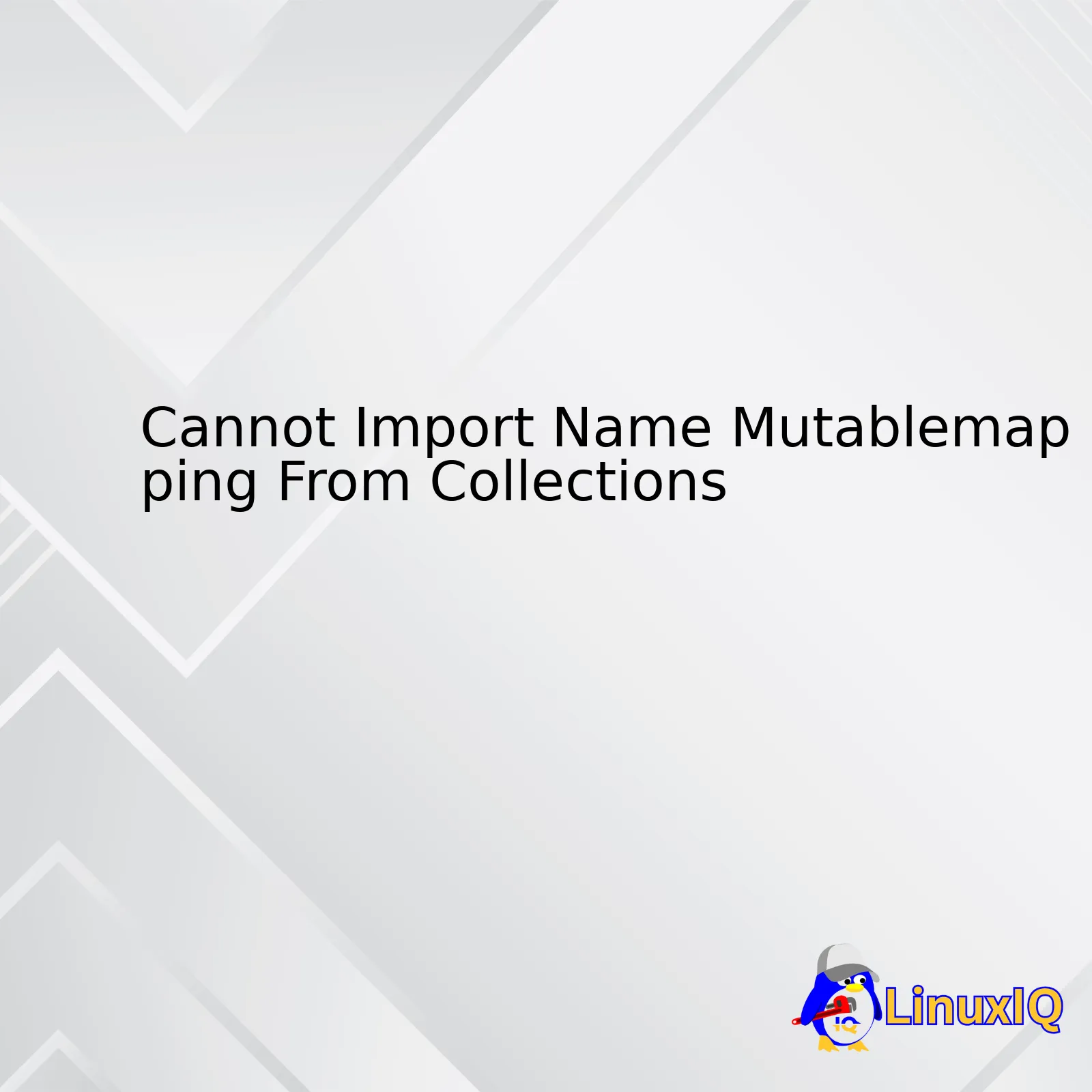
Error | Solution |
---|---|
Cannot Import Name MutableMapping from collections | Use
from collections.abc import MutableMapping |
ModuleNotFoundError: No module named ‘collections’ | Make sure your Python version is up-to-date |
Now let’s talk about this topic in some more detail.
In Python, when you encounter an error like “Cannot Import Name MutableMapping from collections”, it means your code tried to import the MutableMapping class directly from the python standard library’s collections module. It was possible in older versions of python. However, with the arrival of python3.3, these abstract base classes (ABCs), like MutableMapping, were moved to a new module named collections.abc as part of collections.
The solution involves replacing
from collections import MutableMapping
with
from collections.abc import MutableMapping
in the import line of your code. Here’s an example:
Older way:
from collections import MutableMapping
Correct way:
from collections.abc import MutableMapping
The second error – ModuleNotFoundError: No module named ‘collections’ occurs if Python itself isn’t correctly installed or exceedingly outdated. To solve this, you need to ensure Python’s most recent credible release is configured correctly on your system.
Remember that embracing updates as they come along not only provides us with the latest tools but also eliminates inconsistencies and blunders held by older versions. Upgrading your version of Python will invariably assist you in circumventing issues like the ones we’ve discussed here.
You can read more about these import changes based on Python’s official documentation here.
Understanding such import errors and rectifying them plays a crucial role in writing efficient, error-free python scripts. Keeping your Python version updated ensures that you are working with all the recent enhancements and bug fixes offered, thereby making your experience smoother and your code better performing.The error `Cannot Import Name MutableMapping from Collections` arises primarily due to a change in the Python collections module that occurred as of Python version 3.3. Before this shift, one could easily import the `MutableMapping` class directly from the `collections` module. However, with the introduction of the `collections.abc` (Abstract Base Class) module to hold the mutable mapping class and its siblings, such direct importing is no longer possible. Hence, you are encountering the mentioned error.
Previously, you might have written:
from collections import MutableMapping
Now, from Python 3.3 onwards, it should be :
from collections.abc import MutableMapping
So, why has this happened?
Python developers moved these classes to improve organization and structure in the collections module. By grouping the abstract base classes together under collections.abc, they made the architecture more understandable and maintainable.
• **Greater Simplicity** In having the primary data structures separate from their more theoretical abstract base classes, newbies to Python find the language more approachable.
• **Better Practice Encouragement** Segregating the abstract base classes also encourages better programming practices. It invites programmers to create custom, complex data structures inherited from them, which leads to more neatly organized code.
Therefore, it’s essential to understand the origin and context behind an error like ‘Cannot Import Name MutableMapping from Collections’. Being aware of changes in Python’s evolution can help you better adapt your code and avoid such errors in the future, hence allowing you to write cleaner, more effective code.
For additional resources on the topic, the official Python Documentation provides a detailed explanation of the collections.abc module. There you will find more on MutableMapping along with various other classes in this module.
Python, the powerful interpreted language, has a comprehensive collection of modules. One such module is the collections module, used abundantly for handling complex data manipulation tasks. It provides data structures and functions that enhance the already robust capabilities of Python.
The specific problem at hand deals with importing the ‘MutableMapping’ class from the ‘collections’ module in Python. For instance, one might encounter difficulties manifesting in a common ImportError that reads:
ImportError: cannot import name 'MutableMapping' from 'collections'
This error is often due to using an outdated version of Python. The MutableMapping object was moved to the collections.abc module in Python 3.3 according to official Python documentation. If your Python version is below 3.3, it’s time for you to upgrade.
You can verify your Python’s version by typing the following command in the terminal:
python --version
If your Python version is above 3.3 and still, you’re facing this issue; perhaps there’s another underlying cause. You may have a file or module with the same name ‘collections’ somewhere in your PYTHONPATH that conflicts with the standard library’s collections module. In such scenarios renaming the file or folder helps.
Let’s discuss the solution. Instead of trying to import ‘MutableMapping’ directly from ‘collections’, you need to import this from ‘collections.abc’. Here’s how it works:
from collections.abc import MutableMapping
Using the above statement for import will resolve the issue and allow the successful import of MutableMapping. It’s essential to note that progressing along with newer versions of Python will minimize future instances of such confusions, as backward compatibility doesn’t guarantee full functionality for depreciated classes, functions, or methods.
To avoid facing this problem in the future, make sure you:
- Use Python versions that are most up-to-date but stable, ensuring broad community support and maintenance.
- Avoid naming your scripts or creating modules having a similar name to Python’s built-in libraries.
If you’re compelled to use an older Python version, consider looking into backport packages like “future”, which could provide compatibilities for current features like collections.abc on older Python distributions.
In software development, such hitches are widespread. A fellow developer might just be stuck with the same nuance the other day. Online communities like StackOverflow foster a great place to look for solutions and also share yours when you solve one.
You might encounter the error message “Cannot Import Name MutableMapping from Collections” especially when you’re dealing with Python codes. This issue is primarily associated with the changes in Python versions, specifically from Python 3.3 to later versions.
In older Python versions (<=3.3), 'collections' module was used to import 'MutableMapping'. But starting from Python 3.4, 'MutableMapping' and other ABCs have moved to 'collections.abc'. However, they are still accessible via 'collections' but it's no longer recommended; thus, raising an ImportError. Let's break down these solutions into a step by step guide: Dealing with Python Versions (Python 3.3 and Before)
If you’re using Python 3.3 or earlier versions, it’s correct to use:
from collections import MutableMapping
This should work seamlessly without any issues.
Dealing with Later Python Versions (Python 3.4 onwards)
However, if you’re on Python 3.4 or later versions, you need to change your imports as follows:
from collections.abc import MutableMapping
Make sure to replace every instance where ‘collections.MutableMapping’ is mentioned with ‘collections.abc.MutableMapping’. If the former is used in many places within your code, a Find-and-Replace command might be more efficient.
ImportError Due to Third-Party Libraries
Sometimes, the ImportError might arise due to a third-party library that has not updated their package for later versions of Python. In such cases, update all your dependencies to the latest versions.
Furthermore, consider opening an issue or a ticket on the repository of the specific package that causes the import error to inform the maintainers of the issue.
Also, a good practice is to keep up-to-date with Python’s official documentation and its recent changes – this would help you avoid such issues in the future.
To sum up, the solution to “Cannot Import Name MutableMapping from Collections” ImportError involves:
– Recognizing the Python version you’re currently running
– Adjusting the code to suitable import statements depending on the Python version
– Keeping your third-party libraries updated and coordinating with their repositories for unresolved issues.
By executing the appropriate resolution corresponding to your context, you will smoothly resolve the ImportError.
In Python’s collections module, you may encounter various common errors. One prevalent error is: “cannot import name ‘MutableMapping’ from ‘collections'”. This error usually comes about because of changes introduced in Python version 3.3 that started moving some of the classes and objects to new modules.
For instance,
MutableMapping
, was moved to a sub-module called
collections.abc
. As a result, if your code attempts to import
MutableMapping
from the original
collections
module, it raises an ImportError exception. To rectify this error, your import command should be:
from collections.abc import MutableMapping
Another common error in python collection module revolves around the
defaultdict
function. When incorrectly used or misinterpreted, it can lead to an unexpected Keyerror exception. For example, accessing a key that’s non-existent in a
defaultdict
object triggers its default_factory attribute to supply the missing key, often contrary to the programmer’s intention.
As such, to prevent KeyError exceptions from cropping up when accessing nonexistent keys, consider initializing the
defaultdict
with appropriate values type like so:
from collections import defaultdict dogs = defaultdict(str)
Whereas, the error “Cannot import name OrderedDict from collections” usually precipitates for similar reasons like the ‘MutableMapping’ error. Starting from Python version 3.10, the class
OrderedDict
, previously accessible through the collections module directly, had been shifted to its own library. Substitute the import line with:
from collections import OrderedDict
Furthermore, potential issues might entail the
namedtuple
function. Even though
namedtuple
is proficient at creating lightweight object types, supplying wrong parameters can lead to ValueError or TypeError exceptions. Largely, these exceptions arise while using illegal variable names (beginning with digits or containing special characters) or an inadequate number of arguments. You’d need to supply
namedtuple
with valid field names during the declaration of your named tuple subclass:
from collections import namedtuple Person = namedtuple('Person', ['name', 'age'])
Hence, when encountering troubles with Python’s collections module, acquainting oneself with the changes fostered by various python versions and correct usage becomes vital. It’s also worthwhile exploring more comprehensive tutorials on how to correctly use collections in Python from resources the official Python Documentation to better abate unexpected bugs and errors.
It seems we’ve stumbled upon an interesting python challenge: the infamous
Cannot Import Name MutableMapping From Collections
error. It is quite common to encounter this error when attempting to import the
MutableMapping
class from the
collections
module in Python.
But why does this issue occur? The main reason is that the organization of some modules has changed starting with Python 3.3, including the
collections
package. Thus, it may result in import issues if you’re using older versions of Python and trying to employ newer module organizations, or vice versa.
Here are vital points to note:
-
MutableMapping
, along with other ABCs (Abstract Base Classes) such as
Iterable
,
Hashable
, etc., were relocated to
collections.abc
module from their original
collections
package partway through Python 3’s life.
- If your codebase needs to run on both old and new Python versions, this shifting can make things a bit complicated.
- You can solve this by having a try/except block around the import statement. That way, regardless of the Python version that’s used to run your code, it will always import
MutableMapping
correctly.
try: from collections.abc import MutableMapping # for Python 3.3+ except ImportError: from collections import MutableMapping # for Python 2.7
In a nutshell, remember that the structure and organization of Python’s standard library continue to evolve as the language develops. Don’t get too attached to one way of doing things because it might change in the next Python version. Do stay updated about these changes and adjust your Python coding strategies accordingly. For more mature libraries and framework, refer directly to Python’s official documentation.