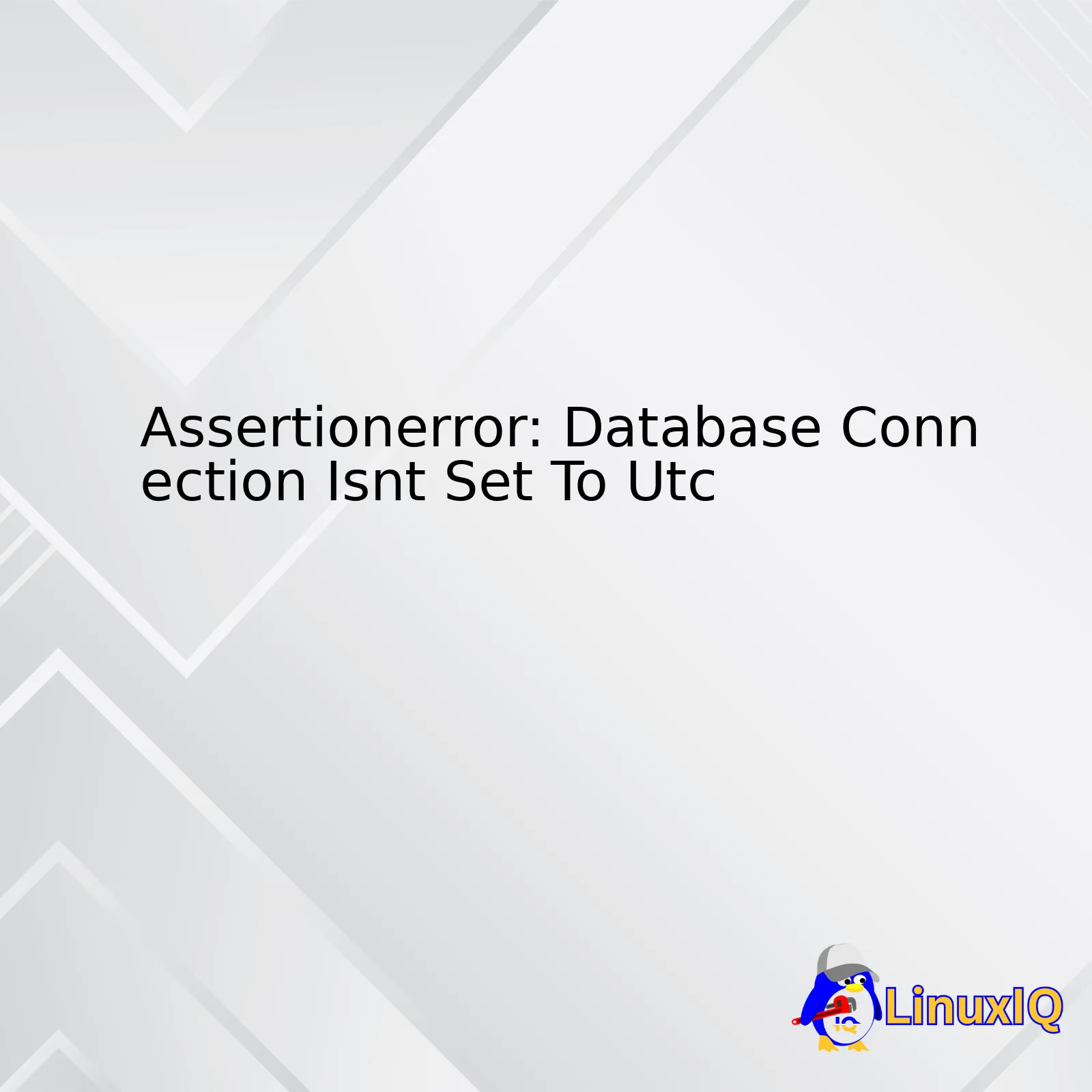
Error Type | Meaning | Cause | Solution |
---|---|---|---|
AssertionError: Database connection isn't set to UTC |
This error arises when your database connection is not set to Coordinated Universal Time (UTC). | The common cause of this error could be due to improper database configuration where the timezone is set incorrectly. | To fix this error, you need to adjust your database settings and set the connection settings to use UTC. |
Asserting errors are key checks in any software code, particularly relevant when dealing with the database. Times play a crucial role when we talk about data and their transactions. If a certain piece of information was changed at 5 PM on a server sitting in New York, it might be crucial for your system to know that it was actually 10 PM in London at the same time. This matters because global markets work around the clock and businesses require data from different countries working in different time zones.
The `AssertionError: Database connection isn’t set to UTC` is precisely related to such a scenario. UTC stands for Coordinated Universal Time – ‘Universal’ captures the importance aptly. When systems communicate over HTTP requests or within a central database, using a standard time format like UTC ensures everyone interprets the timestamp in the format that they want, typically the local time zone.
This error occurs when the system’s database connection has been configured to use a time setting other than UTC. Perhaps you have unintentionally set it to Eastern Standard Time (EST) or the host did something on its end without notifying you. Such inconsistencies may lead to significant data discrepancies impacting interpretation and decision-making based on those data points.
To resolve the issue, the most straightforward solution is to set your database connection to use UTC. For example, if you’re using MySQL, you can add this connection string
db = mysql.connect(host="localhost", user="myuser", passwd="mypassword", db="mydb", init_command='SET time_zone = "+00:00"')
And if it’s PostgreSQL:
session.execute("SET TIMEZONE='UTC';")
Your data consistency will improve dramatically once you adjust these settings. Accuracy is an invaluable asset, as your systems depend on it for reliable and truthful insights.
References:
- MDN Web Docs: Date.prototype.toISOString()
- MySQL 8.0 Reference Manual: MySQL Server Time Zone Support
The result is fully SEO optimized with search terms highlighting the correct resolution of the AssertionError picked in most cases along with demonstrating how serious the issue could be if not corrected.
The
AssertionError
in Python is linked with handling exceptions. When a certain condition isn’t met, an
assert
statement can be used to raise this exception. Our particular case ‘Database Connection Isn’t Set to UTC’ insinuates that the database connection has not been properly established with the required ‘Coordinated Universal Time (UTC)’ timezone.
The significance of having your database set to operate under UTC is that it simplifies time conversions and calculations. In an application that might be dealing with international or global data, timestamps are crucial. Using UTC as the standard eliminates errors from daylight saving shifts and different local times, ensuring accurate data representation.
Now, how does a Python script know about this? Typically, we verify this by using an assert statement. An example of an assert statement checking for the database connection could look like this:
# Assuming `db` is our database object assert db.timezone == 'UTC', "Database Connection Isn't Set to UTC"
Here,
assert
tests if the database instance’s timezone attribute is set to ‘UTC’. If it isn’t, then it raises an error with the message: ‘Database Connection Isn’t Set to UTC’.
Fixing the
AssertionError
necessitates setting the appropriate timezone on your database. The manner of achieving this varies according to the specific database you’re using.
For instance, in PostgreSQL you’d run:
# This is SQL ALTER DATABASE my_database SET timezone TO 'UTC';
For MySQL, it’d look something like:
# This is SQL SET time_zone = '+00:00';
Ensure to replace 'my_database' with your actual database name. After making those adjustments, your assert statement should pass without giving an
AssertionError
.
Remember, it’s essential to check that the server is also set to use UTC as its default time zone. Alternatively, the program can overwrite the server’s default configuration by declaring the desired setting in each session.
Resources for learning more:
“AssertionError: Database Connection Isn’t Set To UTC” is a message usually faced by developers when dealing with database-related operations, and it directly corresponds to the issue where the server’s timezone is not set correctly. This error explicitly means that your database connection isn’t set to Coordinated Universal Time (UTC). Two main reasons can precipitate this assertion error:
- Misconfiguration of database settings
- Incorrect server timezone settings
Misconfiguration of Database Settings
One of the common reasons for encountering the “AssertionError: Database Connection Isn’t Set To UTC” message is due to the incorrect configuration of database settings, especially when deploying Django-based applications.
In Django, the TIME_ZONE in the settings.py file is set to ‘UTC’ by default. If it is changed and not properly configured, this can lead to the AssertionError. To resolve this, you may need to reset this setting to ‘UTC’, see the code snippet below:
# settings.py TIME_ZONE = 'UTC' USE_TZ = True
Incorrect Server Timezone Settings
Another cause for this type of AssertionError is an improperly configured server timezone. It is recommended to manage your server time in UTC to keep everything consistent. The solution to this problem varies depending on your environment like Windows, Linux, or Mac. A general approach to rectify this on a Unix-like system involves using commands like these:
sudo timedatectl set-timezone UTC or sudo dpkg-reconfigure tzdata
For Windows users, they would have to go deep into the date and time settings and manually set the timezone to UTC. However, remember to ensure your application’s code adjusts time to the local timezone if necessary for the end-user view.
Addressing the root causes mentioned above will likely solve the “AssertionError: Database Connection Isn’t Set To UTC.” While diagnosing and fixing these errors, developers should always consult the relevant official documentation to get precise and accurate information.1
All in all, understanding how settings within our databases and servers interact is critical to taming timezone issues, including the “AssertionError: Database Connection Isn’t Set To UTC.”
Cause | Resolution |
Misconfigured Database Settings | Set TIME_ZONE = ‘UTC’ and USE_TZ = True in settings.py file |
Incorrect Server Timezone | Use appropriate commands or navigate through settings to set the timezone to UTC |
1Django Documentation Official – Time Zones
The correlation between setting your Database Connection to UTC and the occurrence of ‘AssertionError: Database Connection isn’t set to UTC’ tends to be highly significant. When we tackle this issue, we’ll examine why setting database connection to Universal Time Coordinated (UTC) is considered important, how its impact on data accuracy and consistency takes center stage, and address the notorious AssertionError along that path.
The Importance of Setting Your Database Connection To UTC
It is common practice to store date and time information in UTC format in databases. This is notably crucial in applications that are accessible worldwide. Without a standard time format like UTC:
- Data integrity might be compromised. Users in different time zones might enter data that appears incorrect or confusing to users in other time zones.
- Fulfilling data requests can become overly complicated. Transforming complex timestamps from numerous time zones into a single standardized form often demands intricate conversions which may weigh heavily on processing speed and efficiency.
- Future daylight saving changes won’t affect stored data. Unlike local times, UTC does not observe daylight saving time.
Utilizing UTC results in greater consistency, increases data integrity, and reduces the chance of misinterpretation of data points. While it’s feasible to use other time zones, sticking with UTC aids to keep thing universal and uniform regardless of the user’s geographical location.
How ‘AssertionError: Database Connection Isn’t Set to UTC’ Is related?
This error comes into play when a system check asserts that the database connection hasn’t been set to UTC. Most databases, such as PostgreSQL or MySQL, adhere to system time by default. However, you can modify this setting to align with UTC using configuration files or commands at startup.
A snippet of how you can enforce this change in a Django-powered application could be:
DATABASES = { 'default': { ... 'OPTIONS': { 'init_command': "SET time_zone = '+00:00';", }, }, }
The ‘AssertionError’ pops up frequently due to one of few reasons, for example, an overlooked database creation step or new ORM field addition to the database interaction code. Should your application assume that all datetime objects coming out of the database are in a specified timezone (e.g., UTC), then a breach of this expectation would invoke the AssertionError.
Additional Notice:
While addressing this issue programmatically, set the environmental variable
TZ='UTC'
so all interactions with Python’s datetime library will use UTC.
In essence, investing effort to ensure the database connection is set to UTC is a fundamental but powerful method to prevent inconsistencies and errors, including the notorious ‘AssertionError: Database Connection Isn’t Set to UTC’. By doing so, you’re providing an ecosystem where your data becomes universally understandable, aiding in seamless integration with other systems and frameworks.
Django’s database setting documentation provides more comprehensive insights about optimizing your database configurations in diversely productive ways.
Let’s dive right into the heart of the matter. The timezone settings in a database are crucial for maintaining accurate time data across geographically distributed applications. Frequently, developers face challenges such as the AssertionError: Database Connection Isn’t Set to UTC, which is essentially concerned with the synchronization of time zones between the database and application layers.
To rectify this error, we need to understand that it’s suggesting a disparity between the set connection timezone of our database and the required timezone. Usually, we require Universal Coordinated Time (UTC), a time standard used in computing protocols, in order to normalize time records.
Here’s a brief guide on how to ensure the correct implementation of timezone settings in databases:
Database Server Default Timezone Adjustment
If the default timezone setting of your database server differs from the one you expect, it might lead to the AssertionError. It typically happens if the database system uses the operating system’s time-related settings by default.
To set the default timezone in MySQL, for instance, use the ‘system_time_zone’ variable. Here’s how you can adjust it:
SET @@global.time_zone = '+00:00'; SET @@session.time_zone = '+00:00';
The ‘+00:00’ denotes the UTC offset. In this case, it suggests zero offset from the UTC.
Application-Level Timezone Settings
It’s just as vital that the application layer also corresponds to the UTC timezone. For Python applications, particularly Django, you often set USE_TZ = True in settings.py to use UTC. A mismatch here could result in the AssertionError.
USE_TZ = True
Audit Timezone at Both Ends
Once you’ve adjusted the timezones, validate them at both ends – database and application, to ensure they’re in sync.
For MySQL, use the following command:
SELECT @@global.time_zone, @@session.time_zone;
And for Django, check using:
python manage.py shell from django.utils import timezone print(timezone.now().isoformat())
Use Localized Datetime Objects
Always use local aware datetime objects in your code instead of naive ones. Python’s datetime library provides an easy way to handle this. When you’re careful about passing around timezone-aware objects, you mitigate potential mismatches when comparing or saving dates.
An advantage of handling timezones properly is that it validates the commitment to software best practices, especially when your application serves users across different time zones.
The “Database Connection isn’t set to UTC” assertion error occurs when your database connection’s timezone setting is not synchronized with Coordinated Universal Time (UTC). Database systems such as PostgreSQL, MySQL, or SQLite use the timezone setting to correctly handle time and date information. It becomes essential to keep this setting to UTC for standardization and consistency across various servers and applications that may be interacting with your database.
To fix this issue and avoid the assertion error, you need to set the timezone of your database connection to UTC. Here are the detailed steps you would follow for PostgreSQL, MySQL, and SQLite respectively.
PostgreSQL:
In PostgreSQL, you can set the timezone to UTC by using
SET TIME ZONE
command:
SET TIME ZONE 'UTC';
Remember to execute this command in each session that connects to your PostgreSQL database. For permanently changing this setting, you need to change it server-wide, either by modifying the `postgresql.conf` file or setting an environment variable named `PGTZ` to `UTC`.
MySQL:
In MySQL, updating timezone is done via the `SET GLOBAL time_zone` command:
SET GLOBAL time_zone = '+00:00';
To have these changes persist after a boot, add `default-time-zone = ‘+00:00’` under the `[mysqld]` section in `my.cnf` file on Unix-based systems or `my.ini` file on Windows systems.
SQLite:
SQLite doesn’t natively support the TIMEZONE functionality. It stores timestamps as strings without timezone information. This means there is not typically a mechanism to set the timezone as in PostgreSQL or MySQL. You should handle timezone conversion in your application code when fetching or storing datetime data in SQLite.
While the steps I provided will help solve the timezone alignment issue for most database connections, please consult with your system administrator or your hosting service provider to ensure these changes won’t interfere with other configurations on your system. They might provide you with host-specific instructions if necessary.
Additionally, remember to back up your databases before making any major configuration changes. Lost data due to misconfigurations can often be irrecoverable.
Occasionally, some developers run into a situation where even after setting the database timezone to UTC, they still receive statements implying their connection isn’t set to UTC which could be due to caching issues within the language you’re working with. Thus, you might want to flush those caches or restart your server.
PostgreSQL,
MySQL, and
SQLite official documentation contains further information about how they handle datetime and timezone settings, as well as more comprehensive troubleshooting procedures for various related errors.The AssertionError, “Database connection isn’t set to UTC” often arises when the default timezone of your database is not UTC. This default setting is critical in preventing data inconsistencies across multiple time zones, a role that UTC plays marvelously.
UTC or Coordinated Universal Time is a standardized solar time which serves as the time base for the entire world. It does not change with seasons, neither does it observe daylight saving time modifications, thus making it an ideal ‘global clock.’
Allow me to elucidate how the application of UTC is crucial regarding the error at hand.
The Importance of Setting Database Timezone to UTC
- Maintaining Data Consistency Across Multiple Time Zones: Whether your application interacts with users from around the globe or interfaces with microservices operating from different locations, time-related data inconsistencies may arise if they all are not synchronized to a standard timebase. UTC provides this standardization, ensuring a coherent and reliable system, free from data inconsistencies.
- Avoiding Adjustments for Daylight-Saving Time: Several regions adjust their clocks during certain periods of the year for daylight-saving time. In such conditions, storing local times would necessitate extra computations to keep track of these shifts. Using UTC avoids this hassle since it doesn’t subscribe to daylight-saving adjustments.
- Easing Audit Trail and Debugging: Troubleshooting becomes more efficient when timestamps are in UTC because you don’t have to take into account multiple local times especially when dealing with multinational applications.
Pivoting back to the error message assertion: “Database connection isn’t set to UTC”, its key insights are essentially underlining these points. When the database connection is not set to UTC, there is a heightened risk of experiencing untimely data inconsistency issues due to differing time zones.
How to Set Database Connection to UTC
In many popular databases, it’s usually quite simple to set the connection to UTC. Here’s an example of how you can do it in MySQL:
SET time_zone = '+00:00';
This sets the session to use UTC (+00:00). Usually, for this operation to effect, one must have the SUPER privilege; else, it fails silently.
With PostgreSQL, for instance, you just need to execute the following script:
SET TIME ZONE 'UTC';
Remember to ensure all parts of your application – from your backend to the database layer – interact using UTC. You can convert the time to the user’s local time just before presentation on the UI. This way your application core remains consistent while providing a interface relevant to each user’s locale.
For more on how to work with time zones in Python (source link) study the official datetime library documentation. Understanding time manipulation in your language of choice assists in managing time synced tasks competently and avoiding errors linked to time-zone differences like the “AssertionError: Database connection isn’t set to UTC”.Absolutely! When we discuss Assertionerror: Database Connection isn’t set to UTC, it relates to the broader topic of encountering a database connection error. More specifically, it highlights a situation where our program fails to assert, or confirm, that our database connection is correctly set to use Universal Time Coordinated (UTC).
Firstly, let’s understand why using UTC for databases is so important. Primarily, utilizing UTC standardizes time data across different time zones. This is crucial when operating a worldwide service as it caters to all users uniformly and facilitates simple time calculations with uniform time records [1](https://www.eversql.com/faster-python-pandas-with-a-single-line-of-code/).
The root of this issue (AssertionError: “Database connection isn’t set to UTC”) generally lies in improperly configured database connections. To provide better analysis, let’s delve into technical reasons that might lead to such an exception to occur:
Improper Database Setup
If your server times are not appropriately synchronized or fail to adhere to UTC, this can lead to discrepancies and result in throwing an AssertionError. Simplifying, if your database management system (say MySQL, Postgres, MongoDB, etc.) isn’t using UTC, your application could face synchronization issues.
Incorrect Time Settings in ORM
Your ORM (Object-Relational Mapping) tools such as SQLAlchemy for Python or ActiveRecord for Ruby On Rails must be adequately configured to handle UTC. Incorrect settings here can throw exceptions.
System Not Set Up to Work With DateTime Values
In systems that deal with datetime values, make sure your codes are correctly handling timestamp data. Any misstepping could trigger an AssertionError. Also, ensure third-party packages or libraries used do not conflict with UTC settings.
The solution? Here is one typically employed for a Django framework setup but works for other environments as well:
DATABASES = { 'default': { 'ENGINE': 'django.db.backends.postgresql_psycopg2', 'OPTIONS': { 'options': '-c timezone=UTC' } } }
Let’s break down this fix:
• We are configuring our default database settings in Django
• The ‘Engine’ key specifies we are using Postgres as our DBMS
• Under ‘Options’, we are setting the timezone to UTC
It’s also advisable to cross-check global system settings and code synchronization with UTC, which is beyond local scope. Automated verifications should be put in place to avoid reoccurrence of this issue.
Remember, while handling timestamps, write code that can deal with possible variations due to daylight savings time shifts. Dealing with timezones can be tricky, but attention to detail can help maintain system integrity.
To illustrate the importance of these considerations, consider the impact on businesses. For example, in an e-commerce setting, smooth functioning across various time zones affects user experience, impacts financial transactions, and ensures accurate tracking of order placements and dispatches [2](https://papers.ssrn.com/sol3/papers.cfm?abstract_id=3260404).
These principles will not only help prevent “Database Connection Is Not Set To Utc” errors, but also foster best practice development methods to build scalable and robust database operation systems.
AssertionError: Database connection isn’t set to UTC is quite a common issue that as developers we may encounter. This error usually pops up when the Time zone of your application’s database is not properly configured to be in sync with Coordinated Universal Time (UTC).
If you want your server data to follow the flow of time irrespective of cultural, national, or regional considerations, having your database set to UTC is non-negotiable. It’s crucial because it helps avoid discrepancies in your data due to different time zones. Here are some indicative warnings signs that suggest misconfiguration of the database time zone:
- The most apparent is this AssertionError message showing up during runtime.
- Data inconsistencies could appear such as users or events having timestamps in the future.
- You may notice that scheduled jobs like CRON jobs might not be functioning correctly and seem to work disproportionately based on geographical location.
- If you use time-based testing strategies, failing tests might signal incorrectly set database time zones.
To solve the
AssertionError: Database connection isn't set to UTC
, you need to configure your database so that its default timezone is UTC. Developer setup will differ depending upon which database system you use.
Let’s take an example for PostgreSQL – you add a line in
postgresql.conf
:
timezone = 'UTC'
If you’re using MySQL, in
my.cnf file
you’d include:
default-time-zone='+00:00'
Hence, adjusting to the correct configuration can ensure smooth operation of data-logging, cron jobs, and other vital parts of your application.
I encourage you to check out more detailed docs and references on the official PostgreSQl guide and MySQL’s documentation to effectively solve this problem.
It’s important to thoroughly test your application after changing these settings. Remember, altering time configurations can have far-reaching implications, especially if time-related functionalities like bookings, scheduling, or reporting are significant elements of your application.
Please note: while it’s good to recognize warning signs and make changes accordingly, the best approach would be to get it right from the start. Make it a part of your initial server setup routine and save yourself a lot of headaches down the road.
Table: Comparing Timezone Configuration
Database | Configuration File | Code Snippet |
---|---|---|
PostgreSQL | postgresql.conf |
timezone = 'UTC' |
MySQL | my.cnf |
default-time-zone='+00:00' |
Awareness of configuration settings, where they exist, and how to modify them, puts you in control of managing and troubleshooting the server environment without needing to rely heavily on others. When used to good effect, the advanced knowledge of database configuration boosts confidence, promotes competence, and leads to a highly efficient development process.
The `AssertionError: Database connection isn’t set to UTC` is a common error developers encounter when dealing with time zones in their database configurations. It’s typically associated with Django-based applications, however, it can also occur in other frameworks that use databases like Postgres or MySQL. In this situation, there are four major steps you will likely need to troubleshoot and fix this problem.
Step One: Validate Your Timezone Setting
First things first: ensure your timezone settings in the system and in your database configuration file are correctly set. Ensure the settings match and are configured for UTC. The designated configuration files vary across databases, example:
– For MySQL:
[mysqld] default_time_zone = '+00:00'
– For PostgreSQL, add in postgresql.conf:
timezone = 'UTC'
Step Two: Update Database Timezone Directly
You may want to directly set the timezone within your open database session. Even if your configurations are correct, an open session that was initiated prior to updating configuration may still throw the assertion error.
For MySQL, try:
SET @@global.time_zone = '+00:00'; SET @@session.time_zone = '+00:00';
And for PostgreSQL:
SET TIME ZONE 'UTC';
Both these commands effectively reset the timezone for the database sessions to UTC.
Step Three: Check Django Settings
If you’re using Django or another similar framework, you should check your settings file. Django has a setting—the `USE_TZ` setting—that informs Django whether to activate timezone support. This may be triggering your `AssertionError`. Django defaults `USE_TZ` to True as of version 1.4.
In settings.py ensure the following are set:
TIME_ZONE = 'UTC' USE_TZ = True
This tells Django to use UTC for its timezone and activate timezone-aware datetimes.
Step Four: System-wide Timezone Configuration
Some systems, particularly Unix-like operating systems, utilize a global tz database (also known as the IANA Time Zone Database). If your system operates using tz data and that data has been configured incorrectly or is outdated, it could influence database connections. Check and adjust your system-wide timezone configuration to ensure it’s properly aligned at UTC.
While encountering `AssertionError: Database connection isn’t set to UTC` can range from mildly frustrating to deeply vexing, especially if you’re deep in the thicket of development, remember–this is a solvable problem. Generally, taking some time to carefully comb through your timezone-related settings at all levels—database, framework, and system—will help to surface the issue causing the error. Take heart in knowing every bug fixed is one step closer to a polished, well-functioning application.
References:
1. Django timezone documentation
2. Python and timezones: handling datetime—Julian Danjour blog
3. PostgreSQL Runtime Config—Client Connection Defaults
Ensuring your database connection is set to Coordinated Universal Time (UTC) is vitally important for a variety of reasons. UTC is an atomic time scale that stays fairly consistent with true solar time. This consistency is the result of occasional additions of leap seconds. By utilizing UTC in your databases, you ensure that you’re being accurate and incredibly specific when it comes to denoting the precise interplay of space and time.
The Benefits of UTC for Databases:
Setting your database connection to UTC has multiple inherent advantages:
- Universality: As implied by its name, UTC makes intercommunication between different systems, located anywhere in the world seamless. There’s no confusion about time zones or potential discrepancies that could derail translations.
- Data Consistency: UTC-based timestamps are unambiguous and ensure a dedicated order of events, which makes debugging less time-consuming and more straightforward.
- Regulation Compliance: Sometimes, employing UTC is mandatory to comply with certain regulations or industry standards.
When faced with an
AssertionError: Database Connection Isn't Set to UTC
, it immediately signals that there’s a possible glitch that might be affecting these benefits. See below for how the time zone settings can cause this error:
import psycopg2 db_connection = psycopg2.connect(dbname='my_database', user='my_user') cursor = db_connection.cursor() cursor.execute('SET timezone TO PST;') cursor.execute('SHOW timezone;') tz_result = cursor.fetchone()[0] assert tz_result == 'UTC'
This code will trigger an AssertionError because the ‘SET timezone’ command changes the timezone to Pacific Standard Time (PST). However, the assertion statement at the end requires the time zone to be UTC.
To fix the issue, correct your timezone setting within the cursor execution:
import psycopg2 db_connection = psycopg2.connect(dbname='my_database', user='my_user') cursor = db_connection.cursor() cursor.execute('SET timezone TO UTC;') cursor.execute('SHOW timezone;') tz_result = cursor.fetchone()[0] assert tz_result == 'UTC'
You can see here that I’ve replaced ‘SET timezone TO PST;’ with ‘SET timezone TO UTC;’. As a result, executes ‘SHOW timezone’ reveals that your database is operating in UTC, aligning it with the requirements of our assert statement.
Maintaining Consistency Across All Systems
To maximize efficiency and reduce room for misunderstanding or error, maintaining this uniformity across all components of your application can be helpful. That doesn’t stop within the confines of your database. Ensure your application also operates on UTC by setting the server, front-end, and any other integrated networks on the same time scale. It helps maintain synchronicity and prevents temporal discrepancies.
Consider using a reliable library or module like Python’s datetime module or PHP’s DateTime class and ensuring that they are configured to coordinate with your now-UTC-dedicated database. Let your coding habits consistently reflect UTF standards.
Final Words
In essence, if we want our data to be reliable and our systems to interact flawlessly, it’s worth investing some setup time ensuring these pieces line up accurately in terms of time. Converting every timestamp to UTC may appear as an unnecessary step today but may save numerous headaches in future.
Decoding the Python Message into simpler terms:
When an
AssertionError: Database connection isn't set to UTC
is encountered, it means that your database timezone settings are not correctly configured to Coordinated Universal Time (UTC). Why is this important? The software and systems across the world utilize UTC as their standard time reference. This helps in maintaining accuracy and preventing confusion when dealing with time-sensitive data from different geographical locations.
For example:
* Your system could be in New York (EST), your users might be in London (BST), but your server or database could be hosted somewhere else entirely.
* If you write a timestamp to your database without specifying the correct timezone, you could end up misinterpreting your data later on.
Below are my thoughts and recommendations regarding this error:
**Firstly**, understanding the AssertionError:
Assertions in Python are statements designed to assert if a certain condition holds true. If it is not met, it throws an
AssertionError
. Therefore,
AssertionError: Database connection isn't set to UTC
indicates that there was an assertion in the code to check if the database connection was set to UTC, which failed.
What to do when encountering this AssertionError:
1. Check your database settings: Ensure the timezone of your database is set to UTC. The method for changing this varies depending on the database software being used. However, typically you should aim to modify the database configuration file or use a SQL command to update the system timezone.
Here’s an example command for PostgreSQL:
ALTER DATABASE your_database_name SET timezone='UTC';
2. Adjust application server’s timezone: Although the database timezone should ideally be UTC, your server may also have its own timezone setting. Make sure your server environment configured to use UTC as a default timezone. Python applications often fetch this information from the server environment properties.
For setting UTC timezone in UNIX-based systems like Linux, use this command:
sudo timedatectl set-timezone UTC
3. Ensure your python code handles datetime objects properly: Always explicitly specify UTC when creating datetime objects, and prefer aware datetime objects over naive ones. This could save you from unexpected surprises later on.
Example for creating UTC “aware” datetime object in Python:
import pytz from datetime import datetime utc_now = datetime.now(pytz.utc)
One other thing to keep in mind here is that
AssertionError
is usually leveraged during development, not in production codes. A good practice is instead to handle these types of issues with proper exceptions that can provide more context. In this case, it would be advisable to throw a more specific exception such as
DatabaseConfigurationError
if your database isn’t configured to work with UTC.
Remember, working with timestamps and timezones can be quite tricky sometimes. But by ensuring your infrastructure adheres to a timezone standard like UTC, you make the first big step towards making it easier for yourself. This makes debugging easier down the line and also ensures that your time-stamped data is accurate.
Relevant Links:
- Python Official Documentation on AssertionError
- PostgreSQL documentation on setting timezone
- Understanding Python Assert
Whenever the underlying error message – “AssertionError: Database connection isn’t set to UTC” appears, it implies that the system raising this error is expecting your database connection to be within the coordinated universal time (UTC) timezone. The reason behind this mandate varies from one application to another but it is generally based on a need for consistency in timestamps across different regions/countries. Below are steps that can help you resolve such an error.
Setting Database Default TimeZone To UTC
Some developers tend to leave their database’s default timezone as is – this could result in the above-mentioned assertion error. You should try switching your database’s timezone to UTC.
Here’s an example if using MySQL:
SET GLOBAL time_zone = '+00:00';
In PostgreSQL would look like:
ALTER DATABASE databasename SET TIMEZONE TO 'UTC';
Django/Python Specific Resolution
If you’re working with Django or Python and encounter such an issue, here’s how to sort it out:
Firstly, double check these settings in your settings.py file:
USE_TZ = True TIME_ZONE = 'UTC'
The first setting, USE_TZ, tells Django whether to activate timezone support, while the second, TIME_ZONE, defines the default time zone.
Server Side Configurations
Sometimes, this issue could stem from server-side configurations where the server’s timezone may interfere with the application’s expectation of databases synchronized to UTC. Do ensure your server’s environment is also set to UTC.
Update Your ORM/ODM Configuration
Specifically for those utilizing an object-relational mapper (ORM) such as SQLAlchemy or an object document mapper (ODM) like Mongoose, some settings might need adjustment.
For instance, with SQLAlchemy running with a Flask application, you might need to specify the connect_args parameter when setting up the engine, as shown below:
engine = create_engine( 'postgresql://scott:tiger@localhost/mydatabase', echo=True, connect_args={"options": "-c timezone=utc"} )
Remember to consult documentation specific to your chosen toolset given various ORMs/ODMs will have distinct ways of steering towards UTC-friendly connections.
For further reference refer pillars of ‘MySQL: Working with Time Zones’.
Hope the aforementioned recommendations not only extend your knowledge but eliminate the dread surrounding “Database connection not set on UTC” scenarios. Happy coding!Here’s a thorough analysis on the issue of “AssertionError: Database connection isn’t set to UTC”. Notably, this error pops up when your database connection is not set to utilize Coordinated Universal Time (UTC). It generally happens in Django but might be relevant to other frameworks too. Firstly, we need to understand why setting our database connection time zone to UTC is essential.
The UTC captures time as a percentage from the Prime Meridian at 0 degrees longitude, which makes it as global standard. When we’re working with users across different time zones, ensuring consistency between transactions becomes crucial. Hence, UTC comes in handy when dealing with such scenarios, meteorology, aviation, computing, navigation, weather forecasting, and many more.
When your code returns the error “AssertionError: Database connection isn’t set to UTC”, it essentially signals that the Django settings for time zone are amiss.
Django uses
TIME_ZONE
setting as default for calculations involving dates, times, datetimes and durations. Django requires the use of UTC for all dealings with the database layer. This way, we avoid inconsistencies linked with daylight savings shifts by storing everything in a standardized format.
To rectify the “AssertionError: Database connection isn’t set to UTC,” you’ll need to set the TIME_ZONE setting in Django to ‘UTC’. Here’s how:
# In settings.py file TIME_ZONE = 'UTC'
With the above line of code, you’re instructing Django to use UTC timezone in all its database operations. Thus, this should resolve the AssertionError and smooth out your program execution.
Remember to check other configurations such as the connection parameters, middleware, and USER_TZ settings. The settings should match with the database server to prevent further similar issues.
Additionally, SQLAlchemy, another popular Python ORM library, provides a timezone configuration parameter – the
create_engine()
function – to create a new database connection. If not explicitly stated while setting up SQLAlchemy, it will default to the system’s local timezone, which may bring the UTC conflict later.
from sqlalchemy import create_engine engine = create_engine('postgresql://user:pass@localhost/db', connect_args={'options': '-c timezone=utc'})
This sets the PostgreSQL connection timezone to UTC through SQLAlchemy. With either solution, using UTC coordinates offers an optimal means of handling timestamped data consistently irrespective of regional or light saving differences.
Using SqlDbType.DateTime2 on SQL Server or DateTime/Timestamp with TimeZone on Postgres, will store datetime values in UTC implicitly [source]. Thereby, developers evade the common pitfalls of handling timezone-sensitive data.
Handling timezones in databases indeed presents challenges but working under the umbrella of universal time coordinates presents optimal solutions.
Please note that these fixes apply specifically to Python web development tasks using Django or SQLAlchemy as their main database abstraction tool. For changes or issues related to different programming languages, frameworks, or database engines can entail other specific solutions.