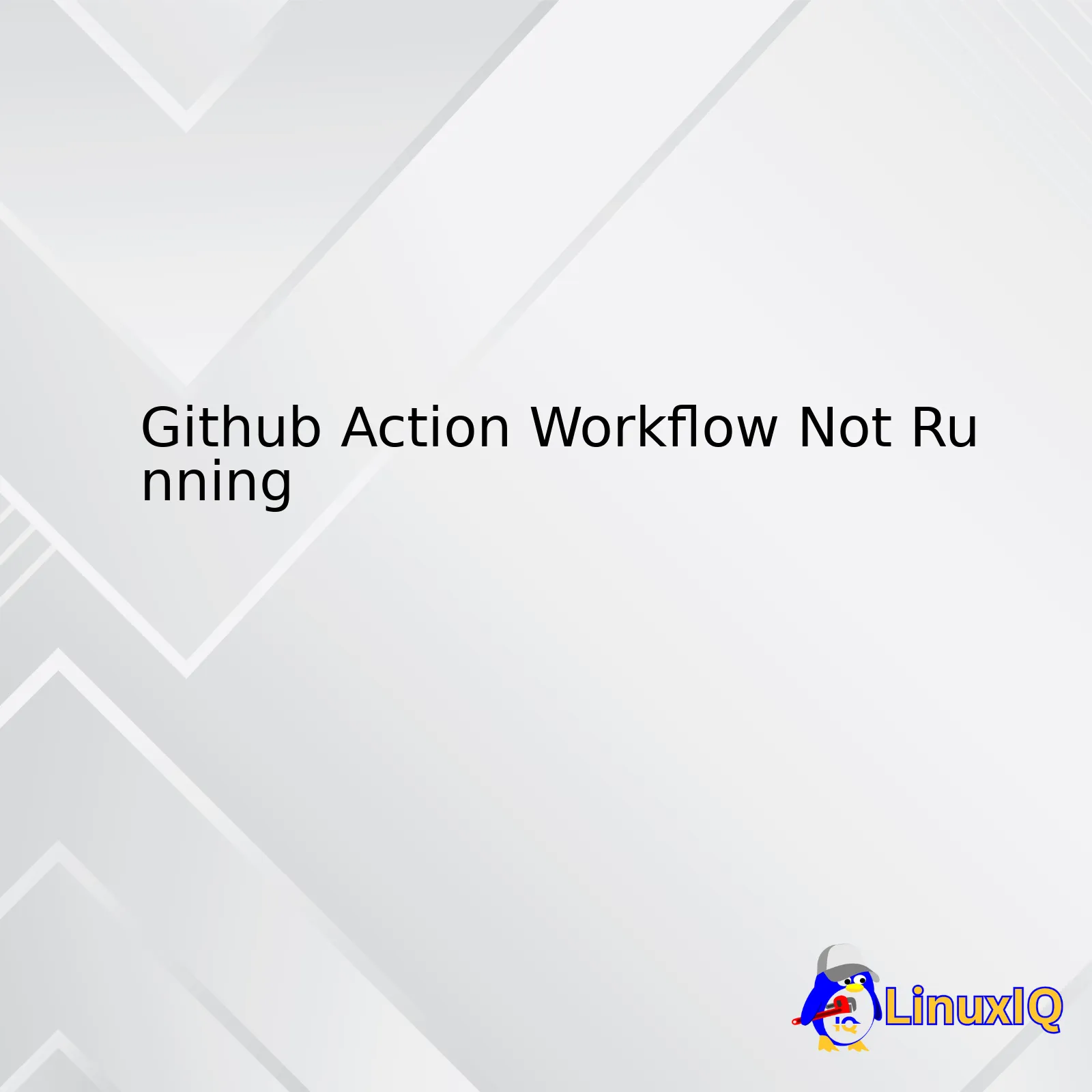
Let’s start with a summarizing table that explains the common reasons and solutions to why GitHub Action Workflow is not running:
Problem | Solution |
---|---|
Workflows are disabled in the repository settings | Navigate to ‘settings’ > ‘actions’. Enable Github Actions if it isn’t already. |
The workflow file has syntax errors | Use a YAML validator like YAMLLint which can help you spot syntax errors on your workflow files. |
Event triggers aren’t formatted properly | Ensure every event name, type etc. matches exactly as given in the GitHub’s official documentation. |
Workflow is on a branch that doesn’t exist | If your workflow is set to trigger on a push or pull_request for a specific branch that does not exist, GitHub Actions will not run. Ensure branches specified do exist. |
Pushes made by Github Apps, Bots etc. | Github does not run workflows for pushes from applications or bots, including Dependabot, thus this be might be stopping your workflow from running. |
The secrets used in the workflow are not available | Check the repository’s Secrets section. If they don’t exist or accessible, add them. The absence of necessary secrets could prevent the initiation of workflow runs. |
GitHub Actions enable us to automate, customize and execute our software development workflows within the GitHub environment. It’s irrefutable how important it is to have these actions perform flawlessly and without interruption.
However, despite its sophistication, there are instances where the GitHub Actions Workflow may not run as expected. The reasons can range from simple syntax errors within the workflow file, improperly formatted event triggers, non-existent branches, to problems related to repository settings, tokens, or secrets. Understanding these issues is the first step towards implementing a reliable solution in order to ensure seamless functioning of your GitHub Actions Workflow.
When dealing with a misbehaving GitHub Actions Workflow, we shouldn’t panic. With careful debugging and interface interaction, we can unearth the causes behind its failure to execute. The solution could be as easy as enabling the GitHub Actions under the repository settings, or perhaps more complex such requiring a revision of the code syntax to eradicate potential errors.
Reference sources:
Enabling a Workflow,
Using the GITHUB_TOKEN in a workflow.As part of your progression in coding, I am sure you have encountered or will eventually bump into GitHub Actions. They are extremely versatile tools that allow us to automate various aspects of our software development workflows within the GitHub ecosystem. However, it can be quite frustrating when the GitHub Action workflows fail to work as expected.
To understand why they might not run, let’s first dive into some core concepts of Github Actions1.
Workflow Files: All action definitions are in YAML files which are placed in the
.github/workflows/
directory in your repository. Each YAML file represents one workflow and holds information about when this workflow should be triggered and what jobs need to be executed.
Events: Events are occurrences that trigger the execution of workflows. Typically, these events correspond to a particular activity like pushing code to a branch, a schedule (cron job), manually triggering, among other possibilities. You define these in the
on:
section of your YAML file.
Jobs: Jobs are sets of instructions that are executed sequentially. Essentially, they do the bulk of the work. Every workflow consists of one or more jobs defined in the
jobs:
section of your yaml file.
Steps: Steps are individual tasks within a job. A step could involve running a shell command or executing an action. All steps within a job share the same runner, hence share data, such as cloning a repository.
Runners: Runners are servers where the job execution takes place. You either use runners provided by GitHub or host your own runners.
Actions: Actions are either made up of Docker containers or JavaScript codes which are reusable units you incorporate within your jobs.2
Now, coming back to why your GitHub workflow might not be running:
Event Triggers: Check if the event intended to trigger the workflow has indeed occurred. If the workflow is tied to the push event and there hasn’t been any new push, the workflow won’t start.
Syntax Errors: YAML syntax errors or incorrect usage of GitHub Actions’ domain-specific language (DSL) could also prevent workflows from running.
Faulty Conditions: Evaluate the conditions for job or step execution in your YAML file. If the
if:
condition does not meet, the job or step will not run.
Repository Settings: Rules set up at the repo level might prohibit Actions from running. Navigate the settings in your repository to verify this fact.
Here’s an example of basic GitHub Workflow:
name: Basic Workflow on: [push] jobs: print-greeting: runs-on: ubuntu-latest steps: - name: Print greeting run: echo "Hello, world!"
Always remember, debugging is key in coding, whether it’s an application code or setting up a CI/CD pipeline with the help of GitHub Actions. Happy Coding!
HyperLinks:
1 Official Github Actions documentation
2 About actionsIf your GitHub Action workflow isn’t running, there could be several probable reasons.
The
GITHUB_TOKEN
might not have the appropriate permissions to trigger workflows. This scenario usually occurs when an attempt is made to create a workflow run from actions like creating issues or assigning labels and collaborators from forked repositories. The
GITHUB_TOKEN
of forked repositories has read-only permissions and can only write to a few chosen API endpoints.
Below is an example of how you would use
GITHUB_TOKEN
in a workflow:
name: CI on: push: branches: - master pull_request: branches: - master jobs: build: runs-on: ubuntu-latest steps: - name: Check out code uses: actions/checkout@v2 - name: Set up Python uses: actions/setup-python@v1 with: python-version: '3.x' - name: Install dependencies run: | python -m pip install --upgrade pip pip install -r requirements.txt # Assuming that Python is being used for development. - name: Run tests run: | python manage.py test env: GITHUB_TOKEN: ${{ secrets.GITHUB_TOKEN }}
Another possibility is that there is an error in the syntax of the .github/workflows/main.yml file which prevents it from being picked up by GitHub Actions. Always cross-check to ensure the correctness of syntax and structure of your workflow yml files.
Whereas, an incorrect configuration under the
on:
key in your workflow file can result in GitHub not knowing the event on which to trigger the workflow. Remember, the workflow will only run when the events mentioned under the
on:
key have occurred in the repository. Be sure to specify events accurately. You can refer here for more information on how to configure triggering events correctly.
To guard against syntax errors in your workflow files, employ YAML linters to ensure the syntactic integrity of your files and correct problems as early as possible. They will help you in diagnosing any potential YAML syntax errors before they cause your workflows to fail. One such online linter tool can be found here.
Remember also to check the restriction settings at both repository and organizational levels. Any restrictions can prevent Actions from running. To allow actions and third part actions, go to your Github organization’s settings > Actions > Policies, and enable them.
Sometimes, GitHub Actions service may suffer interruptions leading to failure in workflows’ execution. Checking the “GitHub Actions Status” page here can provide insights into whether any outages or performance issues are affecting the functionalities of GitHub Actions.
In summary, start your troubleshooting process by:
- Checking your code references for correctness.
- Ensuring your YML syntax is correct by employing a linting tool.
- Determining if your
GITHUB_TOKEN
is equipped with adequate permissions.
- Verifying your configured events under the
on:
key.
- Reviewing your restrictions dashboard within your repository and organizational settings.
- Finally, confirming there aren’t any broader issues with GitHub Actions service itself.
Any documentation or hyperlinks referenced here should serve as useful starting points for diving deeper into various concepts and solutions. In order to optimize your response to a maligned action, understanding thoroughly why your GitHub action workflow is not working leads to faster resolution times and smoother workflow operations moving forward.Sure. GitHub Actions Workflows are incredibly powerful and flexible tools, but as with any complex tool, they can at times pose challenges or behave unexpectedly.
One common issue with GitHub Actions Workflows is that your workflow may not run as expected – or perhaps, not at all. Understanding why this might happen is quite crucial, so let’s dive in.
Workflow Syntax Errors
Incorrect syntax is a common cause of this problem. If your YAML file has any syntax errors, the GitHub Action Workflow will refuse to run.
To correct this, you can use a tool like YAMLLint to validate your YAML syntax.
You can fix the errors by using this sample YAML action:
name: CI on: push: branches: - 'main' pull_request: branches: - 'main' jobs: build: runs-on: ubuntu-latest steps: - uses: actions/checkout@v2
GitHub Secrets Access
GitHub secrets are encrypted and only exposed to selected GitHub Actions. Some workflows fail because they attempt to access secrets they do not have permission to see. The problem can be rectified by ensuring your secrets are available to your workflows.
Base Branch Does Not Contain Workflow File
GitHub Actions require the workflow file (located in .github/workflows) to exist on the base branch of the repository for the action to trigger. Specifically, GitHub Actions does not run workflows in forked repositories by default.
Incorrect Triggers
‘On:’ section of your workflow file dictates when your workflow should run. If this section isn’t defined correctly, your workflow may not run as expected. For instance, if your workflow should run on both ‘push’ and ‘pull_request’ events, your ‘on:’ section should look like this:
on: [push, pull_request]
Now that we’ve explored some possible root causes, it’s evident that thorough understanding of GitHub Actions syntax and expected behavior is crucial in troubleshooting. You can also leverage the official GitHub Actions documentation as a reference guide.
GitHub Actions offer flexible and powerful automation capabilities that can empower developers to robustly manage their work processes. The role of a YAML file in GitHub Actions is particularly important as it helps define the workflows for your applications. For those not sure what YAML stands for, it’s ‘Yet Another Markup Language’, but YAML files aren’t just another markup language, they are at the heart of defining and orchestrating your GitHub workflows.
Let’s delve into this further:
YAML Files – The Key to Orchestration in Github Actions
name: Example Workflow on: push: branches: [ main ] jobs: build: runs-on: ubuntu-latest steps: - name: Checkout code uses: actions/checkout@v2
The above YAML file represents an example of the simplest GitHub action. As you see, the workflow script is held within a YAML file which has a .yml extension and stored in the ‘.github/workflows’ directory of your repository. This YAML file specifies:
- The event(s) that will trigger the workflow (in this case, any push on the
main
branch).
- The jobs that would be run (a single job named ‘build’ here).
- The host environment for each job (‘ubuntu-latest’ OS for the ‘build’ job).
- The steps that each job comprises of (just one step for checkout).
- The actions that each step employs (utilizing ‘actions/checkout@v2’ to carry out the checkout operation).
Anomalies Leading To GitHub Action Workflow Not Running
If you find that your GitHub Action workflow is not running, there could be several reasons why this might occur, all based around the configuration within the YAML file including:
- In many instances, the issue might originate from incorrect naming or placement of the YAML file. It’s important to note that the filename of the action has to be unique for its corresponding repository, and the file must live in the correct ‘.github/workflows’ directory of your repo.
- Errors relating to the syntax or structure of the YAML file could hinder the execution of the action. Areas where these issues typically surface include: inappropriate indentation, lack of proper spacing, misuse of specific keywords, etc. Remember, YAML files are extremely sensitive to formatting and even mistakes as minute as leaving an extra space could lead to errors.
- If the events specified in the ‘on’ field do not get triggered as desired, the associated jobs won’t get initiated. So perhaps the problem lies with events not firing off due to wrong spelling or incorrect use.
Resolving such problems normally entails revising your YAML file and ensuring it aligns with the best practices as laid out in GitHub’s documentation.
Elevating Applications With Right Workflow Configurations
When correctly written, the YAML file can act as a useful tool in taking your GitHub projects to greater heights. It enables running tests concurrently, executing commands after a certain condition has been met, deploying to different environments based on the success of components, among other things.
So, while writing your YAML files for GitHub Actions workflows, be mindful about the configuration and follow the guidelines meticulously. Afterall, every little thing coded in the YAML matters when it comes to the perfect execution of your GitHub Action workflows.
When working with a Github repository, settings can have a massive influence on the workflow, including Github Action Workflows. If your Github Action Workflow is not running as expected, this may due to specific configurations in the repository settings that might be limiting its performance. Let’s dig deeper into several potential reasons.
1. Actions Permissions Settings
The first place you should check if a Github Action Workflow is not running is the GitHub repository’s “Settings” tab. In the “Actions” section of the settings panel, there is an option for “Actions permissions”. This setting dictates who and what can execute actions.
It’s possible that if your Actions are not running, they’ve been disallowed in your repository’s settings. Here’s an example of how to set Actions permissions:
Go to 'Settings' -> 'Actions' -> 'Actions permissions' Select 'Enable local and third party Actions for this repository'
This grants access to Github Actions, but remember always follow the principle of least privilege when dealing with permissions.
2. Check the ‘Workflow’ Setting
Another point of interest would be the “Workflow” tab in the “Actions” section of the repository’s settings. If workflows aren’t enabled, the Github Action Workflow will indeed not run. Thus, you need to make sure they are turned on.
Here’s the process of checking whether Github Workflow is enabled:
Go to 'Settings' -> 'Actions' -> 'Workflows' Make sure 'Allow all actions' or 'Allow select actions' options are enabled
3. Inspect your .github/workflows/ file
Remember that Github Action Workflows are controlled by YAML files located in the
.github/workflows/
directory in your repository. Even minor syntactical mistakes in these files could cause the whole workflow not to run.
Make sure no invisible syntax errors present— these can include extra spaces, misplaced colons, invalid indentation, etc., all of which could disrupt the actions.
For more information about creating a custom action, take a look at the GitHub documentation.
4. Confirm your Github Actions Availability Based on Your Account Type
Github Actions availability can depend on the account type (public vs private). A higher plan will allow more actions on private repositories, while public repositories usually have unlimited access unless the usage has been deemed abusive. Validate this here.
Finally, don’t forget every commit to your default branch can trigger a new workflow. Try pushing a small ‘test’ commit like updating a README.md file to see if it kicks off your action.
Each change to the repository configuration significantly impacts the workflow execution and failure to adhere to such configurations might hinder the Github Actions Workflow from running properly.
GitHub Actions is a great way to automate various protocols in your programming workflows. One of the most common issues faced with Github actions, particularly by newbies, is the workflow not running as expected. Here are some well thought out methods to debug why a GitHub Action Workflow may not be functioning properly.
Firstly, it’s worth noting that understanding the structure of a GitHub Action workflow file is very crucial. It helps enable efficient decoding of errors or anomalies when things don’t go as planned. Analyzed below are a few key points in debugging this issue:
1. Double check your WorkFlow File:
First and foremost, ensure your workflow file (.yml file) is in a correct location (i.e., it must be inside .github/workflows directory). Also, any syntax errors in this file might block the workflow from running. Use an online yaml validator (YAMLValidator) to verify its syntax correctness.
name: My First Workflow on: push: branches: [ main ] jobs: build: runs-on: ubuntu-latest
2. Review GitHub Secret Keys:
Ensure that you’ve used the right secret if your application requires them. If the wrong secret is used, the action will fail to run. You can recheck these secrets in settings/secrets tab on your repository dashboard.
3. Check the Event Triggers:
Make sure the event you want to trigger the action is sorted in the “on” fields in the workflow script. Common events are push, pull request, but there are many other more specific ones like label creation or issue comments. Make sure that the event is specified correctly or the action won’t run.
4. Debugging with logs:
Review the logs of your workflow. They can provide in-depth insight into what’s happening behind the scenes when the workflow is being run. In the logs, you can see each step executed by the action and how long it took, as well as logs set up by the developers of any steps that failed. You can access logs via the `Actions` tab in your repository.
5. Making use of Third-Party Actions:
Consider making use of third-party actions from the GitHub marketplace, they often have more options for outputting troubleshooting information. For instance, the “Checkout” Action allows you to set the fetch-depth parameter to 0 to get detailed logs of the git checkout.
Remember, frequent synchronization with the master branch or the base branch you’re working from will address potential conflicts proactively.
With these debugging procedures, exploring and fixing the reason behind GitHub Action Workflows not running can be systematically done. Happy coding!
For more in-depth knowledge, refer to the official documentation of Github Actions here. When it comes to GitHub Actions workflow execution, there are a few basic concepts that are crucial in understanding why your workflow may not be running.
The steps involved in event trigger execution process:
GitHub Actions operates via a system of events and workflows. When specific activity occurs within your GitHub repository, this triggers an ‘event’. These ‘events’ include things such as:
– A push made to the repository
– An issue or pull request being opened
– The release of a new version
Each event can trigger a workflow – essentially a set of predefined automation instructions you have defined for the repository. Your GitHub Actions configuration file (
.github/workflows/name-of-your-workflow.yml
) sets out these workflows specified instructions designed to take place upon activation by an event, including tasks like building applications, testing code, or deploying software.
A case where GitHub Action Workflow May Not Run:
A common scenario where your GitHub Actions workflows may not run involves the configure activity types list in the
yaml
file. For instance, even though you have set a
push
event trigger, your workflow might not be activated if the changes are pushed to a branch that’s not included in the config file for this action.
Let’s assume this case:
name: CI
on:
push:
branches: [ master ]
jobs:
...
This has been configured to only run when there’s a push event on the master branch. If a developer makes a push event on a different branch say, ‘dev’, the workflow won’t be triggered.
Solutions:
Here is what you can do to troubleshoot and resolve issues with GitHub Actions workflows not running after an event trigger:
– First, check that the YAML syntax in your GitHub Actions workflow file is valid. Misconfigured files will not correctly trigger actions. Use online YAML validating tools to ensure correctness.
– Check the type of event that triggers the workflow. Ensure that the appropriate events (‘push’, ‘pull_request’) are listed for the configured path and any changes made correspond with this path.
– Cross-examine access permissions. Issues concerning organization-owned repositories and user account permissions could prevent workflow executions. Make sure the necessary parties have the correct permissions.
– Also, check whether GitHub Actions’ service is having any ongoing outages by visiting the GitHub Status website.
By carefully observing these considerations and possible solutions, you should overcome obstacles preventing your GitHub Actions workflows from running successfully!As a professional coder, I am often faced with the challenge of troubleshooting and deciphering error messages from failed workflows. When it comes to Github Action Workflows, their failure to run can be quite perplexing and frustrating since they are pivotal for enabling automated software developments in Github projects.
So, how does one go about scrutinizing these mystery-laden error messages? Let us delve into this in detail:
Error Messages from Github Action Workflow
Github provides an elaborate workflow log every time a Github action runs. If it fails to run, identifying the reasons usually begins with a rigorous examination of these logs.
Accessing the Error Logs:
You can simply navigate to the Actions tab within your Github repository to find the logs. From there on, identify the failed workflow run and open it to inspect the detailed logs.
#Visual representation of path to logs Repository > Actions > Click on workflow run > Check Job log
The error logs will show you precisely where the execution of the task was halted and why. The problem could range from syntax errors to dependency failures or lack of necessary permissions.
Scrutinizing Common Errors:
Let’s decipher a couple of frequent error messages we might encounter in the context of Github Action Workflows not running.
– “This check failed”: This is a widespread error message. It means that Github Actions has run as expected; however, the defined jobs inside the workflow did not complete successfully.
Here is a hypothetical example for better understanding:
#workflow job name: Failed job due to test run: | npm install npm run test #npm run test will fail if your test cases fail.
– “No runner matching the specified labels was found: ubuntu-latest.”: This error typically occurs when you have mistakenly written a non-existent runner environment in your code.
Below is an example showing incorrect usage and the correct way to do it:
Incorrect:
runs-on: ubunt-latest
Correct:
runs-on: ubuntu-latest
Error messages, when decoded properly, act as a map guiding you to the exact cause of the issue. While we’ve only touched upon a handful here, virtually every issue causing Github Action Workflows to falter throws an error message that can be scrutinized similarly.
For further insight on understanding Github error messages, looking up their official documentation serves as an excellent starting point.
More so, forums like StackOverflow present a plethora of real-life queries tackled by coders worldwide. You can quickly skim through or even post your unique issues there if stumped.
Bear in mind, these error dialogues were designed to assist, not mystify us. Therefore, whenever encountering an error, take the time to read the message meticulously. Most answers lie right there!Detangling the intricacies of GitHub Actions can be a daunting task, but when done correctly, it is an exceptionally powerful and efficient tool for CI/CD. Understanding how to incorporate proper exception handling mechanisms into your workflow becomes crucial in debugging issues related to the GitHub Actions Workflow not running as expected.
There are several reasons why this might happen:
• Misconfiguration in action workflows: These can result in no response despite multiple tries.
• Syntax errors in .yml files: Incorrect styling syntax or indentation in the YAML file is another common reason.
• Resources exhaustion: This could occur if the maximum number of concurrent runs allowed by GitHub has been reached.
Let’s deep dive into the simple techniques you can deploy to pinpoint the problems and resolve them:
A. Use Debugging Logs to Capture Exception Details:
GitHub uses debug logs for troubleshooting purposes. You can make your workflow print debug messages by setting up a secret called
ACTIONS_RUNNER_DEBUG
to
true
. Here’s how to do that:
1. Navigate to the main page of the repository
2. Click on ‘Settings’ and then ‘Secrets’
3. Click ‘Add a new secret’ button
4. Name it as ‘ACTIONS_RUNNER_DEBUG’ and set its value to ‘true’
In addition to the debug log, GitHub provides step debug log. You can enable it by setting another secret
ACTIONS_STEP_DEBUG
to
true
.
B. Properly Utilize Virtual Environment’s Logging Capabilities:
Remember, all the workflow runs are executed in a virtual environment. Leveraging the logging capabilities of these environments can help you track exceptions and handle them appropriately. For instance, if commands execution failure causes a halt in workflow run, examining the logs can often provide insight into what went wrong.
C. Set Up Automated Notifications:
Automated notifications reduce human detection time for any particular exception. GitHub provides several events which you can use to set up automated notifications. Configure your GitHub Actions to send notifications when:
• A workflow run fails
• There are changes to workflow files
D. Consider Setting up Self-Hosted Runner:
If your GitHub action workflow is not running due to a shortage of free GitHub Runner usage (allotted as per your account), consider setting up a self-hosted runner. It may seem like a big task, but it’s worth the effort considering the extended control it provides.
Here’s example code for deciding whether to use a self-hosted runner or a GitHub-run runner depending on the availability:
jobs: job1: runs-on: ${{ startsWith(runner.os, ‘Windows') && 'self-hosted' || 'ubuntu-latest' }} steps: - …
The above code basically checks the OS type of the current runner and assigns the runner based on that.
To employ proactive and effective exception detection and handling techniques is paramount to enhancing your GitHub Actions efficiency. To learn more about GitHub Actions, their exceptional documentation and community support is a great place to start (GitHub Actions Documentation). Remember, improvement is iterative, and understanding the most common source of exceptions is the first step towards achieving optimal GitHub Action workflow performance!One of the fundamental complexities experienced by coders during the development process is having their GitHub Action workflow not running in response to push events. Push events are supposed to initiate a workflow run, and when that doesn’t happen, it breaks the seamless integration & automation process that GitHub Actions promises to provide.
Often, the crux of this problem can be tracked down to a couple of common causes such as misconfigured workflow files, incorrect branch names in the workflow configuration file or issues with access rights and permissions.
on: push: branches: - main
The YAML code snippet above demonstrates how events (like push) on certain branches should trigger a workflow in GitHub Actions using the correct syntax. However, if your own action is not triggering as expected, there’s an underlying bug that needs to be diagnosed and fixed.
Below are some of the prevalent reasons for a failed workflow initiation:
Workflow file misconfiguration:
Check the structure of your YAML workflow file. A minor typo, wrong indentation, or an unrecognized command field can prevent the workflow from getting started.
Incorrect branch names:
If you’re restricting workflow runs to specific branches, make sure you’ve used the correct branch names. Context switches can commonly lead to developers coding under the assumption that they’re working on the ‘main’ branch, while in reality they might be located at a ‘feature’ or ‘bug-fix’ branch.
No commit changes:
Sometimes, push events won’t initiate the workflow run if there are no new commits added to the branch, meaning you’ve just pushed what was already in the history.
Access rights and permissions:
Lack of permissions or incorrect authorization may also be the cause of your trouble. Make sure the user whose token you are using actually has access to trigger workflows.
These insights should certainly get you closer to solving your problem. If however, you find yourself still stuck somewhere, reach out to GitHub’s official documentation for more troubleshooting.
Also, don’t shy away from exploring the community forums for more advice that could pertain directly to your individual use case. GitHub’s diverse user base promotes a rich sharing culture amplifying the chance that your exact problem has been encountered (and solved!) by others before.
GitHub Actions proves itself a powerful tool that you can use to automate your workflows. Yet, sometimes you may encounter issues where GitHub Action Workflows aren’t running as expected. Whether manually triggered or scheduled workflows, it’s imperative to decode these to streamline processes.
Decoding Manual Triggers Issues
Manually triggered workflows rely on the workflow_dispatch event. Suppose you’re experiencing challenges with GitHub Action Workflow not running even when manual triggers are in place – Here’s what could be wrong:
- You may have defined the
workflow_dispatch
event, but haven’t yet merged the changes into the main branch. GitHub only acknowledges the manual trigger from the main branch. Make sure to merge any changes including
workflow_dispatch
definition.
- Issues with input fields may also contribute to workflow failure – Remember to correctly and entirely fill in all the necessary parameters. Pay attention to data types, options, etc., to avoid run-time errors.
- If still unresolved, tracing back if the file is located on the .github/workflows/ directory may help.
GitHub docs provides extensive insights concerning manual triggers.
Scheduled Workflow Deadlocks
Scheduled workflows come in handy when looking to perform certain actions recurrently – Nevertheless, there might be instances whereby your scheduled workflows aren’t running. So let’s look at the potential problematic areas:
- The YAML file syntax may not be correctly defined for scheduling tasks. Verify your use of CRON syntax in your
on.schedule
. The correct format is a list with the cron key taking 5 space-separated values, represented by asterisks.
- If the repository is archived or doesn’t contain any activity for over 60 days, GitHub cancels scheduled workflows until there’s an action performed.
You can always refer to the GitHub documentation for comprehensive information on scheduling events.
To understand this concept better, let’s analyse a snippet of action code:
name: Workflow name on: workflow_dispatch: inputs: logLevel: description: 'Log level' required: true default: 'warning' schedule: - cron: '*/15 * * * *'
This example outlines a workflow that allows both manual triggering and scheduled running every 15 minutes.
Whether dealing with manually triggered workflows or scheduled workflows, Github Actions employs specific mechanisms to ensure smooth, automated operations. It’s crucial to have proper understanding of how these interact with different sections of your codebase so you can optimize their utility and efficiency.
Sure, I am glad to provide insights into understanding activity logs related to your GitHub Actions Performance and why your Github Action workflow might not be running.
GitHub Actions allows you to orchestrate any workflow, such as software build, testing, or deployment, directly from your repository. When executing the actions workflows, you can track their performance through activity logs. To get a clear view of what’s happening with your workflows, it’s essential to understand how these logs work.
Analyzing GitHub Actions Activity Logs
Activity logs contain the details of each run of your workflows in a reverse chronological order. They provide invaluable information like:
- The workflow that was executed.
- Whether the workflow run was successful or failed
- The reason the workflow run failed, if it did.
- The time taken for each step in the workflow to complete.
- The overall time taken for the workflow to execute.
Accessing these logs is quite straightforward, all you need to do is navigate to the “Actions” tab in your GitHub repository, choose a specific workflow from the sidebar on the left and select a specific run from the list.
Note: It’s crucial to often follow up on these logs because they may help identify what could be misconfigured, leading your GitHub Actions Workflow not to run.
Why Your GitHub Actions Workflow May Not Be Running
If your GitHub Actions Workflow is not running, there could be several reasons causing this:
- Workflow file location: Workflow files should be stored in
.github/workflows/
at the root level of your repository. If they are stored elsewhere, they will not run.
- Event triggers: Ensure that you’ve correctly specified the events that trigger your workflow. For instance, using
push: branches: [master]
only runs when changes are pushed to the master branch.
- Syntax errors: A common issue is syntax errors in the workflow file. These might range from incorrect indentation, wrong keywords or even missing brackets. To validate your workflow syntax, you refer to GitHub’s documentation.
- Billing issues: If your payment fails or if you’ve reached your included minutes for Github Actions, your workflows may not run until the issue is resolved.
- Disabled Actions: In some cases, GitHub Actions may be disabled on a repository-level due to various reasons such as a prolonged period of inactivity.
In short, on top of the readability, utility and code collaboration features GitHub offers, it also provides an ecosystem for CI/CD (Continuous Integration/Continuous Deployment) processes right from the repository through GitHub Actions. Understanding how to interpret activity logs helps ensure efficiency by identifying bottlenecks in workflows, potential errors, or misconfigurations that may prevent action workflows from running.
You can check out this reference to learn more about managing workflow logs for your GitHub Actions.
When you encounter the issue of your Github Action Workflow not running as expected, it’s essential to take a systematic approach to diagnosing and resolving the problem. The good news is that Github has a plethora of tools at your disposal to facilitate here.
Primarily, it’s important to confirm whether the status of Github Action Workflow and check if there are any reported issues or outages using their status page. Sometimes, the problem could be on Github’s end rather than an issue with your workflow code.
The next step is to re-examine the workflow configuration file, typically named
.github/workflows/actions.yml
. Make sure that the
on:
directive contains the correct set of events triggering the actions. Furthermore, pay attention to the indentation since YAML is extremely sensitive to correct indents.
Another vital debugging step is to inspect Github Action logs. You can access these logs under the ‘Actions’ tab of your repository. It has details about the steps executed during the action run and will provide you visibility into where the process might have failed.
If you continue to experience difficulties with your Github Actions not executing, it could warrant assessing third-party actions used in the workflow for any potential bugs. Utilize the power of the open-source community by checking with the maintainers of those actions or reading through closed/open issues on the specific Github Action’s repository.
An alternative workaround could be to manually trigger the workflow to see if the action runs successfully. You can do this using
workflow_dispatch
event directly from Github’s GUI.
Lastly, don’t discount the utility of Github’s forums and communities. The odds are that someone else has also faced a similar issue and solved it – make use of resources such as Github Community and StackOverflow.
Troubleshooting Steps |
---|
1. Check Github Status |
2. Examine the workflow configuration file |
3. Inspect Github Action Logs |
4. Verify Third-Party Github Actions |
5. Manually Trigger the Workflow |
6. Use Online Resources: Github Community, Stack Overflow, etc. |
Github Actions is a potent tool once understood properly. And fixing an action not running usually boils down to thoroughly verifying your workflow configuration and understanding the error logs. Remember, when in doubt, turn up the logging level! Happy troubleshooting!