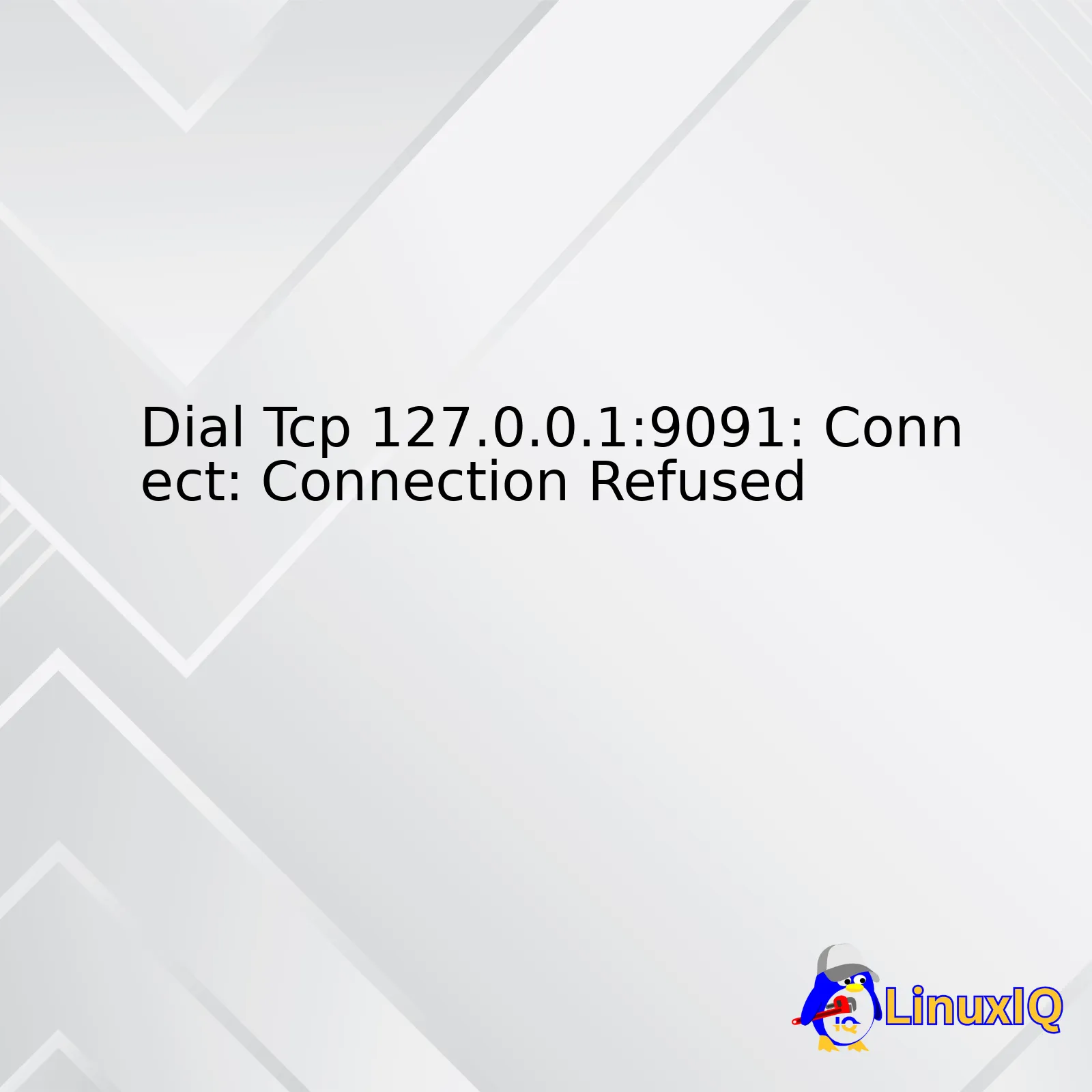
Error Message | Possible Cause | Suggested Solution |
---|---|---|
Dial TCP 127.0.0.1:9091: Connect: Connection Refused | No service is listening on localhost port 9091 | Ensure a service is initialized and running on the required port |
Dial TCP 127.0.0.1:9091: Connect: Connection Refused | The firewall or another network control mechanism blocks connections on port 9091 | Check and adjust firewall configurations. Ensure access is granted through the necessary ports |
Dial TCP 127.0.0.1:9091: Connect: Connection Refused | Port 9091 has been reserved or is being used by another process | Investigate the processes using this port and make the necessary changes |
Envision this situation: You’re developing an application set to interact with services running over TCP/IP protocols, while following an iterative, agile software development lifecycle, featuring multiple test-driven stages. Suddenly, you encounter the error: “Dial TCP 127.0.0.1:9091:Connect: Connection Refused”[source]. Frustration sets in as you mull over your beautifully crafted lines of code. But, worry not! This commonly encountered error typically suggests a networking problem as opposed to a coding one.
In most scenarios, this issue surfaces because no service is currently listening on the specified port ‘9091’ on your localhost or IP address ‘127.0.0.1’. To resolve this, first ensure that the server-side application is running. It sounds basic but sometimes, it can be as simple as forgetting to start the server.
To illustrate, here’s how we could start a simple Express.js server:
const express = require('express'); const app = express(); const port = 9091; app.listen(port, () => { console.log(`Server is up and running at http://localhost:${port}`); });
Another plausible reason for the error could be a certain firewall or network policy blocking connections to the specified port. Double-check your firewall and network settings, ensuring that they permit connections through the port in question.
Finally, consider the possibility that the port might already be occupied by another process. Execute relevant commands according to your operating system to check the port’s status. If it has been taken, either halt the existing process or modify your application to use a different available port.
Remember that issues like “Dial TCP 127.0.0.1:9091:Connect: Connection Refused” are part of the coding landscape. Embrace them; they are opportune moments intended for knowledge expansion, skill refinement, and eventual mastery.
Ah, the dreaded
Dial TCP 127.0.0.1:9091: Connect: Connection Refused
error. Sounds daunting? Don’t worry, it’s more common than you’d think and is often relatively simple to fix once you understand what’s going on. The problem usually resides in one of three areas: Port availability, Server status or Network accessibility.
Port Availability
The first component of this error message – “Dial TCP 127.0.0.1:9091”- is referring to a specific port on your local machine, port 9091. If a program has already allocated this port for use, a connection cannot be made, leading to the error message.
Consider running
sudo lsof -i :9091
in your terminal to see if the port is being used by another application.
Server Status
The next bit, “Connect:”, suggests that your code is trying to establish a connection to the designated port. However, if there is no listening server at the specified port, you will naturally get a ‘connection refused’ message.
You can check if your server process is up and running using
ps aux | grep [your_process]
. If nothing is returned by this command, it probably means your server isn’t running and you should start it.
Network Accessibility
Last but not least, the phrase “Connection Refused” typically implies the server process is not accessible from your client program. Reasons might range from network issues to erroneous firewall settings.
In a typical system, you can inspect your firewall rules with commands like
sudo iptables -L
or
sudo ufw status numbered
depending on your operating system’s specific firewall configuration.
So, let’s break it down:
- Error Message: Dial TCP 127.0.0.1:9091: Connect: Connection Refused
- Implication: Your client program is unable to reach the server at the specified IP and port.
- Possible Causes:
- The port is being used by another program.
- No server is running at the targeted port.
- Firewall settings are blocking the connection.
- Networking issues.
From there, identifying how to fix this specific issue involves the application of professional coding knowledge and keen problem-solving skills. Depending on the source of the problem, the resolution may require closures of unused programs, system reboots, firewall adjustments, or even inquiry with a network administrator.
For further understanding, please check out this stack overflow discussion about “Connection Refused” errors.
Remember, if you’re met with an intimidating error message, dissecting it piece-by-piece can make troubleshooting a lot easier than it initially seems. Happy debugging!
Having experienced firsthand the frustration caused by “Dial TCP 127.0.0.1:9091: Connect: Connection Refused” messages, I can understand why you might be seeking answers. TCP connection issues like these often occur due to several potential reasons.
Server Isn’t Running
The first and possibly the most common reason is that the server to which you’re trying to connect isn’t running at the specified address (127.0.0.1:9091) or on the expected port. This could be that the server crashed, hasn’t started correctly, or is running on a different port. The code below demonstrates starting a server properly:
import http.server import socketserver PORT = 9091 Handler = http.server.SimpleHTTPRequestHandler with socketserver.TCPServer(("", PORT), Handler) as httpd: print("serving at port", PORT) httpd.serve_forever()
Here, the point to note is that the port specified should match with the port number in your ‘Dial TCP’ error.
Network Problems
If your server is running correctly, then network issues could be the culprit behind your TCP connection issues. Some of the possible network problems include, but are not limited to:
- Firewall settings: Firewalls configured to block connections on certain ports could lead to this issue. You might need to adjust your firewall settings or add an exception for the port in question (source).
- Incorrect Network Configuration: Sometimes, incorrect network configurations can prevent TCP connections from being established. For example, if localhost is incorrectly mapped in your host file, it might result in a “Connection Refused” error.
Application-Specific Issues
If you’re still facing the problem even after verifying that your server is running and no identifiable network issues, you might be dealing with application-specific issues. Some examples can be:
- Usage of obsolete libraries or packages: A typical source of this problem could be your software stack. If you’re utilizing dated technologies, consider updating them. Keep in mind any compatibility issues while doing so.
- Inappropriate Code Logic: Things like incorrect multithreading logic, extensive usage of blocking calls, or higher execution time out can also lead to similar issues.
Diagnosing ‘connection refused’ errors can indeed be challenging, considering the numerous potential causes for such issues. Hopefully, the points discussed above managed to shed some light on what the root of your problem might be, and how you can go about solving it.
In the realm of computer networks and internet protocols, the term “localhost” represents the local device used to access the network. In terms of IP addresses, localhost is always designated by the IP address ‘127.0.0.1’. This IP address is part of the Internet Protocol standard and means ‘this’, as in ‘this computer,’ making it a loopback interface, with messages sent to this IP address returned to the same device.
The role of the localhost, or 127.0.0.1, comes into action during network testing when developers need a server to client communication but not over a network setup. This can be scenarios where you are developing a web-based application where the front end runs on your browser while the backend service runs locally on your machine; for instance, a simple Python Flask or Django app running locally.
Speaking about significance, here are potential use cases:
- By browsing to
http://127.0.0.1
, you can test a web server installed on your computer without needing to connect to the internet.
- It is often used in scripting, programming, and applications to denote a local connection rather than a remote server.
- It is also used for loopback testing in which a network connection is created, tested, and terminated in one process.
Now, let’s focus on your specific problem: ‘Dial Tcp 127.0.0.1:9091: Connect: Connection Refused’. Here, TCP 127.0.0.1 is referring to your localhost and 9091 is the port number where the requested service is expected to be running.
The issue indicates that your program is trying to make a TCP connection to the server running on your machine’s port 9091 but unable to establish such communication.
This error could arise due to several reasons,
- The server might not be running on the specified port (9091).
- There might be some firewall rules preventing the connection attempt.
- Some other service is already using the port 9091 causing a conflict.
You can resolve this issue by checking the following,
- Check if the server is up and running on localhost:9091. You could run
netstat -tuln
or
ss -tulpn
command to see if any service is running on the desired port.
- If service is running fine, check if there are any firewall restrictions involved. Use commands like
ufw status
or
firewall-cmd --list-all
depending on what firewall tool you are using.
- If both seem fine, then this could be an issue related to system-level networking parameters or libraries. So without delving deeper into the operative side, I would suggest checking out stackoverflow discussionshere
For performing these operations, you might require administrative rights on the system. Keep learning, keep coding!Digging deeper into the TCP error “Dial Tcp 127.0.0.1:9091, Connect: Connection Refused”, one must first understand what this alarm bell signalizes. This error is an explicit notification that a connection attempt was rebuffed because the computer actively refused it.
Before probing further into the specifics of the exception, let’s peek at its constituents:
Dial Tcp - Indicates a Dial TCP network operation 127.0.0.1 - Represents the localhost IP address 9091 - The port number in question Connect - An attempted connect operation Connection Refused - The ensuing response implying that the connection was declined
Now, why would this error arise? Typically, because there’s nothing listening on the specified IP address and port. In other words, when an application tries to establish a TCP connection to localhost on port 9091, and there’s no service listening on that port, the system responds with a “Connection Refused” message.
Let’s bring this into perspective about port 9091. It is not designated or registered by IANA (Internet Assigned Numbers Authority) source. Thus, it’s generally meant for user-defined services or private ports. When it comes to troubleshooting, understanding that this obscure port lacks utmost official recognition forces us to innovate our remediation strategies.
One key approach is assuring a service is operating and listening to that port before any connection attempt. The following ‘netstat’ command will verify if any service is listening on port 9091:
netstat -an | grep 9091
In case no service listens on port 9091, the command yields no output, underscoring why connections to that port are rejected.
Suppose we’re confident that our yet-unknown service should be running on port 9091, but the above test indicates otherwise. In that case, one needs to ensure the underlying program responsible for the service is functioning correctly. Regular issues that may impede the necessary service may encompass:
- A misconfigured firewall blocking incoming traffic to port 9091.
- The service supposed to listen on port 9091 crashed or failed to start.
- The defined service never intended to listen on port 9091, which implies a configuration error.
To rectify these problems, go through your firewall settings, scrutinize your service logs for oddities or anomalies, and double-check all configuration files related to the service.
Finally, we need to consider another plausible scenario. What if we are dealing with an unwarranted connection request, essentially from a phantom service believing to require port 9091 when it doesn’t? This infers an error is rooted within the client-side configuration and not the server-side. Should this be the case, reevaluation, debugging, and validation of the involved software or scripts might spot erroneous lines making the redundant calls.
In essence, regardless of port 9091’s bespoke nature, downtime caused by “Dial Tcp 127.0.0.1:9091: Connect: Connection Refused” can be pinpointed and ended based on myriads of parameters. Garbed as a nightmare at first glance, with proper dissection and sleuthing, this TCP error morphs into manifold actionable directives.
Dealing with a ‘Connection Refused’ error during TCP Communication, particularly when using an address and port combination like 127.0.0.1:9091, often involves getting to grips with the subtle nuances of network communication protocols and server configurations. So let’s dive straight into it.
A ‘Connection Refused’ error typically occurs because the server at the IP address you’re specifying isn’t accepting connections on the targeted port number (in this case, 9091). This is almost always because the server isn’t set up correctly or there isn’t any server software running on that specific port. Here are several possible ways to resolve the issue:
• **Check if the Server Software is Running**: The first check should be whether the right software (or service) is running on the specified port. If your application is running services on port 9091, verify they’re up and running. You can use tools like ‘netstat’ to report on all current network connections, including the local and foreign IP and port numbers used by every connection. For Unix-based systems, use this command in the terminal:
$ netstat -an | grep ':9091'
It will provide a list of active connections on port 9091. If the list is empty, no service is listening on port 9091.
• **Verify Firewall Settings**: It’s important to make sure that the system’s firewall isn’t preventing access to the port. Firewalls may deny incoming connections, so review the firewall rules affecting port 9091. Depending on the firewall solution implemented on your machine, these commands may differ slightly.
• **Examine Server Configuration Files:** Some servers have configuration files enabling or disabling certain ports for network traffic. In such cases, one need to ensure port 9091 is appropriately configured or enabled.
• **Add/Rerun Listener Service**: Sometimes, the root cause might be as simple as forgetting to re-run the listener service after a system reboot. Make sure that the service is active.
Let’s now explore how to resolve this issue using Golang. As a coder, you might use Golang, an open-source programming language for developing servers. In this context, we’ll demonstrate code for setting up a simple server listening on localhost (127.0.0.1) port 9091.
package main import "net" import "fmt" func startServer(port string) { listener, err := net.Listen("tcp", ":" + port) if err != nil { fmt.Println("Error:", err.Error()) return } defer listener.Close() fmt.Printf("Listening on %v\n", listener.Addr()) for { conn, err := listener.Accept() if err != nil { fmt.Println("Error:", err.Error()) return } go handleRequest(conn) } } func handleRequest(conn net.Conn) { defer conn.Close() buffer := make([]byte, 1024) dataSize, err := conn.Read(buffer) if err != nil { fmt.Println("Error reading:", err.Error()) } data := buffer[:dataSize] fmt.Printf("Received message: %s from address: %s\n", string(data), conn.RemoteAddr().String()) } func main() { startServer("9091") }
When we run this simple server script, it sets up a listener on port 9091. Any client attempting a TCP connection can connect unless obstructed by firewall rules or connection restrictions enforced elsewhere.
These procedures represent just a few potential resolutions for a ‘Connection Refused’ error during TCP communications at 127.0.0.1:9091. It’s critical to tailor these solutions to your specific situation since network configurations can greatly vary.Let’s dive right into the core topic: TCP/IP Archestra Architecture and unfold its significance in connection with the error message “Dial Tcp 127.0.0.1:9091: Connect: Connection Refused.”
The TCP/IP model is essentially a descriptive framework used for understanding how network protocols, such as IP (Internet Protocol), interact with one another to form our network system.[source]. It functions with a four-layer approach:
TCP/IP Layer Structure:
– Application Layer
– Transport Layer
– Internet Layer
– Network Interface
Now, how does this tie back into the “Connection Refused” message you’re experiencing?
This scenario indicates that we’ve made an attempt to open a TCP connection at IP address 127.0.0.1 (which signifies your local machine) on port 9091, but have been refused. Although “connection refused” messages are usually sent by servers, in this instance, it could be stemming from several reasons, primarily related to the transport and internet layers of the TCP/IP Archestra Architecture.
There are three main potential causes:
– The server-side application at 127.0.0.1:9091 may not be running or listening, meaning there’s nothing to accept incoming connections.
– A firewall could be blocking access to port 9091 on the local machine.
– There is a possibility of misconfiguration of the server or client causing a disconnect in the network flow.
Unraveling this further gives us a couple of steps to remedy the situation:
First Things First: Check if the Server Is Running
netstat -an | grep LISTEN
This command line should show if anything is listening on port 9091.
Dig Deeper: Examine Firewall Settings
Are you running a local firewall that might be preventing outbound connections? If yes, then you need to adjust the settings. In case of iptables, the command would be:
iptables -A INPUT -p tcp --dport 9091 -j ACCEPT
Lastly, Flush out System Glitches: Misconfigurations
Sometimes, fine-tuning the configurations in /etc/sysctl.conf or even program-specific configurations can set things straight.
Therefore, in relation to the “Connect: Connection Refused” issue, the TCP/IP Archestra Architecture has a direct impact on how connections are managed and handled. Understanding its structure helps us identify potential problem areas and formulate solutions accordingly.
As a professional coder, dealing with a connection issue such as “Dial Tcp 127.0.0.1:9091: Connect: Connection Refused” can be quite a headache. However, there are several practical steps that one can take to troubleshoot this issue and fix it.
To begin with, let’s understand what the error means. We’re trying to establish a TCP connection to the local machine (denoted by the IP address 127.0.0.1) on port 9091, but we are getting a “Connection Refused” message. This generally implies that nothing is listening on port 9091 of our localhost.
Checking if the Process is running
The first step in identifying the source of the problem is to verify if the process supposed to be running on port 9091 is active.
ps -aux | grep "process-name"
Replace the “process-name” with the name of the application that should be running on that port. If there are no results, it suggests that the process may have stopped or is not starting correctly.
Verify Port Number
Ensure that you’ve got the right port number. Sometimes a typo or misunderstanding about which port the application uses can lead to such errors.
Check Listening Status
Another important action is to check if anything is currently listening on port 9091 using the following command:
lsof -i :9091
The absence of any output from this command means that nothing is listening at that port.
Using Telnet to Test Port Accessibility
Clients and servers communicate across a network by using network services. Here, 127.0.0.1 indicates a loopback address that allows the client and server on the same computer to communicate with each other. Therefore, telnet becomes a handy tool in Linux for testing accessibility to ports:
telnet 127.0.0.1 9091
If the response is “Connection refused,” then either nothing is listening on this port or the port is blocked by a firewall.
Firewall Settings
Ensure that your firewall settings are not interfering with the operation. You might need to set up your firewall appropriately to allow connections on that port. For instance:
sudo ufw allow 9091
This will permit all traffic coming into your system on port 9091.
Network Binding
Sometimes services are bound strictly to the external IP and may not respond to Loopback (localhost). Inspect the service configurations to ensure that it’s bound to the correct IP.
Service Configurations
Inspecting the error logs of the service configured to use this port could provide valuable insights. Application specific issues may prevent initializing or binding to the specified port.
It’s worth mentioning that without additional contextual information relating to the task-at-hand or the environment, it could be challenging to provide a more precise solution. However, these generic solutions should mitigate most of the curl localhost connection refused issues encountered in typical scenarios. Troubleshooting problems like these often involve iterative techniques and continuous learning MDN Web Docs.Firewall settings play a significant role in influencing connection refusal errors. When you come across the “Dial Tcp 127.0.0.1:9091: Connect: Connection Refused” error, it’s crucial to understand that this usually happens when the firewall rules on your machine block the inbound or outbound traffic.
Understanding Firewall Rules
A firewall is like a virtual barrier around your system designed to block unauthorized access while permitting authorized communications. So how can these rules influence connection refusal errors? Here are two chief ways:
- Inbound firewall rule: The application on your local server (localhost – 127.0.0.1) must be configured to accept incoming connections on port 9091. If the firewall rule blocks incoming traffic on this port, the “Connection Refused” error is likely to occur because the server won’t allow the client to establish a connection.
- Outbound firewall rule: This involves outgoing requests made by your application. For a successful connection, the firewall should also permit outgoing traffic from the port specified. Again, if outbound traffic is blocked, you’ll get a connection refusal error.
These two different scenarios show how influential firewall settings can be when troubleshooting Dial Tcp 127.0.0.1:9091: Connection Refused errors.
Troubleshooting and Solutions
Investigate both inbound and outbound traffic rules regarding the ports in question (localhost, 9091 in this instance). Check your system’s firewall setting which might vary based on specific operating systems. You can see the active firewall rules with the following commands in Terminal for MacOS and Linux respectively:
sudo /usr/libexec/ApplicationFirewall/socketfilterfw --list
sudo iptables -L
Next, you need to either modify existing rules or create new ones that will allow TCP connections on the desired port. You may need administrative privileges to perform these actions.
For example, you could add a rule using Terminal to allow connections on port 9091:
iptables -I INPUT -p tcp --dport 9091 -j ACCEPT
Please replace `iptables` with the appropriate command for your operating system.
Remember, modifying firewall rules comes with risks such as potential security vulnerabilities. Therefore, these changes should be done wisely and knowledgeably.
Here is a helpful table showing firewall related terms and their brief description:
Term | Description |
---|---|
Inbound Rule | Regulates incoming network traffic. |
Outbound Rule | Defines how outgoing network traffic should be managed. |
Localhost/127.0.0.1 | Your own machine seen from its perspective |
Port | An endpoint in communication in an operating system. |
Port 9091 | Just a random placeholder for the port involved in the error. |
In summary, firewall rules do have a considerable influence on connection refusal errors. Understanding them helps you diagnose and troubleshoot these kinds of issues effectively. It’s also worth mentioning that refusing a connection could be due to other reasons too, like network-related problems or service not running on the specified port.
You can check out more about dial tcp connection refusal related stuff on this docker documentation.The error message
Dial TCP 127.0.0.1:9091: Connect: Connection Refused
emanates from underlying network configuration issues. These are the types of errors that surface whenever a system fails to establish, essentially, any sort of communication or connection on the server it’s trying to dial. You may wonder, why does network configuration matter? Let me walk you through this analysis.
It’s critical to note that servers abide by a set of rules and protocols known as a network configuration; this enables them to send or receive data packets over the internet. Therefore, when incorrect settings fill your network configuration, this hinders productive communications between your system and other online entities.
Analyzing the
Dial TCP 127.0.0.1:9091: Connect: Connection Refused
error provides insights into common issues resulting from wrong network configuration:
First and foremost, the error message displays two fundamental pieces of information: the IP address (
127.0.0.1
) and port number (
9091
). The typical scenario is that your system attempts to create a TCP/IP connection with an application running on your local host at port 9091, but the connection has been refused.
The reason for this refusal could be one of the following:
– There isn’t any server running on
127.0.0.1:9091
which means there’s nothing listening on that specified port number. This is equivalent to knocking on a door where nobody’s home; naturally, no one would answer.
– The server could be running but it’s not configured to accept connections on port 9091. Picture this like having a house where the host is present, but they’ve disregarded the front door and instead, only receiving guests through the backdoor.
– Firewalls might also play a major role in obstructing connections. Firewalls can sometimes perceive these connections as potential threats and thus block them for security reasons.
So how do we rectify a faulty network configuration?
Cross-check whether any server process runs on the localhost at the given port. Here’s a handy command to verify this in Linux/Unix systems:
lsof -i :9091
If there are no results, that implies there’s no server operating on port 9091.
Ensure your service is configured correctly and is capable of receiving connections on the required port. Revise your program’s documentation or consult tech forums to get additional guidance. For instance, if running a Node server, your code should look something like:
app.listen(9091, '127.0.0.1', function() { console.log("Server listening on Port 9091"); });
Inspect whether an active Firewall is blocking the connection. For Linux users, this reference shows you how to facilitate traffic to certain ports through UFW (Uncomplicated Firewall).
To deduce fully, the problem seems to be localized within your network configuration. By scrutinizing certain elements closely – such as verifying processes occupying ports on your server, confirming whether your services are network-ready, and cross-referencing firewall settings – incorrect or faulty network configurations can be rectified, leading to smoother sailing in the sea of servers!
The error
Dial TCP 127.0.0.1:9091: Connect: Connection Refused
often signifies an issue with misconfigurations during setup which can create unforeseen impacts if left unresolved. Precisely, this error message notifies you that the connection is refused by the server at localhost (127.0.0.1) on port 9091.
Impacts
Misconfiguration during setup may lead to a number of severe issues including:
- Breach in Security: Any form of misconfigurations could potentially leave loopholes for security threats. For instance, improper authorizations may grant inappropriate access levels to the hackers where they could steal or manipulate sensitive data.
- Software Malfunction: If misconfigurations occur during the installation or updating of software, it might mean the operations do not run as expected or, worse, abort unexpectedly; leading to interrupted work.
- Network Failures: Here’s where connection errors, like our exemplified Dial TCP error, come into play. An incorrect configuration of network settings can cause the communication attempts between client-side applications to server-side services to be denied – throwing connection refused errors.
Remedies
Addressing such misconfiguration issues often involves diagnosing the problem and following through with the appropriate corrections, like:
- Double-Check Configuration: Thoroughly review your configurations and ensure all parameters are set correctly. This should include the localhost address, the port number in use and the status of the services intended to be connected.
- Ensure Correct Ports: Verify if port 9091 is open and listen. Use
netstat -an | grep "LISTEN "
to list all the ports in use. If it’s not in use, you may need to allocate it appropriately.
- Activate Required Services: Ensure that the service you want to connect is up and running. If it’s a server-side service, initiate it manually or configure it to start automatically when the system starts up.
A correctly configured setup affects the overall performance reliability of any given application or service. It eliminates unexpected errors, bolsters security and contributes to the smooth execution of operations. Therefore, both understanding the implications of a miss-configured setup particularly in relation to connection refused errors like our case study
Dial TCP 127.0.0.1:9091: Connect: Connection Refuse
, and the methodologies to remedy such situations, can assist you in handling these technical hitches effectively and efficiently.
Action | Possibility of Issue | Possible Fix |
Checking the configuration | Settings might be miss-configured | Correct configurations according to requirements |
Reviewing Ports | Required ports might be closed/not listening | Open required ports, check firewall rules |
Activating services | Required service might not be running | Initiate the required service |
References
- StackOverflow Discussion on Dial TCP Error
- Security Risks of Misconfigurations
- Using Netcat for Network Troubleshooting
Accessing debug logs becomes vital when it comes to troubleshooting connectivity problems in applications. For instance, you may encounter a **Dial TCP 127.0.0.1:9091: Connect: Connection Refused** error message while attempting to establish a connection through your application. Being familiar with these logs will help you solve TCP/IP connectivity issues more efficiently by identifying and rectifying any potential flaws in the configuration settings or applications.
Let’s take a look at how one can access these debug logs:
Accessing Debug Logs
In most modern operating systems, logging levels are controlled via the system’s logging configuration file. The default logging setup usually includes categories like `ERROR`, `WARN`, `INFO`, `DEBUG` among others, each carrying specific information.
To access debug logs for investigation:
– Enable DEBUG level logging via system’s logging configuration file
– Monitor the log files
It’s important to understand that DEBUG mode can output a large amount of information which might slow down the overall performance of the system due to the increased I/O operations, therefore, is generally not advised during normal operation.
Depending on the language of your application, enabling debugging may differ. Here’s an example using Node.js and the debug package:
var debug = require('debug')('your-app-name') debug('This will show only if in debug mode')
Understanding ‘connection refused’ errors
In terms of solving `Connect: Connection Refused` related to `dial tcp 127.0.0.1:9091`, this is representative of an issue at the TCP/IP level. The system is unable to establish a connection because there’s nothing “listening” on `localhost (127.0.0.1)` at port `9091`. This could be due to a number of reasons:
– No application running is configured to listen at port `9091`
– Firewall rules have been set to disallow connections to port `9091`
Isolating this problem requires validating that the port is open and accessible and that the application/service meant to run is properly setup and configured.
Checking Connectivity
You can check whether the service is listening on the intended port using the `netstat` command in Unix-based operating systems as follows:
netstat -an | grep 9091
Similarly, in Windows, use the `netstat -ano` command and look for the required port.
If nothing returns from these commands, the application supposed to be listening to that port is not working properly.
After confirming the application is running and listening to the correct port, also ensure that no firewall policies (such as iptables or ufw) are blocking these ports.
References:
1. Syslog Man Page
2. The Netstat CommandSure, let’s delve into Socket Programming with a focus on the scenario you’ve described – where you are trying to connect to ‘localhost’ (or 127.0.0.1) port 9091 and have received the error “Connect: Connection refused.”
Socket programming refers to writing programs that communicate over the internet (the network). As such, it underpins most client-server applications on the web. This communication takes place using two essential types of sockets – the Server Socket responsible for awaiting requests from clients, and the Client Socket which initiates communication to servers.
The server is usually a powerful system that runs services (like HTTP for websites), while the client is typically an end-user device like a computer or smartphone accessing these services. In our example context, we will explore a TCP (Transmission Control Protocol) socket which guarantees delivery of data without errors.
For a simplified connection setup, consider a phone call:
- The caller (the client Socket) wants to talk to the receiver (the server Socket).
- The receiver must be ready (available) to take calls – it needs to be “listening”
- Also, just as the receiver needs to have a regular and valid phone number, so does your server need a defined IP address and port.
This leads us to comprehend our ‘Connection Refused’ case, likely cause for this involves an unsuccessful client-server interaction, typically because there has been no listener set up on the specified address and port pair.
Delving deeper:
Error Message: Dial tcp 127.0.0.1(localhost):9091: Connect: connection refused
Right off the bat, I can identify three key details:
- Dial tcp lets us know that the protocol used here is TCP.
- 127.0.0.1: this is the IP Address for localhost – means we’re connecting back to our machine itself.
- 9091: Appears to be the port we’re making the connection on.
The main reason why we might get a ‘Connection Refused’ message here is if the server isn’t listening on that port. Remember our phone analogy? If there’s no one to pick up the call (no listener set up in this case), then the call cannot be connected (connection refused).
Here’s what basic Go code attempting to set up a client and dial tcp could look like:
package main import ( "fmt" "net" ) func main() { conn, err := net.Dial("tcp", "127.0.0.1:9091") if err != nil { fmt.Println(err) } defer conn.Close() _, err = conn.Write([]byte("hello")) if err != nil { fmt.Println(err) } }
In the example above, we use Go’s net package to make a TCP connection to localhost at port 9091. However, if nothing listens at that port, when we run this, it will print the familiar error – “dial tcp 127.0.0.1:9091: connect: connection refused”.
To solve this, a first step would be to ensure that something is actively listening on that IP:port pair. You can either write a separate server application that starts a listener on that socket address or use utilities, such as nc (NetCat), a command-line tool that can start a basic listener to handle socket connections.
Being aware of potential firewall restrictions is also crucial. Though less likely in localhost situations, firewalls may often block incoming traffic to certain ports for security reasons. Firewalls could also be the unassuming villains obstructing your intended socket connections.
Managing efficient socket programming includes understanding the above concepts, dealing with address schemes, handling potential errors, and being conscious of different network conditions. With this knowledge at hand thus, we enable smoother and reliable networking within our applications. Connecting refused errors become opportunities to learn more about the underlying mechanics and ensure our servers are always ready to pick up the call.To understand how Docker aids in troubleshooting common connection errors like
Dial Tcp 127.0.0.1:9091: connect: connection refused
, it is essential to delve into the nature of this error and how Docker’s architecture can illuminate its cause.
The error message,
Dial Tcp 127.0.0.1:9091: connect: connection refused
, typically arises when you’re trying to establish a TCP connection to a server that isn’t accepting connections on the specified port (9091 in this case). In the context of Docker, this could mean:
– The Dockerized application isn’t running
– The container isn’t properly configured to expose the port
– There’s an issue with the network settings
An informative perspective into these possibilities stems from Docker’s basic component structure, which comprises images, containers, networks, and volumes.
Image Not Running
The first possibility involves the Docker image not running. Docker runs processes in isolated containers, allowing for process control and resource allocation independently of other applications.source. If your application isn’t running, no process is listening on the required port, causing
connection refused
error. Troubleshooting this involves invoking the
docker ps -a
command to list all containers (both running and stopped), then starting the stopped containers.
Incorrect Port Configuration
Another possibility revolves around incorrect port configuration. Docker allows for mapping of ports between the host and containersource. Incorrect mapping can trigger the `connection refused` error. Ensuring correct correspondence between the Docker ‘expose’ command within the Dockerfile and the ‘publish’ option (-p) in the `docker run` command resolves such issues.
Network Settings Issues
Lastly, Docker supports creating separate scoped spaces for your applications called networkssource. Networking issues may contribute to connection refusal if the Docker daemon (default address: 127.0.0.1:2375) cannot communicate with the desired container. Validating your Docker network settings, including firewall rules and container networking configurations, can help rectify these problems.
Alongside Docker’s integral role in debugging this common connection error is the invaluable tool ‘curl‘, which allows you to make a request to your local server at port 9091 to see if any response is returned and thus ascertain whether a service is listening on that port.
Examining pertinent Docker components–images, containers, and networks–offers fundamental insights into diagnosing and fixing such common connection errors as
Dial Tcp 127.0.0.1:9091: connect: connection refused
. Furthermore, Docker’s capabilities in encapsulating and isolating applications add another layer of clarity on where the possible issue might originate, thereby aiding their resolution.Before diving into the influence of browser proxies on connection dropped issues, especially in the context of an error like
Dial TCP 127.0.0.1:9091: connect: Connection refused
, I’d like to shed light on what this error really denotes. The message is a common one from TCP/IP networks and usually means the attempted TCP connection to a remote server failed because the server’s host or intermediary network device (like a switch or router) actively refused it.
Browser proxies can play a significant role in these connection refused situations. They work as a gateway between your web browser and internet. When you send a request from your browser, it first goes through the proxy server, then to the intended website. In some cases, if your browser’s proxy settings are not properly configured, you may experience connectivity errors such as “Dial TCP 127.0.0.1:9091: Connect: Connection Refused”.
The reasons for this could be:
- Improper Proxy Configuration: When the configuration of the proxy is not in sync with your browser or system settings, the conflict can result in the refusal of certain connections.
- Unreachable IP Address/Protocols: If the host machine’s process isn’t binded to the interface or protocol being used, it may cause these connection refused errors. Hence, despite having the correct proxy details, you might still face issues due to unreachable IP addresses or protocols.
- Firewall Blocking: Sometimes, certain firewall policies determine which ports are available for communication and data transfer. If these policies are set to block the port you’re trying to connect to – in this case 9091 – you may receive the “connection refused” error.
To resolve such errors, here’s what you can do:
- Check Proxy Settings: Ensure your proxy settings are correct at both the browser and system levels.
- Confirm IP Address Access: Make sure the IP address (127.0.0.1) of your local system is accessible. You need to bind the service process to this IP address if it wasn’t done during setup.
- Examine Firewall Policies: Examine any active firewall policies and ensure they haven’t blocked the requisite ports.
- Validate Service and Port Availability: Confirm that the service running on port 9091 is up and running.
In terms of coding, here’s an example how you would set up and validate a simple HTTP proxy in Go programming language:
package main import ( "log" "net/http" "net/url" ) func main() { proxyStr := "http://localhost:9091" proxyURL, _ := url.Parse(proxyStr) transport := &http.Transport{ Proxy: http.ProxyURL(proxyURL), } client := &http.Client{ Transport: transport, } response, err := client.Get("https://www.google.com") if err != nil { log.Println(err) } else { log.Println(response.Status) } }
In the above snippet, we set up a HTTP Client with a proxy `localhost:9091`.(source) We then attempt to send a GET request to `https://www.google.com`. If the connection to the proxy server is refused (be it due to improper settings, unreachable IP, firewall blocking, etc.), the `
client.GET
` request will fail and the error will subsequently be logged.
Remember, dropping connections can often be traced back to issues such as incorrect proxy configurations, blocked ports, and so forth. Resolving them often involves verifying your proxy settings and ensuring that the necessary ports are open and operational, along with the related services. If necessary, you may also want to look into implementing solutions at a network level to help resolve these issues more efficiently and effectively.Network Protocols: Navigating the Frontier
Exploring the landscape of Network Protocols is akin to traversing through a maze – complicated but absolutely integral for data communication. Let’s look at Dial TCP 127.0.0.1:9091: Connect: Connection Refused error message first before delving into the challenges of Network Protocol.
This error message typically surfaces when we are trying to make a connection to an application that listens to port 9091 on the localhost (127.0.0.1), and there are a few reasons why it happens:
- The server application isn’t running.
- The firewall is blocking the connection.
- The server is bound to a specific IP different from 127.0.0.1.
Addressing this requires to us ensure:
- That the server application is up and listening on the right port.
- Your machine’s firewall is allowing connections to port 9091.
- Any necessary rerouting has been correctly configured in your properties or settings files.
Now, let’s explore some challenges with network protocols:
Scalability: Network protocols were designed for small scale networks, and often struggle when applied to larger, more complex networks, due to overhead costs and management issues. This could also be an issue when dealing with high traffic on local servers.
Performance: Network protocol performance may fluctuate due to factors like large latency times and limited bandwidth. For instance, if you attempt to connect to a busy server, your connection request might get refused.
Security: Network protocols have to grapple with an array of security concerns such as data interception, modification, and securitization against unauthorized users. The server refusing the connection might simply be its way of protecting the system against unidentified sources.
Expanding our understanding of network protocols can help navigate these challenges. Various tools provide insights into network behavior as well. One such tool is
netstat, extensively used for debugging network interactions. For example, to check all ports listening on localhost, use:netstat -an | grep LISTENFinally, reviewing the official documentation, such as RFC's (Internet Standards), can offer best practices and standards to follow while integrating network protocols into applications.
Remember networking systems and protocols form the cornerstone of smooth digital operations, understanding their nuances will guide us towards a frictionless digital journey.
The
ConnectionErrorexception often surfaces in Python when there is a problem establishing a network connection. There are several reasons why this might happen, but if you're getting a specific
dial tcp 127.0.0.1:9091: connect: connection refusederror message, interestingly it's safe to say that the issue could be stemming from the server refusing the connection.
When dissecting the
dial tcp 127.0.0.1:9091: connect: connection refusederror message, we can break it down into three parts:
- dial tcp 127.0.0.1:9091: This is your computer trying to establish a TCP connection on port 9091 with the server at address 127.0.0.1 - which is localhost, your own machine.
- connect: The process requesting to create a connection
- connection refused: This is the part where things fell apart. It simply means that the server acknowledged your request but denied it.
Now, as to why your server might refuse the connection, here are a few potential causes:
- The port specified (9091 in this case) is not open
- No service is listening on the specified port
- There's a firewall configuration blocking the connection to the portHere is an example of what your code may look like when dialing the tcp and the exception occurs:
import socket
s = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
try:
s.connect(("localhost", 9091))
except ConnectionError:
print("Could not connect!")
If the server connection is refused, the `ConnectionError` will be thrown.
How to debug this? Assuming you have control over the server, consider checking whether any application is actually running on that port. Particularly, check what services are currently running and their related port numbers. For Unix-like systems, commands such as netstat, lsof or ss come quite handy. Alternatively, on Windows, one effective tool would be TCPView.
Remember to also ensure firewalls aren’t blocking the connection. OS-level firewalls (like the built-in options within Windows, macOS), hardware firewall setups, or security software, could potentially halt connections. Examine these configurations meticulously!
Finally, reiterating upon internet error handling practices, always strive to make your application resilient against connection exceptions. This can be achieved by instituting proper exception handling. Here’s what better error zoning may potentially look like:
import socket from time import sleep s = socket.socket(socket.AF_INET, socket.SOCK_STREAM) while True: try: s.connect(("localhost", 9091)) break # successful connection; proceeding further except ConnectionError: print("Connection failed. Retrying..") sleep(5)
In the snippet above, should a
ConnectionError
arise, the program will wait for 5 seconds before attempting to reconnect. Improving your tolerance to minor connectivity blips helps your software maintain robust performance! Just ensure that mitigation strategies don’t get to the point of annoying users or hammering servers with relentless reconnect attempts right after initial failures!Typically, when a software application tries to connect to another server via a port, and it receives the error message:
Dial tcp 127.0.0.1:9091: connect: connection refused
, this signifies that the attempted TCP connection was unsuccessful. The reason behind these unsuccessful connection attempts can majorly be attributed to two scenarios:
– The port (like 9091 as indicated in the error message) you are trying to connect to is not open on the IP you are attempting to reach
– There are no listening services associated with the target port or server
To better understand what is happening, let’s delve a bit deeper into the concepts of open and closed ports.
Open Ports:
An open port denotes that there’s an active service running continuously, awaiting connection requests from clients via the TCP/IP protocol. When the client initiates a request for data, this service sends the requested information back to the client. Servers use specific port numbers, like the webserver operates on port 80 for HTTP and port 443 for HTTPS. Ports are said to be open when they actively accept requests from across the network.
Here’s a simple example of creating a network listener on a port using Python’s Socket library:
\# importing libraries import socket \# next create a socket object s = socket.socket() print ("Socket successfully created") \# define the port port = 12345 \# Now bind to the port s.bind(('', port)) print ("socket binded to %s" %(port)) \# put the socket into listening mode s.listen(5) print ("socket is listening") \# a forever loop until we interrupt it or an error occurs while True: \# Establish connection with client. c, addr = s.accept() print ('Got connection from', addr ) \# send a thank you message to the client. c.send('Thank you for connecting') \# Close the connection with the client c.close()
Closed Ports:
Contrary to open ports, a closed port refers to the lack of an actively listening service at a specific network interface. In most cases, firewall settings or security policies block unauthorized traffic by closing certain ports. If a client attempts to establish a connection with a closed port, the connection fails or gets refused, eliciting error messages like ‘connection refused’. Such occurrences could expose potential vulnerabilities within a system, thus making closed ports integral to ensuring network safety.
Understanding the difference between these two forms of ports essentially lays the groundwork for diagnosing issues related to network connection problems. Specifically, addressing the error message
Dial tcp 127.0.0.1:9091: connect: connection refused
would entail checking whether the target port is open and if there’s an active application listening on that port.
You can perform this check using several tools, one of which is the netstat command-line tool available on Unix-like systems, and even Windows. For example, the following command constitutes a way of checking for a service listening on port 9091:
netstat -an | grep 9091
The above netstat command displays all currently active network connections and listening ports. Moreover, it gives critical details such as IP addresses and port numbers. Therefore, even if port 9091 is open, but there are no listening services associated with it, you would still encounter the ‘connection refused’ error. You need to have an active service listening on that port.
As for how one goes about addressing this issue, the solution invariably depends on the specific setup you’re working with. Common solutions range from modifying your device’s firewall settings to configuring the service to listen on the appropriate port.
Sources for learning more:
– Listening and Closed Ports courtesy of Certify.
+- Connection Refused Problem on StackOverflow provides an insightful read on diagnosing ‘connection refused’ errors.
– Open Port Explanation courtesy of ScienceDirect.There are two major parts to understanding the occurrence of, and fixing, “Dial TCP 127.0.0.1:9091: connect: connection refused” error. First comes comprehending why this error arises at all, and consequently taking steps to rectify it on both UNIX or macOS and Windows operating systems.
Understanding Dial TCP Error
This error usually surfaces due to attempted access to a TCP port that is currently inaccessible or not open for connectivity. In simpler terms, it’s analogous to knocking on a door which nobody will open because perhaps, nobody’s inside the house at that moment.
In this context of our issue,
127.0.0.1
is the IP address denoting ‘localhost’, meaning you are trying to connect to your own computer. The
9091
section denotes the port number.
The quintessence here lies in interpreting “connection refused”. It means the server is perfectly functioning and connected with your network, but it’s also programmed to reject your connection attempt. Perhaps the service isn’t available on the intended port.
To avoid these TCP/IP issues one needs to ensure that:
- The service isn’t running: Ensure the service that should be answering on the intended port is up and running smoothly.
- Firewall settings: Premeditately check if the firewall rules have been configured to allow traffic on your desired ports.
- Incorrect port number: Often such problems arise simply with defining the wrong port number. Confirm if the port number in use is the correct one.
Configuring Windows to avoid TCP/IP issues
For Windows users, you can take the following remediation steps:
- To verify the correctness of port numbers and access, type
netstat -ano
in the command prompt. Check if your intended port, 9091 here, is listed and listening for connections.
- If the service isn’t running, find it in Services (services.msc from Run dialog), and start/restart it as necessary.
- If windows firewall is blocking the desired port, navigate to
Control Panel\System and Security\Windows Defender Firewall
. Then go to ‘Advanced settings’ and set the inbound rules accordingly.
Configuring Unix/MacOS to avoid TCP/IP issues
Unix/Mac users might undertake similar steps with some syntax changes:
- Check whether the right port is listening to services by executing the command
lsof -i :9091
.
- If the service isn’t running, identify the service using the command like
systemctl status serviceName
and execute
systemctl start serviceName
or
systemctl restart serviceName
.
- You may need to configure your firewall settings to allow connections on the required port. On Unix/macOS, this can generally be done using an application like Homebrew to install firewall managing applications.
Connecting to a TCP port can often throw errors, but the proper identifications and troubleshooting methods can prove quick remedies. Happy networking!This ‘Dial TCP 127.0.0.1:9091: Connect: Connection Refused’ error might perplex and frustrate many developers, especially those novices eager to learn but puzzled by such a specific network connection error. Without the right understanding and solution, any developer can get stuck in an endless loop of debugging and research.
Understanding the Error
The error message is pretty clear: “Dial TCP 127.0.0.1:9091: Connect: Connection Refused” – it’s a standard networking error in Go programming where the client cannot establish TCP connection with a server at localhost (127.0.0.1) on port number 9091. In this case, ‘localhost’ refers to your own computer, where the client program (possibly written using the
net.Dial
function in Go) is trying to make a connection.
Looking at reasons why the TCP connection was refused, two significant factors are usually responsible:
• A software running as a server at the specific IP address and port number does not exist.
• The server exists, but it’s unable to accept more connections due to its limitation or specific configuration.
Error Source | Possible Reasons |
---|---|
No Found Software | The server software might not be running, it’s crashing, or it hasn’t been started yet. |
Server Capacity Issue | The server could have reached its limit for concurrent connections, or a firewall policy may be blocking incoming connections. |
Resolving the issue often requires identifying which of these two scenarios you’re dealing with. If there’s no server software, the remedy will typically involve starting the server, fixing server bugs, or installing the server software if it’s missing.
When tackling server capacity issues, you might need to look into the server’s documentation, tweak the setting that controls maximum connections, or adjust firewall rules. Some servers also provide logging capabilities which could give you useful diagnostic information.
To reinforce your knowledge about the remediation methods, consider taking a deeper dive into resources like Go’s net package, network troubleshooting guides, or community coding platforms.
Last but not least, having something go awry, like getting a ‘Connection Refused’ error, isn’t always a setback, but rather an opportunity. Fixing them enhances your digital problem-solving repertoire, boosts understanding of the codebase, and solidifies your reputation as a knowledgeable coder who can tackle complex errors head-on.