (x)
These permissions are then set for three different kinds of users:
- The actual file owner (User permissions)
- The group that includes a number of logged-in users (Group permissions)
- All other users in the system (Other permissions)
Back to the
Error: Eaccess: permission denied
issue, if you’re getting this error, it means VS Code is attempting to carry out an operation it doesn’t have necessary permissions for. This can occur due to several reasons such as trying to save changes to a read-only file, attempting to execute a non-executable file, or opening a file located in a restricted directory, among others. Essentially, the Permission Protocol prevents unauthorized access, ensuring overall data security.
One way to troubleshoot this error involves altering file permissions using the ‘chmod’ command via Terminal, like so:
sudo chmod {permission} {path_of_file_or_directory}
Remember! Being properly informed about the Permission Protocol helps one understand these mechanisms behind our permission-related issues in VS Code. This understanding becomes crucial when you need to manage a sophisticated file system while preserving its security.
Let this serve as friendly reminder on how only to change file permissions if absolutely necessary – doing so indiscriminately could expose your files to potentially unsafe scrips, applications or users. [Check this detailed document](https://docs.microsoft.com/en-gb/windows/security/threat-protection/security-policy-settings/file-system) from Microsoft on FileSystem Security Policy Settings to ensure safety while tweaking permissions.
With corresponding knowledge and careful practice, we can smoothly navigate around Permission related issues, hence sustaining an optimal coding environment.Dealing with folder ownership issues can become an uphill task, especially while using Visual Studio Code (VS Code), which might raise a ‘No Permissions’ or ‘FileSystemError’: ‘EACCEs: Permission denied’ error. It’s crucial to understand the reasons behind this issue and find suitable solutions for the same.
The underpinning cause:
This error commonly sprouts due to insufficient permission levels offered to VS Code by your operating system. In general terms, it simply means that VS code is forbidden from accessing or modifying certain files/directories because the current user doesn’t possess the necessary permissions.source
How can you resolve this:
Resolving the issue involves following multiple approaches:
1. Running VS Code as a superuser or admin often does the trick. Please proceed with caution when utilizing this approach, as it gives complete access to your system and misuse could lead to undesirable outcomes. Here’s how you can run VS code as a superuser:
$ sudo code --unsafe-perm
2. Altering permissions of the concerned file/directory can alleviate the problem. You may change the owner or provide necessary permissions using Unix ‘chmod’ or ‘chown’ commands.
For instance, if you wish to change the directory permissions:
$ sudo chmod -R 777 /path/to/directory
To change the owner:
$ sudo chown -R $USER /path/to/directory
Also note that, overly permissive settings like chmod 777 might be seen as a potential security risk and hence should be used sparingly and cautiously.
3. You may employ Angular console, which tends to open up at superuser privilege level, thus leaving no room for the FileNotFoundError.
In summation, owning correct user permissions is fundamental to a smooth working experience with VS Code and preemptive measures in maintaining proper permissions significantly reduces the occurrence of such ownership issues. By recognizing the potential causes and applying effective solutions as listed above, one can escape the clutches of the frustrating FileSystemError.
As a programmer, you may sometimes encounter `FilesystemError: EPERM: operation not permitted` issues while using Visual Studio Code (VS Code). Here are effective debugging tips to help troubleshoot and solve this problem.
Understanding EACCES Error:
EACCES or the ‘Permission Denied’ error is an indication that your code or program does not have necessary permissions to read/write/execute a specific file or folder. It could be also be interpreted as ‘Operation not permitted’ in VS Code.
Debugging Steps:
1. Verify Your User Rights:
The user running the VS Code must have permissions for the directory where the files exist. You can use Linux terminal commands (`ls -l`) to check the permissions of the file/directory.
Use the following command replacing “filepath” with the path of your file:
<pre> ls -l filepath </pre>
2. Updating the File/Folder Permission:
If you identify permission issues, you might need to change the access level. Linux/Unix provides `chmod` command to modify file or folder permissions.
Use the following command replacing “filepath” with the path of your file:
<pre> chmod 777 filepath </pre>
Please note that setting the permission to 777 grants all rights (read, write, execute) to all users, which is often considered a security risk.
3. Running VS Code as Administrator:
In some cases, running VS Code as an administrator can resolve these issues. However, be mindful as this action might pose security issues if you aren’t cautious about the code you’re running in the editor.
4. Uninstall & Reinstall VS Code:
If none of the above solves the issue, try reinstalling VS Code completely. There might be some corrupted files causing issues. But before doing so, ensure that you back up any essential settings or configurations.
Remember, debugging involves iterative process of identifying the source of errors and implementing solutions.
Always refer to official documentation:
Github Issues Page for VS Code:
Even googling the specific error message can provide solutions posted by other developers who have faced similar issue. Persistence is key to successful debugging!
Let’s take a look at a real example with a table showing the command line inputs and outputs:
Command Line Input | Command Line Output |
ls -l /path/to/file |
-rw-r--r-- 1 user group 0 Jan 10 12:00 /path/to/file |
chmod 777 /path/to/file |
|
ls -l /path/to/file |
-rwxrwxrwx 1 user group 0 Jan 10 12:00 /path/to/file |
In the example, initial command detailed that the file did not have the right permissions for reading, writing, executing for all users. This was rectified using `chmod 777`. Always remember to exercise caution when changing permissions for sensitive directories or system files.
When dealing with solutions to the VS Code error “NoPermissions (FileSystemError): Error: EACCES: permission denied,” it’s crucial to understand the underlying concept of IHMACL – Identity and Access Management (IAM) and Access Control List (ACL) – that oversees file/folder access rights. This understanding will help us grasp why these kind of denied access errors occur and how they can be fixed within the context of your integrated development environment, Visual Studio Code.
Let’s dive right into it!
I. Unwrapping IAM and ACL
An IAM system is essentially a framework used to manage digital identities and their access in an organization. It provides an efficient way to identify, authenticate, and authorize individuals or groups to have access to specific applications, systems, or network resources source.
On the other hand, ACLs are lists that centralize and itemize who has permissions to various file system objects (files and directories). Each file/directory has an associated ACL that indicates which users or groups are permitted to execute, read, or write the object source.
II. What causes the EACCES: permission denied error in VS Code?
The error “NoPermissions (FileSystemError): Error: EACCEESS: permission denied” essentially means that VS Code is trying to access a file or directory but doesn’t have user permissions to perform the requested operation.
UI components of VS Code such as Explorer, Search, and SCM input validation may restrict access to certain files/directories due to IHMACL, resulting in this error. This often happens if you open or edit files as a regular user that requires administrator level permissions.
III. Ironing out the kinks – steps to resolve the denied access issue
While the precise steps to resolve this situation depend on the specifics of your system configuration and the file/file directory causing problems, here are some general troubleshooting actions.
1. Modify user permissions:
Firstly, try modifying the access rights for the problem file/folder using chmod command. For instance:
chmod 755 <filename>
This sets decent permissions (read, write, execute for owner and read/execute for group and others) on named file.
2. Use sudo:
If adjusting permissions doesn’t solve the problem, deploying escalated privileges might. Run Visual Studio Code with super-user permissions using the sudo command:
sudo code <filename>
Please exercise caution when working with sudo since it offers unrestricted access and potential to cause system wide changes.
3. Update VS Code:
There could be chances where an outdated version isn’t effectively catering to access controls. Regular updates ensure improved security measures and bug fixes.
Remember, understanding the integral role of IHMACL within a system gives insight on just how important access controls are for secure coding practices while solving errors like the EACCES: permission denied error in VS Code.
References:
Troubleshooting permission issues in Visual Studio Code (VS Code) often revolves around addressing the `FilesystemError: Error: EACCES: permission denied` error message. This error signifies that the VS Code does not have the required access rights to perform a certain operation on a file or directory.
You could be experiencing this error for several reasons, but it generally comes down to owner and group permissions, or even incorrect read/write permissions on files or directories within your file system. Here are some actionable steps to diagnose and hopefully resolve this kind of issue.
1. Check File Permissions with Terminal
From the terminal, you can use the `ls -l` command along with the problematic directory or file path as the argument. The output from this command will indicate filesystem permissions for each item.
$ ls -l /path/to/problematic/directory
Each listed line will look something like this: `-rwxr-xr-x 1 name group date filename`. The sequence `-rwxr-xr-x` represents the permissions for the file for different users:
* r = read permission
* w = write permission
* x = execute permission
The first character indicates the type of file (- for regular files), followed by three sets of permissions. The first set is for the user who owns the file, the second is for other members of the group that owns the file, and the third set is for everyone else.
Understanding whether the problem lies at the root level or subdirectory level will offer insights into which sections may need specific modifications.
2. Change File Permissions using chmod
If you determine that the permissions are incorrect, you would likely need to change them using the `chmod` command again via terminal. For instance, if you want to add read, write, and execute permissions to the owner of a file, you could use `chmod u+rwx`.
$ chmod u+rwx /path/to/problematic/file
Remember, modifying file permissions should be done cautiously, as erroneous configurations might harm your system’s integrity and security.
3. Checking the VS Code User
It’s important to check the user under which VS Code is running as that user needs to have appropriate permissions over the relevant files or directories. You can obtain the VS Code user by opening integrated terminal in VS Code (
Ctrl+`
) and running `whoami`.
$ whoami
Ensure that the user returned from `whoami` call has the correct permissions for the desired operations on the affected files or folders.
4. Consider Running VS Code with Superuser Permissions
There are cases where you may be required to run VS Code with superuser permissions using sudo. Please remember that executing VS Code as a superuser is a workaround and not a long-term solution due to possible security risks.
$ sudo code /path/to/directory
5. Disable Root-owned Files from Antivirus Scan
In some instances, antivirus programs interfere with permissions. You might consider adding root-owned files and directories to your Antivirus exclusion list just to check if they are causing such issues.
6. VS Code Extension Interferences
Last but not least, inspect your installed extensions. Some extensions may cause unexpected behavior in VS Code, including permission errors. Try running VS Code with all extensions disabled (use command
--disable-extensions
). If the issue disappears then one of the installed extensions may be the source of the problem.
$ code --disable-extensions
Mastering troubleshooting skills in combating VS Code permission errors enables you to understand better how file permissions work, improving efficiency and potentially saving development time. Remember to perennially test each solution in a controlled environment before integrating changes into a production setting. Be sure to also follow best practices for [secure coding](https://owasp.org/www-project-top-ten/) and handling file permissions.Unveiling the realm of root privileges and their formidable influence on command execution raises our understanding of the possibilities and challenges we face when dealing with denial of access issues in software development. This is especially pertinent to VS Code developers who encounter the FilesystemError: EACCESS Permission Denied error.
When it comes to developing or modifying software, root permissions matter significantly because they dictate who has the authority to perform specific operations within a system. These permissions play a critical role in defining what a user can do and cannot do inside a codebase.
$ sudo su
The ‘sudo su’ command, for instance, temporarily provides root-level privileges to a regular Linux user. With elevated privileges, the user can execute commands that would otherwise be inaccessible due to permission constraint.
VS Code, being a robust and versatile text editor, frequently requires these permissions to manipulate files, directories, and especially when running scripts that require high level permissions.
However, an incorrect configuration or denied access to essential data can result into the dreaded “FilesystemError: Error: EACCES: Permission Denied”. When a file system prohibits VS Code from performing certain tasks, this error surfaces indicating that VS Code does not have the necessary permissions to read, write, or edit a file or directory.
Two fundamental tactics can usually resolve this:
Alter Directory Permissions: Simply changing the permissions on your working directory could solve the problem. The chown command changes the owner of a directory and the chmod command modifies the permissions.
$ sudo chown -R $USER:$GROUP ./
$ sudo chmod -R 777 ./
Run VS Code as a Root User: An extreme measure but occasionally necessary, this approach runs VS Code with complete control over your files. However caution is advised as accidental deletion or modification could damage the system.
$ sudo code
Remember, with great power comes great responsibility. While executing commands with root privileges can overcome stubborn obstacles, misuse can lead to potential pitfalls, complete loss of data or compromise system security.
Take into account this dissertation presented at the University Of Michigan detailing the perils of running software as the root user.
For further clarity, Microsoft’s official documentation on Linux’s permission structure perfectly encapsulates how permissions work in a Unix-based environment and how rights can be adjusted to alleviate access constraints. Furthermore, peeking into the large amount of community-shared knowledge on StackOverflow, will give you more detailed solutions for different variations of access related problems.
As a coder, it’s crucial to ensure your code environments are secure from potential threats. This involves rigorous setup of effective firewalls, implementation of preventive measures against file system errors, and understanding how to deal with potential snags like the infamous VS Code: Nopermissions (Filesystemerror): Error: Eacces: Permission Denied error.
Effective Firewalls Setup
Setting up an effective firewall is a multi-step process that can be simplified into:
- Determine Your Firewall Needs: Start by examining your systems for potential vulnerabilities and craft a security strategy tailored to these needs.
- Pick The Right Firewall: There’s no one-size-fits-all solution when it comes to firewalls. For example, packet filter firewalls cut cost but for more in-depth screening a stateful inspection firewall may be needed. An application-layer or next-gen firewall could provide even more robust security.
- Configure Your Firewall Rules Correctly: Incorrect configuration often defeats the purpose of having a firewall at all. Ensure you block unsecured sources, services, IPs while allowing traffic to necessary ports.
- Consistent maintenance and updates: Keep your Linux iptables or Windows Defender Firewall patched to their latest versions and continuously monitor activity logs.
Preventive Measures against FileSystemError
Here’s where some knowledge on filesystem permissions becomes pivotal. A FileSystemError easily occurs when attempting to perform read/write operations without appropriate permissions. Employ the following strategies to prevent this:
- Set Adequate File Permissions: Assign just-required permissions to users with the LINUX chmod command or the windows icacls or chmod commands.
- Make use of Secure File Transfer Protocols: Use SFTP and SCP as these protocols don’t leave your sensitive data exposed during transfers.
- Create Back-ups Regularly: Establishing regular backups can help restore the system to a functioning state should a FileSystemError occur.
Facing Vs Code: Nopermissions (Filesystemerror): Error: Eacces: Permission Denied
Given adequate context of file system permissions and firewalls, let’s troubleshoot this VS Code error. It usually occurs due to lack of access permissions to open a specific file or directory in Visual Studio Code. Here’s what you need to do:
- Check for correct ownership: If you’re not the owner of the file/directory, you’ll need to take ownership as shown here:
sudo chown -R $USER:/path/to/the/file
- Correct Permissions: Ensure your user account(s) have adequate permission. You can set the permissions using chmod as follows:
chmod 755 /path/to/the/file
- Run VSCode with elevated rights: Last-ditch effort, launch VSCode with higher privileges using sudo if on Linux/ MacOS:
sudo code /path/to/the/file --user-data-dir='.'
Remember, it is essential to maintain the security integrity of the code environment. Forge ahead, employ effective firewall strategies, regularly check for FileSystemError prevention measures, and handle VSCode issues appropriately.
Here are some great reads to deepen your understanding:
Stateful Firewall,
SFTP vs SCP,
Permissions- chmod
and
more on chmod.
It is indeed important to understand both manual and automatic updates in Visual Studio Code (VS Code). Particularly, it becomes crucial when you are dealing with file system error tagged as:
EACCES: permission denied
. This error means that VS Code does not have the appropriate permissions to access specific files or folders. To resolve this error, you need to modify the file or directory permissions accordingly. We can use chmod command here.
Note: Granting full administrative rights to VS Code might solve the problem but it can pose a security risk. Therefore, be mindful of this aspect.
sudo chmod -R 777 /path_to_the_problematic_folder_or_file
When amending any software solution, it’s good to keep tabs on its versioning and updates. Speaking of updates in VS Code, you get two options: manual and automatic. By default, VS Code is set to auto-update.
Automatic Update:
The automatic update takes care of the issue where you don’t have to remember upgrading your software regularly. It keeps your code editor updated with new improvements and features. Occasionally, automatic updates also address specific bugs including those related to permissions. However, this could sometimes lead to unwanted behaviors, such as introducing new unseen bugs to your work environment, or undesirable changes in functions.
Using the VS Code settings, you can control how the editor receives updates:
{ "update.channel": "default" }
Manual Update:
Although automatic updates are beneficial, there are valid reasons to stick with manual updates:
• Some users prefer to schedule their updates precisely.
• Avoid immediate adoption of ‘breaking changes’ until they fit comfortably into their workflow.
• Manual update allows precision, particularly if a new feature/bug affects your coding environment directly.
You can choose to check for updates manually through the Help menu by **Help** > **Check For Updates**.
Accordingly, you will change your configurations inside user’s settings to:
{ "update.mode": "manual" }
Keep experimenting with both options. The choice between automatic or manual depends largely on how much control you want over your working environment versus how frequently you want to stay updated without taking any action.
Take a deep breath and dive deeper into learning more about VS Code configuration, updates and resolving permission issues in the [official documentation](https://code.visualstudio.com/docs). Remember, the understanding of your coding tools is just as essential as knowing how to code.Misconfiguration in our coding environment or system’s permissions can indeed lead to a ‘Permission Denied’ error, also known as EACCES, which effectively creates a denial of access situation. This issue is particularly relevant to VS Code users as they require the correct permission settings to properly operate their files and perform operations.
This error often surfaces due to inappropriate file and directory permission configurations that prevent VS Code from accessing the required data. You might experience this while trying to open, write to a file, or execute commands that require higher privileges than what you currently have.
Understanding basic permission levels
On Unix based systems, there are three basic permission groups:
- User: The person who owns the file.
- Group: Other files belonging to the same group.
- Other: Everyone else not falling into the above two categories.
Three kinds of permissions can be assigned to these groups – Read (r), Write (w), and Execute (x).
To look at these permissions in place, we utilize the command line utility
ls -l
, gathering output akin to:
-rw-r--r-- 1 user1 group1 size date filename
.
Here, we have user1 as the owner with read-write access (-rw-) , group1 having read access only (r–), and other users also limited to read access (r–).
Analyzing file permission errors and solutions
When it comes to VS Code running into an EACCES error, incorrect file permissions are usually the culprits. For instance, if your user does not have proper rights on a file, or if VS Code was run using a different user credential than the file ownership, these permissions conflicts could trigger an EACCES error.
To resolve this, you’d need to revisit and modify your permission settings accordingly. Using the
chmod
UNIX command can help adjust permissions. To grant all necessary permissions (Read, Write, and Execute) to a file owner, for example, you’d use
sudo chmod u+rwx filename
. Remember that such changes could pose security risks, so approach cautiously.
Avoiding directory issues
Directory misconfigurations can also lead to the EACCES error. If VS Code lacks appropriate access to a pertinent folder, or if nested directories where your project resides harbor inconsistent permissions, similar issues abound. Modifying directory permissions similarly can alleviate these concerns, like
sudo chmod -R u+rwx foldername
granting complete owner access to the target directory and sub-folders within.
Note that applying blanket modifications could result in security vulnerabilities, and special attention must be given to critical system files and folders. Only apply necessary changes and avoid propagating full access (-rwxrwxrwx) wherever possible – this helps reduce potential misuse by malicious applications or users.
In some cases, VS Code installation itself could fuel such errors. If installed through Snap on Linux, blame the default strict confinement policy that restricts accessibility to certain parts of the filesystem. In this light, consider alternate mechanisms for VS Code installation to side-step the issue.
Keep these considerations in mind to cut down misconfigurations and truly understand how they impact denial of access in VS code. This way, quick remedies come easy next time EACCES interrupts your coding sessions!
For more details __Techniques for mitigating permission issues__.
If you’ve been utilizing VS Code for some time, chances are you may have encountered the
VS Code: NoPermissions (FileSystemError): Error: EACCES: Permission Denied
, which likely suggests that your current user does not have sufficient permissions to access or operate on a particular file or directory. One plausible reason is tied directly to your operating system specifications, its inherent permission structure, and how these interact with file systems.
Operating systems like Linux, Unix, or macOS employ a permission-based model where each file or directory possesses certain permissions. These permissions govern what actions a user or program can undertake – read, write, or execute. The remarkable fact concerning these permissions is that they are determined by three entities:
- The owner of the file
- The group that the file belongs to
- Others (i.e., other system users)
Let’s say you’re trying to open a file in VS Code, and you get the error
EACCES: Permission denied,
. This could mean your user doesn’t hold the necessary permissions to read that file.
Thus, it would be useful to elucidate an actual chuck of code or command in terminal to rectify these issues. For instance, we can change the ownership of a file or directory with the
chown
command before opening it in VS Code:
$ sudo chown $USER:$USER /path/to/your/file
After running this command, your user should own the file/directory, thereby giving you full access. If the problem persists even after securing the ownership rights, then it’s likely related to insufficient group or others permissions. Try modifying the permissions using the
chmod
.
To give you an idea of what should be done, you can run the command:
$ chmod 744 /path/to/your/file
With
744
, the owner will have read/write/execute (7) privileges, while the group and others only have read permissions (4).
It’s essential to remember that incorrectly setting permissions can pose security risks or lead to system malfunctions, thus stressing the importance of thoroughly understanding these concepts. Refer to the Linux permissions guide for further details.
Opening file permissions might seem a quick fix at times, but inappropriate permissions can make your system vulnerable to attacks or corruption. As professional coders, we must always ensure that we are mitigating potential security threats while troubleshooting errors. Finding the right balance is often the key to successfully eliminating such file-access errors.
The connection between your operating system and VS Code workspace can greatly affect usability and productivity. Understanding it can be instrumental in improving your overall coding experience. Utilize the information provided to fully unlock the capabilities of your OS and your coding environment.
The
VS Code: NoPermissions (FileSystemError): Error: EACCES: permission denied
error message is commonly provoked due to issues related to permissions with your file or directory. Considering this, it highlights the importance of declarative configurations and access controls in coding environment setups such as Visual Studio Code.
In most cases, the issue stems from the inability of the VS code editor to access a particular file or directory due to restrictions. This reflects how robust system security can inadvertently turn into an obstacle when limitations become overly strict or misapplied:
- Conflicting permission levels: One common reason for this error popping up is conflicting permission levels between your user account and the file you’re trying to manipulate. Your account might lack the necessary privileges to write, read, or execute the file.
- User vs root conflicts: When files or directories are owned by the root user while you are operating as a regular user, problems, errors like this can surface due to the limitations associated with a lower privilege level.
To rectify this, we adjust the access permissions associated with a particular file or directory using commands like
chmod
and
chown
depending on your operating system. It’s crucial to not overlook these details in optimizing our development workflow.
// Changing file permissions in Linux or Unix systems $ sudo chmod 755 /path/to/your/file // Changing owner of the file in Linux or Unix systems $ sudo chown $USER:$USER /path/to/your/file
Note: Replace
/path/to/your/file
with the actual path to the file you want to change permissions for.
The process requires careful precision because overdoing it and providing unrestricted permissions can expose your files to security threats. Therefore, maintaining the correct balance in access control becomes significant.
Please refer to this link for further information about Visual Studio Code Permissions: stackoverflow vs code no permissions filesystemerror.
Greenlighting permissions consequently resolves this error, illustrating the weight of role-based access control (RBAC) which is indispensable in a secure coding environment such as Visual Studio Code. By understanding the interplay between user roles and permissions, we manage to safeguard our programs while ensuring efficient operation.