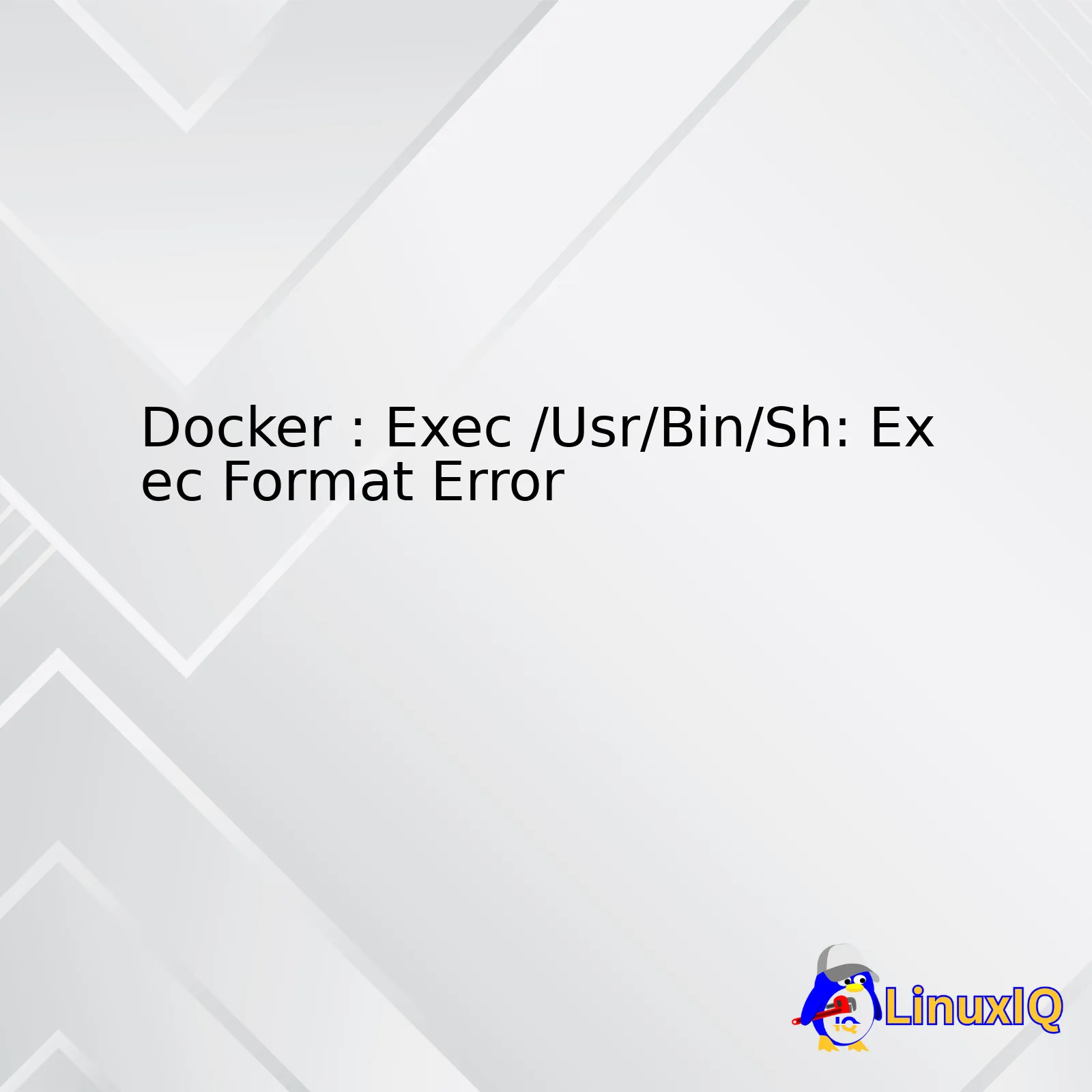
Error | Cause | Resolution |
---|---|---|
Docker: Exec /usr/bin/sh: exec format error | Occurs due to the executable file’s format or the entry point script is not able to be interpreted by Docker. | Specify the correct interpreter in the shebang line of the offending script, rebuild the image after ensuring image details and their compatibility with the platform, or use a base image that corresponds to the architecture required. |
That “exec format error” when you try executing a shell script occurs when your Docker container attempts to interpret its contents but fails. In other words, the script formatting or structure isn’t recognized, leading to the execution failure.
Let’s assume this problem arises because of an incompatible script structure. Your action points should include checking the first line (the shebang line) within your script. That line should essentially look like so:
#!/bin/sh
or
#!/bin/bash
When these shebang lines are arranged correctly, they disclose exactly which interpreter has to be employed to execute the script provided. If you notice something wrong, you can simply alter the shebang line and reboot your container once more.
If this quick fix doesn’t resolve the issue, it’s time for plan B – delving into the Docker build process itself. Check whether there’s something amiss in the way you spin your Docker containers. Perhaps there’s a lack of correspondence between the platform where you are running Docker and the base image you are employing. For instance, if your Docker host is on an ARM-based platform while you’re using an AMD64-based image, conflicts may arise (Docker docs).
Rebuilding your Docker image is often the key to solving these types of issues. The rebuilding process requires that you carefully check the image specifications and ensure they’re fully compatible with the platform wherein they’re supposed to function. Wisely pick a base image that fits well with the Docker host’s architectural requirements. Then, repeat the Docker build process and run the problem script once more to verify if the issue has been successfully addressed.
While working with Docker, a common problem that often crops up is the ‘Exec /Usr/Bin/Sh: Exec Format Error’. This error is mainly encountered when we try to build a docker image for a different platform than our current system architecture. Docker needs to interpret executable binaries in a compatible format with the host machine’s architecture.
The Nature of The Error and It’s Cause
The problem arises due to a “shebang” inconsistency between different operating systems and CPU architectures. “Shebang” are basically the first two bytes of an executable file; they specify the interpreter program for executing the instructions in the file. Our Dockerfile might contain a script that worked on the original system but doesn’t on a different one because shebang line references a location which is different or doesn’t exist in the new system. If a binary is not compiled for your machine’s architecture (like ARM for Raspberry Pi’s, x86_64 for most PCs), and you attempt to execute it, the kernel won’t be able to understand the binary, resulting in the aforementioned error.
Solution to The Problem
The simplest solution could be just to recompile the application on the target system architecture. However, this might not always be feasible or simple.
# Assuming the code you want to deploy is written in golang FROM golang:1.7.3 as builder WORKDIR /go/src/github.com/username/project/ RUN go get ./ RUN CGO_ENABLED=0 GOOS=linux go build -a -installsuffix cgo -o main . FROM alpine:latest RUN apk --no-cache add ca-certificates WORKDIR /root/ COPY --from=builder /go/src/github.com/username/project/main . CMD ["./main"]
The example above shows a dockerfile for a golang project, and compiles the code prior to creating the image, making sure it’ll be compatible with the Linux OS in the docker container. The
CGO_ENABLED=0 GOOS=linux
command ensures that the output executable will work even on alpine linux, despite differences in C library (which golang statically links by default).
You can handle cross-platform building using Docker itself. From Docker version 19.03 onwards, a feature named buildx is available that allows us to build images for multiple architectures simultaneously:
docker buildx create --use # Setup multi-arch builder docker buildx build --platform linux/amd64,linux/arm64 -t image_name:tag . --push
The `–platform` option lets you specify different CPU architectures, allowing Docker to build your image accordingly.
Additionally, verifying the shebangs in your scripts could prevent such issues. Stick to popular and conventional paths such as
/bin/sh
or
/usr/bin/env bash
unless there is a stringent requirement.
Popular Shebang | Description |
---|---|
#!/bin/sh |
This is used where portability is essential. |
#!/usr/bin/env bash |
This is typically used when bash-specific features over sh are required. |
Reference Links
There’s a good deal of aspects to consider when we attempt to shed light on Docker’s architecture and the role of binary executables, especially revolving around the error “Exec /Usr/Bin/Sh: Exec Format Error.”
At its core, Docker’s architecture revolves around the notion of containerization. A Docker container is built around a binary executable, encapsulated within a complete filesystem that also houses system tools and libraries necessary for the binary to run. This ensures that the software consistently runs as intended regardless of its environment.
Docker containers essentially operate via client-server architecture. The Docker client, dealing with commands input by a user, communicates with the Docker daemon which builds, runs, and manages Docker containers. The two elements utilize a REST API over Unix sockets or a network interface.
Below is how the
Dockerfile
starts:
FROM ubuntu CMD ["/bin/echo", "Hello World"]
The importance of binary executables in this light becomes clear. They are the command our Docker container will execute, in this case
/bin/echo
. In essence, a Docker file includes an image (Ubuntu in this case) and a binary located in
/bin/echo
, which the CMD is executing after the image is built.
Now, let’s tackle the unpleasant part – the ‘Exec format error’. One likely cause is that the binary executable at hand is not compatible with the architecture of the host machine running the Docker engine.
For instance, a binary meant for a 32 bit system won’t function well on a 64 bit one. In an ARM-based architecture, the binary compiled for x86 systems doesn’t work out either, consequently leading to issues like the ‘Exec format error’.
Ways to prevent these errors typically involve checking that your Docker architecture aligns with your binary before proceeding:
docker run --rm mplatform/mquery your_image:tag
Also, certain Docker images exist for each kind of architecture out there, such like these:
Processor Architecture | Supported Docker Image |
---|---|
x86-64 | ubuntu |
ARM32v6 | arm32v6/ubuntu |
ARM32v7 | arm32v7/ubuntu |
By taking consistent steps to understand and validate your binary against the target Docker architecture, you can avoid seeing that ‘exec format error’ again.
For further information about Docker’s architecture and debugging such prominent issues, consider reviewing official documentation at the Docker Documentation website, which provides comprehensive insights into the architecture and binary compatibility in Docker containers.Troubleshooting the ‘Exec /usr/bin/sh: Exec format error’ primarily entails comprehending why it arises in a Docker context and potential solutions to address it.
Errors are endemic to the process of software development, and every hardworking coder has likely come across the rigmarole that is ‘error hunting’. Today, we turn our gaze towards one such common errant incident that often plagues Docker users:
Exec format error
. Let’s break this down.
Understanding the Error
The
Exec format error
generally occurs when a program that we’re trying to execute possesses an incompatible binary for the host machine. In simpler terms, an attempt is being made to run an application that does not conform with the architecture of the system. In a Docker environment, this could mean a few things. The most probable scenario would be attempting to run a Docker container image that’s built for an incompatible architecture – for instance, running an ARM-built image on an AMD64 host[1](source).
To understand what’s causing the issue, let’s first look at a sample Dockerfile that results in triggering the error:
FROM debian:latest COPY ./run_my_app /usr/local/bin/run_my_app RUN chmod +x /usr/local/bin/run_my_app ENTRYPOINT [ "/usr/local/bin/run_my_app" ]
Here, we have defined an ENTRYPOINT to a binary executable, i.e., the ‘/usr/local/bin/run_my_app’ that we’ve bundled into the Docker container during the build phase. If this binary’s compiled format doesn’t match your Docker host’s CPU architecture, it leads to the aforementioned exec format error.
Possible Solutions
Below are a few identification methods and remedial measures that can be undertaken to fix this issue:
- Architecture compatibility is key. Ensure to cross-verify if the images you are using are indeed relevant for your Docker host’s CPU architecture. Docker Hub usually provides different tags for distinct architectures. Choose the right one for your system.
- On circumstances where Docker images do not offer variant architecture tags: resort to using an emulator like QEMU (which Docker supports) to enable executing foreign binaries.
- If you’re the one building the Docker image, ensure to use a base image compatible with the target CPU architecture. On Docker Hub, you’ll find numerous base images crafted specifically for different CPU architectures.
- For programmers deploying Go applications in Docker containers, remember to account for the GOOS and GOARCH variables while building your Go interfaces. Docker inherently uses Linux, so you must set the GOOS variable to ‘Linux’ regardless of your host OS.
env GOOS=linux GOARCH=amd64 go build -v my_app.go
This will produce compatible output ensuring a seamless Docker operation.
Identifying the root cause and selecting an appropriate solution can evidently troubleshoot the ‘Exec /usr/bin/sh: Exec format error’.
A Quick Check-Up Tip
A useful way to double-check the binary compatibility is by running the file command on your binary from within the Docker container:
docker run --rm -it your_docker_image file /path/to/your/binary
This emits details about the binary. Look for something like “ELF 64-bit LSB executable, x86-64”. The “x86-64” indicates the binary’s architecture, while the ELF refers to the format of the binary. This information can assist in navigating the troubled ‘exec format error’ waters.
In summary, the cause of ‘Exec /usr/bin/sh: Exec format error’ orbits around architectural mismatch between the binary and the Docker host system. The solutions lie in ensuring compatibility, employing emulation tools if necessary, or targeting correct CPU architectures during application build stages.When working with Docker, you might be occasionally confronted with errors such as “
/usr/bin/sh: exec format error
“. This error primarily refers to a mismatch in binary formats. Essentially, it signifies an attempt to run a binary for a different hardware architecture or a different Operating System(like Linux kernel on Windows).
Root Causes
The leading causes of the “
exec format error
” in Docker usually are:
* Platform Inconsistency: An example is trying to execute Linux docker images on a Windows or Mac system without enabling applicable settings for platform support. Nowadays, Docker desktop provides seamless cross-compilation support which involves emulating your host operating system in the container.
* Architectural Mismatch: Running ARM images on x86 machines or vice versa can cause format errors. For instance, Raspberry Pi employs an ARM architecture. If we build a docker image on this machine and then attempted to use that image on a typical PC (which mostly likely applies an x86 architecture), we would encounter a binary format error.
Solutions
* Enable experimental features: To execute Linux containers on Windows or MacOS:
Execute the command that will enable experimental features.
{ "experimental": true }
You can find these settings in Docker > Settings > Command Line.
* Build multi-architecture images: Docker now supports multi-platform images, which make it possible to pull the appropriate image based on your host’s architecture. To achieve this, you need to use docker buildx: Docker Buildx.
* Emulate a different architecture: Docker can emulate other architectures with Qemu. You would have to register the necessary Qemu binary formats to the kernel.
For cloneable execution, install Qemu User-static Using:
docker run --rm --privileged multiarch/qemu-user-static --reset -p yes
Then, you can start cloneable containers like so:
docker run -d arm64v8/busybox sleep infinity; \ docker exec -it \/bin/sh
Demo
A practical case is running an ARM-based docker image on an x86 machine. Initially, we might experience an
exec format
error:
$ docker run -it arm32v6/alpine sh standard_init_linux.go:211: exec user process caused "exec format error"
After following the emulation solution above, here’s what happens:
$ docker run --rm --privileged multiarch/qemu-user-static --reset -p yes
Running the ARM-based image now succeeds:
$ docker run -it arm32v6/alpine sh
In conclusion, the correct approach relies on understanding your environment and using the right combination of image and architecture. The current Docker technology has significantly simplified cross-environment docker usage, but paying close attention to potential inconsistencies is still crucial.
References:
1. Docker Buildx Docker Buildx.
2. Multiarch/qemu-user-static image Qemu-user-static.Understanding the Docker Container Structure and its relation with the error `exec /usr/bin/sh: exec format error` involves a deep dive into the structure of Docker images and containers.
When creating a Docker image, it follows a specific structure that consists of several layers. Each layer corresponds to an instruction in your dockerfile. The beauty of using Docker comes out clearly here as each change made is only on the layer associated to the directive that modified it or as a new one. In case you need to revise something only on one layer, Docker makes this possible without the need for changing anything else.
Additionally, Docker utilizes a .db file (tag_store.json) to note down meta-information about the image. This includes the checksums of images’ configurations and layers which enable Docker to reconstruct the entire filesystem.
FROM ubuntu:16.04 ADD . /app RUN make /app CMD python /app/app.py
Different Docker Images can have their shell located in different paths depending on the base image used & how it was built. For example: `/bin/sh`, `/bin/bash`, or `/usr/bin/zsh`. You may try `docker run -it imagename sh` or `docker run -it imagename bash` or `docker run -it imagename zsh` based on where it is located.
Now, let’s consider the prevalent error many come across while working with Docker – `exec /usr/bin/sh: exec format error`.
This error typically emerges when the user tries to instantiate another process from within the container but ends up using a non-compatible executable.
$docker run image_name Exec usr/bin/bash: Exec format error
In such context, the biggest culprit can be architecture mismatch. Should you attempt to execute ARM binaries on x86 system or vice versa, you risk running face first into similar error.
Also, unresolved dependencies can contribute to the problem. If you are trying to get an executable running which requires a library not present within the Docker environment, then it may fail to function well.
The key lies in creating a Docker image matching your host system’s architecture. Also, the dependencies should be correctly resolved during the image’s creation phase to avoid any functional glitches later on.
My recommendation : Double-check your Dockerfile to ensure that you are following the prescribed steps for your architecture and all the necessary files and dependencies are included while building your Docker Image.
While these are the solutions generally applicable for the above-mentioned error, the exact solution might vary depending on your individual circumstance. It proves worthwhile to go through the Docker community forums and StackOverflow before deciding on a fix.
Hope the information helps in unpacking the Docker Container structure while handling errors in the right way!Comprehending the inner workings of Docker, specifically around binary files and shell scripts in a Docker image, can be rather complex. I’ll try to give you an analytical and elaborate explanation relevant to the subject. Particularly we will explore the error message “Exec /usr/bin/sh: exec format error”.
To start off, let’s understand binaries and shell scripts within Docker:
FROM ubuntu:latest COPY ./my_script.sh / RUN chmod +x /my_script.sh ENTRYPOINT ["/my_script.sh"]
In this example, the Dockerfile references a hypothetical script “my_script.sh”. The ‘COPY’ command transfers it into the Docker image. ‘RUN chmod +x’ is used to make it executable.
However, when running this kind of setup, some people encounter the issue:
standard_init_linux.go:219: exec user process caused: exec format error
Let’s dive deeper into that. This usually happens due to incorrect assumptions made about the execution environment by the binary or script file, like architecture mismatch(32-bit vs 64-bit), incompatible operating system, or incorrect shebang in scripts. Usually, shebang lines look something like this:
#!/usr/bin/sh
The error “Exec /usr/bin/sh: exec format error” typically indicates that the referenced interpreter in the shebang line does not match with one installed or available in your Docker container image.
Here are some solutions to work around this problem:
Solution 1: Shebang Alignment
Ensuring the first line of your shell script points correctly at the shell interpreter required for the script.
For example, replace #!/bin/bash with #!/usr/bin/env bash if bash is not located under /bin on your Docker image.
Solution 2: Making sure binaries align with Container Architecture
If it’s a binary instead of a script, you might need to ensure that the built binary matches the architecture and OS of the Docker image. If you’re compiling C code, for instance, make sure to do so inside a similar Docker environment to guarantee compatibility.
Solution 3: Using the Correct Line Endings
The script run in your Docker might have been written on a Windows machine using CRLF line endings while Unix systems expect LF. Make sure to convert the line endings to LF before copying the script into Docker.
FROM ubuntu:latest COPY ./my_script.sh / RUN apt-get update && apt-get -qq -y install dos2unix RUN dos2unix ./my_script.sh && apt-get --purge remove -y dos2unix && rm -rf /var/lib/apt/lists/* RUN chmod +x /my_script.sh ENTRYPOINT ["/my_script.sh"]
Solution 4: Directly Running the Script/Command
Finally, Docker does allow interpreting scripts or running binaries directly if the ENTRYPOINT command is passed as a string instead of as a JSON array:
FROM ubuntu:latest COPY ./my_script.sh / RUN chmod +x /my_script.sh ENTRYPOINT "/my_script.sh"
And there you have it, a deep-dive into understanding the nuances of Docker and scripts. By trying the above solutions, it should help eliminate the
'exec /usr/bin/sh: exec format error'
that you may encounter in Docker. For further information, consider browsing through the Docker documentation [source].Addressing the issue of “Docker : Exec /Usr/Bin/Sh: Exec Format Error” means gaining an understanding of common missteps in executing binaries inside Docker containers.
This error commonly occurs when you are trying to execute a binary file that uses a different system architecture than the one your docker container is set up to handle. For instance, if you’re on a 64-bit machine attempting to run 32-bit software without properly setting up Docker, this may result in an error.
Docker’s layered filesystems
Docker uses a layered filesystem where each command in a Dockerfile corresponds to a new layer. While efficient, this model can sometimes cause errors. Suppose you remember a file existing at some point, but can’t access it now. In that case, the file might have been changed or removed in a newer Docker layer.
How Docker handles executable files
It’s essential to know how Docker handles executable files as often missteps occur because of a misunderstanding of this process. Docker executes files based upon their shebangs (i.e., a line at the start of a script that tells the operating system which interpreter to pass this file onto for execution).
For example, suppose you have a Python script marked as executable with a shebang indicating “python3”. In that case, Docker will attempt to call “
/usr/bin/env python3
“, not “
/bin/sh
“, to execute the file.
Here’s what a code sample causing this error might look like:
CMD [“./my-script.sh”]