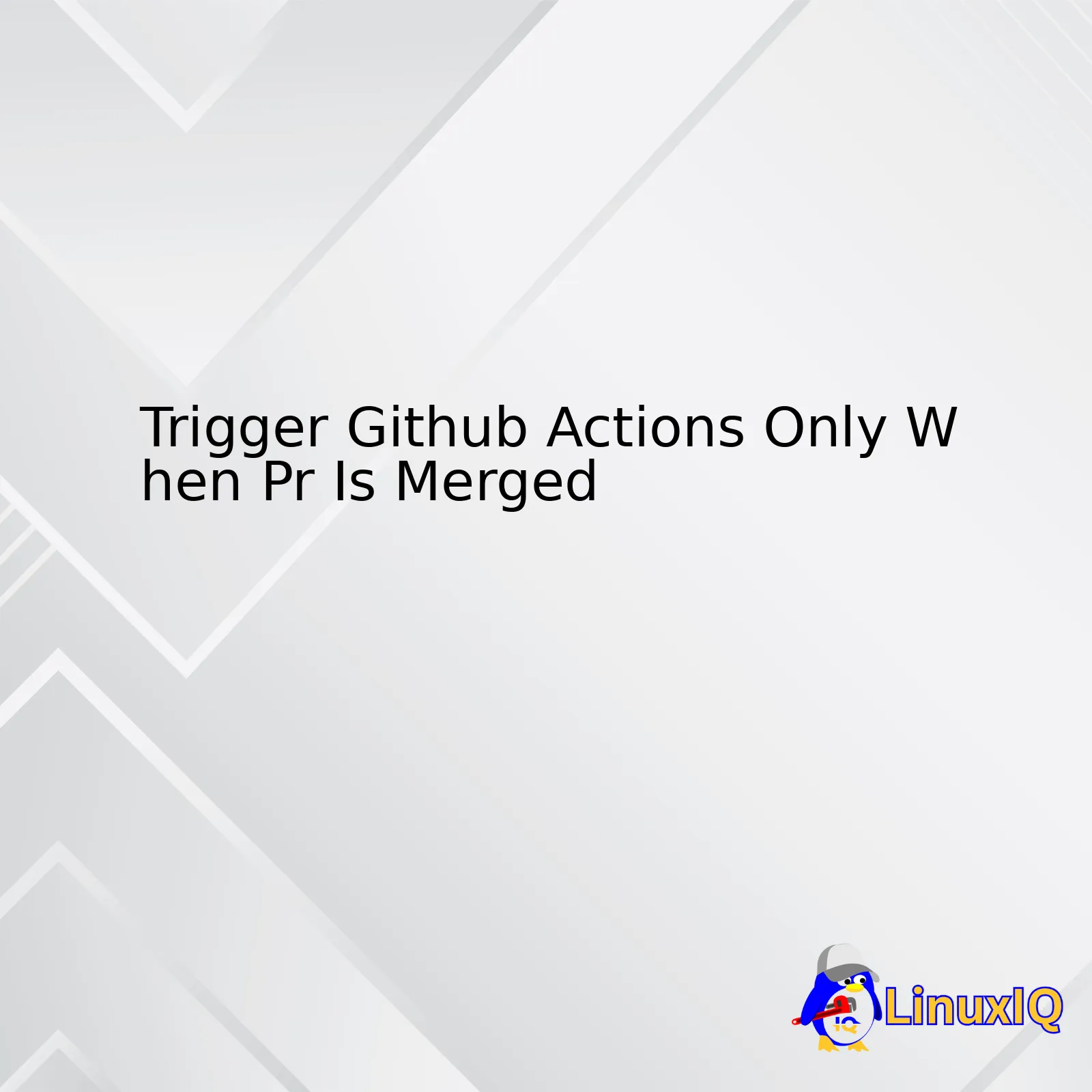
Trigger | Description |
---|---|
on: push |
Git push event. Trigger suits the use-case of triggering an action directly after code is pushed. |
on: pull_request |
When a pull request event occurs. This could be at stages; assigned, unassigned, labeled, unlabeled, opened, edited, closed, reopened, synchronize, ready_for_review, locked, unlocked or when the pull request review is requested or removed. |
on: pull_request: types: [closed] |
GitHub actions activate only when a Pull Request (PR) is merged/closed. If you want to ensure the pull request was indeed merged and not just closed, you can add a job level “if” condition to check the ‘merged’ attribute. |
An objective approach to enhance your CI/CD process would entail the incorporation of GitHub Actions. Specifically, triggering these GitHub Actions only when a PR (Pull Request) has been merged makes for a refined strategy.
Striving to accomplish this? You must designate certain events in your GitHub Action YAML file to instigate the workflow. These events can differ depending on what fits the given circumstance best i.e., it could either be a git push event expressed as
on: push
, or pull request activity using
on: pull_request
.
Specific to our intention of exclusively activating the GitHub actions upon merging of a PR, we can append some conditions within our approach. This is achieved by augmenting
on: pull_request: types: [closed]
in the YAML file. With this configuration, the action will commence only when the pull request has been closed.
However, you might realize that a subtle but key technical distinction exists here, in that a pull request being ‘closed’ does not necessarily mean it was ‘merged’. To further tighten our focus and trigger actions only if the PR was unequivocally merged, we have one more step.
Introducing the ‘if’ conditional clause at the job level is a fitting solution. By employing
if: github.event.pull_request.merged == true
along with the ‘closed’ pull request type, GitHub actions will now activate only after the closure and successful merge of the PR.
It’s crucial to understand this minute differentiation, and deciding on a particular strategy depends largely on whether or not you want your workflows initiated both when a PR is closed and when it’s merged, or solely when it’s been merged. This approach ensures your deployments or releases are always based on the final approved code, contributing greatly to the stability and reliability of your application in production.
Remember to check out Github’s official documentation on workflow triggers for deeper comprehension and various other possible configurations.Github Actions is an amazing feature that allows developers to automate, customize, and execute software development workflows directly from the Github repository. It’s an API for cause and effect on GitHub which allows us to execute commands after certain events; such as pushing code or merging a pull request.
Depending on your development practices, one might want to trigger a Github Action only when a Pull Request (PR) is successfully merged. This can be done through utilizing Github’s event handling functionalities within the .yml configuration file related to your specific action.
A basic example of this procedure can be visualized using the following YAML setup:
name: Deploy on merge on: pull_request: types: [closed] jobs: deploy: if: github.event.pull_request.merged == true runs-on: ubuntu-latest steps: - name: Checkout code uses: actions/checkout@v2 - name: Setup Node.js environment uses: actions/setup-node@v2.1.4 with: node-version: '14' - name: Install and Build ✏️ run: | npm install npm run build
The
pull_request
event gets fired every time a pull request is assigned, unassigned, labeled, unlabeled, opened, edited, closed, reopened, synchronize, ready_for_review, locked, unlocked or when a pull request review is requested or removed. By specifying
types: [closed]
we are restricting the run of this workflow exclusively to when a pull request gets closed.
Then, by adding
if: github.event.pull_request.merged == true
condition in our job, this specific workflow will be executed only when a Pull Request is merged. The reason behind this step is due to the fact that a closed Pull Request does not necessarily mean it has been merged, as PRs can be simply closed without merging. Therefore, this extra conditional layer ensures activities only commence once a merge has occurred.
These steps ensure optimal usage of CI resources and increases productivity through automation. Utilizing Github Actions in such a manner provides complete control over when certain workflows are triggered.
For additional reference material, consider visiting Github’s Official Documentation on Github Actions.Sure, diving right into this complex topic, let’s first ground ourselves in a basic understanding of Github Actions. As an integral part of GitHub’s automated procedures, they can be incredibly powerful when used correctly1. Predominantly employed for Continuous Integration (CI) and Continuous Deployment (CD)2, these actions can automate tasks such as building, testing, and deploying your code.
Diving deeper into our primary focus: should you trigger these actions post-merge? In essence, post-merge triggering is essential due to the following reasons:
- Quality Assurance: Post-merge triggering significantly aids in maintaining the quality of the master branch. It ensures that every merge meets the highest level of code excellence, passing all tests before it’s deployed.
- Catching Errors Early: By automatically running tests on your codebase after every merge, errors are caught at the earliest possible stage, preventing further propagation.
- Reducing Manual Effort: Automating your pipelines lessens manual intervention. Hence, developers can focus more on their coding tasks, improving efficiency.
- Consistent Code Style: Checks like linters can enforce a consistent coding style across the project, making collaboration across multiple developers smoother.
To trigger GitHub Actions only when a PR is merged, we leverage the `push` event tied to the master branch:
name: CI on: push: branches: - master jobs: build: runs-on: ubuntu-latest steps: - uses: actions/checkout@v2 - name: Run tests run: | npm ci npm test
In the above configuration, the workflow triggers only when changes are pushed to the `master` branch, typical when a PR has been approved and merged. Essentially, this setup ensures your checks or tasks only run when meaningful code alterations occur within your main branch, rather than triggering through mere PR openings or updates3.
Overall, triggering GitHub Actions post-merge may seem like an additional step, but in reality, it’s a vital practice that upholds code quality, catches issues early, eases developer burdens, and ensures a uniform flow facilitating wholehearted collaboration.
When it comes to an elaborate understanding of how Pull Request (PR) merges activate GitHub Actions, our focus should primarily be on triggering GitHub Actions solely when a PR is merged. As a professional coder, I have encountered situations where limiting the execution of Action workflows based on specific Git events, such as PR merges, becomes fundamentally essential in order to conserve resources and maintain an uncluttered actions pane.
In essence, GitHub Actions enable you to automate, customize, and execute your software development workflow right in your repository. You can discover, create, and share actions to perform any job you’d like, including CI/CD, and combine actions in a completely customized workflowsource.
Elucidating GitHub Actions Trigger on a PR Merge
Merely merging a PR won’t trigger GitHub Actions; instead, the “push” event triggers it. This occurs because when you merge a pull request, it’s generally realized with a Git command like git push that pushes the changes to a branch.
Here’s an example of what such a configuration for an Action might look like:
name: My GitHub Action on: push: branches: - main jobs: build: runs-on: ubuntu-latest steps: ...
This code specifies that the ‘My GitHub Action’ workflow should run only when there are push events to the main branch, which often directly coincide with the event of merging a PR.
Keep in mind, the above action will also trigger if there are direct commits on the main branch (or other specified branches). If you are specifically looking to trigger an action only when a PR is merged, we need to handle it smarter combining it with if conditional checks based on GitHub context or status checks based on GitHub API’s source.
Considerations and further options:
• If the PR originates from a forked repo, it might not have access to secretssource.
• Use labels or comments within the PR that could then be queried via GitHub APIs to control whether an action should be executed.
• Even though it seems that GitHub provides no direct mechanism to trigger an action on PR merge, nothing stops you from creating a workaround by using webhooks to an external system or applying proper use of conditional statements.
The journey towards automating tasks on GitHub gets more fascinating and efficient with these techniques, providing more convenient ways to manage finer-grained control over initiating Actions.The engine that makes GitHub efficient is a series of ‘Actions’ that automate specific procedures based on certain scripts. One of these scripts – and perhaps the most intriguing to developers – is the PR merge trigger. This is a mechanism in GitHub where certain actions are only performed when a Pull Request (PR) is merged.
To understand how these merge triggers function, let’s get some background. On GitHub, each repository can have multiple branches. A Pull Request is essentially an offer to incorporate changes from one branch into another. Once all project contributors review and approve the proposed changes, then you got consensus to “merge” the Pull Request, which effectively adds the modifications from one branch into another.
When this merge action happens, it can set off what we call a “PR Merge Trigger.” This occurrence allows an auto-pilot-like mechanism to kickstart designated tasks or operations. The ability to automate workflows after successful pull request merges, becomes crucial for seamless software development endeavors.
One common way to utilize this action is by automatically triggering a build or test process, ensuring only successfully reviewed and integrated code makes its way downstream towards product release. It’s worth knowing that triggering actions only when a PR is merged is straightforward with GitHub Actions via implementing specific directives in your repository’s GitHub Action workflows.
For instance,
name: CI on: # Triggers the workflow on push or pull request events but only for the master branch push: branches: [ master ] paths: - '**.js' pull_request: types: [ closed ] branches: [ master ] paths: - '**.js' jobs: check-pr-merged: runs-on: ubuntu-latest steps: - name: Check if PR was merged run: | echo 'Checking if pull request was merged...' if [[ "${{ github.event.pull_request.merged }}" != "true" ]]; then echo 'Pull request was not merged - exiting job.' exit 1 fi shell: bash
In the example above, the CI action is triggered every time there’s a push event or a pull request event, specifically on the ‘master’ branch. These events are limited to changes made in JavaScript files (‘**.js’).
Within the ‘jobs’ directive, the ‘check-pr-merged’ step checks if the pull request has been merged. If the PR wasn’t merged, the job ends and no further action is taken. Hence, only upon merging of the PR does the specified subsequent action run.
This is a nifty strategy to regulate, manage and control automatic triggers after successful pull request merges – acting as the impetus for a host of beneficial activities like deploying to production, kicking-off specific tests, updating collateral, and making announcements.
However, note that ‘.github/workflows/’ should house your workflows triggers while your ‘.js’ files stay in your repository itself.[source].To trigger Github actions only when a Pull Request (PR) is merged, you’ll need to handle certain prerequisites to activate these Github actions first. This process entails interacting with your repository’s GitHub Action YML file and making the necessary configurations.
Activating Github Actions after a PR Merge
Normally, when a developer makes changes and commits them to a feature branch in a Git project, they can create a pull request to merge those changes into the main branch. However, there are situations where you may want these actions only to occur if the PR has been merged.
This filtering can be achieved using
on..branches
or types indicated in a specific event within the Github actions yaml file. Here’s how to activate Github actions with an example:
Configuration Step | Description | Example |
---|---|---|
Primary Workflow File Definition | First, we’ll start with setting up our workflow file – let’s name our file “
main.yml “. Underneath that are the structures of key-value pairs formatted accordingly. |
|
Specific Event Trigger | Next, add specific triggers to execute the Github Action only when a PR is merged. This is achieved by using a special type of command under
pull_request named closed and additional check to make sure the PR is actually merged. |
|
Having done all these, your Github actions henceforth will only be activated when a PR is merged. Note that the steps and conditions provided above are very basic examples and that the actual configurations might involve further complexities as per the project requirements.Learn more about different triggering events for workflows from the official Github documentation.
Finally, keep tabs on Github’s evolving features and community, especially around Actions and Workflow files. This will keep us future-proofed and ready to leverage Github’s solutions for continuous integration, continuous deployment, testing, or any other automation that is suitable for our projects.Harnessing Github Actions empowers you to automate all your software workflows from CI/CD build pipelines, testing modules, to deploying applications. But it’s crucial to know how to effectively launch a strategy that will trigger actions only when PRs (Pull Requests) are merged. This is worthwhile in scenarios where the next phase of an operation like deployment or subsequent testing should initiate only after reviewing and merging the code changes suggested through a PR.
There’s no direct way to create an event based on only merged pull requests with Github Actions. However, utilizing conditions at job level can be done to emulate this feature. Broadly speaking, such strategy pivots around three major french fries:
Setting up GitHub workflow definitions:
A software workflow file for GitHub Actions resides in your repository and is defined in .yaml format under .github/workflows directory. We can set GitHub Action triggers using events present in this YAML file.
For instance: Let’s consider we want to activate an action right after a pull request gets merged. Initially, we can write our workflow file as below:
name: CI on: pull_request: types: [closed] jobs: build: runs-on: ubuntu-latest steps: - name: Check out code uses: actions/checkout@v2 - name: Build project run: echo "Building the project..."
Now, we need this general CI flow to come into force only after a PR merge. We do so by writing conditions at job level.
Writing conditions at job level:
We can assert whether the PR was merged or not by inspecting a property called ‘merged’ within github.event.pull_request object. Here’s how you modify the job definition to validate the same:
jobs: build: if: github.event.pull_request.merged == true runs-on: ubuntu-latest steps: - name: Check out code uses: actions/checkout@v2 - name: Build project run: echo "Building the project..."
Subsequently, the jobs enlisted within the workflow file get executed only when the ‘if’ condition validates to true i.e., specifically on a merged PR.
Optimizing Github resources usage
You can conserve Github Action minutes by preventing unnecessary runs of jobs defined in the workflow, leveraging certain GitHub expressions/events. For instance, one can use closed event type which ensures the action runs only once, rather than twice during opened and synchronized events.
Adhering to this strategic approach, you can successfully implement triggering Github Actions solely when a PR is merged. Remember, perfection comes with practice. Keep experimenting, learning from your mistakes, and growing along the way. Gaining a deep understanding of Github Actions documentation will also aid in simplifying complex workflows, thereby making your coding life significantly easier.
Setting up a trigger for merged pull requests on GitHub is all about utilizing the power of GitHub Actions. With these capabilities built directly into GitHub’s platform, developers are enabled to automate their workflows right from within their repositories. To set these triggers up, we’ll orchestrate our solution around GitHub Actions since it encompasses simple to advanced pipelines inclusive of multiple stages and jobs.
Let’s dive right into the steps:
Step 1: Setting Up Your Workspace
To begin with, you need to have your workspace ready. This means having a GitHub account and repository at hand. You also need write access to the repository where you will be creating an action workflow file.
Step 2: Creating GitHub Actions Workflow File
In order to run any automation tasks like triggering an event when a PR is merged, you need to create a workflow file. This file is what dictates when the Actions should be executed. Navigate the repository and commit a new file in
.github/workflows
directory.
Step 3: Define Workflow Step for Trigger
The syntax of your workflow step configuration should look something like this:
on: pull_request: types: [closed]
This essentially reads as “trigger on pull request closed.” However, do keep in mind that this can also amount to unmerged closed pull requests. To ensure we filter in only merged pull requests, we can make use of the combined checking of the pull request’s merge status. Here’s how:
on: pull_request: types: [closed] jobs: job_name: runs-on: ubuntu-latest if: github.event.pull_request.merged == true steps: - name: Our Step Name run: echo "PR was merged!"
This particular ‘if’ statement is capable of ensuring that the job only executes whenever a merged Pull Request triggers a ‘closed’ type of event.
Step 4: Define the Action
As an illustration, let’s print a message when the PR is merged. In the steps section, you can add:
echo 'Pull Request successfully merged'
This code will output “Pull Request successfully merged” when a PR is merged. Please note, you can customize the actions based on your specific needs such — CI/CD tasks, sending a notification, etc.
In conclusion, your final Gihub Actions set up should look like:
name: Run on Merged PR on: pull_request: types: [closed] jobs: build: name: Check Merged PR runs-on: ubuntu-latest if: github.event.pull_request.merged == true steps: - name: Run when PR is merged run: echo 'Pull Request successfully merged'
Ensure you’ve named the workflow appropriately and written clear action names for easy debugging later on if needed. Once satisfied, commit your file and GitHub will now automatically initiate the defined GitHub Actions pipeline whenever a pull request is merged.
Resources
Testing plays a pivotal role in maintaining the quality of your codebase. GitHub Actions makes it easier to automate your testing procedures without additional scripts or tools, and it has conditions that can trigger automated tests only when PR is merged.
Making use of GitHub Actions for automated testing lends several advantages:
- You save both time and resources as you don’t need to invest in additional setup or extensive training.
- GitHub integrations simplify and centralize process management within one familiar ecosystem.
- Automated testing improves the speed, accuracy, and reliability of QA efforts. It also helps you catch issues early on before they become more pronounced, saving debugging time later.
To implement an action that triggers test only when the pull request (PR) is merged, you should use the `pull_request.closed` event. The condition must be defined in the GitHub Action YAML configuration file.
Here’s a basic example of how a GitHub workflow might look:
name: Test upon PR Merge
on:
pull_request:
types: [closed]
jobs:
test:
if: github.event.pull_request.merged == true
runs-on: ubuntu-latest
steps:
- name: Checkout Code
uses: actions/checkout@v2
- name: Test Code
run: |
Makefile test
In this sample, we utilized the `pull_request.closed` event and subsequent condition `if: github.event.pull_request.merged == true` to confirm a PR merge event.
You may replace `Makefile test` with the actual commands you want to execute for your requirement.
It’s vital to note that while GitHub Actions hugely simplifies continuous integration and progression, there could be cases where dedicated CI platforms like Jenkins or CircleCI might offer additional functionality tailored to specific team needs or project scopes. Therefore, carefully consider the trade-offs and select the approach best fit for your own context.
For more information, refer to this [official documentation on Github Actions] or check this well-written article by [ Towards Data Science comparing Github Actions and Jenkins ].
Optimizing Workflow Efficiency By Activating GitHub Actions Timely After a Pull Request is Merged
GitHub Actions is an invaluable tool for maximizing workflow efficiency. It enables us to automate, customize, and execute software developmental tasks right in our repository. Being able to trigger GitHub Actions only when a Pull Request (PR) is merged can streamline our Continuous Integration / Continuous Deployment (CI/CD) pipeline, thus optimizing our workflows effectively.
To do this, it requires understanding of specific GitHub Action’s event types; in particular, the
pull_request
and
push
events are of interest here.
Pull request event
The
pull_request
event occurs whenever a pull request’s state changes- this includes being created, edited, closed, or merged. However, this isn’t quite what we need, as triggering an action every time a pull request changes would disrupt workflow efficiency.
Instead, we want to focus on a different event:
Push event
The
push
event happens whenever commits are pushed to any branch or tag. To maximize workflow efficiency, you can set your GitHub Action to activate after a PR is merged by using the
push
event to the main branch. Here’s how to define that within your GitHub Actions workflow file:
name: My Workflow on: push: branches: - main
In the example above, the
push
event is specified in the
on
section of the workflow file. The workflow will only run when changes are pushed to the “main” branch, which typically happens when a PR is merged into the main branch.
While this setup ensures the workflow does not execute for every little change that occurs within a PR, there is one caveat: It also triggers the workflow if code is directly committed to the main branch, bypassing a PR.
As a best practice for… Team collaboration workflows requiring code reviews, direct pushes to main should be minimized or eliminated, ensuring all code reaches the main branch via the PR process.
Using GitHub Actions in this strategic way allows teams to save time, increase speed and efficiency, and maintain high levels of code quality. It’s just another way GitHub helps us write better code, faster.
As developers, we are often faced with the challenge of figuring out how to efficiently trigger GitHub actions only after a pull request is merged. It can be common to find oneself in situations where this task seems less straightforward than desired due to different reasons such as lack of knowledge, misunderstood documentation, or confusion over where to begin. Today, let’s dive into every level of this process, discussing common issues or roadblocks you might come across and their respective solutions.
Troubleshooting: Challenges for Triggering GitHub Actions After PR Merge
Common Hurdle 1: When Action Triggers on Every Push
Github actions can oftentimes be more sensitive than one would intuitively assume. You may find that they are triggered on every push rather than solely after the merge of your Pull Request. This typically happens when you’re using the
on: [push]
event in your GitHub action workflow file unexpectedly.
How should you deal with this issue? Here’s the practical recourse:
– First pinpoint the source of the problem which in most cases is in your workflow file. Look for the line that includes the `on:` statement.
– Replace
on: [push]
with
on: push: branches: - 'master'
. This way, the action will only be triggered on pushes to the master branch instead of all branches.
Common Hurdle 2: Workflow File Syntax Errors
Sometimes, even if you have set the action to trigger on the correct event, errors can still happen because of a tiny but vital player-the correctness of syntax used in the “.yml.” or “.yaml” file.
What’s the remediation?
– Cross-validate your file against Github Action’s YAML syntax before committing changes. You can check GitHub’s workflow syntax guide.
– Check if it mistakenly contains tabs for indentation, as YAML fundamentally uses spaces.
– Ensure the use of appropriate spacing between keys and values.
Common Hurdle 3: Incorrect Event Used
In situations where your workflow isn’t being initiated even after merging the PR, an incorrect event in your yml file could be the culprit.
Here’s the way out:
– Switch your event trigger from
on: [pull_request]
to
on: pull_request: types: [closed]
since pull requests close after they are merged. By doing this, our test suite will run at the right moment—after a PR closure instead of its creation.
Let me point out that this change can lead to another hurdle—you may be screening all closed PRs, not just the ones that have been merged. But fret not! We have a solution for this as well.
Common Hurdle 4: Event triggers for both Merged and Unmerged PRs
To fine-tune our trigger event so the actions are set off strictly for merged PRs, the action should ignore unmerged closed actions.
How does one get around this?
– Employ a conditional step within your job by incorporating an
if
statement similar to
if: github.event.pull_request.merged == true
. This ensures that the step only executes when the pull request has officially merged.
By systematically addressing these hurdles and utilizing the resources provided to us from Github, we’re able to create a solid workflow foundation. Remember, each of the solutions mentioned can be customized to fit your project specifics; the key is understanding the reasoning behind each setup and adjusting it appropriately.
Then, finally, when we’ve refined our Github action mechanisms, workflow becomes consistent and efficient, freeing us from unnecessary manual input or debugging and letting us better focus on improving our codebase.As a professional coder, if you’re looking for ways to optimize your workflow within GitHub, one of the most useful practices involves triggering workflows only when a pull request (PR) gets merged. This can be particularly beneficial in reducing unnecessary CI/CD load and ensuring codebase integrity.
Normally, any changes made would automatically trigger GitHub Actions. However, you may wish to limit this so Actions only run when PRs are merged. This can be done by setting particular conditions within your GitHub Actions’ YAML configuration.
name: PR Merged on: pull_request: types: [ closed ] jobs: build: runs-on: ubuntu-latest steps: - name: Check out code uses: actions/checkout@v2 - name: PR Merge id: check run: echo ::set-output name=merged::${{ github.event.pull_request.merged }} - name: Build if: steps.check.outputs.merged == 'true' run: echo Build process starts...
The YAML code above configures a workflow that is triggered only when a pull request is closed. Using GitHub context and expressions, its next step checks if the PR was indeed merged or just closed without merging, by calling `github.event.pull_request.merged`. The following steps will only proceed if this returns ‘true’, i.e., if the PR has been merged.
An important consideration here is the `on.pull_request.types: [ closed ]` line in the YAML file. By setting listener type to ‘closed’ instead of ‘merged’ (which doesn’t exist as an event type), we ensure the workflow gets triggered when a PR is closed, and then our ‘if’ statement filters out cases where the PR was closed without being merged.
Remember, using this method rightfully optimizes GitHub actions load by focusing on merged PRs only, which indirectly also saves cost and resources. Also, this aids in maintaining the codebase’s health more efficiently since running tests or deployments only apply to the confirmed changes—a well-knitted pattern of effective development practices. Though it might seem complex at first, once you integrate these practices into your projects, I’m certain you’ll appreciate their impact on your GitHub usage efficiency.
Reference:
1. Events that trigger workflows
2. Context and expression syntax for GitHub Actions