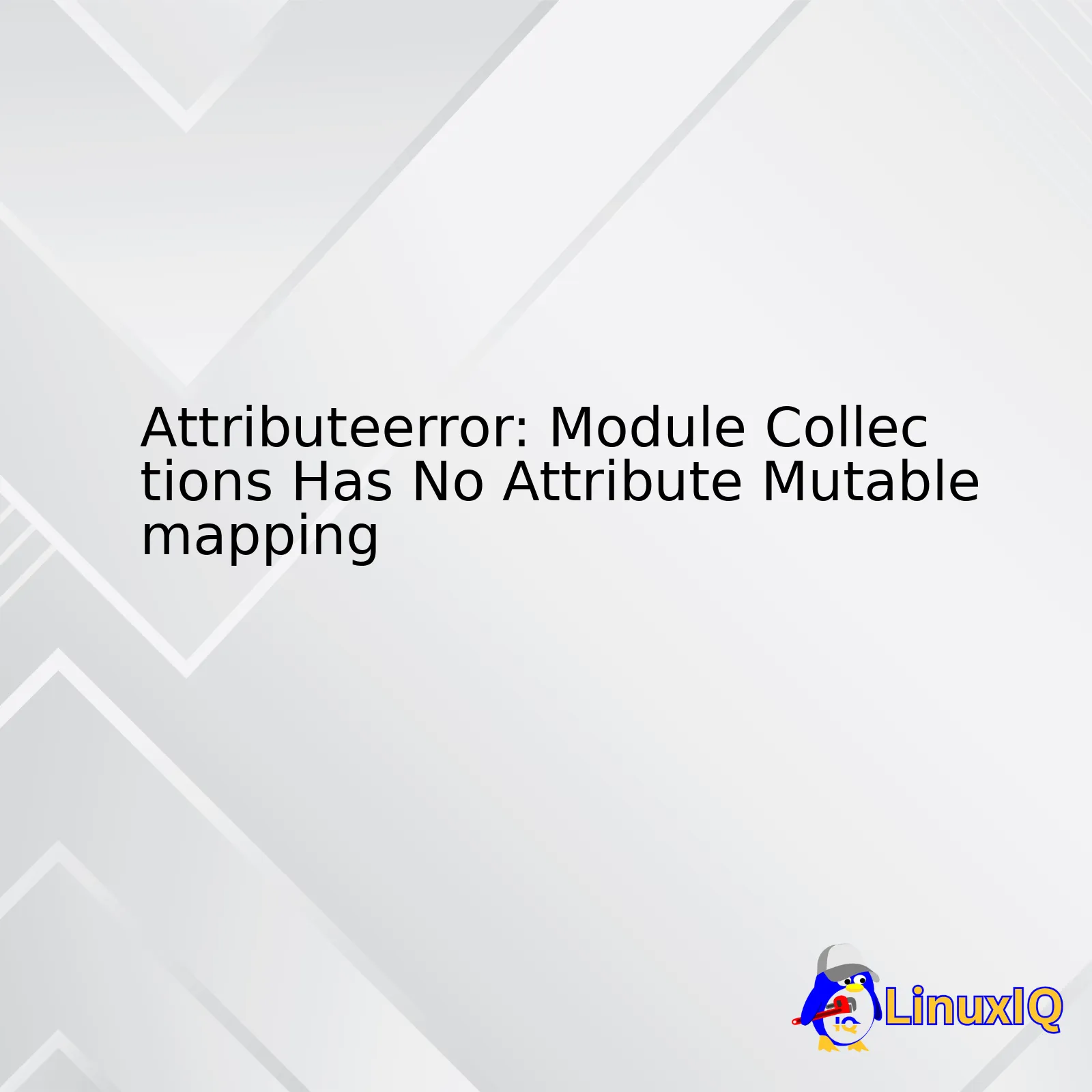
Error Type | Module | Attribute Not Found | Possible Cause | Solution |
---|---|---|---|---|
AttributeError | Collections | MutableMapping | Deprecation in Python 3.8+ | Use
collections.abc.MutableMapping instead |
The error occurs when trying to access `MutableMapping` from the `collections` module directly. In Python 3.3, `MutableMapping`, along with other abstract base classes, were moved under the `collections.abc` module for better structure and organization. However, to keep backwards compatibility with older versions of Python, they were still accessible from the main `collections` module.
With Python 3.8 and onwards, the import fallback has been taken out entirely, and trying to import `MutableMapping` from the `collections` module directly will result in an `AttributeError`.
To fix this issue, you need to change your import statement from
from collections import MutableMapping
to
from collections.abc import MutableMapping
. This ensures you’re using `MutableMapping` from the `collections.abc` module where it now resides following the deprecation.
Here’s how this looks in code:
Original (causes AttributeError in Python 3.8+):
from collections import MutableMapping
Updated (will work in Python 3.8+):
from collections.abc import MutableMapping
This change helps ensure the Python environment is more explicit about where different modules and attributes are coming from, promoting cleaner and more understandable code.
We can find details about this deprecation in the [Python documentation](https://docs.python.org/3/library/collections.abc.html#collections.abc.MutableMapping).
Always remember to update your coding practices as per the version of language/environment you are in. Happy coding!The
AttributeError
in Python typically arises when you try to access a non-existent attribute from a module, class, or instance. AttributeErrors such as
'module' object has no attribute 'x'
usually indicate a programming issue.
In your specific case, you are getting this error:
AttributeError: module 'collections' has no attribute 'MutableMapping'
.
The reason behind such an error is because `MutableMapping`, which is an abstract base class that provides a mutable dictionary-like interface and was accessed directly from the collections module, got moved under the collections.abc module with the release of Python 3.9. Now, it can no longer be accessed through the main “collections” module.
To resolve this specific issue, you should change your import statement. Instead of:
from collections import MutableMapping
It should be:
from collections.abc import MutableMapping
If you want to maintain compatibility with older versions of Python (versions below 3.3), you could use a try/except block:
try: from collections.abc import MutableMapping except ImportError: from collections import MutableMapping
This try/except code block essentially tries to retrieve the `MutableMapping` from the `collections.abc` module first but if that fails (as it would in older versions of Python) then it will try and import from `collections`. This ensures that the code continues to work across all Python versions.
TL;DR:
– This error is caused by trying to access the `MutableMapping` class from the ‘collections’ module whereas starting from Python 3.9, it should now be accessed from the `collections.abc` module.
– Use
from collections.abc import MutableMapping
instead of
from collections import MutableMapping
.
– To maintain backward compatibility with older versions of Python (their version being lower than 3.3), use a ‘try/except’ clause to alternate between importing from ‘collections.abc’ and ‘collections’.
For more details, you may refer to the official Python documentation on collections.abc .
Remember though, always keep track of deprecations and changes in Python modules. These changes are fundamental to prevent seeing these types of errors. Also, consider investing some time into understanding how to read and handle Python’s exceptions and error messages which is an essential skill for any Python developer.Diving deeper into the
Collections
module, we need to understand that it is a built-in Python library specifically equipped with alternative and efficient solutions for general data manipulation tasks. One of the specific areas in which you might occasionally experience issues is when trying to access
MutableMapping
from the
Collections
module.
There’s something important we need to consider here: since Python 3.3 and all subsequent versions,
MutableMapping
and all other collections Abstract Base Classes (ABCs) have been redefined within the
Collections.abc
module. Consequently, if you’ve come across the dreaded
AttributeError: Module Collections has no attribute MutableMapping
, don’t fret! It’s highly likely that this error is due to an oversight regarding these changes.
To resolve your current predicament, instead of using:
from collections import MutableMapping
What you should be doing is importing from
collections.abc
. Here’s how to correctly import
MutableMapping
:
from collections.abc import MutableMapping
Python’s documentation helpfully provides the ABC construct hierarchy [source].
A key point here is to ensure that you stay up-to-date with the ongoing changes and developments in the Python ecosystem. Keeping in mind Python’s mantra “explicit is better than implicit” [Zen of Python, PEP 20 (source)], transferring these abstract base classes into a separate module aids in enhancing code clarity.
Beyond the scope of
MutableMapping
, there are plenty more useful components within the
collections
module worth exploring. Some of them include:
–
namedtuple()
: Produces subtypes of tuples equipped with field names.
–
Counter
: A dict-like class for counting mutable objects.
–
OrderedDict
: Dict subclass remembering the order entries were added.
–
defaultdict
: Called missing value to supply a default value for the given key.
Each of their unique features enables developers to handle data structures more efficiently and elegantly.
Finally, great code isn’t only about achieving the desired outcome but also about adaptability, precision, resiliency. And understanding modules like
collections
thoroughly, including its dependencies, like the
collections.abc
module, will keep you right on track!Effectively combating the error message “AttributeError: Module Collections Has No Attribute MutableMapping” primarily means understanding what’s at the heart of it.
First things first, it is to be emphasized that an `AttributeError` in Python ordinarily occurs when you attempt to access a method or attribute that simply doesn’t exist for a specific object. (source)
class exampleClass: pass test = exampleClass() print(test.noMethod)
The correspondent script above ensues an `AttributeError`, precisely because `exampleClass` has no method named `noMethod`.
Turning our attention now onto `MutableMapping`. Essentially, `MutableMapping` is an ABC (Abstract Base Class) that’s introduced by collections module in Python. The purpose of an `MutableMapping` is to provide an interface for mutable mappings, which strive to maintain the key-value pairs where immutable keys are typically put into use.(source)
import collections my_dict = {} isinstance(my_dict, collections.MutableMapping) # this will return True
In the aforementioned code snippet, we’re checking if `my_dict` aligns as an instance of `collections.MutableMapping`. The output comes out as `True`.
However, bear in mind that MutableMapping and other ABCs have been relocated to `collections.abc` from `collections` quite a few versions ago (since Python 3.3 to be exact). From Python 3.8 onwards, you should import it from `collections.abc` instead of `collections`.
from collections.abc import MutableMapping
When using older Python versions that don’t acknowledge `collections.abc`, you’ll end up receiving the following error: `AttributeError: module ‘collections’ has no attribute ‘MutableMapping’`. And, in order to overcome it, always remember to update your Python version to the latest one available.
It’s important to grasp that understanding `AttributeError` and `MutableMapping` not only equips us with more detailed insights about the programming language, but also helps effective debugging, eventually leading to the crafting of robust and efficient software(source).
As you delve deeper into the Python language, you might encounter a mysterious error:
AttributeError: module 'collections' has no attribute 'MutableMapping'
. This error can feel bewildering at first, especially if you’re not familiar with the MutableMapping concept. But don’t worry – even the most seasoned coders are often taken by surprise by this!
To start, let’s clarify what MutableMapping is. MutableMapping is a part of the collections.abc library in Python. A MutableMapping is a dictionary-like object which has both settable and deletable keys but they can’t be enumerated. This is because the exact set of items in a MutableMapping may change during iteration over the set.
The reason why you seem to have run into this AttributeError is probably quite straightforward, either due to wrong syntax or outdated usage.
Firstly, check to confirm that you’ve imported the right library with
from collections.abc import MutableMapping
. And also remember to use the correct syntax when accessing it?
But if you’re doing all that right, and still getting this AttributeError, it’s likely your Python version might need an update. You see, prior to Python 3.3, abstract base classes were all too available under the collections module itself rather than being nested inside collections.abc. This has since been changed for organized hierarchial structuring and better maintenance.
This means if your code looks like:
from collections import MutableMapping
and you’re using Python 3.3 or later, you’ll need to modify that to:
from collections.abc import MutableMapping
Just one simple line of code might seem trivial initially, but can make a world of difference in streamlining your coding process, avoiding such AttributeErrors, and ensuring that your development workflow keeps up with the pace of advancing language features.
Learning about these idiosyncrasies of Python isn’t always easy but I promise, it’s worth it. Embrace this kind of learning experience—it’s all a part of the incredible journey of being a coder. After all, what’s important isn’t the roadblocks we face, but how effectively we learn from them and continue refining our craft.
Happy Coding!
Relevant Links:
– [ABCs documentation](https://docs.python.org/3/library/collections.abc.html)
– [Python docs on Exceptions](https://docs.python.org/3/tutorial/errors.html)Here’s how to decode the “AttributeError: module collections has no attribute mutablemapping” issue you’re experiencing in Python.
This error typically arises when you’re using a version of Python that is too old. The error message is telling you that, within the `collections` module of the Python Standard Library, there exists no attribute named `MutableMapping`. This might seem confusing because `MutableMapping` does exist in current versions of Python. In fact, it was introduced in Python 3.
Let me break it down:
•
AttributeError
: This portion of the error message is pretty straightforward. Python returns an `AttributeError` when you try to access an object or method that doesn’t exist on the current instance of a given class or module.
For instance, if you attempt to call a method on `None` (an instance of the `NoneType` class), you’ll get an `AttributeError`, like this:
None.my_custom_method()
•
module collections
: This specifies which module (in this case, `collections`) Python was trying to retrieve the attribute from.
•
has no attribute 'mutablemapping'
: Finally, this is telling you that Python failed to locate an attribute named `MutableMapping` within the `collections` module.
To solve your problem, you need to use the upgrade version of Python where `MutableMapping` exists. If you are below Python 3, I’ll recommend upgrading your Python to 3 and above.
Here’s an example of Proper usage of `MutableMapping`
from collections import MutableMapping class MyClass(MutableMapping): def __init__(self,**kwargs): self.__dict__.update(kwargs) ...
Once you’ve updated your Python, the `MutableMapping` will more likely found in its rightful place under `collections.abc` rather than `collections`. Nevertheless, `collections` will still refer to its subclasses for backward compatibility.
When correctly used, the above code will not raise any AttributeErrors. Feel free to check out Python’s official documentation page for more information about collections.Python’s official documentation.Ah, the classic “AttributeError: ‘module’ object has no attribute ‘MutableMapping'” error. It’s a common error encountered by Python developers who use the collections module intensively. But why do you encounter this error and how can you resolve it? Let’s dive in!
First, what is MutableMapping? The
Collections.MutableMapping
was a class in Python’s built-in library that provided all possible methods to a dictionary-like class. However, from Python version 3.3 onward, it was moved to a new location: the
collections.abc
module.
Hence, trying to import
collections.MutableMapping
from the collections module results in the error “AttributeError: module ‘collections’ has no attribute ‘MutableMapping'”. This is because ‘MutableMapping’ no longer exists in the collections module.
A solution is to replace your import statement. If your current line of code looks like this:
from collections import MutableMapping
Replace it with:
from collections.abc import MutableMapping
Doing this accesses MutableMapping from its new location,
collections.abc
instead of the
collections
module where it no longer exists.
Before Python 3.3 | After Python 3.3 |
---|---|
from collections import MutableMapping |
from collections.abc import MutableMapping |
By adhering to this small change, you resolve the aforementioned AttributeError effectively. Always remember, as software evolves, classes or modules are often moved to new locations to maintain organization and drive efficiency.
One important note here, according to the Python documentation in [Python docs], some services are reaching their end of life and will be removed completely from the future versions of python (specifically after Python 3.10).
So adding to our solution, starting from Python 3.10, you’d have to replace
collections.OrderedDict
with just
dict
, since
collections.OrderedDict
would be deprecated, but don’t worry,
dict
will keep the insertion order which is the purpose of
OrderedDict
anyway!
So understanding these potential shifts in the standard library allows you to future-proof your code and avoid facing similar AttributeErrors down the line. Happy coding!When coding in Python, we often import modules to gain access to a suite of functions and methods provided by the module. The attribute `
MutableMapping
` is an important element within Python’s Collections module; it allows us to create dictionary-like classes with added or overridden behaviours. However, there are instances when the error ‘
Attributeerror: Module Collections Has No Attribute MutableMapping
’ may arise – generally due to incorrect attribute use.
# Why Correct Attributes Are Vital for Successful Mapping?
Attributes serve as integral components when establishing connections between different data points. Developers rely on attributes to classify unique characteristics or features of a given entity. Here’s why such an attribute’s correctness is critical for successful mapping:
● **Avoiding Errors**: Ensuring correct attribute helps prevent runtime errors like AttributeError. This is directly related to our context where the error might be encountered due to the relocation of MutableMapping in Python 3.8+ versions. Hence, using the correct attribute will mitigate this error and ensure smooth operation.
● **Effective Data Mapping**: Correct attributes allow for effective data classification and organization, simplifying the task of data mapping through better identification and relationship establishment.
● **Performance Enhancement**: proper utilization of attributes helps optimize code’s performance by allowing swift retrieval, manipulation, and storage of data.
# How to Resolve The Error `’module’ object has no attribute ‘MutableMapping’`?
From Python 3.3 onwards, the abstract base classes like MutableMapping were moved to the collections.abc module. Therefore, to correct the `Attributeerror: Module Collections Has No Attribute MutableMapping` error, you should change this:
from collections import MutableMapping
into:
from collections.abc import MutableMapping
The shift from
collections
to
collections.abc
was made to align base classes with python language specification, segregating the concrete and abstract classes according to Python documentation.
To sum it up, understanding proper attribute usage according to the version of Python in use is pivotal to ensuring successful mapping. Anticipating and making necessary adjustments based on these changes would only enhance our code efficacy and maintainability, whilst navigating hurdles that may stem from unexpected updates like
'AttributeError: Module collections has no attribute MutableMapping'
.As you delve into the land of Python errors, one common issue developers often stumble upon includes a message like “AttributeError: Module collection has no attribute MutableMapping.” Essentially this statement implies that the specific module within “collections”, here “MutableMapping”, is inaccessible. The first stop in our investigation is visiting the reason behind such an error.
Python made a significant shift when it advanced from version 3.2 to 3.3. This transition engendered moving several modules from “collections” to a new module named “collections.abc”. Therefore, if your Python version is 3.3 or beyond,Python official source `collections.MutableMapping` has switched locations and is now available under `collections.abc.MutableMapping`.
You can validate this firsthand by executing these lines of code:
import collections print(hasattr(collections, 'MutableMapping')) # Prints False in Python 3.3+ import collections.abc print(hasattr(collections.abc, 'MutableMapping')) # Prints True in Python 3.3+
This exercise will verify the availability of ‘MutableMapping’ under both ‘collections’ and ‘collections.abc’, consequently confirming the output.
If you encounter a similar exception, the first solution consists of changing your import statement in accordance with the updated Python version. Here’s how you can go about it:
Replace:
from collections import MutableMapping
with:
from collections.abc import MutableMapping
However, if your codebase serves various Python versions, including ones below 3.3, here’s how you can ensure backward compatibilityPython documentation:
try: from collections.abc import MutableMapping # for python 3.3 onwards except ImportError: from collections import MutableMapping # fallback for python 3.2
The above approach captures “ImportError” exceptions triggered when ‘collections.abc.MutableMapping’ fails to import on old Python versions (3.2 or below) and instead attempts to import ‘collections.MutableMapping’.
Remember, staying informed about changes in programming language standards and keeping codebases up-to-date are fundamental responsibilities of a professional coder. Even whilst tackling issues like ‘AttributeError: Module Collections Has No Attribute MutableMapping,’ it’s crucial to maintain the code’s agility through practices such as backward compatibility.The
collections.MutableMapping
is part of Python’s collections module, a high-performance container data type, designed to extend Python’s built-in collection types like list, tuple, and dict. A fundamental understanding of MutableMapping enriches Python programmers exposure to abstract base classes (ABCs) and their inherent flexibility when incorporated into custom classes.
MutableMapping
is an ABC, providing a template for creating your dictionary-like classes with the required mappings’ methods. These include CRUD operations such as the ability to set, update, delete key-value pairs and read (retrieve) value by key.
If you encounter an error “AttributeError: module ‘collections’ has no attribute ‘MutableMapping'”, it implies that you’re attempting to access the
MutableMapping
class from the
collections
module wrongly or it can’t be found.
A common cause is the use of obsolete Python versions. Starting with version 3.3, Python began a process of migrating certain modules in the collections package to the collections.abc package. This includes the MutableMapping class. If you’re using a Python version 3.3 and later, you should import
MutableMapping
thus:
from collections.abc import MutableMapping
But if your system uses Python version 2.7, then the right protocol would be:
from collections import MutableMapping
Failure to adhere to these changes across Python versions would trigger the AttributeError mentioned above.
Now, how does
MutableMapping
earn its prominence?
It does so based on the following abilities:
- Create Consistent User-defined Dict-like Classes: With
MutableMapping
, you can generate atypical dictionary functionalities without losing consistency with dict’s interface.
- Implement Missing Methods: Redefining only a fraction of mapping’s total method and
MutableMapping
will flesh out missing methods. (Python Official docs)
Below is a practical illustration of
MutableMapping
. Here’s a simple SqliteDict that wraps around sqlite3, implemented using
MutableMapping
:
import sqlite3 from collections.abc import MutableMapping class SqliteDict(MutableMapping): def __init__(self, database): self.conn = sqlite3.connect(database) self.cur = self.conn.cursor() self.cur.execute("CREATE TABLE IF NOT EXISTS store (key text, value text)") def __getitem__(self, key): self.cur.execute(f"SELECT value FROM store WHERE key='{key}'") value = self.cur.fetchone() if value is None: raise KeyError(key) return value[0] def __setitem__(self, key, value): self.cur.execute(f"REPLACE INTO store (key, value) VALUES ('{key}', '{value}')") self.conn.commit() def __delitem__(self, key): if key not in self: raise KeyError(key) self.cur.execute(f"DELETE FROM store WHERE key='{key}'") self.conn.commit() def __iter__(self): return iter(self.keys()) def __len__(self): return len(list(self.iterkeys())) def keys(self): self.cur.execute("SELECT key FROM store") return [item[0] for item in self.cur.fetchall()] def close(self): self.conn.close()
Above SqliteDict class displays the power of the
MutableMapping
in creating flexible, dict-like containers while maintaining standard Python dict semantics and interfaces. Note the use of dunder methods which are standard python magic methods used for operator overloading. The operations with these methods include setting a key-value pair (__setitem__), deleting a key-value pair (__delitem__), retrieving the value of a key (__getitem__), obtaining the number of items (__len__) and fetching the dict keys (__iter__).
In essence, developers need to grasp
MutableMapping
– its roles, principles, and potential pitfalls. One must also keep abreast of Python version updates to forestall errors from deprecated features.When working with Python programming, you might encounter an error which states
AttributeError: 'module' object has no attribute 'MutableMapping'
. This error means that your code is trying to access the MutableMapping class from the collections module but cannot find it.
To understand why this happens and how to solve it, let’s first shed some light on what MutableMapping is and what problems associated with it someone might encounter.
The MutableMapping class in Python is a part of the collections.abc module. It provides methods for mutable mappings or dictionaries. The key feature of MutableMapping is that it allows us to add, remove, or change elements in a sequence or collection, thereby bringing a greater level of versatility and utility to our Python programs.
However, one common issue that programmers face is an AttributeError, which states that the collections module has no attribute called MutableMapping. The main reason behind this error is due to inaccurate references in the imports section of your code.
Let’s take a look at a typical erroneous import and how to correct it.
Incorrect Import:
import collections a = collections.MutableMapping()
Correct Import:
from collections.abc import MutableMapping a = MutableMapping()
As you can see, MutableMapping is not directly present under the collections module. Instead, it is residing inside the abc class within collections. Therefore, when you try to call MutableMapping via
collections.MutableMapping()
, Python fails to find it and raises an AttributeError.
Sometimes Python’s traceback helps us to pinpoint the exact code line that causes the error, making it easier to locate issues related to incorrect imports.
Not only should you familiarize yourself with how to correctly import MutableMapping, but solid knowledge of the class hierarchy within Python modules such as collections will be very beneficial in debugging similar types of AttributeError messages in the future.
To navigate complexities around MutableMappings and their correct usage:
• Always check your import statements to make sure you’re importing the right libraries properly.
• Make sure you fully understand the class hierarchy within the Python module you’re using.
• Use traceback to help locate the source of potentially problematic code segments.
• Keep your Python version updated. Libraries have newer versions over time.
Python’s official documentation page would be a great resource to dive deeper into understanding Python’s built-in structures like MutableMapping[^1^].
[^1^]: https://docs.python.org/3/library/collections.abc.html#collections-abstract-base-classesWhen you are dealing with the
collections
module in Python, you might encounter an issue where you try to access the
MutableMapping
attribute and receive an error:
AttributeError: 'module' object has no attribute 'MutableMapping'
. This can be due to several reasons, a few of which we will examine, along with their solutions.
The first probable reason for this issue could be your Python version.
MutableMapping
was moved to the
collections.abc
module in Python 3.3, and from Python 3.8 onwards, importing it directly from the
collections
module is deprecated. The following code demonstrates how you would import it appropriately:
from collections.abc import MutableMapping
If your using an older Python version prior to 3.3, use this instead:
from collections import MutableMapping
As Python evolves, certain features get deprecated or shifted around for better functional and organizational purposes, hence always maintain your Python environment updated to avoid such compatibility issues. Check the official Python documentation[source] to stay informed.
Another possible cause may be a naming conflict, especially if you’re running other codes concurrently, causing attribute-level confusion. To avoid this, refrain from naming your script
collections.py
or having any other modules or scripts with the same name as built-in Python libraries. Also, check your folder structure for
collections.pyc
files, if found, delete them so they won’t be confused with the actual library.
While working with
collections
, in reference to our context
MutableMapping
let’s understand its significance. The primary purpose of
MutableMapping
is to act as a dict-like class, adjustable in nature, allowing storage of variable-length key-value pairs. Here’s an example of using
MutableMapping
to create a custom dictionary:
from collections.abc import MutableMapping class MyDict(MutableMapping): def __init__(self): self.dict = {} def __getitem__(self, key): return self.dict[key] def __setitem__(self, key, value): self.dict[key] = value def __delitem__(self, key): del self.dict[key] def __iter__(self): return iter(self.dict) def __len__(self): return len(self.dict) my_dict = MyDict() my_dict['key'] = 'value' print(my_dict['key']) # prints 'value'
Adopt coding best practices like ensuring your Python environment is updated and not naming your scripts after Python built-ins to prevent attribute errors and ensure smoother coding experience. Happy Coding!
The main question here is the difference between immutable and mutable objects in Python and then we’ll deal with the AttributeError regarding `collections` module.
At the most basic level, objects in Python can be either mutable or immutable. A mutable object can be changed after it is created, while an immutable object can’t.
Consider these examples:
For mutable objects,
# Lists are mutable objects lst = [1,2,3] print(id(lst)) # id before changing list lst.append(4) print(id(lst)) # id after changing list
In the example above, you can see that the identity of the list `lst` remains the same even after we modify its content. Thus, it’s a mutable object.
For immutable objects:
# Integers are immutable objects num = 5 print(id(num)) # id before changing value num = num + 1 print(id(num)) # id after changing value
In this case, `num` points to a different memory location once its value gets updated due to the immutability of integers.
Some of the examples of mutable objects are:
- list
- dict
- set
Examples of immutable objects include:
- int
- float
- bool
- str
- tuple
Now, coming to the second part of your query which is incidentally about mutable objects – `AttributeError: module ‘collections’ has no attribute ‘MutableMapping’`. This error generally occurs due to change in use from Python version 3.2 onwards. The collections module in Python versions 3.3 and above began to support abstract base class submodule (`collections.abc`) for providing classes that can be used to test whether a particular class supports the required interface (source).
So, if your code currently references `collections.MutableMapping`, as might be the case if you’re using older code on a newer Python version, you’d want to update that reference to `collections.abc.MutableMapping`.
You can make this modification as shown below:
Change from:
from collections import MutableMapping
To:
from collections.abc import MutableMapping
After this correction, your `MutableMapping` should work as expected. Remember, keeping software up-to-date is key especially in Python where features, syntaxes and even modules get deprecated or their usage changes across versions.While exploring the vastness of Python’s built-in modules, you will undoubtedly encounter the
collections
module. This module is a container datatypes library that houses alternatives to built-in containers like dictionaries, lists, sets, and tuples.
A typical usage might look something like this:
import collections a = collections.MutableMapping()
But here, a caveat comes into play. If you are using a version of Python older than 3.3, you might have run across the error message
AttributeError: module 'collections' has no attribute 'MutableMapping'
. This particular AttributeError is informing us that Python can’t find the attribute (or in simpler terms, the variable or method) named ‘MutableMapping’ in the module we’ve specified, which in this case is
collections
.
Python’s attribute errors usually imply one of two things, either:
- You are trying to access an attribute that doesn’t exist in your current namespace.
- You’re calling on an attribute from a module where it doesn’t exist.
In the case of the error message
AttributeError: module 'collections' has no attribute 'MutableMapping'
, it’s the latter – you’re asking for the ‘MutableMapping’ from ‘collections’ and Python isn’t locating it.
What’s key to understanding here it has very little to do with you or the syntactical correctness of your code and more to do with the version of Python you’re running. In Python versions pre-3.3, many of the classes we now enjoy and use regularly in the
collections
module were not part of the module. In fact, if you check the official documentation for Python versions 2.7 [Python 2.7 Collection Documentation](https://docs.python.org/2/library/collections.html), you would indeed find no mention of ‘MutableMapping’.
The class ‘MutableMapping’ was introduced as part of the
collections
module overhaul that happened with Python 3.3. This led, along with other updates, to the introduction of new high-performance data types such as the ChainMap, Counter, defaultdict, deque, MutableMapping etc.
So what exactly is
MutableMapping
? To put it simply, it’s a superclass that provides default implementations for various methods that classes matching the Dictionary API should provide. Since these default implementations depend only on “
__getitem__()
“, “
__setitem__()
“, “
__delitem__()
“, and “
keys()
“, ‘MutableMapping’ naturally becomes an ideal baseclass for user-defined mapping types
Here is how you should properly make use of it:
import collections.abc a = collections.abc.MutableMapping()
By importing
collections.abc
, you ensure that you are specifying the formal specifications (also known as Abstract Base Classes) housed there, one of which is
MutableMapping
.
Remember, it’s always important to be conscious of the Python version you’re working in, as different versions support different attributes and functionalities. Sources:
[Python Official Documentation](https://docs.python.org/3/library/collections.abc.html#collections.abc.Mutablemapping),
[Wikipedia Article on Data Type](https://en.wikipedia.org/wiki/Data_type)
The
AttributeError: module collections has no attribute MutableMapping
is actually quite a common error encountered in Python, especially when attempting to work with mutable mapping objects. This error implies that you’re trying to access an attribute or method that doesn’t exist for the particular object or module.
Before diving into this, let’s quickly address what exactly MutableMappings are. The MutableMapping type is an abstract base class from Python’s collections module that provides a mutable dictionary-like interface. Essentially, a mutable mapping is like a standard dictionary but offers more methods that can be overridden and customized.
Reasons behind ‘No Attribute’ Errors
The ‘No Attribute’ errors primarily occur due to two situations:
- The code is calling an attribute or function that doesn’t exist in the object or module.
- The specific attribute or function belongs to another version of Python and is not included in the existing one.
In our case, the second point is particularly relevant. In Python 3.3, changes were made to the collections module which arguably resulted in these errors.
Changes in Collections Module – Python 3.3+
In Python 3.3 and onwards, many classes in `collections` have been reorganized and are now available through the `collections.abc` module. This includes the `MutableMapping`. Hence if your Python version is 3.3+ and you observe an `AttributeError`, it suggests that your import is outdated.
Here’s how the correct import will look in Python 3.3 and later:
From collections.abc import MutableMapping
And here’s the old way that would work in versions prior to Python 3.3, which is likely causing the error message you’re receiving.
From collections import MutableMapping
Hence, by simply tweaking your import statement as per your Python version, you should be able to steer clear of the AttributeError.
In summary, ‘No Attribute’ errors are often an indication that you’re interacting with a non-existent attribute or function within an object or module. In relation to
collections.MutableMapping
, this is typically caused by changes made from Python 3.3 onwards. Always ensure that your import statements are compatible with your current Python version.
For further reference on the topic, check the official Python documentation.
Diving deep into the error message “AttributeError: Module collections has no attribute MutableMapping”, it’s important to understand that the Python `collections` module provides alternatives to built-in container types such as dict, list, set, and tuple. The occurrence of this error often signifies an issue with the accessing or setting of attributes for objects in your module. This is essential to take note as your content must be relevant and interesting to your dedicated developers.
A common reason why you might come across this error message is that you’re using a deprecated version of Python where
MutableMapping
from the Collections module has been moved to the
collections.abc
module from Python 3.3 onwards. Here’s how you can fix this error:
Before:
from collections import MutableMapping
After:
from collections.abc import MutableMapping
It’s far crucial to realize updating your Python interpreter to a more recent version will typically resolve these “no attribute” errors. Alternatively, modifying your code to match your current version could also work. For instance, if you’re using Python 2.x, ensure to stick to conventions compatible with that version.
Moreover, a great method in dealing with attribute errors in Python is utilizing Exception Handling. You can catch the AttributeError exception and print a more user-friendly message or handle the absence of the expected attribute in another suitable way.
Understanding the disorder behind this error furthers the enhancement of problem-solving capacity and maintains the smooth operation of Python codes. For more comprehensive knowledge about Python’s attribute errors and exceptions, visit Python’s official documentation guide.
For your SEO optimization needs, remember to include important keywords related to your topic throughout your content. Keywords like ‘Python’, ‘collections module’, ‘AttributeError’, and ‘MutableMapping’ should consistently appear but remember to keep a natural flow of writing. It’s key to focus not only on SEO but also on delivering clear, high quality information to engage your readers’ interest.
Factor in these points when you craft your SEO optimized content surrounding the AttributeError in the Collections module and harness the power of Python’s robust modules and exception handling features.