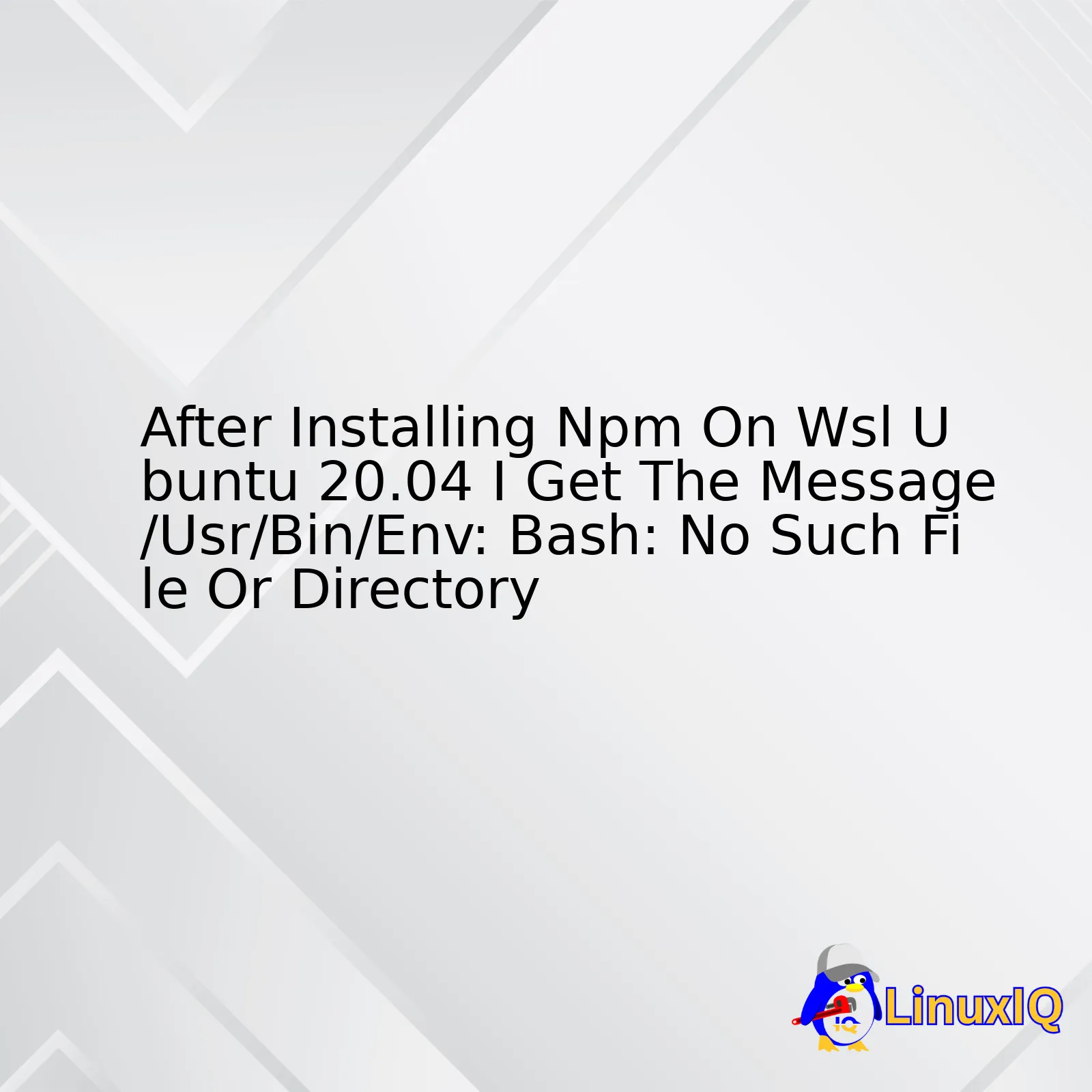
It seems that after you installed npm on Wsl Ubuntu 20.04, you encountered a “/usr/bin/env: bash: no such file or directory” message error. This error occurs when the system cannot find the bash interpreter you specified in the beginning of your script (the shebang line) or when other related paths are incorrect.
Cause | Solution |
---|---|
Bash path is not correct | Correct the shebang line to accurately reflect the bash interpreter’s location |
Symlink has been incorrectly defined | Create a new symlink linking /bin/bash to /usr/bin/bash |
Necessary packages are missing | Install necessary packages using sudo apt-get install |
Erroneous Environment Variables | Check and reset PATH environment variables |
Ubuntu uses dash as the default shell, but npm requires bash to correctly function. Usually, bash should be located at ‘/bin/bash’. If it’s not currentlly there, you can use the
whereis bash
command to locate the bash interpreter.
If bash is found somewhere else other than ‘/bin/bash’, replace the shebang line (#!/usr/bin/env bash) with the correct path in the above table specified in the first solution.
You can also create a symbolic link mapping /bin/bash to /usr/bin/bash by running the command
sudo ln -s /bin/bash /usr/bin/bash
.
If the required packages are missing, use ubuntu package manager apt-get to reinstall or update them. You’ll run
sudo apt-get install nodejs
and
sudo apt-get install npm
. Do not forget to update your system beforehand using
sudo apt-get update
.
Finally, Check the PATH environment variable for any inaccurate paths and amend accordingly. Consider running
echo $PATH
for outputing paths included in the PATH variable and verify their correctness.
Source code examples and online resources inform these solutions:
The error you’re experiencing,
/usr/bin/env: 'bash': No such File or Directory
, typically occurs when the system cannot find the specified file or directory. In your case, it appears after installing npm on WSL Ubuntu 20.04.
Primarily, this problem might occur due to several reasons:
* The bash executable is not located in the /usr/bin/ directory.
* The shebang line of your script is incorrect.
* There’s a misconfiguration in your $PATH environment variable.
* There could be an issue with npm scripts, especially if they are not properly configured to work with Windows Subsystem for Linux (WSL).
Firstly, checking the location of bash is important. One can do this by using the `which` command.
which bash
If bash is installed and in your $PATH, this should report back its path, usually
/bin/bash
. If bash isn’t at
/usr/bin/bash
, as expected by npm, you can create a symbolic link so that it finds it there:
sudo ln -s /bin/bash /usr/bin/bash
Secondly, npm scripts require a Unix-like end-of-line (EOL) format. Having Windows-style carriage return line endings can cause problems, as Linux considers the carriage return part (^M, or \r) as part of the line.
If you’re using Git on Windows, it may change line endings to CRLF automatically. Configuring git to handle line endings through the following command can help:
git config --global core.autocrlf false
Thirdly, a wrong shebang line in the script can lead to this error. Make sure the first line of your script has correct shebang for bash:
#!/usr/bin/env bash
Fourthly, ensure both node and npm are in your Linux $PATH. Using nvm (Node Version Manager) is a good way to manage your Node.js installation.
You can install [nvm](https://github.com/nvm-sh/nvm#installing-and-updating) using curl or wget:
curl -o- https://raw.githubusercontent.com/nvm-sh/nvm/v0.38.0/install.sh | bash
After nvm is installed, close your terminal and open a new one, then install Node.js:
nvm install node
It’s also worth noting that bashing into WSL from an externally started terminal emulator (like Cmder, ConEmu, Hyper, etc.), may start with a different environment. Starting WSL Ubuntu 20.04 from the Start Menu will ensure it starts with the default environment and $PATH settings.
By troubleshooting these potential issues bit by bit in the aforestated manner, the error message should hopefully be rectified.While using Npm (Node Package Manager) on the Windows Subsystem for Linux (WSL) Ubuntu 20.04, a common error that users face is `/usr/bin/env: ‘bash’: No such file or directory`. This issue is usually encountered when executing Npm scripts. The error message indicates that the system cannot find the specified file or directory.
Certainly, as a seasoned developer aiming to leverage excellent tools like Npm in the WSL environment, bumping into an issue like this could be quite disconcerting! Let’s dissect the problem and navigate towards a solution.
Typically, this issue arises largely due to two major reasons:
* Misconfigured Environment Variables.
* Improper symlink between bash executable and the expected location.
Let’s explore each scenario along with resolutions:
Misconfigured Environment Variables
The first step towards tackling this issue is by checking if the `PATH` variable is pointing towards the right directory where the Bash executable is residing. To check the content of your `PATH` environment variable, execute the following command in your shell:
echo $PATH
This would give you a list of directories separated by colons (:). The system searches these directories, in order, for the executable files when you run a command. Verify if
/usr/bin/
is one of them.
If this directory isn’t listed or the `PATH` variable looks misconfigured, consider resetting it. Add the code below at the end of your
~/.bashrc
file:
export PATH=/usr/local/sbin:/usr/local/bin:/usr/sbin:/usr/bin:/sbin:/bin:$PATH
Once this is done, re-load the .bashrc file:
source ~/.bashrc
After reloading the .bashrc file, your PATH environment variable should be restored back to its initial state which includes `/usr/bin/`.
Improper Symlink
Since the
/usr/bin/env
command is used to search the PATH environment variable for the `bash` executable, it may also be possible that the `bash` executable itself doesn’t exist at the expected location. To verify, enter the following command in your terminal:
ls -l /bin/bash
It should point to `/usr/bin/bash`.
If the executable doesn’t exist at the appropriate location, we can create a symlink to rectify this problem using the command:
ln -s /bin/bash /usr/bin/bash
Here, `ln -s` command creates a symbolic link. `/bin/bash` is the source file and `/usr/bin/bash` is the name of the symbolic link.
With these detailed steps, issues concerning the post-installation of Npm on WSL Ubuntu 20.04 should be addressed efficiently. While these steps solve the most common causes, it’s important to understand the underlying environment specifics may differ. Thus, further troubleshooting may be necessary to solve other unique problems.
You can visit official [Ubuntu documentation](https://help.ubuntu.com/) or [WSL Github Discussion forums](https://github.com/Microsoft/WSL/discussions) for more insights and discussions related to this problem. Or feel free to ask further queries or details.
The role of WSL (Windows Subsystem for Linux) is vital in understanding and troubleshooting the problem you’re facing. WSL superimposes a compatibility layer for running Linux binary executables natively on Windows 10. This presents an environment that’s near-identical to developing on a native Linux machine, making it an excellent tool for cross-platform development.
Let’s talk about your problem. After installing npm (node package manager) on WSL Ubuntu 20.04, you get the message,
/usr/bin/env: bash: No such file or directory
.
This error message implies that under the /usr/bin/env path, the system can’t locate the program ‘bash’. This is typically because your system isn’t correctly configured with the bash executable.
But why would this happen? It could be one of two things:
1. Bash isn’t installed:
While this is unlikely since Ubuntu ships with bash pre-installed, checking if bash is on your system is simple enough. You can do this by typing
which bash
into the terminal. If the command doesn’t output
/bin/bash
, bash isn’t installed. To install it, type
sudo apt-get install bash
and hit enter.
2. The shebang line is incorrect:
At the start of execution files (including npm), there commonly is a line called a “shebang”. It directs the system to the interpreter for the instructions in the text file. A typical node shebang line could be
#!/usr/bin/env node
. If it’s not written correcty, it may be directing the system to an incorrect interpreter.
To fix this second issue:
2.a Inspect and edit the shebang:
Find and open the script causing trouble in a text editor. Look at the first line-the shebang. Accommodate changes required for it to run in your environment.
2.b Correct the npm configuration:
You might have an incorrect setup in npm that’s pointing the node interpreter wrongly. Run
npm config get prefix
. If your global modules are installed somewhere other than your expected home directory, you might need to change it with
npm config set prefix /usr/local
.
Here read more on WSL troubleshoot. Remember, it’s perfectly reasonable that certain paths differ between systems; addressing these differences will be key in resolving your error.
That’s the skinny on the error and potential fixes in relation to WSL and npm. By reviewing these idea, you should be able to resolve the
/usr/bin/env: bash: No such file or directory
error after installing npm on WSL Ubuntu 20.04.Let’s analyze and debug this error message when encountered after npm installation on WSL Ubuntu 20.04.
Context Understandings
This error occurs when the system is unable to locate the shell specified in the shebang (#!) present at the top of your scripts, typically via `#!/usr/bin/env bash` or `#!/bin/bash`. This means either:
– Bash is not installed (which is extremely unlikely if you’re running a Linux-based distro).
– Bash isn’t located where it’s expected (this might be happening since WSL operates slightly differently from typical Linux systems).
Analyzing The Problem
Before adopting any resolution method, let’s identify the problem’s root cause. You can run the following command to see if bash is available:
whereis bash
The terminal will output the `/bin/bash` file path if bash is installed and its location is configured into PATH correctly. If it cannot find bash, there may be an issue with your system configuration.
Also, check for improper shebang declaration in any specific script that may throw the error.
Possible Solutions
1. Fix Shebang: Edit the first line of the offending script to reflect the accurate location of your Bash interpreter.
#! /bin/bash
2. Path Variable: Ensure your system’s Path Variable contains /usr/bin and /usr/local/bin paths. To print the PATH environment variable, use the following command
echo $PATH
3. Symbolic link: This error is frequently observed in environments using Windows Subsystem For Linux (WSL). In some cases, creating a symbolic link can rectify the issue.
ln -s /bin/bash /usr/bin/bash
4. Reinstall bash: If none of the above attempts works, try reinstalling Bash. Although it would be quite rare for Bash to be missing in your Linux Distro.
sudo apt-get install --reinstall bash
Remember to review these solutions one by one, considering the nature of your unique scenarios. It may be beneficial to understand more about the Shebang mechanism[^1^] and how the Linux system manages its PATH variables[^2^].
With the help of these outlined steps, it should be easier to diagnose and resolve the ‘/usr/bin/env: bash: No such file or directory’ message. Should the problem persist, it could be a deeper, far less common issue requiring additional debugging efforts.
[^1^]: Using the Bash Special Parameters
[^2^]: How to Set Your PATH on Linux
The
/usr/bin/env
file path is a critical part of Linux environments, including WSL Ubuntu 20.04 setups. It’s responsible for setting and managing environment variables — specific dynamic values that can affect the behavior of different applications on your system. However, when you encounter /usr/bin/env: bash: no such file or directory after installing npm, it could indicate a complication with your Linux structure.
Before diving into the solution, let us understand what exactly is happening here:
-
Your system is unable to find ‘bash’ in your
$PATH
. The
/usr/bin/env bash
command is used as a shebang (#!) line in scripts to execute them using the Bash shell. Now, while every Linux distribution usually comes with a default bash interpreter installed by default, it may happen that it isn’t present explicitly in your PATH.
-
The problem might stem from wrongly set environment variables, — more specifically the PATH variable, which is a fundamental variable in Linux/Unix systems that contains a colon-separated list of paths. When you instruct the system to execute a command, it looks through these directories in the order they are listed.
-
Another scenario might be an issue with npm itself. After installing npm on WSL Ubuntu 20.04, it uses the env utility to execute globally installed binaries/scripts with the shebang (#!/usr/bin/env node). But if there’s a mishap with the npm installation or if npm global installations are not properly included in the PATH, you might receive error messages.
The following steps outline the possible method to troubleshoot this problem:
$ echo $PATH
This will print out the value of your PATH. Check and ensure that it includes
/bin
, which is where bash is usually located.
If ‘/bin’ is missing from your PATH, you’ll need to add it. This can typically be accomplished by modifying the ‘.bashrc’ or ‘.bash_profile’ file (based on your setup), which is in your home directory:
export PATH=/bin:$PATH
Do not forget to restart your shell so that the changes take effect.
An alternate method can also be enforcing the direct execution path inside npm scripts instead of utilizing the env utility:
#!/usr/bin/node
Instead of
#!/usr/bin/env node
The last resort can be reinstalling npm or the bash shell if the issue persists.
This should hopefully rectify the
/usr/bin/env: bash: no such file or directory
error. Remember to always validate your environment variables and the integrity of your npm installation!
If you want to dig deeper into Environment Variables and learn how to manipulate them, check these references:
How To Set Up Environment Variables on Linux |
Understanding Environment Variables |
How to set npm path in the command line |
The error message
/usr/bin/env: 'bash': No such file or directory
usually surfaces when a software, in this case, Npm on WSL Ubuntu 20.04, cannot find the Bash shell program in your system. Bash is an acronym for Bourne Again Shell and serves as a command language interpreter for various operating systems, particularly those in the Unix familysource.
This insightful primer necessitates a deeper analysis of:
– The correlation between Bash Shell and /usr/bin/env
– Why there could be a missing Bash Shell in /usr/bin/env error
– How to resolve the missing Bash Shell error after installing npm on WSL Ubuntu 20.04
## The Connection Between Bash Shell and /usr/bin/env
/usr/bin/env
is a common inclusion in the shebang line (the first line of a script in Unix-like operating systems) that helps to locate the program to interpret the script, which in typical scenario is the Bash Shell (
/bin/bash
)source.
By including
/usr/bin/env bash
, you provide flexibility by not hardcoding the path to the bash interpreter into the script. The essentiality of this innocent line lies in its portability across different systems where bash is not always in the same location.
For instance, assume you have a bash script with a starting shebang line as follows:
#!/usr/bin/env bash echo "Hello, World!"
When you execute your script, the kernel will interpret the shebang line, parse the rest of the script through /usr/bin/env which checks in PATH environment variable for the first instance of bash, and then launch it.
If Bash can’t be located, you get the error we’re discussing about.
## Why There Could Be a Missing Bash Shell in /usr/bin/env Error
There are several reasons why this error might come up.
– Wrong Path: You may have installed Bash, but its path isn’t included in the list of directories stored in the PATH environmental variable the env function traverses.
– Bash Not Installed: Maybe unlikely for most users since most Unix based installations come with bash pre-installed, but still not impossible.
– Broken Symlink: The Bash executable may be located correctly in some other directory say /opt/local/bin/bash, but the symlink pointing to it in the /usr/bin directory may be broken causing
/usr/bin/env
to fail locatating bash.
## Resolving the Missing Bash Shell Error After Installing npm on WSL Ubuntu 20.04
Here are steps to possible solutions:
– Verify Installation: Confirm that bash is installed using
which
command;
$ which bash
. If the shell interprets back the path to bash, it is installed and may have a wrong path issue.
– Correcting the Path: If bash was installed in a non-standard directory, you should include that directory in PATH.
$ export PATH=$PATH:/path/to/bash
Remember to replace /path/to/bash with the actual path to the bash binary executable.
– Reinstalling Bash: If Bash isn’t installed, install it via the package manager for your specific OS, for Ubuntu, run:
$ sudo apt-get install bash
## Summary of Solutions
Solutions | Commands |
---|---|
Verify Installation |
$ which bash |
Correct the Path |
$ export PATH=$PATH:/path/to/bash |
Reinstall Bash |
$ sudo apt-get install bash |
Essentially, to succeed in setting up Npm on WSL Ubuntu 20.04, understanding the relationship between
/usr/bin/env
and the Bash shell becomes crucial. It also emphasizes the necessity of ensuring your system paths are correctly configured, especially where multiple versions of the same software – in this case, Bash – exist. Following these best practices will ease working with scripts within Unix-like systems.
Once you install npm on WSL Ubuntu, it’s not uncommon to encounter execution issues due to a variety of reasons. If you’re bumping into an error such as “/usr/bin/env: ‘bash’: No such file or directory” after installing npm on WSL Ubuntu 20.04, there are several causes and remedies for scripts failing to execute.
## Incorrect Shebang Path
Many bash scripts start with a shebang (#!) at their top defining the script’s interpreted path. It might be something like `#!/usr/bin/bash` or `#!/usr/local/bash`. The interpreter directive should point to where the bash program is located in your system. Now if the path to the bash interpreter is incorrect or if bash is not installed at that path, the error message “/usr/bin/env: ‘bash’: No such File or Directory” occurs.
To solve this issue, inspect the first line of the problematic scripts. Is it `#!/usr/bin/bash` or `#!/usr/local/bash`? Change it to #!/usr/bin/env bash as it is portable across different systems since it uses whatever bash executable appears first in the running user’s $PATH.
#!/usr/bin/env bash echo "Hello World"
## Misconfigured Environment Paths
Another cause can be misconfigured environment paths. Ensure that bash is installed properly and the PATH environment variable includes its correct location. You can print the PATH variable using echo:
echo $PATH
The output should include /usr/bin which usually contains the bash binary. If it doesn’t, modify your shell profile to include these directories and restart your terminal window or source the shell profile:
export PATH=$PATH:/usr/bin
Then, check whether Bash is installed there with ls command:
ls -l /usr/bin/bash
If Bash is not installed at all, use apt to install it:
sudo apt-get install bash
Check once again if any errors have been resolved.
## Default Shell Set Incorrectly
You must ensure your default shell is set to Bash in case it has been changed to an unrecognized format. Use the chsh command which changes the login shell for the life of a session:
chsh -s /usr/bin/bash
I trust one of these solutions will help resolve the “/usr/bin/env: ‘bash’: No such file or directory” error you’re experiencing when executing scripts post npm installation on WSL Ubuntu 20.04.
For further reading, I’d recommend the official documentation on working with the Windows Subsystem for Linux, and npm JS documentation, which provides some powerful insights into troubleshooting common issues while dealing with npm on various environments, including WSL Ubuntu 20.04.Sure, let’s tackle this issue systematically. The message ‘/usr/bin/env: ‘bash’: No such file or directory’ signifies a system configuration problem, which usually manifests after installing npm on WSL Ubuntu 20.04.
Solution Steps:
To decode this syntax error and make your Node.js packages run smoothly again:
* Ensure that your system environment is correctly set up.
* Verify the location of bash within your system.
* Correct the Shebang line in your bash scripts.
Let’s dive to each solution step:
1. Correcting System Environment:
The first thing that strikes me here is an issue with environment variables. On Ubuntu, there are many environment variables that define system properties, including the PATH environment variable that specifies all directories where executable programs are located. If it’s not properly configured, you could encounter errors like the one you’re experiencing.
You can verify your PATH environment by utilizing the echo command:
echo $PATH
It should include /usr/local/sbin, /usr/local/bin, /usr/sbin, /usr/bin, /sbin, and /bin.
2. Verifying the location of bash:
This problem may also arise if the ‘bash’ interpreter is absent from the expected directory indicated in the Shebang (‘#!’) line. Therefore, it’s essential to verify the location of bash. When running Ubuntu 20.04 on WSL, bash should be located at /bin/bash.
To verify the bash location:
which bash
If bash isn’t present in the aforementioned location, you can create a symbolic link to correct this:
sudo ln -s /bin/bash /usr/bin/bash
3. Correcting the Shebang Line:
In Unix-like operating systems, like Ubuntu, the shebang is used in scripts to indicate an interpreter for execution. Thus, ensure your bash scripts start with ‘#!/bin/bash’.
For Node.js scripts, they should have ‘#!/usr/bin/env node’, particularly when the scripts are meant to be run directly instead of being required as modules in another script.
From these corrections, you ought to resolve the error ‘/usr/bin/env: ‘bash’: No such file or directory’.
Do remember how crucial it is to maintain system stability; refrain from modifying system files or directories unless absolutely necessary. Misconfiguration could lead to other errors, turning maintenance into a game of whack-a-mole.[2]
Lastly, always update & upgrade your software to the latest stable version with apt-get:
sudo apt-get update sudo apt-get upgrade
Your system will benefit greatly from the updates and security patches provided by software vendors. This could ultimately help prevent issues causing errors like ‘/usr/bin/env: ‘bash’: No such file or directory’.
While setting up the Node Package Manager (NPM) on Windows Subsystem for Linux (WSL) Ubuntu 20.04, it’s common to encounter some hitches. One of the common issues you could face is the “/usr/bin/env: bash: no such file or directory” error message.
The problem usually arises due to a conflict between Windows and WSL over file environments. This is attributable to each running a different end-of-line character convention (*.nix systems operate with LF, while Windows functions with CRLF). So, when operating from WSL, system commands may fail because the shebang #!/usr/bin/env bash doesn’t recognize “bash\r”, which would if it was just “bash”. Even git can sometimes automatically alter line-endings upon cloning a repository.
Apps like Notepad++ can expose these hidden characters:
– \r equates to CR (Carriage Return)
– \n stands for LF (Line Feed)
Let me share how you can practically fix this issue:
Step 1: Implement dos2unix. Install it first on your ubuntu by using:
sudo apt-get install dos2unix
Step 2: Execute dos2unix. Locate your npm’s global directory, translated as
/c/Users/YOUR_USERNAME/AppData/Roaming/npm
within the WSL syntax. Run:
dos2unix $(which npm)
If dos2unix throws a permission denied message, try using `sudo`, like so:
sudo dos2unix $(which npm)
Step 3: Verify NPM’s version. If steps one and two were successful, there should be an output indicating the installed version when you run:
npm -v
Before we wrap up, take note of this additional insight:
If you still encounter problems despite following the above steps, check the path where git has been installed. OSPs like Cygwin and others sometimes install their version of git, causing conflicts. You’d need to modify your System Environment Variables’ PATH to point to the right location, for instance,
C:\Program Files\Git\bin\
.
Please reference this StackOverflow thread here about overcoming /usr/bin/env: bash issues for broader perspectives.
When diving into the process of setting up npm on WSL Ubuntu 20.04 and seeing a message such as /usr/bin/env: bash: No such file or directory, it can signify that there are certain discrepancies or issues related to the file permission settings impacting the operation of npm. Understanding these complexities is vital to narrow down potential causes, implement effective solutions, and enhance the overall functioning of npm.
File Permission Layers
There are three primary types of file permissions in Unix and Linux filesystems – owner, group, and other. These permissions dictate how the responding categories of users can interact with the files or directories:
- User (u): This category refers to the file or folder creator or owner.
- Group (g): This refers to the selection from the other users who also share the file permissions of their group.
- Other (o): Anything that isn’t classified under user or group comes under other. They have the least privileges.
Npm Operation Problem Analysis
Based on the error message, the issue likely pertains to the bash executable not being found in the path where npm defaults to find it (/usr/bin/env). When dealing with such scenarios, they often stem from the PATH environment variable not being properly set or bash not being located in the correct location due to file permission inconsistencies.
Potential Solutions
Fixing these could involve checking whether ‘bash’ is in /usr/bin or fixing the PATH environment variable.
\$ which bash
This command shows you where bash is actually located. If it’s not in ‘/usr/bin’, you might need to create a symlink:
\$ ln -s /path/to/bash /usr/bin/bash
To fix the PATH variable, edit the ~/.bashrc file and append this line to it:
export PATH=$PATH:/usr/bin
Remember, after editing ~/.bashrc, you won’t see changes take effect until you open a new terminal or run source ~/.bashrc. Check your path with echo $PATH. Bash should now be accessible for npm to run its scripts.
However, if the problem persists after following these steps, you might want to check the npm scripts for any hardcoded paths to bash that don’t exist on your system. A detailed npm script guide may aid you in addressing potential issues within your scripts.
In essence, understanding the multifaceted layers of file permissions and their impact on npm operations is a critical aspect of troubleshooting permissions-based issues and ensuring smooth npm performance after installation.
Experiencing an error “usr/bin/env: ‘bash’: No such file or directory” after the installation of Node Package Manager (Npm) on Windows Subsystem for Linux (WSL) Ubuntu 20.04 can be incredibly frustrating. However, examining the root cause is essential to attaining a feasible solution and steering our journey towards a smoother coding experience.
At its core, this error stems from a mix-up in the environment variables. Normally, WSL initializes Bash, which further points to the /usr/bin/env location. When this doesn’t occur as expected, it births challenges that could have developers scratching their heads in confusion.
Here’s a chunk of illustrative code:
export PATH=$PATH:/usr/local/bin/node
The above command is adding the path of node into system PATH. PATH is an environment variable. It basically tells the shell which directories to search when executing commands.
Shifting the problem-solving gear, adjusting the .bashrc or .bash_profile file could be the recommended move to tackle this issue [source]. These files run whenever a new interaction shell is opened, helping restore the correct environment variables.
Here’s an example of how the adjustment may look like:
cd ~ echo "export PATH=$PATH:/usr/local/bin/node" >> .bashrc
This amendment introduces the desired path of the node folder into system PATH, ultimately mitigating the roadblock.
Understanding that every user’s software setup could bear unique characteristics, consulting specific documentation [source] or reaching out to expert communities [source] may serve as an extra resource pool.
Attending to this error carefully not only ensures the successful installation and running of Npm on WSL Ubuntu 20.04 but also frames a solid foundation for sophisticated programming environments in the future. Every bug dealt with brings along strengthened troubleshooting skills and deepened practical knowledge about the intricacies of development tools. Let errors serve as stepping stones to your broader coding expertise!