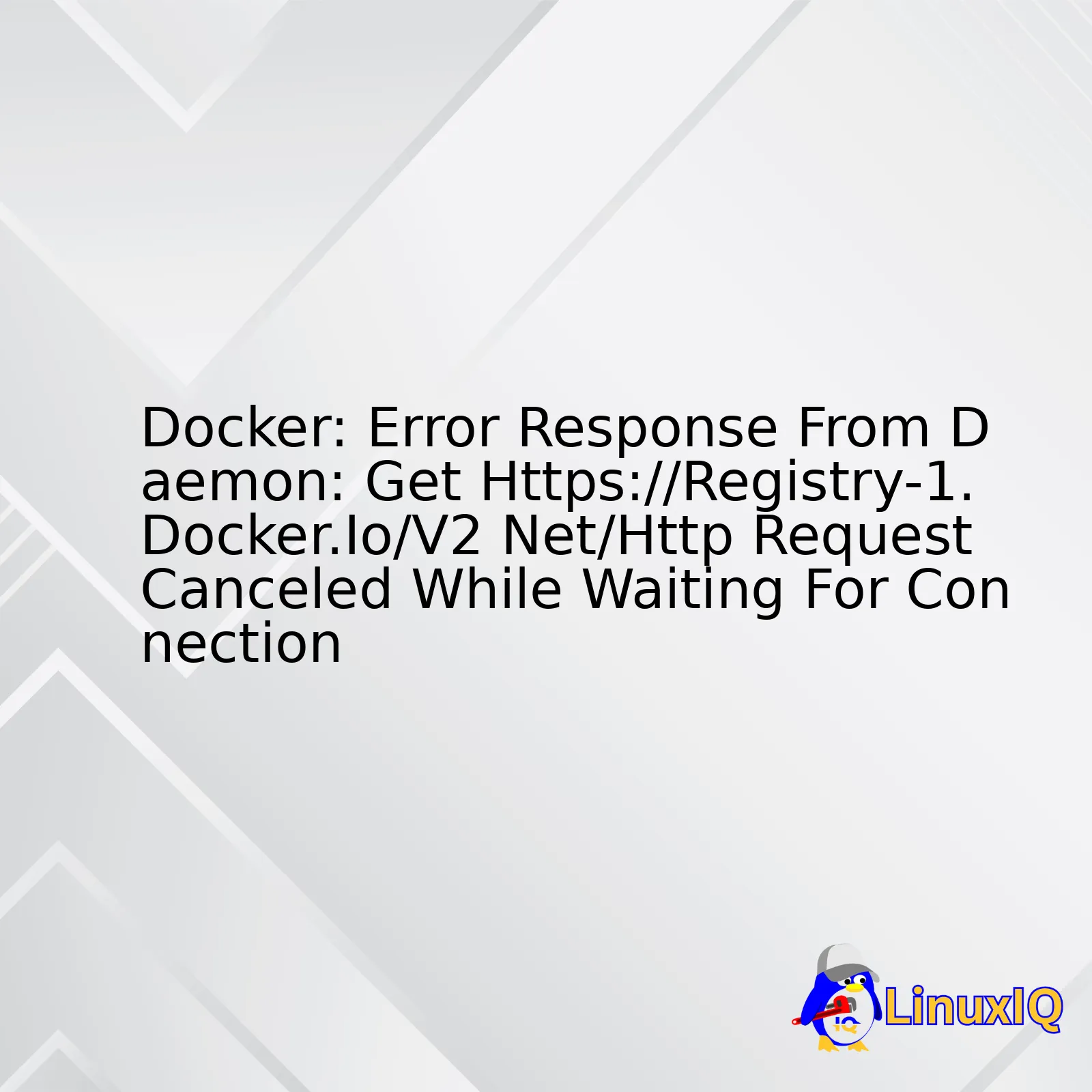
Error Message | Possible Causes | Suggested Solutions |
---|---|---|
Docker: Error Response From Daemon: Get Https://Registry-1.Docker.Io/V2 Net/Http Request Canceled While Waiting For Connection |
|
|
This error message, “Docker: Error Response From Daemon: Get Https://Registry-1.Docker.Io/V2 Net/Http Request Canceled While Waiting For Connection”, generally implies that Docker is having trouble interacting with the specified registry (in this case, registry-1.docker.io). In most cases, the causes of this error are tied to networking problems on the user’s end, rather than issues with Docker itself.
One common cause is intermittent or slow internet connectivity. Docker needs an active and stable network connection to communicate with its registries, so a disruption in service can cause requests to time out or fail completely. To ensure this isn’t the issue, you could simply check your network connection and try operating Docker while the internet speed is optimal.
Firewalls or security software could also be the culprits. If they’re configured too restrictively, they may be blocking or hindering Docker’s attempts to connect to the registry. This can typically be remedied by reviewing and adjusting these settings, ensuring that Docker has necessary permissions for accessing the network and making outbound connections.
Lastly, the error you provided might also come about due to issues in DNS configuration. Docker uses DNS to resolve registry endpoints, like registry-1.docker.io. A misconfigured DNS or one that’s merely functioning suboptimally might prevent Docker from successfully resolving these addresses. This problem can often be fixed by checking your computer’s DNS configurations and changing them if needed.
Knowing these common issues and their suggested solutions will certainly aid in quickly rectifying such occurrences when using Docker. In this way, you’d continue to harness Docker’s full potential for development without much interruptions. One interesting tidbit to read up on fixing DNS errors pertaining to Docker can be found at Docker Documentation. Here, you’d notice that they dive deeper into showcasing how systemd-resolved or dnsmasq can interfere with Docker containers and provide ways to mitigate these issues.
To handle this error programmatically, you would usually begin by provisioning for some error handling mechanisms. For instance, writing custom exception classes tailored specifically for these error instances could be helpful. An example in Python looks like this:
class NetworkError(Exception): pass ... try: # Code that interacts with Docker except ValueError as ve: raise NetworkError("There was a problem with the connection") from ve
This approach should allow you more control over tackling these exceptions and offer more freedom in deciding how to handle them. However, this topic roams into detail worth exploring separately.Understanding the “Error Response from Daemon” issue necessitates a deep dive into Docker – a popular open-source platform used for developing, shipping, and running applications. This error often stems from Docker being unable to establish a proper connection with the Docker Registry.
When you see an error message along the lines of “Get https://registry-1.docker.io/v2: net/http: request canceled while waiting for connection,” it generally means that when Docker tried to reach out to the Docker Registry, something went wrong. The display of this error is central to one major reason – connectivity problems.
Here are pertinent causes of such an error:
* Poor internet or network connectivity
* Proxy settings misconfigurations
* DNS issues
* Firewall or security group restrictions
* Docker daemon not properly configured
Ways to resolve could include the following:
1. **Check your Internet Connection:** Ensure that the internet connection you’re using is stable and reliable. Unstable connections can disrupt communication between Docker and the Docker Registry.
ping registry-1.docker.io
This command helps to verify if the connection to Docker Registry is successful or not. If ping does not receive any packets back, this indicates a connectivity problem.
2. **Review Proxy Settings:** Misconfigured or incorrect proxy settings can interfere with Docker’s ability to interact properly with the Docker Registry.
You might need to define your HTTP/HTTPS proxy environments in your Docker configuration file:
{ "proxies": { "default": { "httpProxy": "http://127.0.0.1:3001", "httpsProxy": "http://127.0.0.1:3001" } } }
3. **Address DNS Issues:** The Docker daemon uses the system’s DNS server by default. If you have problems with your DNS server, you might modify the Docker settings to use 8.8.8.8 DNS servers.
{ "dns": ["8.8.8.8"] }
4. **Examine Firewalls/Security Groups:** Sometimes, specified firewall rules or stringent security groups may block Docker’s access to the Docker Registry.
5. **Configure Docker Daemon Appropriately:** Sometimes, docker hosts remain misconfigured causing communication hardships.
If none of these solutions work, you might need to further analyze your system or connect with Docker support.
Here’s the table summarizing both the problem and possible steps to solution:
Error Message | Possible Causes | Steps to Resolve |
---|---|---|
Error response from daemon: Get https://registry-1.docker.io/v2/: net/http: request canceled while waiting for connection | Poor Internet Connection Incorrect Proxy Settings DNS Problems Restrictive Firewall Rules Misconfigured Docker Daemon |
Ensure Reliable Internet Connect Check Proxy Configuration Identify DNS Issues Review Firewall/Security Group Constraints Configure Docker Daemon Correctly |
For more in-depth information, consider checking official Docker documentation or connecting with the active community on Docker forums. These resources can provide a wealth of troubleshooting tips and expert advice.
Errors like this can be frustrating, but they are also an opportunity to understand Docker and its interaction with your systems better. Happy Dockering!Every now and then, as a coder, you might encounter issues while trying to interact with the Docker registry. One such problem that’s quite common is the ‘Error response from daemon: Get https://registry-1.docker.io/v2: net/http: request canceled while waiting for connection’ error message.
The primary reason for this issue is a disruption in the network connectivity between your local docker client and the docker registry server. Here are some culprits that might be causing the Docker connection errors:
Firewall or Security Policies:
Specific corporate policies or firewalls prohibit outbound connections from internal networks to the public internet. If you’re operating from corporate or highly secure networks, ensure that the firewall rules allow your client to connect to https://registry-1.docker.io/.
sudo ufw allow out toport 443
Network Settings and Proxy:
Incorrect proxy settings or network configurations like DNS settings also can lead to this issue. Please check if your proxy settings are correct in the Docker config file or system variables. Docker supports HTTP/HTTPS proxy and FTP proxy.
{ "proxies": { "default": { "httpProxy": "http://127.0.0.1:3001", "httpsProxy": "http://127.0.0.1:3001", "noProxy": "*.test.example.com,.example2.com" } } }
HTTPs Not Enforced:
If HTTPS isn’t enforced while connecting to the Docker registry, it can lead to this Docker connection error. Use the HTTPS endpoint while interacting with Docker’s registry.
{ "disable-legacy-registry": false, "Insecure-registries" : [], "debug" : true, "registry-mirrors" : ["https:///"] }
Infrastructure Issues:
Sometimes, infrastructure issues at Docker–like planned maintenance or an unexpected outage–can cause connectivity issues. You can always verify the status of Docker services by visiting their status page at https://status.docker.com/.
Remember, when dealing with this Docker connection error, the actual solution will entirely depend on your individual context (network configurations, security policies, et cetera). I hope taking into consideration these mentioned probable causes aids you in rectifying the issue and getting back to containerizing without any hindrance!
Arguably, one of the most critical facets of software development and deployment are containers- which is a method of virtualization that enhances consistency in deployment environments. Docker, being one of the most commonly used container management tools, makes tasks easier for developers by simplifying software delivery processes. Thus, it’s crucial to understand certain issues related to Docker operations and how to resolve them.
Error Response from Daemon
Before diving into the error response from daemon (that happens due to an HTTP Request failure), we need to have a basic understanding of what a Docker daemon really is:
The docker daemon is a persistent background process that manages Docker containers – meaning, it controls all aspects of Docker, including running Docker service requests, building Docker images, etc. It can run on the host system or be connected via socket or a RESTful API.
In the given context, Docker encounters an error while trying to pull an image from Docker Hub’s registry. Subsequently, the error returned is related to the failure of the HTTP request because it was canceled while waiting for the connection. The error message ‘docker: Error response from daemon: Get https://registry-1.docker.io/v2/: net/http: request canceled while waiting for connection’ could stem due to several reasons:
- Internet Connection Issues:
The most common cause is connectivity problems. A broken internet connection, an unreachable Docker registry server due to network congestion or DNS issues can lead to these errors. - Firewall or Network Policy restrictions:
Sometimes firewall settings might be stopping Docker from reaching the server it needs to connect to. If your system has strict rules set in place, these might hinder the Docker daemon from accessing external servers.
HTTP Requests within the Docker Ecosystem
The Docker Engine utilizes a client-server architecture. The Docker client communicates with the Docker daemon primarily via HTTP requests. When you use Docker CLI commands, the client sends these as HTTP requests to the Docker daemon, which then communicates back to the client with HTTP responses.
For instance, when Docker tries to pull an image, the Docker client sends an HTTP GET request to the Docker daemon. In turn, the daemon sends an HTTP GET request to DockerHub – the default public Docker registry.
The error message explicitly indicates a connection issue during this HTTP request process. While the Docker daemon attempts to make an HTTP GET request to retrieve specified files from DockerHub, the request is cancelled due to an unsuccessful connection. You can assume that there is either a networking issue from the system or a possible outage or high load on the DockerHub side.
$ docker pull hello-world Using default tag: latest Error response from daemon: Get https://registry-1.docker.io/v2/: net/http: request canceled while waiting for connection (Client.Timeout exceeded while awaiting headers)
This code snippet presents a situation where you’re trying to pull the ‘hello-world’ image using Docker. However, due to some connectivity issue, the HTTP request fails, and therefore, Docker can’t fetch the desired image.
Solution | Explanation |
---|---|
Check Internet Connectivity | Start by checking if your internet connection is working correctly. Try pinging Google servers or Docker’s registry servers. Ensure DNS is resolving properly. |
Verify Firewall Settings | Scrutinize your firewall configurations to confirm whether they’re not obstructing Docker from making HTTP requests to external servers. |
Check For Outages | If everything else seems to work fine, check if DockerHub isn’t down. This possibility can be quickly explored at Docker’s status page at https://status.docker.com/. |
HTTP requests play a significant role in how Docker operates. Any interruption in these requests, such as network instability, configuration discrepancies or server unavailability can lead to different Docker daemon errors. Understanding and analyzing these errors thoroughly allows us to troubleshoot and respond effectively to maintain a smooth operational flow in our deployment environment.
Absolutely, I’d be happy to help untangle this error message and provide a solution.
If you’re facing issues with the error
docker: Error response from daemon: Get https://registry-1.docker.io/v2: net/http: request canceled while waiting for connection (Client.Timeout exceeded while awaiting headers)
, it suggests that there’s a networking issue when your Docker client tries to connect to the Docker registry.
A piece of the puzzle comes from understanding how Docker works. Docker, in its essence, is nothing more than a client-server application. The Docker client communicates with the Docker daemon, which does all the heavy lifting of building, running, and managing Docker containers. When we run commands such as
docker pull
or
docker run
, the Docker client sends these commands to the Docker daemon, which carries them out. While running these commands, the Docker daemon needs to fetch relevant Docker images hosted on Docker registries. In this case, it seems, the Docker daemon is having trouble connecting to the Docker registry at `https://registry-1.docker.io/v2`.
Before we go over the potential fixes, let’s broadly break down some of the primary causes behind this issue:
– Network connectivity issues
– Docker daemon configuration problems
– DNS resolution problems
To work our way through the issue, we can address these common culprits one-by-one:
Network Connectivity Issues:
Attempt to ping registry-1.docker.io by typing:
$ ping registry-1.docker.io
. If the ping fails, then you have a network connectivity issue. This may require a connection troubleshooting or contacting your internet service provider for further analysis.
Docker Daemon Configuration Problems:
Ensure Docker daemon is configured properly. Misconfiguration could lead to unexpected behavior like timeouts. You can check and modify Docker configuration using Docker’s built-in command-line interface. Use the following commands to view, inspect and update Docker configurations:
docker info
,
docker config inspect
, and
docker config update
DNS Resolution Issues:
Docker uses DNS servers to resolve domain names, and the most commonplace it could go wrong is the misconfiguration of the DNS settings. As a simple test, try pinging a well-known address:
$ ping www.google.com
. If the ping resolves to an IP address, DNS is working fine. Alternatively, you might want to use custom DNS settings, say Google’s public DNS servers. That can be done by editing the Docker daemon’s JSON file, usually located at /etc/docker/daemon.json. Remember to restart Docker for changes to take effect:
$ sudo systemctl restart docker
.
While the above steps will likely help clear up any potential networking issues, do remember that Docker errors – like many technology-related issues – can sometimes stem from rather unique and situation-specific scenarios. Hence, if the problem persists, it could be valuable to seek out expert advice on platforms like [Stack Overflow](https://stackoverflow.com/questions/tagged/docker), [Docker Forums](https://forums.docker.com/) and GitHub’s [Docker CLI Issue Tracker](https://github.com/docker/cli/issues).The error message “
Error response from daemon: Get https://registry-1.docker.io/v2: net/http: request canceled while waiting for connection
” is Docker’s way of communicating that it’s unable to reach a Docker registry for downloading images.
There are several scenarios and setups where you may get this error. A few of these instances are explained below:
1. Network Connectivity Issues:
This error often shows up when Docker can’t connect to the internet due to network connectivity issues. It could be due to an unstable WiFi, or down Ethernet connections. Resolutions for this would include ensuring your machine has stable internet access, retrying after sometime, checking firewall and security software settings.
2. Incorrect Proxy Configurations:
This problem may occur if there are incorrect proxy configurations. If you’re relying on proxies for networking, ensure that the details are correct. The Docker client must be configured to use the right proxy. This could typically look something like this:
{ "proxies": { "default": { "httpProxy": "http://192.168.1.100:3128", "httpsProxy": "http://192.168.1.100:3128", "noProxy": "*.test.example.com,.example2.com" } } }
3. DNS misconfigurations:
DNS Misconfiguration can also cause this problem. It’s possible Docker might not be able to resolve https://registry-1.docker.io/v2.
You can check for this by running:
ping registry-1.docker.io
If the domain doesn’t resolve, you might consider using a different DNS server in your Docker configuration.
4. Security software interference:
There’s always a possibility that antivirus or another piece of security software may be interfering with Docker or blocking its connections. You might want to review the settings or temporarily disable such software just long enough to test whether they’re causing the issue.
To debug these kinds of errors, it’s always beneficial to start with the basic troubleshooting steps, progressing towards more complex ones only if necessary. The above suggestions aren’t exhaustive but serve as a good starting point for any ensuing docker connection issues.
Whether you’re an experienced coder or just starting with Docker, it’s always frustrating when an error like
Error response from daemon: Get https://registry-1.docker.io/v2
appears. Fortunately, this issue can be tackled by understanding its root causes and finding the solutions for each one of them.
The Problem:
The error related to Docker registry basically indicates that your Docker client is unable to connect to the registry server. If you look closely at the error message, connectivity is the primary issue here. This happens because Docker CLI communicates with Docker registries over HTTP/HTTPS. Therefore, when it fails, it might stem from several underlying issues.
Potential Causes:
If you’re receiving the ‘net/http: request canceled while waiting for connection’ error, these could be probable reasons:
- DNS configuration problems: Your system might have trouble resolving registry-1.docker.io.
- Firewalls or Proxies: Any sort of firewall or proxy restrictions could prevent your Docker client from interacting with the Docker registry.
- VPC settings: If you’re using Cloud-based resources, check your VPC network configurations.
- General Connectivity Issues: This can range from planned server downtime to network instability on your end.
Investigating and Fixing the Issue:
Now that we’ve discussed potential causes, let me guide you on how to probe further into them:
- For DNS configuration problems, try pinging registry-1.docker.io from the terminal. If you cannot reach the host, it might be a DNS resolution issue. Something like the following code should do the trick:
ping registry-1.docker.io
Look into modifying your DNS to use Google’s public DNS.
- If you suspect it’s a firewall or proxy issue, momentarily disable them and attempt to execute your Docker commands again.
- In case of VPC settings, make sure the necessary ports (like port 443 for HTTPS) are open for communication.
- If these steps fail to resolve the problem, check your internet connectivity. Run other services to verify if the connectivity issue persists.
Docker Configuration Check:
Additionally, inspect the Docker client configuration file present at
~/.docker/config.json
. A misconfiguration in this file might lead to the issue. A typical configuration looks something like this:
{ "auths": { "https://index.docker.io/v1/": { "auth": "your-auth-token" } } }
Please replace the ‘your-auth-token’ with the actual one. It’s a base64 encoded string of your username and password. Finally, please ensure that Docker is properly installed and updated to the latest version.
Hence, by systematically investigating all plausible problems, you can deduce the cause behind the
Error response from daemon: Get https://registry-1.docker.io/v2
error message and apply suitable fixes. Always ensure to keep your systems up-to-date and correctly configured to avoid such problems. More information regarding Docker errors and their solutions can be found on the official Docker documentation.
When you encounter the error message “Docker: Error response from daemon: Get https://registry-1.docker.io/v2 net/http request canceled while waiting for connection,” it suggests an issue with Docker’s ability to connect to the remote Docker registry. This might be caused by network problems, proxy settings, DNS issues, or occasionally outdated Docker versions.
Understanding Network issues
Docker functions rely heavily on networking, so connectivity issues can lead to the error above. The RAM, hard drive and CPU usage of your system should be checked using:
$ top # for Linux $ Activity Monitor # for macOS $ Task Manager # for Windows
Tools like SpeedTest could be used to check the network quality. A stable high-speed internet connection is necessary to download images from Docker Hub. If the issue persists following these checks, then a possible solution would be checking Docker’s configurations.
Checking Docker Proxy Settings
By acting as intermediaries, proxies control the flow of information between servers and clients. Incorrectly built or absence of a configured proxy could result in this Docker error. Your Docker system proxy can be adjusted via below commands:
// To set proxy variables permanently add the following lines in /etc/environment/ on Linux, ~/.bashrc on Mac
$ export http_proxy='http://proxy-server.io:8080' $ export https_proxy='http://proxy-server.io:8080' $ export ftp_proxy='http://proxy-server.io:8080' $ export no_proxy="localhost,127.0.0.1,localaddress,.localdomain.com"
For accurate proxy settings for macOS and Windows, look at the official Docker documentation or StackOverflow posts.
Resolving Docker DNS issues
Docker may fail to resolve domain names due to DNS issues. Try to ping registry-1.docker.io and see if you’re getting responses. If that fails, you can consider setting up public DNS servers (like Google 8.8.8.8, 8.8.4.4) in Docker or local machine settings.
// On Linux and Mac machines, include the following command:
$ echo "nameserver 8.8.8.8" | sudo tee /etc/resolv.conf > /dev/null $ docker info --format ''
// Docker Desktop users (Windows and Mac) can manually set DNS servers through GUI under ‘Resources -> Network’.
Updating Docker
Ensure that you are using the latest version of Docker. Outdated Docker software can cause problems including network disruptions. Docker versions can be updated with these commands.
On Linux:
$ curl -fsSL https://get.docker.com -o get-docker.sh && sh get-docker.sh
On MacOS:
Use Docker Desktop.
On Windows:
Update to the latest release here.
Following the above troubleshooting steps, adjusting network settings, resolving proxy issues, DNS troubleshooting and ensuring your Docker is up-to-date will potentially resolve the “Get https://Registry-1.Docker.Io/V2 Net/Http Request Canceled While Waiting For Connection” error. However, remember that Docker relies on many sub-systems of your computer. If the problem remains insistent, further investigation might have to be performed within your operating environment.The error message
Get https://registry-1.docker.io/v2/: net/http: request canceled while waiting for connection
typically indicates a connectivity issue between your Docker client and the Docker Hub repository. Several factors can contribute to this problem, one of which being your firewall settings.
Firewalls are designed to monitor and control incoming and outgoing network traffic based on predetermined security rules. While their primary purpose is to create a barrier between your internal network and incoming traffic from external sources (to prevent attacks), they can unintentionally block or interfere with legitimate traffic, depending on the security rules in place.
When you are running commands that interact with the Docker Hub repository, such as
docker pull
or
docker push
, your Docker client needs to establish a connection with the Docker registry situated at https://registry-1.docker.io. Docker uses HTTP/S for transmitting communications back and forth – known as API calls (source here). If the firewall identifies these connection requests or the subsequent traffic as potentially harmful or not white-listed, it could block or drop the packets which results in disruption in communication and leads to the aforementioned error.
To solve the above docker error related to the firewall, there are a few methods:
• Check your Firewall Settings: Validate if any rule in the firewall is blocking the connection between the Docker Client and Docker Hub. In case of firewalls like iptables, ufw or firewalld, you could use their respective commands to check and manage the configuration
# iptables example iptables -L # ufw example ufw status # firewalld example firewall-cmd --list-all
• Modify Firewall Rules: Based on the output, modify the specific rules which block the connections. Most often, allowing outgoing connections to registry-1.docker.io should help. You can achieve this by adding an allow rule to your firewall configuration.
Just remember, while modifying the rules keep your company’s IT and security policies in mind, we do not wish to open up unwanted gateways exposing our infrastructure to potential risks.
• Using a Proxy server – If your enterprise has strict firewall rules, consider utilizing a Proxy server with Docker. This allows the Docker daemon to access external networks through that proxy. This would require configuring the Docker service to make HTTP/HTTPS requests via the proxy.
Here is an example of how to configure the Docker client to use a proxy:
# Linux export http_proxy="http://proxy.example.com:8080" export https_proxy="http://proxy.example.com:8080" # Windows $Env:http_proxy = "http://proxy.example.com:8080" $Env:https_proxy = "http://proxy.example.com:8080"
These are just a few potential ways that firewalls can impact Docker’s registry and connections, and some solutions to mitigate these issues. For more complex setups, additional factors and solutions might be relevant.Network proxies are crucial in administering internet traffic and they significantly affect Docker operation. In essence, a network proxy represents a computer server acting as an intermediary for requests from clients seeking resources from other servers. Essentially, it serves as the gateway between your local network and large scale networks like the internet.
It’s important to understand that during Docker operation, one might encounter errors such as:
Error response from daemon: Get https://registry-1.docker.io/v2/: net/http: request canceled while waiting for connection
Several reasons might evoke this error response and curiously, a misconfigured network proxy could be one of them. Here’s why:
• Incorrect Proxy Settings: This typically surfaces if you’re operating behind a proxy and Docker isn’t configured to use it. Consequently, Docker cannot connect to the outside world, leading to the error message. It’s important to ensure that the HTTP_PROXY or HTTPS_PROXY environment variables are set correctly. For example,
HTTP_PROXY=http://proxy.example.com:80/ HTTPS_PROXY=http://proxy.example.com:80/ NO_PROXY=*.test.example.com,.example2.com