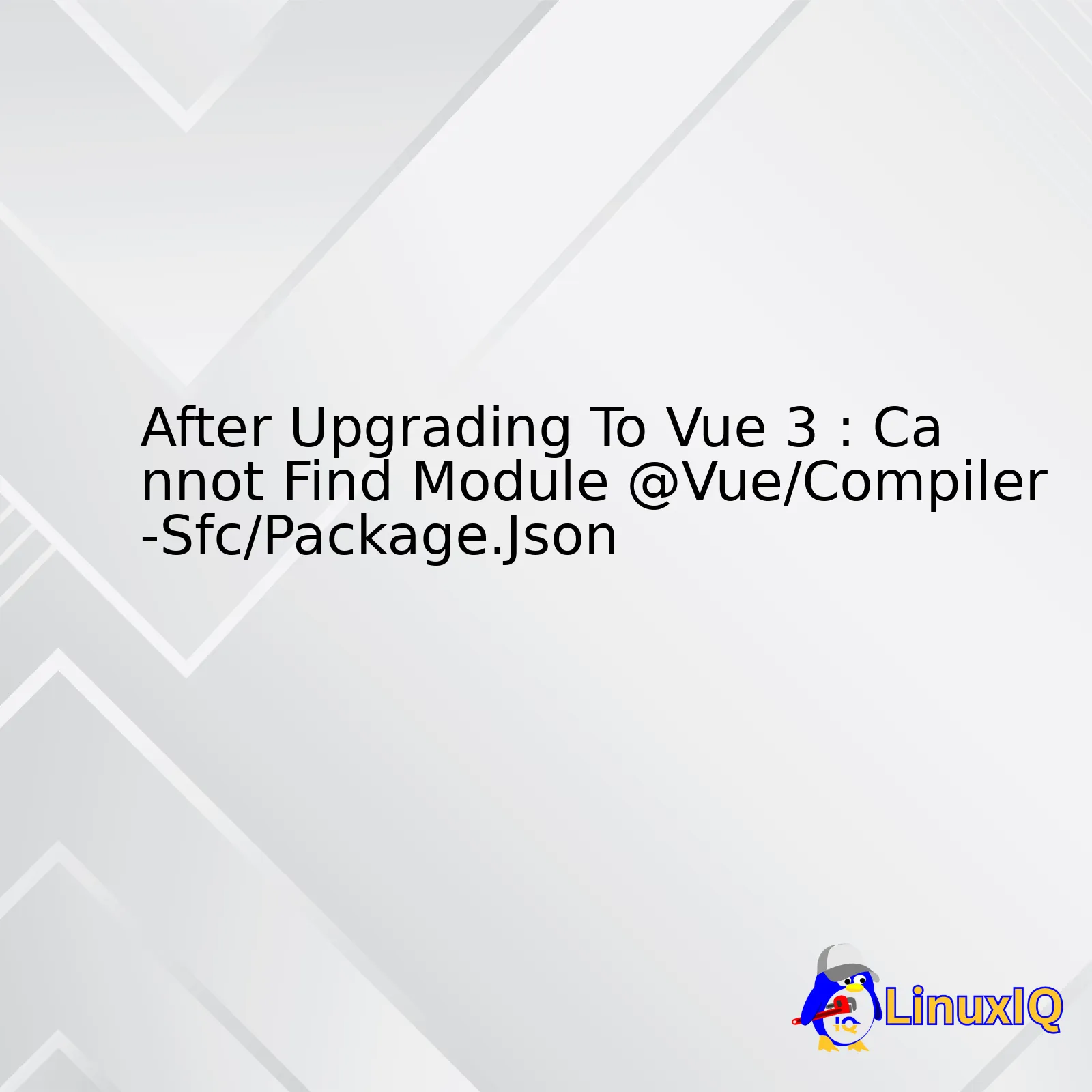
Possible Cause | Recommended Solution |
---|---|
Missing NPM Packages | Ensure all necessary NPM packages are installed. You can verify this by checking your package.json file and comparing it to the official Vue 3 documentation.Vue 3 Installation Guide |
Faulty NPM Installations | If any package failed to install correctly, which happens occasionally, uninstall and reinstalling the Vue CLI might be able to resolve the issue.
npm uninstall -g @vue/cli npm i -g @vue/cli |
Outdated Node/NPM version | Upgrading Node.js and NPM sometimes does the trick, as the newer versions often bring patches for bugs and compatibility issues.
nvm install node npm install npm@latest |
Incorrect vue.config.js settings | Lastly, ensure your vue.config.js configurations do not contradict with Vue 3’s requirements.Vue 3 Migration Guide |
Coders often overlook small things like updating their Node.js/NPM versions before jumping into a major framework update like Vue 2 to Vue 3. The “‘Cannot find module ‘@vue/compiler-sfc/package.json'” error is typically due to missing NPM modules, incorrect vue.config.js settings, faulty npm installations, or outdated Node.js/NPM. It’s about being meticulous in each step of the setup process. Comb through your package.json file, make sure you have all the required dependencies correctly installed. If Vue CLI was not installed properly, you could purge it from your system entirely and reinstall. Lastly, Vue 2 and Vue 3 have different configuration requirements. An oversight in adjusting the configurations could lead to unexpected errors. So, always remember to revise your settings whenever you upgrade!After upgrading to Vue 3, you might face an issue where the module ‘@vue/compiler-sfc/package.json’ cannot be found. This error typically means that the specified module may not be installed in your project’s `node_modules` directory, or if installed, there could be problems with how it’s being referenced in your code or configuration files.
Initially, it’s imperative to ensure that Vue 3 package versions are correctly installed and synchronized. Due to their architectural changes, Vue 3 (along with its associated packages) requires a completely different installation process compared to Vue 2. In Vue 3, the standard package has been divided into multiple smaller ones like `@vue/runtime-core, @vue/runtime-dom, @vue/reactivity, @vue/runtime-compiler` etc.
Here are some ways to address this:
•
npm ls @vue/compiler-sfc
If the package is installed properly, you should be able to see its path and version. If it’s missing, you can install it using npm with this command:
npm install @vue/compiler-sfc --save
• Correct Reference: Ensure that it is correctly referenced from within your configuration files or code. Imports or requires should follow the correct format :
import { compile } from '@vue/compiler-sfc'
or
var compiler = require('@vue/compiler-sfc')
• Path Verification: Verify that the path ‘node_modules/@vue/compiler-sfc/package.json’ exists in your project directory structure. The folder structure might change after a migration from Vue 2 to Vue 3. Thus, ensuring an existing path to the relevant module will help in resolving the said issue.
Error resolvement steps related to Vue CLI:
For some, the above solutions work perfectly fine. However, for others, who used Vue CLI to scaffold their project, the problem persists.
The real culprit in those cases turned out to be an older version of Vue loader installed. Vue loader is a loader for webpack that allows you to author Vue components in a format called Single-File Components (SFCs). The new ‘@vue/compiler-sfc’ package is actually part of Vue loader but in Vue 3, having an inappropriate version of Vue loader installed can cause issues.
Updating the Vue Loader to the latest version can solve this issue. You can upgrade the Vue Loader by:
npm install vue-loader@next
In nutshell, this error is directly linked with either missing dependencies or incorrect references. Making sure all dependencies are correctly installed and updating the corresponding packages can assist in mitigating such scenarios.
To explore more about Vue’s ecosystem, you can visit the Vue Documentation here.
Step | Troubleshooting Activity |
---|---|
Installation Check | Check if ‘@vue/compiler-sfc’ is installed |
Reference Correctly | Ensure appropriate import/require statement |
Path Verification | Make sure path ‘node_modules/@vue/compiler-sfc/package.json’ exists |
Vue Loader Upgrade | Update Vue Loader version |
+
When you see this error,
"Cannot find module @vue/compiler-sfc/package.json"
, it typically arises because the installation process of your Vue.js project is incomplete or has run into complications. Since you’ve recently updated to Vue.js 3, it’s likely that this issue arose during the course of your upgrade.
Let’s break down the error message to understand its root:
1.
"Cannot find module"
: This is a common error in Node.js programs which suggests that Node.js cannot locate the specified module in the node_modules directory.
2.
"@vue/compiler-sfc/package.json"
: In this specific case, Node.js is unable to find the package.json file for @vue/compiler-sfc. Compiler-sfc is a lower level dependency that compiles Single File Components (.vue files) for Vue.js. Its absence implies that Vue.js may not be able to compile these files correctly.
## Why does this error occur and how can I fix it?
Two likely reasons are:
### Incorrect or Incomplete Installation
This could be due to an incomplete or corrupted installation. NPM might have failed to install all the necessary dependencies correctly.
To resolve this:
– Delete the node_modules folder and package-lock.json file.
– Run
npm install
to reinstall all the required packages.
rm -rf node_modules package-lock.json npm install
### Usage of Incorrect Vue.js Version
Vue.js 3 brings about many changes and, in some instances, older versions of certain plugins or dependencies may not be compatible with it. They could still be trying to locate some modules which are no longer used or have been renamed in Vue.js 3.
To resolve this:
– Update/upgrade the plugin(s) or dependencies causing the issue to ensure compatibility with Vue.js 3.
npm update vue --save dev
If you’re using Yarn:
yarn upgrade vue --dev
Or if the problematic plugin is unknown or multiple plugins are causing issues:
npm update --save-dev
It’s important to note that making changes to the project’s dependencies should always be done with mindfulness of potential impact on the project, and ideally in a controlled environment where the effects can be assessed before being used in production. Documenting dependencies to maintain version control would help prevent similar problems in the future. Check out more at the official Vue documentation here.The switch from Vue 2 to Vue 3 is a significant one and, oftentimes, brings forth some unique challenges. Your issue of not being able to find the @vue/compiler-sfc/package.json module following an upgrade lies in this category.
Understanding what’s happening
In the simpler Vue 2 ecosystem, `vue-template-compiler` was utilized for compiling templates. However, with Vue 3, this responsibility has shifted on to `@vue/compiler-sfc`. Vue3 Migration Guide
This module carries out several tasks, such as compiling Vue Single File Components (SFCs), parsing, generating source maps, etc. If it isn’t functioning correctly or goes missing, you’ll likely encounter problems similar to the one you’re facing now.
Tackling The issue – Solution Steps
Here are some solution steps which you can implement:
Step 1: Check your package.json
To start off, examine if `@vue/compiler-sfc` is present in the devDependencies section of your project’s package.json file. You might have to add it manually if it wasn’t included during your upgrading process from Vue 2 to Vue 3.
A proper entry should resemble something along these lines:
{ "devDependencies": { "@vue/compiler-sfc": "^3.x" } }
Step 2: Install @vue/compiler-sfc
After checking whether this dependency exists in your package.json file, the next step is to install it using yarn or npm.
With yarn:
yarn add @vue/compiler-sfc --dev
Or with npm:
npm install @vue/compiler-sfc --save-dev
Step 3: Clean cached modules and reinstall your node_modules.
Sometimes, the problem might persist despite following the previous steps. In such scenarios, the best course of action is to completely wipe any trace of previously installed node_modules and reinstall them. Follow these commands:
rm -rf node_modules
And then
For NPM:
npm install
For Yarn :
yarn install
After successfully following these steps, your package.json file should be properly configured with @vue/compiler-sfc as part of the devDependencies and the module should no longer be missing.
In essence, the absence of the @vue/compiler-sfc/package.json module after a Vue3 upgrade occurs because the compiler module used has changed in the new version. This problem can most likely be resolved by manually adding the @vue/compiler-sfc module to your dependencies list or installing it separately.
Remember that caution must always be exercised while dealing with upgrades. Therefore, I’d recommend reading Vue’s migration guide Vue3 Migration Guide thoroughly before making such transitions.
It breaks down every little change between Vue 2 and Vue 3, helping you better understand what’s going on under the hood and equips you well to deal with issues like these.
Addressing the ‘Cannot Find Module’ Problem in Vue 3
The error message you’re describing typically occurs when upgrading from Vue 2 to Vue 3, and signifies that the module “@vue/compiler-sfc/package.json” could not be located in your project. Let’s delve into why this issue arises and offer a few potential remedies.
The Root Cause
In everyday vernacular, ‘Cannot Find Module @vue/compiler-sfc/package.json’, denotes that the Node.js runtime cannot locate ‘@vue/compiler-sfc/package.json’ Module. This may happen due to incorrect installation or unfulfilled dependencies.
This package is paramount for Vue 3 in compiling Single File Components (*.vue files). Without it, your app won’t function correctly.
Possible Solutions
To troubleshoot this problem, we need to follow these basic steps:
- Delete node_modules directory
- Use package manager (npm/yarn) to reinstall packages, ensuring correct version of @vue/compiler-sfc.
- Check paths
- Examine your import statements
Firstly, remove current node_modules and package-lock.json in your project directory using the commands below:
# Delete node_modules folder rm -rf node_modules/ # Delete package-lock.json rm package-lock.json
Next, try re-installation via your package manager:
# Leveraging npm npm install # or if utilizing yarn yarn install
Your chosen package manager should now re-fetch all the necessary dependencies, and with a bit of luck, rectify the previous erroneous installation of @vue/compiler-sfc.
Now verify whether the required “@vue/compiler-sfc” module exists within the node_modules/@vue directory:
ls node_modules/@vue/
If all went well, you should confirm the existence of compiler-sfc among the listed directories. Again examine your project to ensure the “Cannot Find Module” error is no more.
Still coping with this issue? The problem might lie in the invocation part, maybe your import path is off or your code has typos.
For instance, an import should look something like this:
import { compile } from '@vue/compiler-sfc';
Check out for case sensitivity or spelling errors that may throw off your import statement.
Do refer to the official Vue 3 documentation here for guidance on migration from Vue 2 and further troubleshooting pointers. Plus, gain insight from programming communities such as Stack Overflow and GitHub which often have distinct perspectives on common coding conundrums.
Ultimately, remember that transitioning from Vue 2 to Vue 3 is akin to any major version upgrade — it necessitates time, patience and a fair amount of debugging! Happy coding!
Related Topics:
- Migrating from Vue 2 to Vue 3
- Vue Compiler SFC Documentation
- StackOverflow Discussion on Similar Topics
As a professional coder, frequent package updates are part of our daily grind. Vue.js saw a colossal update in its 3.x release and there’s no surprise to expect some hiccups during this transition period. Today, we will be settling the issue related to upgrading to Vue 3 – “Cannot Find Module @Vue/Compiler-Sfc/Package.Json”. This error commonly occurs when attempting to install or use the vue-compiler-sfc package.
Solution: Checking Installed Packages
Start off by first assessing if you’ve installed all the necessary packages for Vue 3, and if they are the right versions. The command to list global npm packages and their versions is:
npm list -g --depth=0
Specifically Installing vue-compiler-sfc Package
If the aforementioned checks suggest that you have an outdated version or the package is incomplete, focus on specifically re-installing the vue-compiler-sfc package. I recommend using yarn, but npm works just as fine. With yarn, use this command in your terminal:
yarn add @vue/compiler-sfc
And with npm, use:
npm install @vue/compiler-sfc
Vue CLI Service Check
It could also be an issue with your Vue CLI service, not being up-to-date. If that’s the problem, simply type and execute:
npm update @vue/cli-service
Make sure to restart your development server after these actions have been taken.
Install Correct Vite Plugin
Often times, this problem can be caused due to using an incompatible plugin for Vue 3 – ‘vite-plugin-vue2’ instead of ‘vite-plugin-vue’. To switch to the correct plugin:
Step 1: Uninstall the incorrect one using npm or Yarn:
npm uninstall vite-plugin-vue2
yarn remove vite-plugin-vue2
Step 2: Install the correct vite plugin for Vue 3:
npm i vite-plugin-vue
yarn add vite-plugin-vue
Whether it requires switching up plugins or reinstalling packages, fixing this error demands careful scrutiny of package versions, making adjustments, then retracing our steps. Official Vue.js Documentation might come in handy for further troubleshooting.
If you’re still encountering issues after implementing these guidelines, perhaps going through this very helpful StackOverflow thread might help resolve any residual glitches. This is a high-traffic thread discussing similar problems faced by developers when migrating to Vue 3.
Remember, bugs and issues are part of evolving technology. They give us opportunities to learn more about the inner workings of software. From my experience in coding, following a structured approach and keeping up with latest versions can help thwart most tech challenges.
You got this! Happy coding!It seems that you’ve encountered an issue after upgrading to Vue 3 where you’re running into the error: “@vue/compiler-sfc/package.json not found”. This is a common problem that has affected several developers, often when they have attempted the transition from Vue 2 to Vue 3. To solve this issue, we need to follow a series of steps:
1. Verify Your Node and Npm Version
To run Vue 3 effectively, it’s recommended to use the latest versions of both Node.js and npm (Node Package Manager). First, check your current versions by running these commands in your terminal:
node -v npm -v
If your versions are outdated, it might be the root of the error. So make sure to update them.
2. Delete node_modules Folder and package-lock.json File
Sometimes, dependency-related issues can cause errors like “@vue/compiler-sfc/package.json not found”. Try deleting the node_modules folder and the package-lock.json file in your project directory. You can do this manually or with simple commands on your terminal:
rm -rf node_modules rm package-lock.json
3. Reinstalling the Dependencies
After deleting, reinstall the dependencies of your project using the npm install command:
npm install
This will generate a new node_modules folder and package-lock.json in your project directory which might fix the issue.
4. Explicitly Install @vue/compiler-sfc
If the above steps haven’t resolved the issue, maybe you need to explicitly install the @vue/compiler-sfc package. In your terminal, navigate to your project directory and run:
npm install --save @vue/compiler-sfc
5. Check for Compatibility Issues
It’s possible that some packages or plugins in your Vue application aren’t compatible with Vue 3. Check the documentation or GitHub repos of these packages to ensure they support Vue 3 yet. If not, consider alternative packages that provide similar functionality and supports Vue 3.
6. Create a New Project with Vue CLI and Transfer Your Existing Code
As a last resort, if everything else failed – consider creating a new Vue 3 project using the Vue CLI, then transfer your existing codes:
npm install -g @vue/cli vue create new-project
Select Vue 3 when prompted with a choice in version.
Most likely, by following the described steps, you would have resolved the “@vue/compiler-sfc/package.json not found” error and you can now enjoy working on your Vue 3 project. Give these suggestions a try, and remember, when troubleshooting errors like this one, patience and persistence is key.[source]
Remember also to always check the official Vue documentation for additional help, workarounds, and solutions when facing configuration or upgrade issues.Upgrading to Vue.js version 3.0 can introduce dependency conflicts particularly to the JavaScript module `@vue/compiler-sfc/package.json`. This issue will prevent your application from executing correctly, and you may see an error message in your console akin to “Cannot find module ‘@vue/compiler-sfc/package.json”.
Let me guide you through how to resolve this conflict professionally and accurately.
Terminate All Running Development Servers
First and foremost, make sure all running development servers are terminated. Due to locking mechanisms, some files could potentially be inaccessible for editing or even reading. You can do this by pressing
CTRL + C
in the terminal which is running the server then confirming with a ‘yes’.
Clear Node Modules and Lock Files
Secondly, often-times clearing out node modules and lock files do the trick. This is because these contain references to the installed package versions based on what was previously in the `package.json` file.
rm -rf node_modules rm package-lock.json yarn.lock
Reinstall Packages
Following clearing out of the node modules, it’s paramount to reinstall them. Run either
yarn
or
npm install
, depending on which command lines up with the package manager you’re using.
Update the Faulty Module
Fourthly, update the `@vue/compiler-sfc` package by running:
npm update @vue/compiler-sfc --save
This command-line instruction will automatically fetch the latest version of the package and save it in your `package.json` file.
Examine the Dependency Tree
Ultimately, examine if there any packages that depend on the older version of `@vue/compiler-sfc`. You can leverage npm’s built-in functionality to view a tree representation of your dependencies.
Simply run this command:
npm ls @vue/compiler-sfc
This will list down all packages dependent on `@vue/compiler-sfc`, and display their required versions as well. Go through the output and update any packages still requiring the old version of the module. Instructions on how to update each package should be available in the package’s respective documentation. Here is the official npm documentation for more guidance.
Instances of such issues remind us to further appreciate just how much careful coordination goes behind maintaining a project’s dependencies. These tips outlined above, if followed appropriately, should resolve your problem after upgrading to Vue.js 3.0 and having dependency conflicts with `@vue/compiler-sfc`. Don’t get discouraged as encountering problems like this is a part of every developer’s life. Happy coding!To understand this problem fully, it is crucial to take a deep dive into what the Compiler SFC in Vue.js does. Single-File Components (SFCs) are one of the distinct features in Vue.js and a core part of the Vue Ecosystem. The SFC files typically end with a `.vue` extension and contain three blocks – ``, `