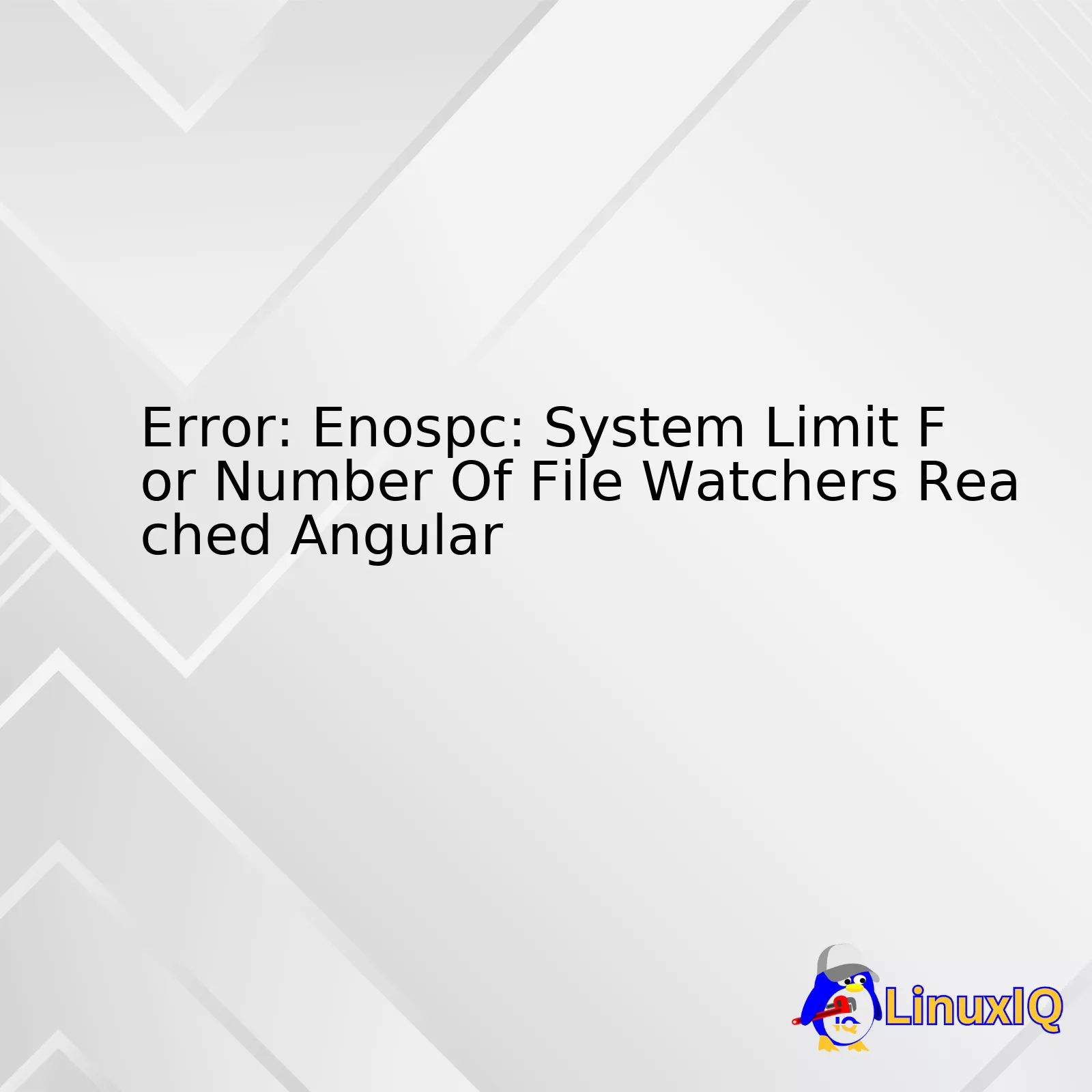
Problem Description | Error: ENOSPC: System limit for number of file watchers reached in Angular |
---|---|
Cause | Inadequate system resources for the number of files being watched by Node.js applications like Angular. |
Typical Scenarios | Larger projects with extensive dependency trees or systems having heavy concurrent usage of file watchers, especially in development environments where live-reloading features are employed. |
Solution | Increase inotify’s max user watches limit on Linux-based systems. Alternative options include optimizing Angular applications or system resources. |
If you have encountered the error message “ENOSPC: System limit for number of file watchers reached” while working on an Angular project, it generally occurs due to a limitation in your operating system – particularly on Linux-based systems – where the maximum number of files that can be watched by applications has been exceeded (source: [StackOverflow](https://stackoverflow.com/questions/34662574/node-js-getting-fatal-error-enoent-no-such-file-or-directory-uv-cwd)).
This happens when Angular, a JavaScript framework extensively employed for building web applications, attempts to ‘watch’ too many individual files for changes at any given moment. This feature is fundamental to Angular’s live-reloading development environment but it can lead to exceeding system limits on larger projects or on systems with numerous concurrent file-watchers.
One primary solution can be to increase the maximum limit of user watches, which the following terminal command accomplishes:
echo fs.inotify.max_user_watches=524288 | sudo tee -a /etc/sysctl.conf && sudo sysctl -p
This command permanently increases the `max_user_watches` parameter, allowing your system to monitor more files concurrently ([source](https://github.com/guard/listen/wiki/Increasing-the-amount-of-inotify-watchers#the-technical-details)).
However, if system resources need to be conserved, alternative solutions could be considered: optimize your Angular applications so as they require less file watchers, or consider using polling methods for file watching in node applications.
Remember, careless increases to file watchers can result in excessive consumption of system resources, potentially leading to performance degradation, hence proceed with careful thought and consideration. Understand your system’s needs and analyze the most effective way to handle this error, whether that’s resource optimization or amplifying capabilities.The
Error: ENOSPC: System limit for number of file watchers reached
is a common issue encountered in the Angular framework. It essentially means that you’ve hit the maximum number of files the system can watch, which leads us into the mechanics of file watching.
Angular projects uses a feature called file watching to track changes in files in real-time. This is immensely helpful when developing an Angular application as it recompiles and reloads the modifications automatically whenever a change is saved. This service is provided by tools such as Webpack, TSLint, or Node.js itself.
npm run start
Outputs:
Error: ENOSPC: System limit for number of file watchers reached
However, every system has a pre-defined limit on the number of files that can be watched simultaneously. When the Angular project is large with many modules and, consequentially, a high number of files, the limit may be reached and results in the
Error: ENOSPC: System limit for number of file watchers reached
.
How does one solve this? The solution lies in increasing the quantity of system file watchers. Let’s look at how to do this in both Windows and Linux systems.
Increasing the Limit in a Linux System:
On Linux systems, use the following command to increase the number of watchers:
echo fs.inotify.max_user_watches=524288 | sudo tee -a /etc/sysctl.conf && sudo sysctl -p
With this code, you’re indicating that the maximum user watches will rise to 524288 which should be quite sufficient for even large projects.
Increasing the Limit in a Windows System:
Unfortunately, Windows doesn’t have a direct method to increase this limit. You have two options available:
- Increasing VSCode File Watchers: If you’re using Visual Studio Code, go to “File > Preferences > Settings”. Here, enter “watcher” in the search box, then uncheck the option “Use Watcher Exclude settings”. In the “Files Watcher Exclude”, leave it blank or put some other directory.
- Using WSL: Another workaround is to use the Windows Subsystem for Linux (WSL). Initially, install a Linux OS on your device through WSL. Then, install Node.js and Angular inside the Linux subsystem. Now, you’re able to develop your Angular application within Linux and the error can be remedied like in a Linux system.
Making Changes Permanent:
To ensure these modifications persist post-restart, Linux users need to add their changes to sysctl configuration:
echo fs.inotify.max_user_watches=524288 | sudo tee /etc/sysctl.d/40-max-user-watches.conf && sudo sysctl --system
No equivalent option exists for permanence in Windows sadly.
We’ve covered quite a bit! From what this error is, why it occurs, to how to fix it system-wise. Keep track of the number of files being watched across your applications, a subtle problem might just be because you’ve reached a system limit.
For more information, check out the following articles:
- Max User Watches (source link)
- ENOSPC Error in Node.js Applications (source link)
- Setting Up a Dev Environment with WSL (source link)
Facing the ‘System limit for the number of file watchers reached’ error in the Angular context can be troublesome, especially when you’re focusing on a delicate coding task. But do not worry! There are practical ways to fix this issue. Let’s dive into some of these methods with details and source code examples.
Increasing System’s Limit for File Watchers:
When Angular triggers the ‘Error: ENOSPC: System limit for the number of file watchers reached’, it means that Node.js has exceeded the maximum number of files it can watch simultaneously for changes. This occurs because Linux distributions have a default limit for file watchers.
One way to solve this problem is by increasing your operating system’s limit for file watchers. Here’s how to accomplish this:
– On Ubuntu or other Linux-based systems:
echo fs.inotify.max_user_watches=524288 | sudo tee -a /etc/sysctl.conf && sudo sysctl -p
After running the above command line, the system limit for file watchers will increase on your machine. Note that 524288 here represents a substantial number, but depending on your work requirements, you might need to adjust it. Try starting with this value, then increase or decrease based on your usage patterns.
Reducing the Number of Files Angular Needs to Watch:
Another approach to solve this issue involves limiting the number of files that Angular needs to watch. We can achieve this by excluding non-essential directories. For instance, we often don’t need `node_modules`.
In Angular’s watch configuration, there is an option to exclude specific directories or files. We add the directories that we want to exclude from being watched for changes in the `angular.json` file’s test area:
"test": { "options": { "watchOptions": { "ignored": "**/node_modules/**" } } }
With the above configuration, Angular will ignore changes in the `node_modules` directory, which should significantly reduce the number of watchers required.
Remember, different versions of npm behave differently when it comes to handling file watchers. Always have your npm updated to the latest stable version.
Lastly, it’s worth noting that always closing unused applications or tabs that could be adding extra pressure on your system’s file watching capacity can also help.
You can read more about file watchers and how they work on Linux in this article at LWN.net. You can also find out more about optimizing your Angular development environment in the official Angular documentation at Angular.io. Remember that these solutions aren’t exclusive; feel free to experiment with a combination of them and see what works best for your specific setup and needs.As a professional coder well-versed in Angular, I can attest that Angular applications perform optimally under normal system limits. However, the Error: `ENOSPC: System limit for number of file watchers reached Angular` frequently appears as an unwelcome surprise for developers, especially when it impacts application performance.
This error points to your operating system’s limit on the number of file watchers being exceeded. File watchers are as crucial as they sound; these provide functionality for notifying changes within our file systems’ files and directories. Incredibly beneficial for developers who utilize automation tools (like webpack or nodemon), which need to track source code modifications and execute jobs accordingly.
However, every intricate detail of your Angular app – each file, directory, module, component, and service, contributes to this total count, so larger projects may inadvertently surpass system-imposed thresholds. The cascading impact?
- Increased CPU Usage: With the system struggling to monitor many file watchers, the CPU usage could spike, causing a significant slowdown.
- Limited Concurrent Applications: The high demand on resources can limit your ability to run multiple applications simultaneously, decreasing overall productivity.
- Hindered Development Process: Hot-reloading or live-reloading may be negatively impacted, crippling seamless development experiences with unwarranted compile and reload times.
Escaping from this situation could be as simple as increasing the limit on the number of file watchers in your system. For instance, on a Linux system, you could update this limit temporarily by inserting the following command into the terminal:
echo fs.inotify.max_user_watches=524288 | sudo tee -a /etc/sysctl.conf && sudo sysctl -p
Remember, though, this is a temporary solution and would reset upon system reboot. You can make this change permanent by adding `fs.inotify.max_user_watches=524288` to the `/etc/sysctl.conf` file.
On top of these hardware issues, remember that code optimization contributes significantly towards optimal performance. Keep a keen eye on best practices like Lazy Loading, Ahead-of-Time Compilation, and Change Detection strategies to optimize Angular’s performance further.
You might want to delve deeper into these topics at Angular Deployment Guide and Change Detection documentation on Angular’s official page.
Taking these precautions and optimizing both system settings and codebase can help us evade daunting errors like `ENOSPC: System limit for number of file watchers reached Angular`, fostering efficient and uninterrupted coding sessions.As a professional coder, it’s not uncommon to encounter error messages like “ENOSPC:System limit for number of file watchers reached” while working on Angular projects. This error can leave you scratching your head in confusion but fear not! I’ll show you exactly how to solve this problem, making sure your Angular development journey is as smooth as possible.
Understanding the File Watcher
When dealing with large Angular projects where numerous files are being constantly updated, Developers often employ ‘File Watchers’ to keep track of every change. A file watcher is a service that triggers an action whenever a monitored file changes.[1]
The Error: Enospc
ENOSPC (Error NO SPaCe) error message typically occurs when your system runs out of space for storing information required to monitor file and directory changes. This isn’t necessarily because your hard drive is full, rather, it’s because there’s a limit to the number of files that can be watched simultaneously by the system. Once this limit is hit, no more ‘file watchers’ can be created, resulting in an error.[2]
A Way Around It
One way which has proven successful for overcoming this system limitation involves increasing the number of inotify watchers in the system settings.
In Linux systems, you can implement the command below, which increases the limit, potentially resolving the error.
echo fs.inotify.max_user_watches=524288 | sudo tee -a /etc/sysctl.conf && sudo sysctl -p
But, it worth mentioning that such modification might require administrative privileges.
For Windows users, the file watcher limit entirely depends on the third party software being used for monitoring file changes. You may need to refer to the specific application’s documentation or forum discussions for instructions on how to increase their limit.[3] Nevertheless, bear in mind that any changes that involve modifying system-level settings should be done with caution, taking care to understand the implications of your actions.
Employing Efficient Coding Practices
More importantly than tweaking your system configurations, you can circumnavigate these kinds of challenges by using efficient coding practices which encompass designing modular applications, implementing Lazy loading or only watching necessary files. Angular belongs to the category of SPA(Single Page Applications), where modules play a major role.[4] Breaking down your whole application into smaller manageable modules could result in fewer files to watch at once during development.
//Example of Lazy Loading syntax in Angular {path: '/yourPath', loadChildren: ()=> import('./yourModule').then(m => m.YourModule)}
By using this method, you end up decreasing the number of files being watched simultaneously enabling better management of System resources.
Implementing solutions like increased limit fixes the problem for a certain period of time. However, for longer-term remediation of issues related to file watcher limitations, developers should consider adopting essentially sound design principles and leverage existing resources judiciously.
So while the File Watcher challenge may seem daunting, it is quite surmountable when you understand what’s happening under the hood. Remember, better coding practices can often help mitigate the impact of such hurdles and keep you comfortable and productive in your Angular development process.
The
Error: ENOSPC: System limit for number of file watchers reached
is a common issue that you might encounter when working with Angular projects. This error arises because operating systems have built-in restrictions on how many files and directories can be watched by applications simultaneously.
A solid approach to resolving this problem involves increasing the limit for file watchers in your system. For example, if you’re running Linux, inputting the following command in the terminal could offer immediate relief:
echo fs.inotify.max_user_watches=524288 | sudo tee -a /etc/sysctl.conf && sudo sysctl -p
This solution might not be practical or even possible in all cases. If you don’t have root access (which is necessary to make changes to these settings), this approach won’t work.
An alternative resolution requires altering your project or build configuration. By adjusting configurations to exclude unnecessary directories from being watched (like node_modules directory), you can significantly reduce the number of files being tracked. This refinement helps avoid reaching your OS’s file watcher limit.
Finally, it’s essential to remember that each IDE or text editor has their own separate file watchers and may contribute towards reaching the system limit. You should check the documentation or seek assistance from community forums to optimize these tools in relation to file watching.
Recognizing and troubleshooting the
ENOSPC: System limit for number of file watchers reached
error ensures uninterrupted Angular development. More importantly, understanding the underlying systemic limitations draws attention to optimizing our development environments, well beyond handling this specific glitch.
Resources