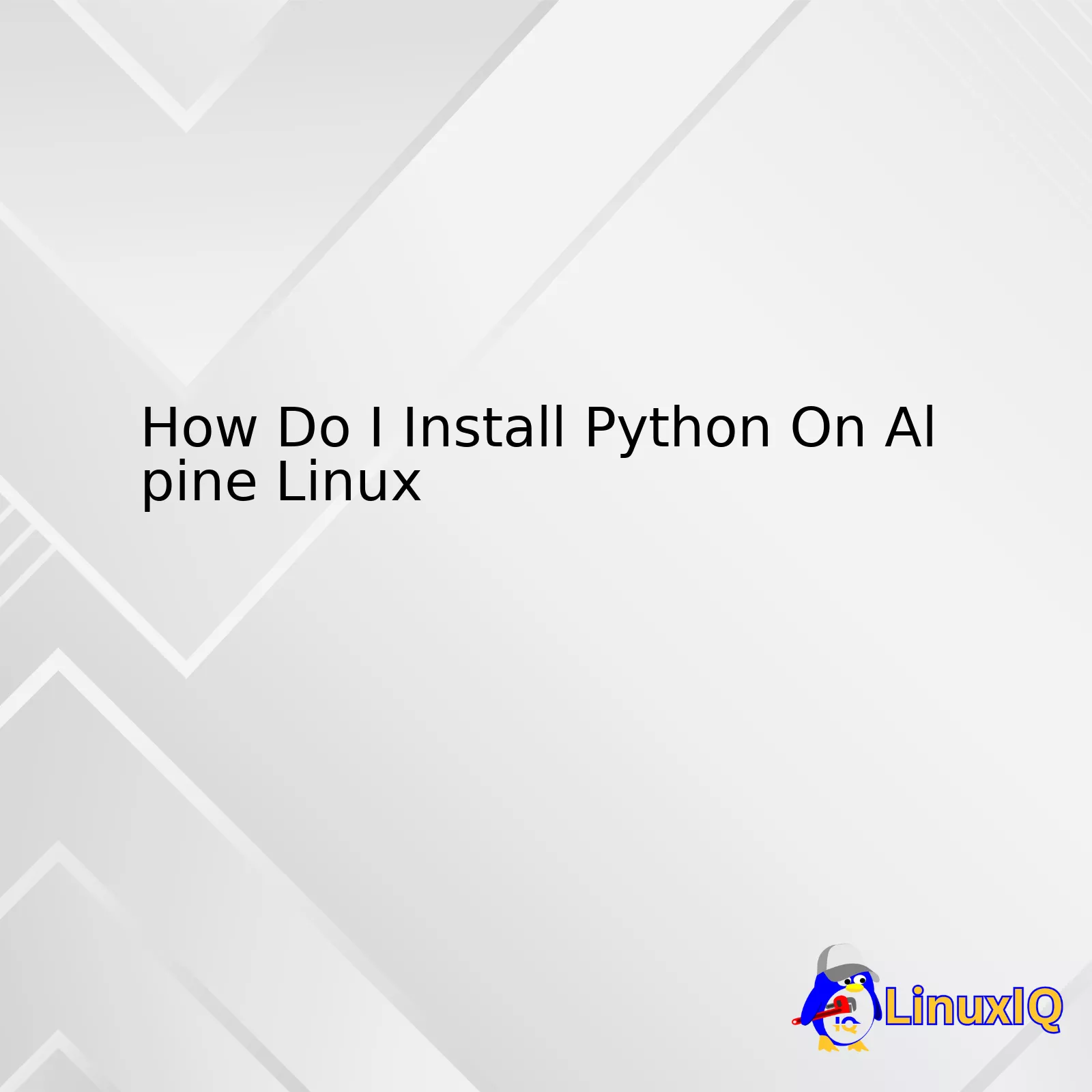
Step | Action |
---|---|
1 | Update existing packages list |
2 | Install Python and its package manager pip |
3 | Check Python version |
Firstly, before installing Python on Alpine Linux, it’s handy to update the list of available packages. This ensures that you will be downloading the latest version of Python. You can do this by entering
apk update
in the terminal.
The next step involves actually installing Python along with pip, which is Python’s package installer. For many coding projects, you will need additional libraries or ‘packages’ that aren’t included in the core Python language, so installing pip at this stage saves time later on. Type
apk add python3 py3-pip
and hit enter.
Finally, check that Python has been installed correctly by typing
python3 --version
. The terminal should display the Python version, confirming that it is ready to use.
Remember, when working with any new software or operating system, it’s crucial to refer to the official documentation or referable resources like Python docs or Alpine Linux package management page for accurate information.Sure, let’s dive right into it.
Installing Python on Alpine Linux is simple and straightforward; all you need to do is follow these steps:
Firstly, update the package list for Alpine Linux. For this, use Alpine Linux’s package manager, apk. The command should look like this:
apk update
After running the command, your system will connect to its configured repositories and pull down the latest package information.
Next step is to install the Python package on Alpine Linux. To do this, simply type in the following command:
apk add python3
Upon executing the given command, APK will find the correct package that fits the python3 virtual package and install it.
Once Python is installed, you can verify its installation and check the installed version by using the below line of code –
python3 --version
This command prints out the version of Python that’s currently installed, giving you an output similar to: “Python 3.8.2”. This means Python has been successfully installed on your Alpine Linux system.
To make development environments more manageable, consider installing pip, the Python package installer. Pip allows developers to switch between different projects employing unique libraries. To install pip, you use –
apk add py3-pip
With pip, you can install specific versions of a library directly from the Python Package Index (PyPI). PyPI is the default software repository for Python developers to store created libraries.
For those interested in a lighter variant of Python, pypy could be a better fit due to its lower memory consumption. Installing pypy follows similarly with:
apk add pypy3
Table with commands:
apk update
apk add python3
python3 --version
apk add py3-pip
apk add pypy3
Remember, while the regular Python package is still widely used, lightweight variants such as pypy are often preferred in environments like Docker containers where less is always more.
Find out more about Docker and its environments here: What is Docker?.
Summing up, keep in mind that the above steps install Python3, a modern version of Python vastly used nowadays. For specific versions or needs, please refer to Python’s user guide or the desired software documentation.
Here is an example of how everything looks in a Python file.
print("Hello, World!") a = 5 b = 10 print(a+b)
Executing the above script with Python gives you: “Hello, World! 15”.
Alpine continues to gain popularity due to its small footprint and decent collection of pre-packaged tools. These instructions to install Python makes it possible to enjoy the lighter operating system without sacrificing the power of Python.
Don’t forget to regularly update Python and other installed packages with:
apk upgrade
.
For additional guidance, refer to the official Apk documentation: apkrepos.Installing Python on an Alpine Linux system encompasses understanding the prerequisites and going about the actual installation process.
Let’s first delineate key considerations and prerequisites before giving a step-by-step guide on Python installation in Alpine.
The Prerequisites:
– Up-to-date System: Before any new software or application installation, it’s essential to ensure your system is updated. This includes your current Linux distribution, pre-installed softwares etc. You can use
apk update
command, which fetches the latest index of available packages from the repositories.
– Super User Privileges: Installing applications on a Linux based operating system typically requires super user privileges. Make sure you have these rights. We identify this requirement by seeing the word ‘sudo’ before many commands.
– Package Manager: Alpine uses the ‘apk’, that is ‘Alpine Package Keeper’ for package management. Being familiar with apk commands will help smooth out the installation process.
– Internet Connection: Since Python will be downloaded from the online repository, a stable internet connection is required.
Now, lets navigate towards the process of how you can install Python on your Alpine Linux.
How To Install Python On Alpine Linux:
Below is a precise sequence of commands used for installing Python:
– Firstly, update your existing list of packages:
sudo apk update
– Post updating the packages, proceed ahead to install Python3 via this command:
sudo apk add python3
A successful execution of this command will complete the Python 3 installation process. If you want to verify the installation and check python version, use:
python3 --version
This command will output the installed Python version, ensuring its successful setup in your system.
For Python related coding, another pertinent software might be pip- a package manager for Python. This can easily be installed using the following command:
sudo apk add py3-pip
So, there you go! With an up-to-date system, superuser privileges, familiarity with apk commands, and stable internet access, you can smoothly go about installing Python on Alpine Linux.
Remember, when jumping into the sea of coding and Python programming, getting an environment set up correctly is the very first stepping stone.source It not only eases further steps but also aids in avoiding potential hindrances. Once done, you’ll have both Python3 and pip installed in your Alpine Linux system and are ready to dive into Python programming.If you’re a Python developer venturing into the realm of Alpine Linux, you might find yourself in an unfamiliar environment. Many Python developers are more comfortable with Debian-based distros due to widespread compatibility and ample resources. However, the appeal lies in Alpine’s inherently lighter footprint and security focus will inevitably lead some developers towards this seemingly daunting mountain.
Here’s your guide on how to install Python on your Alpine Linux and troubleshooting common issues along the way:
Step 1—Essentials: Start by making sure that your package manager is updated with the latest packages:
# Update apk repositories apk update # Upgrade all the installed packages apk upgrade
Step 2—Install Python: Proceed with Python installation using apk:
apk add python3 py3-pip
Now, when installing Python on Alpine Linux, you might encounter some common hic-ups:
Trouble 1 – Installation command not found: If you’re getting an “apk command not found” response from the terminal, it’s likely because apk package manager isn’t installed on your version of Linux. To resolve this, you would need to switch to a distribution that does include apk package manager like Alpine Linux, or use the package management system that comes with your distribution (like apt for Ubuntu).
Trouble 2 – Package not found: In certain instances, despite completed updates, Python installation may fail due to it being unable to locate the Python package. This could be possibly due to mismatches in the repositories. To circumvent this issue, it’s helpful to manually add the main repository or mirror closest to your location manually. Sample implementation would look like:
echo "http://dl-cdn.alpinelinux.org/alpine/latest-stable/main" >> /etc/apk/repositories apk update
Trouble 3 – Python versioning: Python 2 was considered a legacy language after its end of life. As a result, the traditional ‘python’ command might not work. Since Python 3 is the current version, you’ll need to use the ‘python3’ command instead.
To solidify your new settings, don’t forget to verify your Python version installation:
python3 --version
Trouble 4 – Pip package: You may also face issues with pip, Python’s recommended package installer. If this happens, make sure you’ve installed pip alongside Python:
apk add py3-pip
It’s important to remember that Alpine Linux presents its own unique roadblocks to Python installation. Armed with a basic understanding and the ability to think through these potential obstacles, you can pave your way to becoming a successful Python developer on Alpine Linux.
There are additional resources available online, such as the official Python website, Alpine Linux Wiki’s package management page, and community forums should you encounter highly specific challenges beyond the scope of these steps.
After successfully installing Python on your Alpine Linux system, it’s vital to confirm that everything is set up correctly. This can be done through several post-installation checks and fine-tuning adjustments ensuring optimal performance and usage of Python features.
Post-Installation Checks
Firstly, verify the installation using the terminal command:
python --version
The response should display the installed Python version, confirming successful installation.
However, since we are concerned with Alpine Linux – a security-oriented, lightweight Linux distro – you need to use:
python3 --version
This will reveal the Python3 version, as Alpine Linux does not pre-install Python due to its dedication to minimalism and security.
Secondly, you need to check the Python package manager – pip, which aids in managing Python packages. Use the following command:
pip3 --version
If these commands return correct versions, Python is installed properly and ready for use.
Python Configuration on Alpine Linux
For the best Python experience, it’s crucial to configure Python settings to meet your unique development needs. Let’s explore the major configuration elements:
– Environment Variables: One significant setup includes environment variables like PYTHONDONTWRITEBYTECODE and PYTHONPATH.
PYTHONDONTWRITEBYTECODE ensures Python doesn’t try to write .pyc files on the import of source modules, giving a performance boost during development. To set this variable:
export PYTHONDONTWRITEBYTECODE=1
PYTHONPATH is another key variable that extends the system path to include directories hosting python libraries and packages. You can add directories as:
export PYTHONPATH=$PYTHONPATH:/my/new/path
– The .bashrc and .bash_profile files: These files store environmental settings and ensure Python works seamlessly even after reboots or new sessions. Modify these files by opening them in the editor and adding appropriate lines.
vi ~/.bashrc
– Virtual environments: Working with isolated Python environments suits situations where different projects require separate dependencies. Modules like venv make this possible:
python3 -m venv project_env source project_env/bin/activate
Configuration of installed Python on Alpine Linux ensures smooth integration with your workflows, facilitating great coding experiences. However, it’s important to understand that configurations depend entirely on use-cases and individual requirements.[1].
With all the steps covered to install Python on Alpine Linux, it is clear that this routine involves the effective use of the package manager. One thing about
apk
you should keep in mind is that it accesses install packages from a variety of sources including its own repository and others online.
# Start by updating apk repository apk update # Install python with apk apk add python3
The process takes only two commands, making this process a rather straightforward one. But remember, using Python efficiently requires knowledge of coding concepts – functions, libraries, classes, syntax, and expressions. It’s an essential script language for data analysts, scientists, software developers, and network engineers alike because of the increasing demand for big data processing capabilities.
Language | Used For |
---|---|
Python | Data Analysis, Web Development, Machine Learning, AI |
Becoming a pro involves practicing constantly. But before you start coding, ensure your version of Python runs in your ALPINE LINUX environment. You can check it using the command:
python --version
There are indeed several ways to achieve Python installation on diverse linux environments. This could be via compilation from source code or obtaining from alternative repositories. It’s crucial to consider these alternatives when running legacy systems not compatible with up-to-date Python versions.
For advanced users who need specific Python versions, consider PyEnv, an excellent tool for managing multiple Python installations in an OS Independent manner. No matter your skill level today, installing Python provides you access to a world of possibilities in tech, so start creating today!