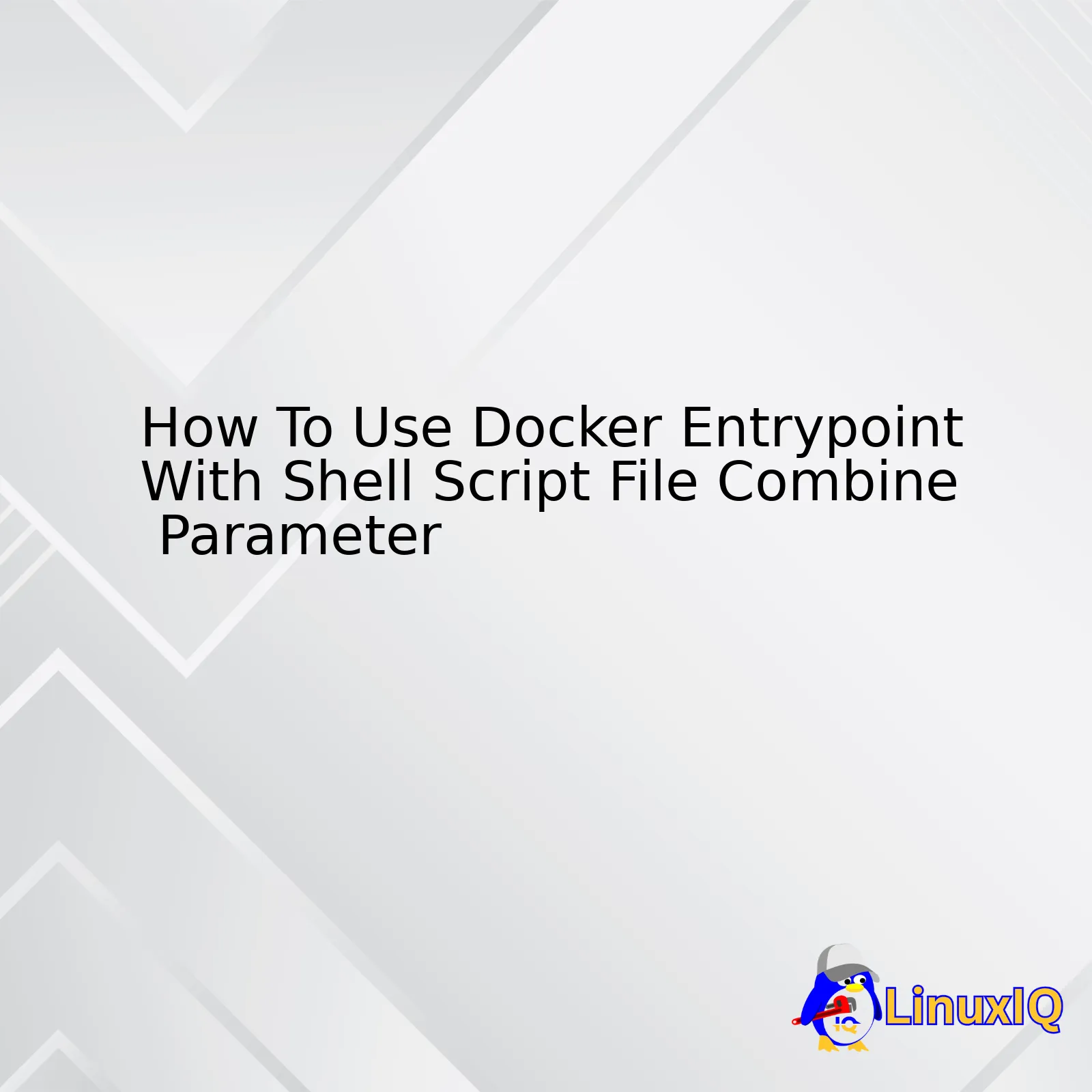
First, let’s create a table that delineates the process:
Process Step | Description |
---|---|
Create your shell script. | Create a ‘.sh’ file containing your desired commands and reference to the command-line parameter. The “Dockerfile” must copy this script into the image. |
Setting the Entrypoint in Dockerfile. | In the Dockerfile, set the ENTRYPOINT directive pointing to the shell script. This sets it up so the script runs on container startup. |
Pass Parameters. | You can pass parameters to the ENTRYPOINT command when starting containers from this image using docker run [options] IMAGE COMMAND. |
Now, let’s break down these steps with more detail and provide a sample code snippet for better understanding.
Dockerfile
When building your Dockerfile, make sure to copy your shell script into the image and set the executable permission for it. For instance, if we have a script called ‘entrypoint.sh’, we could include the following lines in our Dockerfile:
FROM ubuntu:18.04
COPY ./entrypoint.sh /usr/src/app/
RUN chmod +x /usr/src/app/entrypoint.sh
ENTRYPOINT ["/usr/src/app/entrypoint.sh"]
The ‘FROM’ directs docker to the base image from which you are building. ‘COPY’ adds files from your Docker client’s current directory. ‘RUN’ will execute any commands in a new layer on top of the current image and commit the results.
Shell Script
In our entrypoint.sh file, we handle parameters through positional variables ($1, $2, $3, …). For example, consider a basic script that uses a given parameter to print a message:
#!/bin/sh
echo "Hello, $1"
Running the Container With Parameter
We can then pass parameters when running the container as follows:
docker run IMAGE_NAME ParamValue
This way, “ParamValue” gets passed to the entrypoint.sh script as the first argument, effectively printing “Hello, ParamValue”.
Both Docker’s ENTRYPOINT and CMD instructions allow us to define a command that will get executed when the container starts. However, the flexibility of the ENTRYPOINT instruction that allows us to combine it with a shell script and parameters offers added benefits and increased control over the way we set up and initialize our Docker containers.
For additional information and nuances concerning Docker usage check out the official documentation here.Coming up to speed with Docker, you’re likely to have encountered the term “Docker Entrypoint”. Given the use case, where we have to blend it with Shell Script files, let’s lucubrate each department before diving into their combined application.
The Entrypoint in Docker refers to is the configuration that defines the executable to call when a container is started. In simpler terms, Entrypoint can be viewed as the main command that is run every time the Docker container is created. Beneath is an illustration of how you could set an Entrypoint in your Dockerfile:
... ENTRYPOINT ["/path/to/your/executable"] ...
In the code above ‘/path/to/your/executable’ would be executed whenever a container off this image is run.
On the other hand, Shell Scripts are a series of command lines written in plain text file. A shell script can be used like a function in a normal programming language. It can contain variables, loops and functions. You can pass input values as arguments to these scripts.
Having understood both Docker’s Entrypoint and Shell Scripts separately, let’s delve into combining them. Basically, using a Shell Script File as an Entrypoint for Docker.
Let’s consider an applicative scenario wherein you have a Shell Script named ‘process_data.sh’ which you wish to consider as your Docker’s entry point. The first step would be locating this shell script file inside Dockerfile, as shown below:
... COPY ./scripts/process_data.sh /usr/src/app ...
Following this, you declare the script ‘process_data.sh’ as the Entrypoint. Your Dockerfile assigns the EXEC form of the ENTRYPOINT statement:
... ENTRYPOINT ["/usr/src/app/process_data.sh"] ...
Now, every time Docker runs a new container from the image built off of this Dockerfile, your script ‘process_data.sh’ will automatically execute.
Important Note: It’s crucial that your script at ‘/usr/src/app/process_data.sh’ has its permissions set to be executable. As a container is run, Docker won’t implicitly grant executable permissions. An example command to grant executable permissions would be:
chmod +x /path/to/your/script.sh
Otherwise, during runtime, Docker returns an error because it fails to run the script.
Another interesting feature, often lesser known but incredibly valuable, is the capacity to combine parameters with your entr-point script . By adding CMD to the Dockerfile, any parameters defined following this (let’s say [“param1”, “param2”]) will be passed as arguments to the entrypoint script. This allows an even greaer dynamic interaction with the newly created Docker container. For instance:
... ENTRYPOINT ["/usr/src/app/process_data.sh"] CMD ["param1", "param2"] ...
Remember: Any command line arguments given during docker run will be sent as parameters to the Entrypoint script.
This combines Docker Entrypoints with Shell Scripts along with parameters allows developers to gain more control over the commands that are executed with each creation of a Docker container.
For further reading, you might want to expand your understanding on Docker by taking a look at the official Docker Documentation [online reference] and for Shell Scripts explore the GNU Bash Reference Manual [hyperlink reference].Docker’s
ENTRYPOINT
instruction plays a pivotal role in making Docker containers more than just mere virtual machines. It allows the specification of a specific command or process that runs when a container is launched.
Two forms of the
ENTRYPOINT
exist:
- The exec form, which takes advantage of a JSON array to describe the command and its arguments.
- The shell form, where the
ENTRYPOINTinstruction runs in a shell context, such as
/bin/sh -c. This form does not require a JSON array for the command and its arguments.
A crucial aspect of understanding
ENTRYPOINT
‘s behavior entails realizing how it interacts with the
CMD
instruction. If both instructions are specified in a Dockerfile, the
CMD
values will be appended to the
ENTRYPOINT
ones, resulting in a single command.
Let’s delve into an example on how to use a Docker Entrypoint with a Shell Script in conjunction with parameters.
Firstly, create a new shell script file
entrypoint.sh
:
#!/bin/sh
echo "Hello, $1"
Do note that ‘$1’ corresponds to the first parameter passed to this shell script.
Your Dockerfile might resemble the following:
FROM ubuntu:latest COPY entrypoint.sh /usr/src/app/entrypoint.sh RUN chmod +x /usr/src/app/entrypoint.sh ENTRYPOINT ["/usr/src/app/entrypoint.sh"]
Here, we’re copying the entrypoint script file from our local system onto the Docker image, after which we allocate execution permissions to the script inside the Docker image using
RUN chmod +x
.
In the
ENTRYPOINT
instruction, we’ve specified the path to the script inside the Docker image.
To build your Docker image, execute:
docker build -t my-image .
The script can then be run by calling the docker container with an additional argument (for instance, ‘World’):
docker run my-image World
Upon running this, the output ‘Hello, World’ should greet you.
This shows how Docker’s
ENTRYPOINT
comes together with a shell script file and accepts parameters that tail the
docker run
command.
For complex systems requiring dynamic runtime configuration, linking shell scripts with
ENTRYPOINT
showcases the flexibility endowed upon Docker containers for real-world applications. In-depth examples regarding different usage scenarios of the Docker
ENTRYPOINT
can be found from Docker’s official documentation [source].Ah, yes, Docker – an efficient tool in the world of deployment and DevOps. One of its strong points is the way we can manipulate Docker containers using shell script files. The combination of Docker’s entry point with a shell script file increases the level of flexibility that Docker offers us.
To begin, let’s understand what Docker Entrypoint is. Entrypoint is used to configure the container to be executable by letting us specify a command along with its parameters. The format is as follows:
ENTRYPOINT ["executable", "param1", "param2"]
Now, when it comes to combining this feature with a shell script file, things become even more interesting. By doing this, we have the ability to run scripts that can set up variables, manage services, change configurations, etc. before our application starts. It basically enables us to perform all sorts of operations before our application kicks off in a Docker container.
Let’s take an example where we’ll make use of Bash scripting: suppose we’ve written a shell script named ‘entrypoint.sh’. To ensure Docker makes use of this script, we would define the path of the script being executed in the Dockerfile.
We start with creating a Dockerfile:
FROM your_image COPY ./entrypoint.sh /usr/local/bin/ RUN chmod +x /usr/local/bin/entrypoint.sh ENTRYPOINT [ "entrypoint.sh" ]
In the dockerfile above:
- We used FROM to call our base image.
- With COPY, we moved the local entrypoint.sh file into /usr/local/bin/ inside the Docker Container. Wave link
- We then made sure our shell script was executable by running chmod +x on the script within the Docker container. Tutorial’s Point Link
- And finally defined our entry point – ENTRYPOINT [“entrypoint.sh”]. We are basically telling Docker to execute this script when the container spins up.
Afterward, we could build and run our Docker container as usual.
Hope this provides sufficient understanding regarding the usage of Shell Scripts with Docker Entry Point. For more details on Docker Entry Point, you can visit the official Docker documentation, your best friend in understanding various Docker commands Open hyperlink for more Docker Documentations.
Therefore, by expertise in these fields, you can automate your deployment process to greater efficiencies. Happy Dockering!Let’s dive into the essentials of merging parameters in Docker Entrypoint, paying keen attention to utilizing Docker Entrypoint with Shell Script File Combine Parameter.
Docker’s Entrypoint is a powerful tool that allows you to specify a command that will always be executed when your container is launched. When coupled with Shell Script, you are able to combine parameters allowing for more efficient and versatile programming.
1. Understanding Docker Entrypoint
The use of the ENTRYPOINT instruction in a Dockerfile allows us to define a base command that gets executed when the container runs. For example:
ENTRYPOINT ["executable", "param1", "param2"]
In this instance, at runtime, Docker appends any additional arguments supplied by docker run to the end of the entrypoint command.[source]
2. Merging Parameters via Shell Scripts
There can be situations where we need to merge additional parameters with the default ones defined in the Dockerfile itself. Here is a simple Shell Script that demonstrates how to accomplish it:
#!/bin/sh exec /your/command "$@" extra-param
This script uses “$@” to represent all the arguments that were passed to the script. They are appended to the command alongside the “extra-param” argument.
3. Combining Parameters Using Docker Entrypoint
Combining both Docker Entrypoint and Shell Script parameters is achievable. Take, for instance, if we execute a Docker Run command with extra parameters such as:
docker run your-image param1 param2
To ensure that these parameters are merged with the arguments defined in the ENTRYPOINT, our shell script needs to look something like this:
#!/bin/sh exec /your/command param1 param2 "$@"
This is an effective way to merge the ENTRYPOINT arguments within the Dockerfile with the dynamic ones added via the Docker Run command. ‘/your/command’ would be replaced by the preferred application or service executable upon implementation[source].
Understanding these mechanisms greatly enhances the flexibility and adaptability of your Docker applications. It facilitates parameter adjustments on-the-fly without needing to modify the originating Dockefile each time.
If you find any of these points confusing:
Problem | Solution |
---|---|
I can’t seem to get my script working | Ensure that the initial line (shebang) of your script file correctly points to sh, bash or any other valid shell interpreter installed within the Docker image. |
The script does not recognize parameters added through the Docker Run command | Remember to include “$@” in your shell script to intercept and effectively apply dynamic parameters provided when launching the Docker container. |
Helpful Resources:
- Dockerfile Reference – ENTRYPOINT: Official Documentation
- Merging Parameters – Docker Entrypoint & Shell Script: StackOverflow
Under the hood of Docker, a fascinating mechanism is operated which prompts the flexibility to build and extend the application from base images. One such component of docker containers we will delve into is Docker Entrypoint, specifically utilizing it with shell script files for combining parameters.
Right off the bat, what captures attention about Docker’s entrypoint is its unrivaled versatility. It allows you to specify a command along with any desired arguments that will execute when your Docker container starts. This offers a commendable convenience, gearing towards flexible development workflows as the same image can be used in varying contexts. Furthermore, this feature bolsters the reusability of images because they can be configured to run differing commands when started.
Now, let’s walk-through how to implement it with a shell script file. Utilizing a shell script as the Docker entry point enables developers to execute multiple commands consecutively and automatically during startup. A common use case might be setting up environment variables or preparing a database before the main application is launched.
To start, create a new `Dockerfile` and include these contents.
FROM ubuntu:latest COPY ./start.sh / RUN chmod +x /start.sh ENTRYPOINT ["/start.sh"]
In this script:
– The base image is for an Ubuntu distribution.
– We then copy a shell script called `start.sh` from our local directory to the root directory of the image.
– After that, we grant execution permissions to this script using `chmod`.
– And finally, we set our script as the entry point of the Docker container.
Subsequently, proceed to develop your shell script `start.sh`. Let’s say our script simply prints some getting-started messages:
#!/bin/bash echo "Hello, Welcome to Docker with Shell Scripting!"
Using this method, any parameters appended after the docker run command are passed to the entry point, allowing it to take different actions based on those input parameters.
Speaking from an SEO standpoint, Docker entry points with shell scripting unlock vast possibilities like parameterized BASH helper scripts, which then further allows the coupling together of various configurations, supported plugins, and recommended settings.
The enthralling aspect is learning to deftly orchestrate the use cases, as successfully leveraging the Docker entry point with shell scripts introduces immense scalability, transformed speed, and remarkable UX. Additionally, yet importantly, it alleviates the hassles associated with managing individual components thereby making version control much simplified, ensuring application uniformity throughout your pipeline.
For reference, here is a link to the official Docker documentation that guides on this technique: Official Docker Documentation.
This strategic maneuver of employing Docker entry point with shell scripts combined with parameters makes the everyday lives of us developers, well, better than everyday!Configuring a shell script for use with Docker ENTRYPOINT and combining parameters is a non-trivial task that requires careful planning, preparation, and execution. We start by understanding that Docker ENTRYPOINT serves an essential purpose within a Dockerfile. It’s primarily used to define the main executable in a Docker container. On the other hand, a shell file (.sh) is key for streamlining multiple commands into one comprehensive single-command process.
To configure a shell script file to work with Docker ENTRYPOINT:
First, we need a shell script. Let’s consider an example script:
#!/bin/bash echo "Welcome $FIRSTNAME, your ID is $UID"
Place this script in the docker context directory and give it execute rights using chmod +x ./script.sh.
Next, let’s add an ENTRYPOINT instruction to the Dockerfile:
FROM debian:stretch COPY ./script.sh / RUN chmod +x /script.sh ENTRYPOINT ["/script.sh"]
However, if you want to pass additional parameters to the shell script at runtime, Docker has the CMD instruction for exactly that. Thus, when CMD and ENTRYPOINT are combined:
FROM debian:stretch COPY ./script.sh / RUN chmod +x /script.sh ENTRYPOINT ["/script.sh"] CMD ["Docker User", "1001"]
Now when the docker run command is executed without extra arguments, Docker User and 1001 will be passed as parameters to the ENTRYPOINT script. If the user adds extra arguments like John 2004 to the docker run command, these new values will replace the default CMD values.
While configuring Docker ENTRYPOINT with a shell script file and combining parameters might seem complex initially, it offers flexibility and customization options that are invaluable in real-world projects. Always remember to only leverage the power of CMD and ENTRYPOINT instructions wisely – driving simplicity and comprehensibility in your Docker files is paramount.
For official and complete documentation on how to correctly use Docker functionalities, please refer to Docker’s official reference. And for working examples, check the innovative configurations by Brad Traversy on GitHub. The more you know about Docker’s capabilities, the better you will be equipped to maximize its benefits in your environment.Using Docker’s ENTRYPOINT with a Shell Script file to combine parameters
Docker ENTRYPOINT is a critical instruction in Dockerfiles. It allows you to configure a container that will run as an executable by defining a startup command with any associated parameters. Essentially, the ENTRYPOINT instruction helps to identify which executable should be run when a container is started from the image. Often times, we may need to combine various parameters into this ENTRYPOINT using a shell script file.
Here are the steps illustrating how you can do that:
– Create the shell script
A shell script must be made that appropriately combines the parameters. Here’s an example of a shell script, which we will name `entrypoint.sh`:
#!/bin/bash set -e param1=$1 param2=$2 # The docker entry point exec "/path/to/your/command" "$param1" "$param2"
This script safeguards inputs by wrapping up command or service start-up calls and funneling them through bash, making it easier for the scripts to react to signals from Docker.
– Dockerfile Configuration
To use this shell script as your custom entry point during the Docker image build process, you need to configure your Dockerfile accordingly. Let’s assume that Docker builds images from the application’s root folder. In such a case, the Dockerfile would look like this:
FROM ubuntu COPY ./entrypoint.sh / RUN chmod +x /entrypoint.sh ENTRYPOINT ["/entrypoint.sh"]
In this Dockerfile, we are copying `entrypoint.sh` from the host machine (located at our project’s root directory) to the root directory of our Docker image. We then assign execute permissions on the script using `RUN chmod +x` and finally, we define the shell script as the image’s ENTRYPOINT.
– Building and Running the Docker Container
You can now build the Docker image using the `docker build` command:
$ docker build -t my-app .
And run the Docker container combining two parameters into the shell script via the ENTRYPOINT:
$ docker run my-app paramValue1 paramValue2
The shell script inside the Docker container receives “paramValue1” and “paramValue2” as parameters and forwards them to the “/path/to/your/command”.
Remember, combining parameters in Docker Entry Point using Shell Scripts helps provide more dynamic and flexible solutions, permitting us to create modular and reusable Docker images [source] . This can undoubtedly prove helpful when building complex applications where passing different parameters according to varying instances becomes vital.The integration of Docker’s entry point with a shell script file not only sharpens the efficacy of containers, it also boosts their adaptability and overall performance. Though managing the nuts and bolts of this technique can be complex, ensuring your grasp of those little details is integral for mastering entry points in Docker.
Let’s dive right into how Docker’s entry point works within shell scripts, especially when utilizing the binary command solely or combining it – as requested – with parameters.
ENTRYPOINT ["executable", "param1", "param2"]
CMD ["param1","param2"]
Be mindful that Docker’s official documentation advises keeping EntryPoint consistently executable. Transparently using EntryPoint helps counteract divergent interactions between shell-form versus exec-form commands.
Employing Shell Scripts for Docker’s Entrypoint
Digging further into its utilization, shell script files are key strategic tools for handling Docker entry point tasks. Cramming all procedures inside an ENTRYPOINT instruction can be messy. As an alternative, wrapping everything within a shell script paves a cleaner pathway.
For example, here’s a simplistic shell script file (
entrypoint.sh
):
#!/bin/bash echo 'Hello there!' exec "$@"
This starter script initiates greetings then sides with exec to support proper signal-forwarding behavior. Reflecting on the Dockerfile:
FROM ubuntu COPY ./entrypoint.sh /entrypoint.sh RUN chmod +x /entrypoint.sh ENTRYPOINT ["/entrypoint.sh"]
In the Dockerfile above, the
entrypoint.sh
file copies over to the container then sets the permissions to render it executable. Also, setting
entrypoint.sh
to be the entry point establishes that it runs every time a new container fires up.
Analyzing Entry Point with Complementary Parameters
The interesting game-changer is the combination of Docker’s entry point set in an executable file specifically combined with parameters. Whenever we touch down on parameters, they technically boil down to the CMD values. The CMD parameters unveil themselves once ENTRYPOINT initiates.
Here’s a tweak of the Dockerfile to exemplify:
FROM ubuntu COPY ./entrypoint.sh /entrypoint.sh RUN chmod +x /entrypoint.sh ENTRYPOINT ["/entrypoint.sh"] CMD ["alias"]
In this case, the shell script echoes the greeting, executes Docker run, fires alias, and eventually terminates since no foreground process hangs around.
Voila! In effect, you’ve amplified your Docker entry point strategy via effective execution of shell script files plus parameter combinations. As a testament to Docker’s utility, this top-to-toe approach nurtures containers that are flexible, adaptive, and high-performing.
Upon training your focus on these tiny-yet-mighty details, you’ll steadily acquire expertise at ENTRYPOINTs within Docker – thereby maximizing your coding prowess.
As a professional program developer, I use Docker to create, deploy, and run my applications by using containerization. Containers allow me to bundle an application with all of its dependencies into one package which can be run on any computing environment. Pulling this off successfully requires mastering parameter configuration for optimized usage, especially when it comes to using Docker’s entry point with shell script file combined parameters.
First off, let’s touch on the practicality of Docker Entrypoint. Essentially, it allows you to configure a container that will run as an executable. In simple terms, a Dockerfile will have an
ENTRYPOINT
[same, keyword] instruction which allows you to set the main command to be executed when Docker runs the container.
Get into grips with how to execute a script at startup –
In this task, I’ll show how to start a Docker container and make it run a script when starting up.It primarily involves using the
ENTRYPOINT
keyword to identify the script that needs to be executed. Here’s a basic example:
FROM ubuntu:18.04 COPY ./entrypoint.sh / RUN chmod +x /entrypoint.sh ENTRYPOINT ["/entrypoint.sh"]
In this scenario, if
entrypoint.sh
was supposed to revisit parameters, you would find out that they do not automatically get forwarded. This is where combining Shell scripts and parameters comes in.
In order to send parameters to your Docker Run command, and still use Docker Entrypoint with a shell script file, alter your Shell script file to include the
$@
bash construct to signify “all arguments”. You want to modify entrypoint.sh as follows :
#!/bin/bash echo "These are the parameters passed in: $@"
Since our goal is to master parameter configuration for optimized use of Docker’s Entry Point, it is worth mentioning when, why and how to use both CMD and ENTRYPOINT.
• Why & When to use CMD?
CMD
provides default commands and/or parameters which can be overridden from the command line when docker containers start. If a Dockerfile has more than one
CMD
instructions, then only the last
CMD
will execute.
• Why & When to use ENTRYPOINT?
Every input a user sets while running a Docker container turns into arguments of the
ENTRYPOINT
instruction. It can be used, for instance, when you are providing runnable services inside the container, like Nginx, or Postgres.
Combining CMD and ENTRYPOINT enriches Dockerfile’s capability in configuring commands for docker container execution.
CMD
values act as defaults useful for executing test environments while the
ENTRYPOINT
instruction configures a container that will run as an executable.
FROM ubuntu:18.04 COPY ./entrypoint.sh / RUN chmod +x /entrypoint.sh ENTRYPOINT ["/entrypoint.sh"] CMD ["param1","param2"]
In this combination, when ENTRYPOINT is set up in exec form and CMD is also in exec form, CMD provides the parameters to ENTRYPOINT.
Then, execute docker run without any parameter, CMD parameters will take effect, otherwise if some parameters are given, CMD parameters will be overridden.
Learning to leverage the Docker command tools such as
ENTRYPOINT
and combining them effectively with Shell scripts and other parameters gives you the flexibility and control needed when managing multiple containers in your application’s ecosystem.Given that the question asks about incorporating shell script files with Docker Entrypoint, we first need to understand what Docker and Entrypoint are. Docker is an open-source tool designed to automate deployment, scaling, and operating applications through containerization. It serves as a way to package up software and its dependencies into one unit. The Entrypoint in Docker is used to set a starting point for the containerized application.
Let’s now delve into the process of using Docker Entrypoint with a shell script file. Typically, you would place the entrypoint.sh (or your preferred-named script) at the root level of your application. You’ll then use this script to bootstrap your application.
Here is an example of a Dockerfile that copies an entrypoint.sh script into the image and runs it at start-up:
bash
FROM node:10
WORKDIR /app
ADD . /app/
COPY ./entrypoint.sh /
RUN chmod +x /entrypoint.sh
ENTRYPOINT [“/entrypoint.sh”]