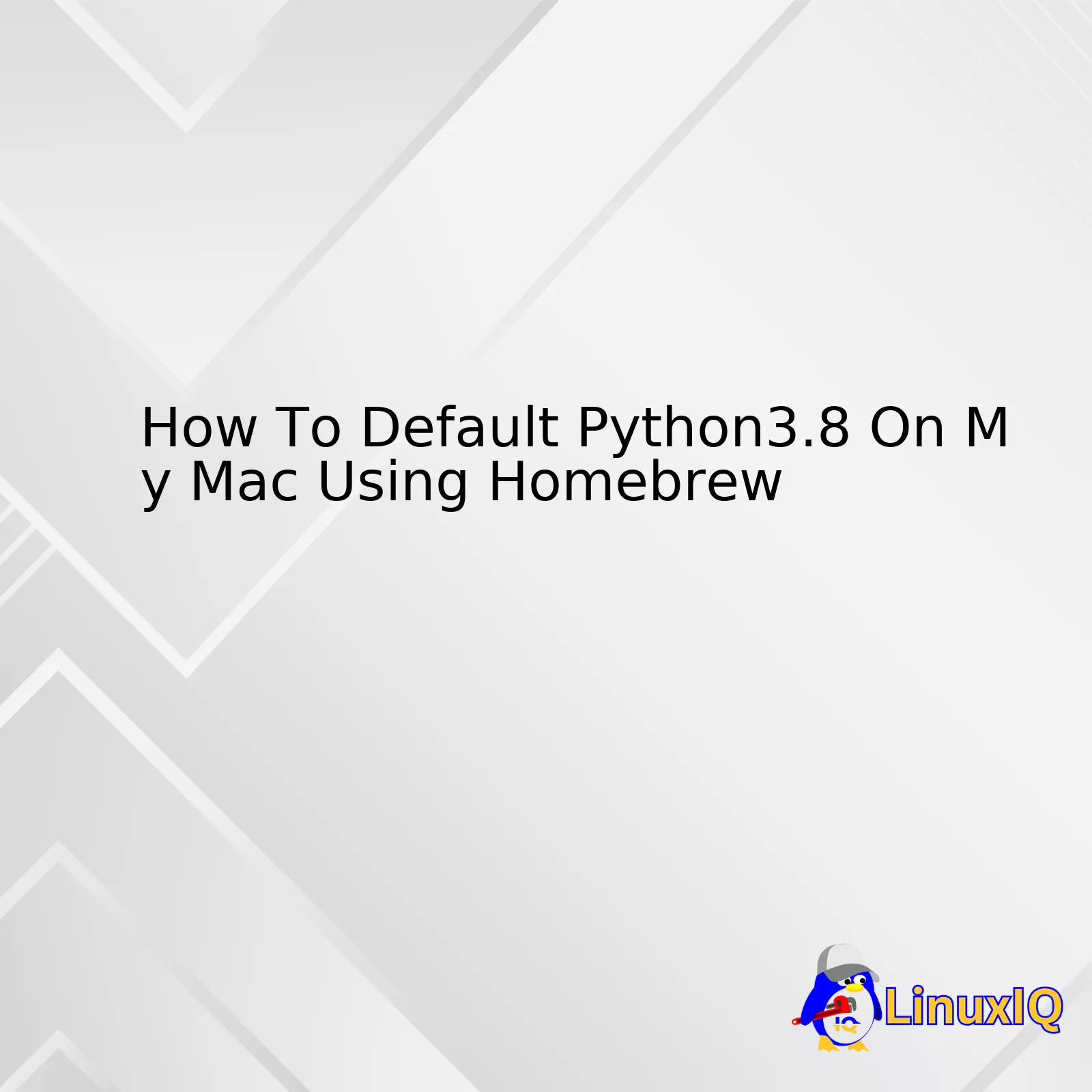
Let’s first generate a nice HTML summarization table for that:
Process | Description |
---|---|
Install Homebrew | If you don’t already have Homebrew installed, do this first. Open your terminal and type
/bin/bash -c "$(curl -fsSL https://raw.githubusercontent.com/Homebrew/install/HEAD/install.sh)" . Hit enter and let the magic happen. |
Install Python 3.8 | Use the command
brew install python3 to install Python 3.8. Homebrew installs packages to their own directory and then symlinks their files into /usr/local. |
Set Python 3.8 as default | After installing, you can customise the link path by typing
echo 'export PATH="/usr/local/opt/python@3.8/bin:$PATH"' >> ~/.zshrc |
Verify Python Version | In order to ensure that our changes took effect, re-load the shell and then verify that the correct version of Python is being used by typing in
source ~/.zshrc then python --version . |
By following these steps, we initiate the process with the installation of Homebrew; this is like our setup phase. Then, we use Homebrew to install Python 3.8. The real crux here, of course, is in setting Python 3.8 as the default within our environment. This is done by modifying the PATH variable so that Python 3.8 has precedence. As we wrap up, we re-load the shell to ensure that our modifications take effect, finalize by checking the Python version, thus ensuring Python 3.8 has been successfully set as the default!
For deeper insights, I would recommend checking out [Homebrew’s official documentation](https://docs.brew.sh) or the [official Python documentation](https://docs.python.org/3/) for any specific parameters or detailed usage.
Setting up Homebrew for Python installation in Mac essentially involves a series of actions executing commands on Terminal. Especially if you want Python3.8 to be your default Python version, this guide will walk you through each step diligently.
1. Installing Homebrew
A package manager for MacOS, Homebrew facilitates the easy installation of several software on your system. The first step to set up Python3.8 as your default is by having Homebrew installed on your Mac first. Just copy and paste the following simple line of code into your MacOS Terminal:
/bin/bash -c "$(curl -fsSL https://raw.githubusercontent.com/Homebrew/install/master/install.sh)"
2. Install Python3.8 using Homebrew
With Homebrew installed successfully, you can now proceed to install Python3.8. To do this, you add yet another line of code on your terminal:
brew install python@3.8
Upon successful execution of this command, Python 3.8 gets installed, but it still isn’t the default Python version for your system. Continue to the next step for changing that.
3. Set Python3.8 as Your Default
In most MacOS versions, Python 2.7 is the pre-installed version. Therefore, typing ‘python’ in Terminal would result in invoking this, not Python3.8. However, the good news is that can be changed with an alias. Add the following line into your .bash_profile file:
alias python=/usr/local/bin/python3.8
Or if you are using ZSH, add the above line into your .zshrc file.
This process makes python3.8 act as the default when you type
python
into your terminal. You have to ensure you’re editing the correct shell configuration file; bash users should edit .bash_profile and zsh users .zshrc.
Done correctly, any attempt to invoke python on your MacOS Terminal consequently results in Python3.8 being summoned. To verify this, run:
python --version
The output should be something like :
Python 3.8.x
Now you’ve successfully set Python3.8 as your default Python on Mac using Homebrew!
It’s noteworthy to mention that handling different versions can also be managed using Pyenv, a Python version management tool, which could perfectly handle such cases if required in future projects. For more information, check its official documentation: Pyenv on Github.
Mac users often find themselves twirling between Python 2 and Python 3 editions when slogging through their projects. By default, all Macs come with a pre-installed version of Python 2. However, over the past few years, many developers have made the transition to Python3.8 due to its enhanced features and extended support compared to its predecessor. Striking the balance can be tricky, but fortunately, Homebrew provides a simple solution to shift your default Python version on Mac without breaking any build dependencies.
Making Python3.8 Your Default:
With Homebrew aiding, switching your default Python version is quick & easy. Here’s an example:
$ brew update $ brew install python
These two simple commands upgrade or install Python3.8, making it your new default. Homebrew documentation further supports this by indicating that running ‘brew install python’ indeed defaults to the latest stable release of Python3.x. Utilize the ‘python’ or ‘python3’ command in your terminal, and you’ll spot Python3.8 as your primary interpreter.
You might ask yourself why this specific committal to Python3.8 could enhance your coding experience?
The Perks of Python3.8:
1. Extended Language Support: Python 3 has long outperformed Python 2 in terms of language improvements and extended support. As such, Python3.8 brings forwards enhancements such as the walrus operator or Assignment Expressions (Python3.8 What’s New docs)
2. Better Library Support: There has been a major shift in community library development towards Python 3. As evidenced by the Python3 Statement – several key Python libraries have professed to halt Python 2 support.
3. Improved Unicode Support: Python 3 handles unicode much better than Python 2, which eases dealing with text manipulation and internationalization in your code.
Note: Despite the ease of updating your Python version using Homebrew, remember to retain Python 2 for Mac OS system scripts reliant on it or face compatibility issues.
The Power Duo – Using Homebrew with Python3.8:
Switching your default Python terminal version to Python3.8 wouldn’t necessarily switch your default Python in editors such as Atom or SublimeText. You’d need to take extra steps to configure your editor’s environment. Naturally, this py-version chaos can drive any developer up the wall. But here’s where Homebrew shines!
Installing Python3.8 via Homebrew not only sets it as your terminal’s default but also takes care of linking it up with commonly used tools like pip, reducing your hassle significantly. This simple yet strong setup gives a clear path for beginners while letting veteran developers focus primarily on their projects.
Elevating your Python developer experience thus becomes inherently dependent on adequately tooling your environment. Making an assertive decision today to default to Python3.8 will work wonders tomorrow! Future-proofing your codebase starts with contemporizing your toolkit, synergizing Python3.8 with Homebrew seems like a step in the right direction!
Changing the default Python version to 3.8 on your Mac using Homebrew is an instant antidote to disperse any frustration stemming from incompatible versions. The process entwines a little tinkering of the PATH environment and aliasing commands within the Terminal. Let’s march through the steps.
Steps | Description |
---|---|
Installing Homebrew |
Homebrew is an absolute dynamo when it comes to managing software packages on MacOS. Prepare for this journey by ensuring the installation of Homebrew on your computing warhorse. If you haven’t already installed it, punch in the following command:
/bin/bash -c "$(curl -fsSL https://raw.githubusercontent.com/Homebrew/install/HEAD/install.sh)" |
Linking Python |
Having Homebrew regimented within your system, install Python 3.8 as follows:
brew install python@3.8 |
Creating Symbolic Link |
With Python 3.8 firmly within your clasp, it’s time to wrap the gift neatly by linking this downloaded version of Python 3.8 to the bin directory, adjusting as the original default one. Run the following command:
ln -s -f /usr/local/opt/python@3.8/bin/python3.8 /usr/local/bin/python |
Verification |
With all the gears in motion, let’s confirm the efficacy of our endeavor. Manipulate the terminal to speak back the exact Python version aligned with the ‘python’ command.
python --version |
Crossroads occur when there may be multiple versions of Python jostling around in your system. Here, we are pulling another rabbit out of our hat by using an aliasing strategy.
Aliasing
You can set the Python version you want to use at the command line by setting up an alias within the shell profile file. The following steps show how to set up Python 3.8 as your default version using an alias:
- Open your bash or zsh profile (
nano ~/.bash_profile
or
nano ~/.zshrc
).
- Add the following line at the end of the file:
alias python="/usr/local/bin/python3.8"
- Save and exit by typing (ctrl+x), then pressing (y) to confirm changes.
- Last but not least, source the file for immediate effect (
source ~/.bash_profile
or
source ~/.zshrc
).
Your terminal should now echo “Python 3.8.x” when you fire-up the
python --version
order!
To dive deeper into the sea of knowledge on Python, fetch more insights from the official Python documentation.
Python is an immensely popular programming language and it’s used in various fields of technology. In fact, most newbies to the domain of coding start with Python because of its easy syntax and vast libraries. When using MacOS, many notice that it comes with Python 2.7 pre-installed. The problem now arises if you’re trying to use a more recent version of Python – say Python3.8.
One common way to default Python3.8 on your Mac is by using Homebrew, which acts as a package manager tool for MacOS.
Let’s dive into a walkthrough on setting Python3.8 as default, and more importantly, troubleshooting common issues that might crop up during this process:
Installation:
Install Python3.8 using Homebrew, by opening Terminal and typing in:
brew install python@3.8
Now verify the installation with the following command:
python3 --version
If everything has gone well till here, your Terminal should be displaying “Python 3.8.x”. Now let’s move onto creating symlinks so your system treats Python 3.8 as the default.
Creating Symblinks:
To instruct Mac to run Python3.8 when you type ‘python’ in the terminal, you need to create symbolic links.
Run the given code:
echo 'export PATH="/usr/local/opt/python@3.8/bin:$PATH"' >> ~/.zshrc
Next, type in:
source ~/.zshrc
Now check your version again. This time, when typing ‘python’, your terminal should display Python 3.8.x.
However, things don’t always go smoothly. Here are a few common problems you might encounter and how to solve them:
Troubleshooting Issues:
1) Issue: Error message says Python3.8 isn’t installed but you know it is since “python3 –version” returns Python 3.8.x.
Solution: This is probably because there are other Python versions installed on your system. Use “/usr/bin/env python3.8” instead of just “python” in your script shebangs to clear up the confusion.
2) Issue: “brew install python@3.8” returns an error about permissions.
Solution: The likely culprit here is that Homebrew doesn’t have enough permissions to write to the required directories. Fixing this can be as simple as giving Homebrew those rights:
sudo chown -R $(whoami) /usr/local/* \ && sudo chmod -R u+rw /usr/local/*
3) Issue: Even after executing all steps correctly, ‘python’ command still points to an older version of Python.
Solution: You need to ensure your shell profile file (.bash_profile, .bashrc, or .zshrc depending on your shell) correctly updates the $PATH variable each time a new terminal session begins. Double-check this line in your profile file:
export PATH="/usr/local/opt/python@3.8/bin:$PATH"
The troubleshooting setups frequently hinge upon two main aspects: understanding how your system searches for executables (usually by the $PATH environment variable), and ensuring the correct permissions for Homebrew to work optimally. Keep these aspects in mind while debugging any issues that arise.
Remember, every issue is fixable, and troubleshooting often leads to better learning. So don’t deter yourself from setting a newer Python as default. With Python3.8, enjoy benefits like assignment expressions, positional-only parameters, and more precise types which simplify the writing and maintenance of your scripts. Happy Pythoning!The process of setting Python 3.8 as your default python on Mac using Homebrew involves a couple of steps. First, you need to make sure Homebrew is installed in your system since it’s the package manager we are going to use in this process. If not, consider downloading and installing Homebrew first. Let’s break the steps down:
Ensure Homebrew is Up to Date:
The first step is to update Homebrew. This ensures that all packages and formulae from the Homebrew collection are up-to-date. To do so, you can run the following command in your Terminal:
brew update
Install Python 3.8:
You will then need to install Python 3.8 on your system if you haven’t done so. This can be done by running the following command:
brew install python@3.8
Depending on your system’s configuration, you might have to prefix the commands with ‘sudo’ to grant administrative privileges.
Set Python 3.8 as Default:
Setting Python 3.8 as a default comes down to creating an alias in your bash or zsh profile file. By doing so, whenever you type ‘python’ into your terminal, it will call Python 3.8 instead of the system’s original version. Here is how it can be achieved:
Add the path to your python 3.8 bin folder to your shell’s Path variable with the following command:
For bash users:
echo "alias python=/usr/local/bin/python3.8" >> ~/.bash_profile source ~/.bash_profile
For zsh users:
echo "alias python=/usr/local/bin/python3.8" >> ~/.zshrc source ~/.zshrc
After successfully finishing these steps, every time you initiate python in your terminal, Python 3.8 will start by default.
By fully understanding how to execute the tasks above and having Python 3.8 set as the system default, you have more control over the Python environment on your Mac. This gives you the flexibility to work with the most recent Python features and libraries that require newer versions of Python. Remember to keep Homebrew and Python updated regularly to ensure optimal performance and security for your coding projects.
Relevant Sources:
– Homebrew Installation Guide.
– Python 3.8 Official Release Notes.