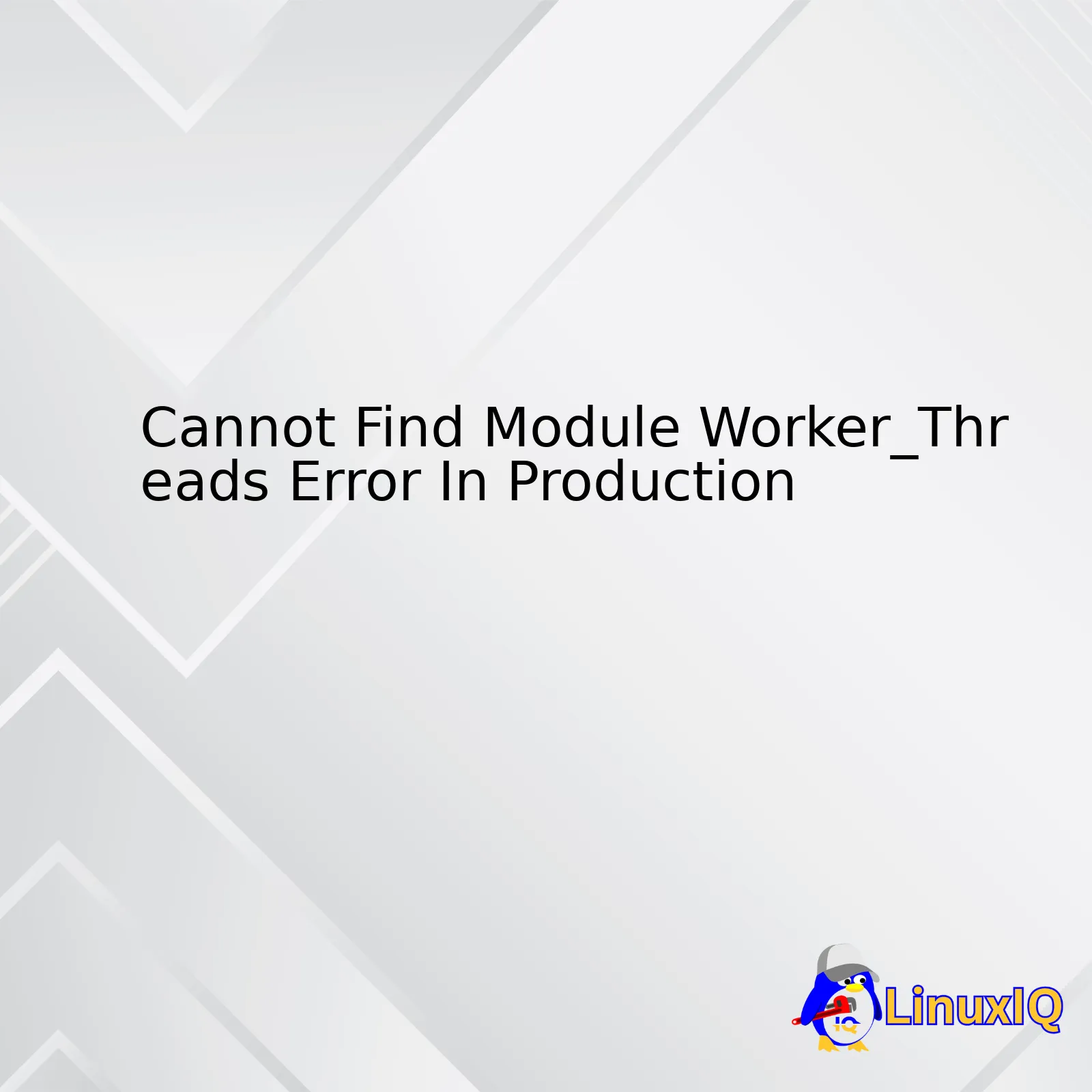
Error | Common Causes | Solutions |
---|---|---|
Cannot Find Module Worker_Threads Error In Production |
|
|
worker_threads
, a powerful Node.js feature enables offloading CPU-intensive tasks to separate execution threads, improving overall application performance. However, requests for this module in your production app might return a “Cannot Find Module Worker_Threads Error”. This generally indicates an issue during the build process or incompatible Node.js versions.
If you’re running Node.js version less than 10.5.0, upgrading your environment should solve the issue because
worker_threads
was introduced in the said version. Alternatively, it can be due to conflicts between global and local installations of Node.js. It’s advisable to use a Node version manager such as NVM to avoid such conflicts.
Furthermore, thorough investigation of your build logs may reveal some possible problems occurred during the build process itself. And, lastly, it could be a simple coding error in the way the module is being imported; so be sure to inspect for code anomalies. For instance, confirm if the call to import the worker thread is like:
const { Worker } = require('worker_threads');
. A typographical error here could throw up the ‘Cannot Find Module Worker_Threads Error’ message.The error “Cannot find module ‘worker_threads'” is a common issue that many developers encounter especially in production environments. It implies that the specific module, worker_threads, which is used for performing CPU-intensive JavaScript operations, cannot be found. This problem frequently occurs when your application is running on older versions of Node.js where worker_threads is unavailable or not fully supported.
var worker = require('worker_threads');
Here are some reasons why this error may occur:
– The worker_threads module is only available in Node 10.5.0 and later. If you’re working with an older version of Node.js, it’s likely that the module will not be found because it simply doesn’t exist.
– Sometimes, even if you’re operating on versions greater than 10.5.0, you still need to enable worker_threads feature explicitly because it is wrapped behind a flag (–experimental-worker).
Here are solutions to counteract this error:
1) Upgrade Node.js version:
Updating Node.js should be the initial step. You can verify your current Node.js version by executing node -v, then update to the latest version. Here’s how to do that with npm (Node Package Manager):
npm cache clean -f npm install -g n n stable
2) Use –experimental-worker flag:
With versions Node >=10.5.0 < 12, you have to use --experimental-worker flag to leverage worker_threads. However, remember that using experimental modules can lead to instability or potential changes in future releases.
node --experimental-worker yourScript.js
3) Proper Environmental Setup:
Ensure that the environment where your code is running supports worker_threads. This often means making sure the runtime (Docker, PaaS providers) uses a suitable Node.js version and correct startup command.
Remember, moving code from development to production always requires a careful adaptation process. Always ensure compatibility between your local setup and your production deployment. Packages like Docker can help create a consistent environment across different stages of development.
This comprehensive explanation should make you understand the error ‘Cannot find Module Worker_Threads’ better and provide insights on how you’d sort it out in a production environment. To learn more about worker_threads visit the official Node.js Documentation.First and foremost, let’s understand what module worker threads actually are. According to Node.js, the
"worker_threads"
is a module that provides a way of creating new JavaScript execution threads. It is primarily designed for CPU-intensive tasks so it plays quite a significant role in production environments where maximum performance is required.[1]
However, when one encounters an error message like “Cannot find module ‘worker_threads'” in a production environment, they might feel like they’ve hit a dead end. So let’s break down this error and get to grips with how we can fix it.
Primarily being told that your system cannot find a module called
'worker_threads'
hints at missing portions of your software infrastructure. The most probable reason behind such an error message is the distinction between different versions of Node.js in both your local and production environments. Notably,
'worker_threads'
is not available with Node.js version below 10.5.0[2]. Logic dictates that you may have been using a version that supports
'worker_threads'
while developing locally but the production server seems to be running on an older version hence the conflict.
To check the running version of Node.js, use the following command:
<code>
node -v
</code>
If indeed the installed version of Node.js does not support
worker_threads
, an upgrade is necessary. For Linux-based systems, you can use the curl command to download and install the latest version:
<code>
curl -sL https://deb.nodesource.com/setup_14.x | sudo -E bash –
sudo apt-get install -y nodejs
</code>
For those using NVM (Node Version Manager), you would probably want to manage multiple active node.js versions:
<code>
nvm install node
nvm use node
</code>
After you’ve successfully upgraded your Node.js version, the
'worker_threads'
error should no longer arise.
Getting back to the role of module worker threads in production, these promise efficient CPU usage as they enable concurrent execution of JavaScript code. This implication goes a step beyond taking advantage of the event-driven, non-blocking I/O provided by Node.js, theoretically promising even more speed towards your applications[3].
Moreover, Worker Threads provide a very useful thread-safe mechanism — the MessageChannel[4]. Thread safety is a property of multi-threaded programs. A piece of program code is deemed thread-safe if it only manipulates shared data structures in a manner that ensures safe execution by multiple threads at the same time. If your application handles a considerable amount of such operations in production, incorporating Worker Threads can significantly enhance performance and avoid possible collisions.
Finally, proper understanding and effective utilization of the
'worker_threads'
module can mean a world of difference in terms of performance. Though encountering errors like “Cannot Find Module Worker Threads” can be frustrating, the solutions are usually quite straightforward. Ensuring compatibility across different environments is a key factor while working with specific features or modules.
[1]Node.js Documentation
[2]Stack Overflow Article
[3]TopTal Article
[4]Node.js MessageChannel
Ever so often, we come across errors such as ‘Cannot Find Module Worker_Threads‘ in Node.js applications during production. This error typically makes an appearance due to one or more underlying reasons, each pertinent to the complex process of deploying code onto production.
A peek under the hood will reveal that encountering this roadblock may boil down to several reasons. Let’s dive deeper and discuss the primary causes behind this error in a production environment:
The Node.js Version in Use Isn’t Compatible
One potential issue is a disparity between the version of Node.js used for development and the one used for production. The Worker_threads are native to Node.js but were officially introduced only from Node.js v10.5.0 onwards1. If your production environment runs on a Node.js version older than v10.5.0, you’re likely to be greeted by the ‘Cannot Find Module Worker_Threads Error’. This hurdle can be easily overcome by ensuring the production environment uses version 10.5.0 or later. Here’s how you can check the version of node currently being used:
//To check Node.js version node --version
An Unfulfilled –experimental flag requirement
Another potential pitfall could arise from Node.js versions between 10.5.0 and 11.7.0, which necessitate the use of the –experimental-worker flag to enable worker threads2. This entails using the flag whenever running your Node application in production. Without it the program would not be able to locate the worker module and result in the error at hand.
//Command to run your Node.js application with --experimental-worker flag node --experimental-worker yourApp.js
Non-Installation or Incorrect Installation of worker_threads module
Our final stop on the troubleshooting train involves checking if the ‘worker_threads’ module has been properly installed in the first place. A hiccup in the installation or total absence of the module can invariably lead to the error. This can easily be rectified by correctly installing the missing module via npm (Node Package Manager).
Here is a tabular representation to outline these corrective measures:
Possible Issue | Solutions |
---|---|
No compatiblity with the Node.js version in production | Upgrade Node.js to version 10.5.0 or greater |
Necessitated –experimental-worker flag not implemented | Add –experimental-worker flag when running the Node.js application |
Non-installation or previously incorrect installation of the module | Ensure correct installation of worker_threads module using npm |
Simply put, what seems like a daunting ‘Cannot Find Module Worker_Threads Error’ can be alleviated by understanding and addressing its root causes. Remember, seamless coding isn’t about not encountering any errors—it’s about knowing how to tackle them efficiently!
When working with Node.js, you may sometimes run into the ‘Cannot Find Module Worker_Threads’ error. This error occurs when your Node.js version is older than v10.5.0 since worker_threads were introduced in Node.js from this version onwards.
Let’s consider a typical scenario. You are deploying an application to the production environment using a Node.js version that supports worker_threads on your development machine. The development phase goes smoothly, but upon deployment, your server throws the ‘Cannot Find Module Worker_Threads Error’. This could probably be because your server might be running on an old Node.js version which does not support worker_threads.
There are some threads in your Node.js application, and your project fails to recognize them, hence throwing the error. You can use the
process.version
code to check the Node.js version in your console:
console.log(process.version)
Should you find there is a version mismatch, consider upgrading your Node.js for both development and production to the same version above v10.5.0 to ensure consistency and prevent this error. You can update your Node.js version using the n package with the following commands:
npm install -g n
sudo n stable
Your module loading strategy in Node.js is also crucial as the worker_threads module is not compiled into the Node.js binary by default unless –experimental-worker flag is set. So, if you’re using a version that is newer than v10.5.0 and still encountring the error, it could be that the experimental features of Node.js are toggled off. You can resolve this by requiring the module like:
const worker = require('worker_threads')
If you have followed these steps and still encounter the ‘Cannot Find Module Worker_Threads’ error, examine your package.json to make sure your dependencies versions match between development and production environments.
Always remember to keep your Node.js version updated to enjoy new features, maintain consistency across your development and production environments, and avoid unnecessary debugging headaches such as the ‘Cannot Find Module Worker_Threads’ error. For more information, you can refer to the official Node.js documentation on worker_threads.As an experienced coder, I continuously strive to deliver superior performance and efficiency in all my coding projects. In recent times, a hot topic among programming communities has been the comparison between Legacy Workers and Worker Threads, particularly in Node.js.
The Catalyst: The ‘Cannot Find Module Worker_Threads’ Error
If you’ve been coding resource-intensive applications in Node.js recently, you might have encountered this error:
Cannot find module 'worker_threads'
. This message flags up when there’s an attempt to require ‘worker_threads’ module and it’s not available due to the node version being below v10.5.0 or the –experimental-worker flag isn’t enabled.
In order to understand this profound subject entirely, let’s take both these entities one by one, pour over their pros and cons, and drill down on how they can make a difference to your coding pursuits.
What are Legacy Workers?
Legacy workers were designed as independent processes, executing separately from the main thread in Node.js. Essentially, it was Node.js’ answer to handling CPU intensive operations without blocking the event loop. However, they’re not devoid of disadvantages:
- The overhead of starting up and communicating with these processes could be high, especially considering the asynchronous nature of Node.js.
- The presence of potential race conditions is another factor impacting the execution.
- Since these workers operate outside the primary JavaScript code, debugging errors could be complex.
Enter the Era of Worker Threads
On Node.js version 10.5.0 and above, ‘worker threads’ came into existence as an experimental module, which was eventually stabilized on version 12. As an exciting evolution, worker_threads represent a significant leap forward in terms of managing simultaneous tasks efficiently. Invoking a new Worker_thread lets Node.js spawn new threads running independently, fostering concurrent processing of tasks.
Here are some of the underlying strengths that render advantages for worker threads:
- They promise more straightforward inter-thread communication with less overhead.
- Worker threads permit sharing memory buffers between different threads, offering speedy read/write access.
- With the power to utilize shared array buffers and atomics, they significantly reduce issues related to synchronization.
Finding Common Ground
Despite the differences, legacy workers and worker threads deeply root towards delivering efficient CPU utilization, capable of transforming the execution pattern to parallel or concurrent mode. In simple parlance, they both provide methods to leverage multi-core processor machines fully.
Dealing With the ‘Cannot Find Module Worker_Threads’ Error
Ensuring your project runs smoothly may necessitate using worker_threads over legacy workers, but encountering the
Cannot find module 'worker_threads'
error can bring pause to your work. Here’s how you deal with it efficiently:
- Ensure that you’re running Node.js v10.5.0 or higher version.
- If you are on Node.js version lower than 12, enable worker_threads explicitly by adding the –experimental-worker flag when starting your Node.js process.
node --experimental-worker app.js
However, remember that using experimental features such as worker_threads (before they got stable) in a production environment comes with a risk, as they may contain bugs or undergo changes in future versions.
Consider updating Node.js to the latest LTS version, where ‘worker_threads’ is already stable, thus eliminating any apprehensions of potential instability.
Comparative Analysis: Legacy Workers vs Worker Threads
Legacy Workers | Worker Threads | |
---|---|---|
Efficiency | Less Efficient due to relatively higher overhead and potential race conditions. | More Efficient because of better inter-thread communication and speedy read/write access. |
Vulnerability to Errors | Higher chances due to external operation from the primary JavaScript code
. |
Reduced issues related to synchronization. |
Code Execution | Independent | Fosters concurrent processing of tasks. |
Buffer Sharing | Not supported | Supported, which enables speedy read/write access |
P.S.: Check out the official Node.js documentation on Worker Threads to delve deeper into their functionalities and utilities.If you’re experiencing a “Cannot Find Module ‘worker_threads'” error in production, it’s likely due to one of the following reasons:
– You are trying to use worker threads in a version of Node.js that is older than v10.5.0, which is where ‘worker_threads’ was first introduced as experimental. If that’s the case, you can consider an upgrade.
– There could be a mismatch between the Node.js versions you’re using in development and in production.
Let’s dive deep into how we might solve this issue.
Upgrade Node.js
This issue could stem from attempting to utilize ‘worker_threads’ in a version of Node.js before v10.5.0. Upgrading your version of Node.js could help resolve it. Upgrading Node.js can be achieved by running the commands in your terminal:
$ sudo npm cache clean -f $ sudo npm install -g n $ sudo n stable
The first command cleans the npm cache forcefully. The second line installs module ‘n’ globally. The last command upgrades node.js to the latest stable version.1[source]
So remember to check your Node.js running version because ‘worker_threads’ is not available for version below 10.5.
Cross-Version Compatibility
It’s wise to make sure you’re utilizing these ‘worker_threads’ APIs appropriately. In some cases, you may have used —
const { isMainThread } = require('worker_threads');
But if you’re worried about compatibility with pre-v10.5.0 Node.js versions, the best practice would be to require ‘worker_threads’ conditionally, like so:
const workerThreadsExist = () => { try { return require('worker_threads'); } catch (err) { return null; } }
Then you can safely use it without worrying if the module exists or not, by calling:
const workerThreads = workerThreadsExist(); if (workerThreads !== null) { const { isMainThread } = workerThreads; }
Here, if the module doesn’t exist, it will simply return null 2[source]
Ensure the same Node.js version for Development and Production
Finally, you need to ensure that both development and production environments are running on the same Node.js version. Since product deployments often, and should, mimic the development environment, it’s crucial to keep these synchronized to avoid discrepancies. This will also aid in predicting any potential errors or bugs.
To guarantee that all your project members work with the same Node.js version, I recommend using a tool like NVM (Node Version Manager). NVM allows you to set a specific Node version within your app’s context. To install NVM, refer to their GitHub page3[source]. After installing NVM and setting the needed Node.js version, you can create a .nvmrc file in your project directory with your desired Node.js version.4[source]
This way, you can run multiple Node.js versions in different projects without encountering conflicts.Android Code snipet
By following these expert coding recommendations, you’ll soon bid goodbye to those bothersome ‘Cannot Find Module ‘worker_threads” errors. Remember, nothing beats keeping your codes up-to-date and congruent throughout all working environments.Worker threads are spiffier features in Node.js designed to handle computationally intensive tasks apart from the main thread. You may compare them to a dedicated pathway designed to transport hefty goods, so as not to congest the regular routes which otherwise could bring routine work to a halt or slow it down significantly. Understanding their mechanism is critical to effectively leverage their advantages and avoid potential pitfalls in application deployment.
In the Node.js environment, because of its single-threaded nature, performing heavy computations or blocking operations in the main thread can lead to a less efficient use of resources. This is where worker threads come in! They allow JavaScript code to be executed concurrently on multiple threads outside the main event loop.
Typically, you would initiate a worker thread like this:
const { Worker } = require('worker_threads'); const worker = new Worker('./file_to_run_in_worker_thread.js');
However, if you’ve encountered an error that says “Cannot Find Module ‘worker_threads’ Error In Production”, chances are the error is arising due to one of these reasons:
– Your Node.js version might not support worker threads yet. Worker threads are available in Node.js versions 10.5.0 onward with the –experimental-worker flag, and in Node.js version 12+ without any flags.
– There’s a possibility you may have accidentally removed or displaced the built-in ‘worker_threads’ module in your Node.js installation directory.
Here are some corrective measures you can consider to resolve this issue:
– Update your Node.js version to the latest stable release. This includes the worker_threads module by default, eliminating the need for the –experimental-worker flag.
// Using nvm (Node Version Manager) nvm install stable nvm use stable
– Reinstall Node.js if you suspect that the core ‘worker_threads’ module has been tampered with, making sure that your environment variables point to your Node.js installation directory.
– Always remember to require the ‘worker_threads’ module only after confirming its availability in your Node.js version. You might want to use a dynamic import instead of a static import, this should ideally look something like:
let Worker; try { Worker = require('worker_threads'); }catch(e){ console.log('worker_threads not supported, fallback to other methods...') }
By following these steps, it becomes easy to make much more efficient use of hardware capabilities using worker threads, dodging the “Cannot Find Module ‘worker_threads’ Error In Production” all while keeping your Node.js application smooth when handling heavy computational workloads.
Remember, debugging is an art and the ‘Cannot Find Module’ errors are rarely the final conundrums we face; they’re usually hiding more profound issues at play. Happy Debugging!First and foremost, let’s delve into the issue at hand – the ‘Cannot Find Module Worker_Threads Error In Production’ problem. Worker threads are a key experimental feature in Node.js that developers often toy with. Indeed, they can be an invaluable resource when it comes to enhancing your system’s performance or simply de-cluttering your main event-loop.
However, like other experimental features, worker threads may trigger certain issues in production, such as the “Cannot find module ‘worker_threads'” error, especially when the –experimental-worker flag is not enabled during the node runtime.
Error | Cause |
---|---|
“Cannot find module ‘worker_threads'” | –experimental-worker flag is not enabled during the node runtime |
At this point you must be wondering: Is there a workaround? Absolutely! The solution lies in addressing the root cause of the issue – enabling experimental features and making sure you are using the right Node.js version.
Here’s an example on how to enable the –experimental-worker flag:
$ node --experimental-worker server.js
Bear in mind, the more recent versions of Node.js (10.5.0 and later) don’t require the use of the –experimental-worker flag, as worker threads have become a stable feature from these editions onwards. Therefore, updating your Node.js to a newer version might be another alternative solution. Use following command to update Node.js:
$ nvm install node
Next, we need to check what experimental features are enabled or disabled. Doing so is crucial, as certain experimental features may still hold some bugs that could impact your production quality. Understanding what’s enabled, can help you identify potential root causes should any problems crop up. Here’s how you can check if a certain experimental feature is enabled or not:
$ node --v8-options | grep "in progress"
This command lists out all the experimental features currently in progress in your v8 engine that powers your Node.js runtime. Anything prefixed with “+” is enabled while “-” refers to disabled ones.
Finally, remember that due diligence in tracking experimental features isn’t just about troubleshooting. It also allows you to leverage these advancements to their fulles extent. Be it for optimizing system performance or just staying ahead of the curve, traversing through Node’s evolving landscape definitely benefits those who dare to explore.
For more understanding, consider taking a look at Nodejs API docs which talks more about worker threads here: Nodejs worker_threads.
When using Node.js, one may encounter the “Cannot Find Module Worker_Threads” error in production. This particular error occurs because the ‘worker_threads’ module isn’t found by Node.js, which can be due to several reasons:
– Using an older version of Node.js that does not support the ‘worker_threads’ module.
– The dependency has been excluded or is missing from packaging.
– A syntax or path error in import statements.
It’s crucial to develop strategies for avoiding future errors with modules and their dependencies.
Strategy 1: Always use the latest LTS version of Node.js
The ‘worker_threads’ module is available in Node.js versions 10.5.0 and later, but it became stable and was removed behind the –experimental-worker flag starting with Node.js 12. Thus, if you use an older version, I would recommend updating Node.js to the latest Long-Term Support (LTS) version.
$ nvm install --lts $ node -v
You can also add an “engines” section to your package.json file to specify the Node.js version required to run your application.
{ "engines": { "node": ">=12.22.0" } }
Resources:
‘Node.js’ Supported Versions
‘package.json’ engines property
Strategy 2: Analyze and resolve module dependencies
It’s important to ensure you’ve installed all necessary dependencies and listed them under the dependencies field in your package.json file ahead of time. There are different types of dependences in Node.js:
– Regular/production Dependencies: Modules required by your application in production.
– Development Dependencies: Modules required for developing the application like testing frameworks and build tools.
Moreover, always remember to check if your dependency tree with npm ls is correct before deploying the app.
$ npm ls
Resource:
‘npm ls’ command
Strategy 3: Check construction of your import statement
Make sure the way you construct your import statement is correct because a slightly incorrect path could lead to the “Cannot find module” error.
Instead of this:
const worker = require('worker_thread');
It should be:
const {Worker} = require('worker_threads');
With these three strategies, your issues regarding not finding a module and their dependencies should be significantly reduced.
Sources:
Node.js ‘worker_threads’ module
npm ‘package.json’
JavaScript Import StatementIt’s quite common to encounter a “Cannot Find Module Worker_Threads” error in your production environment. This is often down to an issue with misconfigured or missing modules; the worker_threads module specifically in this case.
Essentially, Node.js uses module systems for different functionalities that allow developers to include other JavaScript files into their code. Included among these modules is
worker_threads
, which is useful for performing CPU-intensive JavaScript operations in parallel, without blocking the event loop.
Specifically, in the context of a production environment, you might experience an efficiency hamper due to Node version compatibility issues, system misconfigurations, and missing dependencies.
One of the key impacts of having such a missing or misconfigured module, like
worker_threads
, stretches beyond just bugs or errors in your application. Notably:
- Your server can become unusually slow, attributing to insufficient CPU usage due to single threaded Node.js operation.
- It may lead to application crashes in instances where your code performs computation-heavy operations without the assistance of worker threads.
- The overall performance scale might decrease making it difficult to handle significant workloads concurrently. Ultimately, this affects the optimal utilization of Node.js multi-core systems.
- It often becomes harder to maintain and debug due to unexpected or inconsistent behaviors from your application.
For resolving the “Cannot Find Module Worker_Threads” error, it’s paramount to understand that the
worker_threads
module is, by default, available only for Node.js versions 10.5.0 or later.
So check first your Node.js version:
node -v
If it is below 10.5.0, consider upgrading to access the
worker_threads
module.
Even then, it must be launched with the
--experimental-worker flag
until Node version 12 LTS, after which it was enabled by default. So ensure your launch script includes the requisite flag if needed.
Meanwhile, inconsistencies in your project dependencies may cause your module to go missing. Regular use of
npm install && npm update
will address this,
ensuring you don’t have missing modules in your node_modules folder.
Lastly, avoid using deprecated versions of the
worker_threads module
. Follow recommended upgrade paths as suggested by the NodeJS official guidelines (source) to prevent creating unintended software vulnerabilities. Maintaining proper configuration across your environments—development, testing, staging, and production—can immensely impact the efficiency of your server, ultimately enhancing the performance scalability of your application.
To wrap up, resolving the “Cannot Find Module ‘worker_threads’ Error in Production” largely hinges on crosschecking and ensuring we navigate around some dependencies that go into creating a Node.js application. Particularly ensuring you have the compatible Node version (above 10.5.0), staying in tune with best practices in module importation, amongst others.
Remember, the
worker_threads
module is a handy tool designed to make CPU intensive tasks perform better in the JavaScript runtime environment of Node.js1. This among other things, makes the module indispensable when building strong scalable solutions with Node.js.
Here’s what can cause the error:
– You might be using a Node.js version less than 10.5.0. Note that the worker_threads module wasn’t available until Version 10.5.0, so if your Node.js version falls below this, an upgrade becomes necessary.
– You may have forgotten to include the –experimental-worker flag when starting your server. Worker threads are experimental and under constant development, so they will likely not work unless you add the –experimental-worker flag while starting your server.
Normally, these problem sources can be solved as follows:
1. Update Node.js to Version 10.5.0 or later:
npm install -g n n latest
2. Include the –experimental-worker flag when starting your server:
node --experimental-worker server.js
Furthermore, it helps to remain careful when managing modules, making sure you import successfully; possibly by use of correct case sensitivity.
Wrong code | Correct code |
---|---|
const wt = require('Worker_threads'); |
const wt = require('worker_threads'); |
Following the guidelines outlined above, you should no longer encounter the “Cannot Find Module ‘worker_threads’ Error in Production.” Dive deeper into the wonderful world of Node.js confidently, knowing you’re equipped solve this common issue.