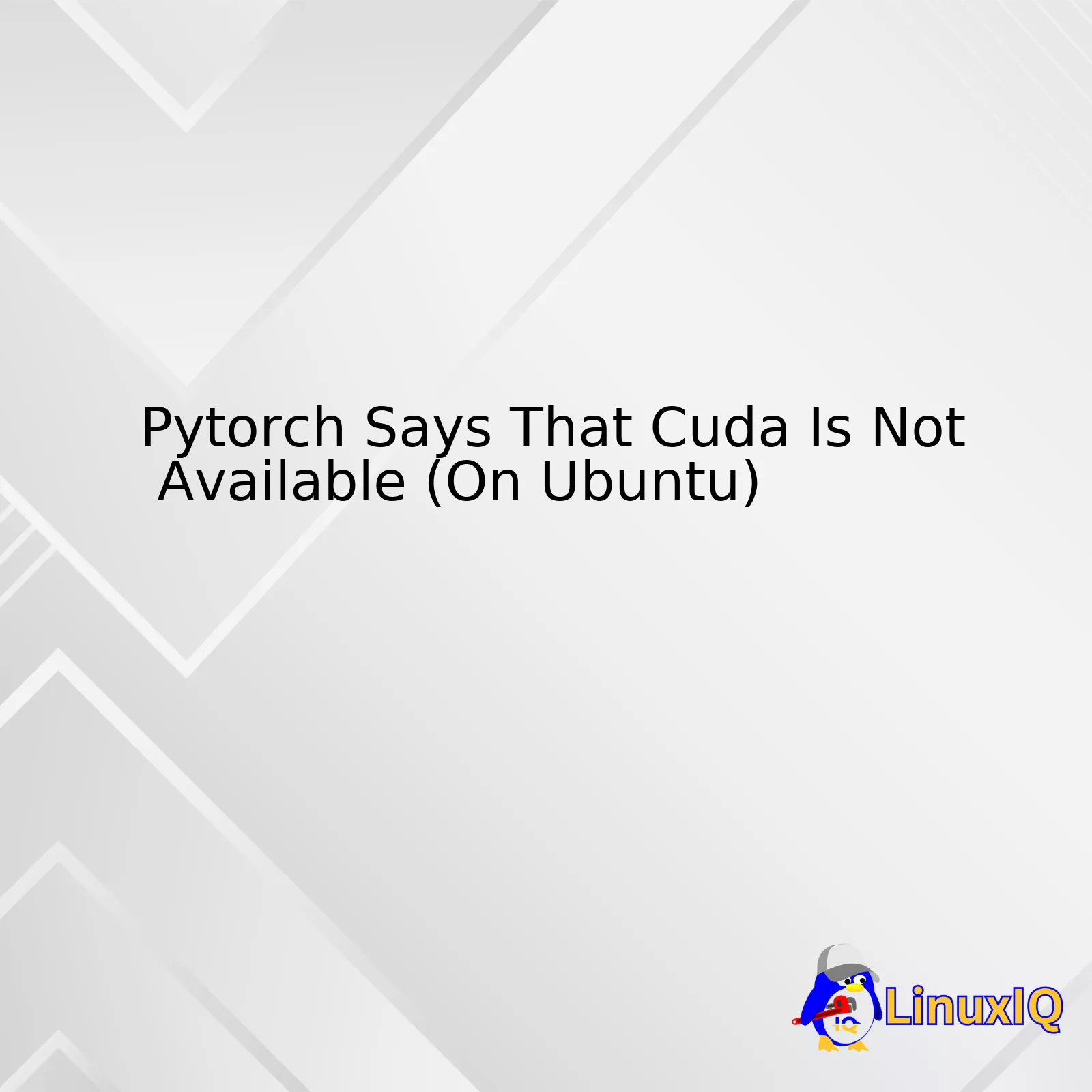
Issue | Possible Cause | Solution |
---|---|---|
PyTorch says that CUDA is not available | GPU not CUDA-capable or CUDA drivers not installed | Upgrade GPU or install/update CUDA drivers |
Incorrect version of PyTorch installed | Install the correct version of PyTorch that supports CUDA | |
Environment variables not set correctly | Correctly configure environment variables |
When it comes to PyTorch indicating that CUDA is unavailable on an Ubuntu system, there are several possible reasons behind this. One possibility is that your GPU is simply not CUDA-capable. An unfortunately common oversight, especially in machines which aren’t built with computation-intensive tasks such as deep learning in mind.
If you’re certain your GPU is indeed CUDA-capable, then the issue might lie in the absence, non-updating, or incorrect installation of the requisite CUDA drivers. Ensure to have the compatible NVIDIA CUDA Toolkit installed. You can verify this by running
nvidia-smi
in your terminal. It should give you information about your driver and CUDA version.
Beyond these hardware and driver-related issues, an incorrectly installed version of PyTorch could also be the culprit. It’s important to install the build of PyTorch that corresponds appropriately with your CUDA version. If you’ve installed PyTorch via pip or conda, ensure you specify the correct CUDA Toolkit version like so:
conda install pytorch torchvision torchaudio cudatoolkit=10.2 -c pytorch
.
Lastly, if there’s still an unavailability message, it’s possible that your environment variables (like PATH and LD_LIBRARY_PATH) associated with CUDA may not be set properly. Be it due to manual adjusting or some upsets during installation processes. Here, checking and rectifying any inaccuracies in the variable settings would be the necessary step.
To handle any uncertainties, checking official PyTorch and/or CUDA documentation, consulting online tech forums like [StackOverflow](https://stackoverflow.com/), or assistance from knowledgeable peers can go a long way in resolving such issues. (source).When using PyTorch on Ubuntu, you may encounter a common problem: PyTorch fails to recognize the availability of CUDA. This issue often manifests itself with error messages that reflect `
torch.cuda.is_available()
` returning False, thus leading to an understanding that CUDA is unavailable.
Why does this happen?
This error can emerge due to several reasons:
– CUDA is not installed properly or at all.
– PyTorch version installed doesn’t support the available CUDA version.
– NVIDIA drivers are either missing or outdated.
– Incompatible GPU (limited to GPUs that supports CUDA).
How to resolve these issues?
To resolve these emerging matters, you could simply follow these steps:
1. CUDA Installation.
In the event you do not have CUDA installed, you’ll need to download and install it from the NVIDIA website. You will find detailed Linux command line installation instructions specific to your Linux distribution.
Example code snippet would be:
sudo apt update sudo wget https://developer.download.nvidia.com/compute/cuda/repos/ubuntu1804/x86_64/cuda-repo-ubuntu1804_10.0.130-1_amd64.deb sudo dpkg -i cuda-repo-ubuntu1804_10.0.130-1_amd64.deb sudo apt-key adv --fetch-keys https://developer.download.nvidia.com/compute/cuda/repos/ubuntu1804/x86_64/7fa2af80.pub sudo apt-get update sudo apt-get install cuda
After installing, add CUDA to the environment path using:
export PATH=/usr/local/cuda-10.0/bin${PATH:+:${PATH}} export LD_LIBRARY_PATH=/usr/local/cuda-10.0/lib64${LD_LIBRARY_PATH:+:${LD_LIBRARY_PATH}}
To confirm successful installation use command:
nvcc --version
2. PyTorch Version.
Ensure that the installed PyTorch version aligns with your CUDA version. To check which PyTorch version you have, run:
pip3 show torch
Should there be any inconsistencies, reinstall PyTorch to match the CUDA version. For example, if CUDA 10.0 is installed, the required PyTorch installation command would be:
pip3 install torch==1.5.0+cu101 torchvision==0.6.0+cu101 -f https://download.pytorch.org/whl/torch_stable.html
3. NVIDIA Driver Update.
You can check your current NVIDIA driver version by executing:
nvidia-smi
Should your system require an updated driver, proceed to the official NVIDIA website and download the latest/necessary driver. After installation, reboot your system.
Finally, it’s worth mentioning that not all graphics cards support CUDA. Thus, before proceeding with the above steps, please ensure that your GPU adheres to the CUDA-enabled GPU list by NVIDIA.
Sidebar: Torch Test for CUDA
The simplest method to verify that PyTorch is recognizing CUDA after these steps is by running this short diagnostic test:
import torch print(torch.cuda.is_available())
It should return ‘True’ if everything is configured correctly. If ‘False’, review the steps stated earlier, ensuring every detail is addressed meticulously. Remember, correct configuration assures optimized PyTorch performance!One common issue when using PyTorch, particularly on Ubuntu, is getting the message: “Cuda Not Available.” We will now explore several key steps and strategies that can assist in resolving this issue.
Check System Cuda Compatibility
Firstly, you need to make sure your system is capable of supporting CUDA. The NVIDIA card installed in your device must be compatible. You can check this by going to the NVIDIA website and checking their list of CUDA-enabled GPU chart.
If your GPU is in the supported list then you have the capability to utilize CUDA technology. If not, unfortunately, you cannot progress further as CUDA requires a compatible NVIDIA graphics card.
Ensure Correct Installation Of CUDA Toolkit and cuDNN
The next step involves ensuring that the CUDA Toolkit and cuDNN library have been correctly installed. Below are some commands for installing these tools:
sudo apt-get install nvidia-cuda-toolkit
sudo apt install libcudnn7-dev
Remember, you need matching versions of Nvidia drivers, CUDA, cuDNN and PyTorch for everything to work smoothly.
Install PyTorch With CUDA Support
PyTorch needs to be installed with CUDA support. When installing PyTorch via pip or conda, ensure you specify a PyTorch version that corresponds to your version of CUDA. Here’s an example installation command:
pip install torch==1.8.0+cu111 torchvision==0.9.0+cu111 -f https://download.pytorch.org/whl/torch_stable.html
This will install PyTorch 1.8.0 with CUDA 11.1 support. Make sure to select the PyTorch version that is compatible with your CUDA version.
Verify Your PyTorch and Cuda Installation
After completing installation, it is crucial to verify that both PyTorch and the CUDA libraries are working correctly.
You can test your PyTorch installation using:
import torch print(torch.__version__)
To check CUDA availability in PyTorch, execute:
torch.cuda.is_available()
Ideally, this should return ‘True’. If it returns ‘False’, CUDA isn’t properly recognized by PyTorch on your device.
Avoid Multiple Installations
Multiple installations of CUDA can cause conflicts resulting in CUDA not being available in PyTorch. If you have different CUDA versions installed try removing them. It’s recommended to keep only one version that is required by your application.
All these steps, when carefully followed, should solve the CUDA-not-available issue in PyTorch. Remember, an integral aspect of diagnosing and fixing problems is patient, methodical troubleshooting.
The unavailability of CUDA on PyTorch for Ubuntu often has several repercussions, especially centered around performance degradation and increased computation times. But before delving into the details, let’s quickly understand what CUDA essentially is.
CUDA (Compute Unified Device Architecture) is a parallel computing platform and application programming interface model developed by NVIDIA. It uses the power of NVIDIA’s Graphics Processing Units to achieve better computational performance in applications such as Machine Learning and data processing that involve heavy Matrix calculations.
PyTorch, a popular deep learning framework extensively used in artificial intelligence and machine learning projects, can leverage the computational power of CUDA to run operations on the GPU instead of the CPU. This execution style offers a significant speed boost, considering a GPU can handle a large number of threads simultaneously, making it faster and suitable for vast computations involved in deep learning. Not having CUDA could thereby slow down the overall processes considerably.
# Check if CUDA is available import torch print(torch.cuda.is_available())
Running the above code snippet should return True if CUDA is available. If it returns False, PyTorch would resort to using only your system’s Central Processing Unit (CPU), affecting PyTorch performance.
1. Increased Computation Time
Without access to GPUs through CUDA, computations take more extended periods because CPUs are not as efficient at performing mathematical calculations required in machine learning tasks, slowing down your model’s training and prediction timeframes.
2. Restricted Access to Libraries
Some libraries optimized for GPUs, such as cuDNN, require CUDA, which boosts Convolutional Neural Networks’ performances. Without CUDA, such libraries cannot be utilized, further hampering the potential development speed and efficiency.
3. Hindered Scalability
Larger models requiring more memory space or demanding more substantial computational power might face hindrances without CUDA. This limitation restricts scalability to larger datasets or more sophisticated neural networks.
If you’re grappling with CUDA’s unavailability issue on PyTorch while operating on Ubuntu, there could be a few reasons:
- Your GPU might not be compatible or supported by CUDA.
- CUDA may not have been installed correctly.
- There may be some issues with the PATH environment variable regarding CUDA toolkit’s location.
- PyTorch version you’re using may not be compatible with your CUDA version.
Solutions generally include verifying your setup or rectifying any underlying compatibility, installation or environmental issues. The best course of action is checking PyTorch’s official installation guide or NVIDIA’s CUDA installation guide to ensure all requirements are met.
Note: Keep in mind that running models in GPU through CUDA doesn’t always signal a speed boost if your model is relatively small or your batch size isn’t significantly large. The benefit becomes evident with large, complex models where the CPU’s processing power would be a considerable bottleneck.
Before digging into the nitty-gritty of the problem, let’s first understand the context. PyTorch is a popular open-source machine learning library primarily developed by Facebook’s AI Research lab. CUDA, on the other hand, is a parallel computing platform and application programming interface model created by Nvidia allowing developers to use Nvidia GPUs for general purpose processing. Now, when PyTorch says CUDA is not available, it simply means your GPU (Nvidia) cannot be utilized for computations.
So, why does this happen? Here are some possible causes:
– There is no CUDA capable device installed.
– The installed version of PyTorch has not been compiled with CUDA capabilities.
– Incorrect version of CUDA toolkit is installed.
Now, focus on solutions that can get rid of the issue ‘PyTorch says CUDA is not available.’
First: Verify CUDA-capable Device Exists
Run the following command in your terminal to confirm if you have a CUDA-capable device:
lspci | grep -i nvidia
If it returns something, it means you have a CUDA-capable device.
Second: Reinstall PyTorch With CUDA Support
It could be possible that you installed PyTorch with CPU-only support. Check this by running:
python -c "import torch; print(torch.cuda.is_available())"
It’ll return False if you do not have CUDA support. Reinstall PyTorch but this time, make sure to include the CUDA toolkit. For instance, if you have CUDA 10.2, install PyTorch using:
pip install torch==1.7.1+cu110 torchvision==0.8.2+cu110 torchaudio===0.7.2 -f https://download.pytorch.org/whl/torch_stable.html
Third: Install Correct Version of CUDA Toolkit
Check what CUDA version PyTorch needs by using the following Python commands:
import torch print(torch.version.cuda)
This will return the CUDA version that PyTorch is looking for. Installation process may vary depending on your Ubuntu version. Usually, you download the correct CUDA toolkit from Nvidia’s official website.
After downloading the correct version, navigate where it was downloaded and run the following commands:
sudo dpkg -i cuda-repo-ubuntu1604-8-0-local-ga2_8.0.61-1_amd64.deb # Substitute with your downloaded file sudo apt-get update sudo apt-get install cuda
Then, add the below lines to your .bashrc file for the system to find the executables.
echo 'export PATH=/usr/local/cuda-8.0/bin${PATH:+:${PATH}}' >> ~/.bashrc # Substitute with your appropriate CUDA folder
Finally, restart your computer for changes to take effect.
Following the aforementioned steps should typically solve the occurrence of PyTorch CUDA non-availability on your Ubuntu machine. Thousands of earlier troubleshooting experiences suggest that, majority of issues are attributable to either incorrectly installed CUDA toolkit, or unavailable CUDA support in PyTorch on your system. Therefore, ensuring correct versioning and re-installation processes, specifically tailored towards CUDA support, should help get your GPU acceleration up and running!As a professional developer, troubleshooting code issues like “Pytorch Says That Cuda Is Not Available (On Ubuntu)” is part of our everday tasks.
Before diving into the solution, let’s first understand what these technologies are on a basic level. PyTorch is a famous open source machine learning library and CUDA stands for Compute Unified Device Architecture, a parallel computing platform created by NVIDIA.
This issue generally occurs when PyTorch cannot find the CUDA installation on your Ubuntu system. There can be several reasons behind this, some of which include:
- Incorrect version of CUDA installed
- Improper PATH environment variable settings
- Mismatch between PyTorch and CUDA versions
Having understood how crucial it is to ensure proper compatibility and installation of PyTorch and CUDA, here’s how you can verify whether they’re installed correctly:
import torch print(torch.__version__) print(torch.cuda.is_available())
The above Python code simply prints out the current version of PyTorch in use and checks if CUDA is available or not.
Here are a few tips that could help manage such issues:
- Always cross-check the system requirements before installing any software.
- Ensure proper installation of CUDA and compatible drivers from the NVIDIA website.
- Make sure to match the PyTorch version with the CUDA toolkit version installed.
- Verify that the
PATH
environment variables are set correctly.
I hope this article provides you with a comprehensive understanding of the prevalent problem where PyTorch states that CUDA is not available on Ubuntu. Further, I hope the proposed solutions will assist in resolving the issue effectively. Keep in mind that correct installations and an update-to-date environment always aid in smooth and uncomplicated coding operations.
Remember, coding is fun and debug process enlightens us towards better coding style!