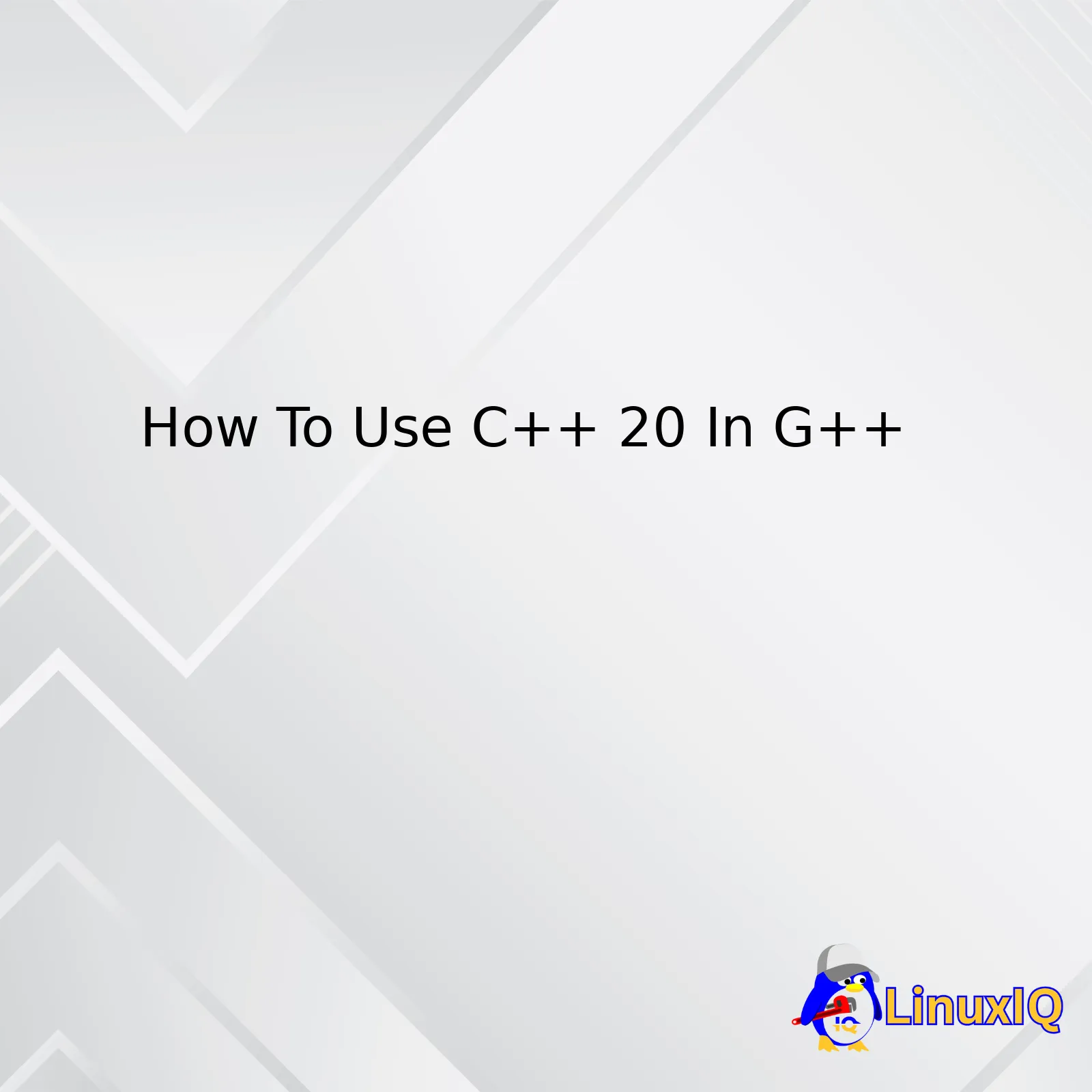
Steps | Description |
---|---|
Install the Latest Version of G++ | You need to ensure that g++ is up-to-date, as only recent versions support C++20. You can get the latest version from this link. |
Check the Installed Version of G++ | Use the terminal command
g++ --version to confirm that you have the right version installed. |
Add the C++20 Flag | While compiling your code, add the
-std=c++20 flag to enable C++20 features. |
Write and Execute Codes | At this point, you can write codes utilizing C++20 features and execute them using G++. If there are any issues, they’ll likely be due to not following new syntax rules or there being glitches in the newly implemented features of C++20. |
It’s crucial for any coder to be updated with newer standards and conventions as they often optimise existing functionalities and bring in novel, efficient ones. For someone well-versed with C++, employing the 2020 standard in G++ isn’t a considerable hassle. The tricks lie in details.
The first step is installing an updated version of G++. As of now, G++ 10.1+ supports C++20. After confirming the same via `g++ –version`, including a small `-std=c++20` while compiling activates the required functionalities. Post this, it’s about diving deep into the coding marathon.
Cautions? Not all C++20 features might be bug-free initially, and some may be prone to tweaks later on. Hence, if any errors arise even after complying with C++20 syntax, it might be owing to unpolished features. A handy practice would be to maintain compatibility with a stable (older) version until C++20 matures. Patience is key when embracing novelty!Alright, let’s dive right into it. C++ 20, released in December 2020, brought a wealth of essential additions that optimize and streamline developers’ work with the language. Notably, some of its significant updates include modules, coroutines, various new libraries, ranges library, and concepts. Working with these features in G++, the GNU g++ compiler, requires understanding their specifics, utility, and implementation techniques.
One primary update was Modules. Modules minimize header dependencies plaguing earlier C++ versions, making your code more readable and enhancing compile-time performance. Also, it permits better encapsulation by creating boundaries between API and its implementation detail. You can implement Modules set up by setting up the .cppm extension for module interface units, declaring export module ModuleName in the file. Here’s an example:
//Math.cppm export module Math; export int add(int a, int b){ return a+b; }
By doing that, you have created a Math module exporting an addition function. Importing it elsewhere in the app would entail:
import Math; std::cout<
Coroutines is another fabulous C++ 20 feature promising asynchronous programming optimization: it boosts performance by saving memory overhead incurred from numerous thread allocations. With G++, Coroutines are implemented by employing co_await, co_yield, and co_return keywords.
Concepts introduced in C++ 20 provide specification templates to check a template argument against a sequence of requirements. Think of it like a blueprint, ensuring that the objects used in template parameters adhere to a specific form. A very basic use case of a concept in C++ 20 can be:
templateconcept Integral = std::is_integral_v ; template T double_value(T value) { return 2*value; }
In this snippet, "Integral" concept ensures the type passed in the template parameter of double-value function is an integer. If it's not, the code will never compile.
Several new libraries added to the standard specify a below-listed range library like date and time library, text formatting library, and others. Especially relevant to our discussion, the Ranges Library is a powerful tool redefining the way we write algorithms in C++. Instead of a pair of iterators, it provides you with a single range.
Incorporating C++ 20 features in G++ necessitates updating your development environment. As of the moment, GCC version 11 supports most of these features under a flag -std=c++2a or -std=c++20. So, ensure that you run a proper G++ version compatible with C++ 20 for the best working experience.
To install the latest version, use:
sudo add-apt-repository ppa:ubuntu-toolchain-r/test sudo apt-get update sudo apt-get install gcc-11 g++-11
And activate it utilizing:
g++-11 -std=c++20 your_file.cpp
From there, enjoy exploiting all C++ 20 innovations. Bear in mind keeping tabs on GCC updates since future releases might provide extended support for additional features. For up-to-date information on your GCC version support for C++20 features, visit C++ Standards Support in GCC.
As a professional coder, appreciating modern standards accelerates your productivity while reducing complexity. Hence, be open to learning and integrating them into your workflows. This should provide a comprehensive understanding of how to use C++ 20 with G++. Happy coding!With the continuous advancements of technology, the field of coding needs to keep evolving. The latest in C++ development has been the transition from earlier versions to C++20. This newer version brings numerous benefits, including improved functionality and efficiency. The GNU Compiler Collection (GCC) offers G++, a compiler system that supports C++ languages. Currently, GCC provides full support for the latest standards including C++20 through its g++ compiler. If you're considering upgrading to this relatively new programming language extension, here's an analytical guide on how to navigate the upgrade path:
Step 1: Make sure you have the right tools
To get started with C++20, you need to use a version of g++ that supports it fully. As of early 2021, this means using GCC version 10 or later.
$ g++ --version g++ (GCC) 10.2.0 ...
The above command shows if your g++ version is 10 or higher.
Step 2: Enable C++20 in g++
By default, g++ uses the latest stable version of the C++ standard, which may not be C++20. So, you'll need to explicitly tell g++ to use C++20. You can do this by adding the
-std=c++20
flag when compiling your code.
$ g++ -std=c++20 my_code.cpp -o my_program
This tells g++ to compile my_code.cpp as C++20 code and output the result to my_program.
Step 3: Start utilizing C++20 features
Once C++20 is enabled, you can start taking advantage of its many useful features like ranges, concepts, coroutines, and more. Here's an example of how you might use the new range-based for loops in C++20:
#includeint main() { std::vector numbers = {1, 2, 3, 4, 5}; for (auto number : numbers) { // Do something with each number. } return 0; }
Step 4: Troubleshooting issues
If you encounter any problems while upgrading, refer to error messages and online resources for help. For example, if you're getting error messages about missing headers, make sure your g++ installation is up-to-date and includes all necessary C++ libraries. In case of more complex errors or problems, it's often helpful to simplify your code and isolate the problematic part. A variety of online forums and programming communities like Stack Overflow are available to assist with troubleshooting.
Upgrading to a new coding standard might seem daunting, but the advantages of the upgrade significantly outweigh the initial hurdles faced during the transition. From streamlining existing processes to exploring exciting new features, the move toC++20 with g++ will definitely prove advantageous in the long run. Remember, mastering a new tool or technology is always a matter of practice and continual learning, so take your time and enjoy the journey into C++20's innovative resource box.To understand how to use C++20 in GCC (GNU Compiler Collection), we'll focus on one of the most significant features introduced in C++20 - modules. Modules aim to provide a new method of code organization through a mechanism that is significantly different from headers and source files.
Setting Up GCC for C++20
$ g++ --version
If it's less than 10, you'll need to upgrade. On Ubuntu, you can use these commands:
$ sudo add-apt-repository ppa:ubuntu-toolchain-r/test $ sudo apt update $ sudo apt install g++-10
Now, let's ensure g++-10 is our default C++ compiler:
$ sudo update-alternatives --install /usr/bin/g++ g++ /usr/bin/g++-10 100 $ sudo update-alternatives --config g++
G++ Compilation Flags for C++20
Post setup, to make use of the latest language features, we need to indicate this preference when compiling our programs. G++ utilizes particular flags to specify the C++ standard we intend to use:
$ g++-10 -std=c++20 your_program.cpp
Alternatively, to express the latest version available, you can type:
$ g++-10 -std=c++2a your_program.cpp
The `-std=c++2a` keyword may soon be replaced with `-std=c++20`, subject to full ratification of the standard.
Understanding and Implementing C++20 Modules
A simplified example of using modules would involve creating two files: `hello.cppm` – the module interface file (.cppm being the extension for C++ Module files) and `main.cpp` that consumes the module.
Here's what our `hello.cppm` might look like:
export module hello; export void say_hello();
Notice how our `say_hello()` function declaration uses the 'export' keyword? That means it will be usable by our `main.cpp` file.
Our `main.cpp`:
import hello; int main() { say_hello(); return 0; }
In `main.cpp`, we've used the 'import' keyword to include our module. It also directly calls the `say_hello()` function.
At the time of writing, G++ still lacks direct module support. A workaround, however, exists:
$ g++-10 -std=c++2a -c hello.cppm -o hello.o $ g++-10 -std=c++2a -fmodule-file=hello.o main.cpp
Firstly, we compile our `hello.cppm` module into an object file, namely `hello.o`. Secondly, while compiling the `main.cpp`, we link to `hello.o` by using `-fmodule-file=hello.o` flag.
Modules are greatly beneficial in providing superior code organization, privacy, and compilation speed compared to traditional header files. However, their recognition and standardization across compilers may still undergo refinement requiring us to stay updated with GCC's C++20 implementation status.
There you have it; a brief overview of setting up GCC & implementing modules in C++20, marks noteworthy strides for C++ development. And although it's but one feature of the significant advancements made in C++20, understanding them individually allows us to appreciate the language’s evolution and progress.Delving into the expansive world of C++, there's plenty to explore, especially when it comes to embracing new conventions and functionalities introduced in C++20. On stage is the 'concepts' and 'requires' clause in G++, part of the newest specifications that have caught the attention of developers around the globe.
Concepts in C++20 can be thought of as predicates. They introduce a way to express the intended behavior of template arguments which can lead to better error messages, documentation and, overall, more expressive code.
Here's a small example:
templateconcept EqualityComparable = requires(T a, T b) { { a == b } -> std::same_as ; }; template bool my_equal(T a, T b) { return a == b; }
Here, we are defining a concept named `EqualityComparable`. It has one requirement: You should be able to use `==` with two instances of the type `T`, and it should result in something convertible to `bool`. We then declare the function `my_equal` which checks for equality between two `EqualityComparable` types.
GCC began partial support for Concepts in version 6.1, with full support landing in GCC 101. This feature is enabled by using `-std=c++20` or greater during compilation.
To compile your program with C++20 because you want to include; Concepts or Requires Clause in G++, use the following command:
g++ -std=c++20 myfile.cpp
It's also useful to remember updating your G++ compiler version through an online repository2 before you try using syntaxes only provided in newer versions like C++20.
Requires clause, on the other hand, allows fine-grained control over template instantiation. It puts forward some prerequisites: For example, if a certain member function should exist, or a specific operation ought to be valid. Essentially, the `requires` clause halts the compilation just in time if the precondition—as specified within the clause—does not hold true.
Consider the following example:
templaterequires requires (T x) { x + x; } T double_value(T value){ return value + value; }
In this case, our function `double_value` uses a requires clause to stipulate that its template argument `T` must support the `+` operation. Thus increasing the expressiveness yet again and allowing us to encode more complex requirements in our templates.
It's a truly exciting period for C++ as these additions echo a broader trend towards safer and more expressive typesafe programming. Mastering the concepts and requires clause in G++, catering specifically to C++20, surely seems like a competitive edge worth cultivating.
--
1: GCC, Concepts - Status Link
2: Linux Package Manager - Arch Wiki LinkThe use of Coroutines in real-world projects provides an alternative, and often more effective way of managing asynchronous operations. A Coroutine is a generalization of subroutines (or functions) meant to be used for non-preemptive multitasking, where a process willingly yields control periodically or when idle so that other tasks can run concurrently. The most outstanding feature of coroutines is their ability to manage the suspension and resumption of execution in a manner that is efficient and capable of handling many routines concurrently.
When dealing with C++20 in G++, one of the features offered are Coroutines. As of GCC 10, experimental C++20 Coroutine support is available using the -fcoroutines flag. Here's how you might apply them in real-life scenarios:
//This is a simple example of a Coroutine #include <experimental/coroutine> #include <iostream> struct Task { struct promise_type { auto get_return_object() { return Task{}; } auto initial_suspend() { return std::experimental::suspend_always{}; } auto final_suspend() { return std::experimental::suspend_always{}; } void return_void() {} void unhandled_exception() { std::exit(1); } }; }; Task asyncFunction() { co; } int main() { asyncFunction(); }
In this code example, we have defined an `asyncFunction` Coroutine that yields control upon calling. Although it seems simple, it can be easily expanded for complex real-life operations.
For instance, consider a system where multiple devices send sensor readings for recording and analysis. Fetching these readings in blocking I/O operations would inevitably slow down system operation as the number of devices increase. Using C++ Coroutines, we can perform these operations asynchronously, allowing for scalability and efficiency.
//Fetching sensor readings through RESTful API #include <chrono> #include <iostream> #include <httplib.h> #include <json.hpp> using json = nlohmann::json; httplib::Client client("http://localhost:8080"); struct Resumable { struct promise_type { ... bool await_ready() const noexcept {...} void await_suspend(std::experimental::coroutine_handle<>) {...}; HttpResult await_resume() {...}; }; }; Resumable GetSensorReadings(const string& deviceID) { std::this_thread::sleep_for(std::chrono::seconds(3)); //Simulating network delay httplib::Result result = client.Get(("/devices/" + deviceID).c_str()); if (result && result->status == 200) co_return json::parse(result->body)["readings"]; co_return json{}; } int main() { Task getReadings = GetSensorReadings("deviceX"); ... }
We've created a `GetSensorReadings` coroutine that performs an HTTP request to fetch sensor readings from a device with the specified ID. It makes use of sleeper seconds to simulate a network delay, showcasing how coroutine can help when dealing with latency.
There are different scenarios where Coroutines become particularly handy - network programming (inputs/outputs), simulation, game programming, multi-threaded GUI event-driven programming and cooperative task scheduling just to name a few.
For further details on the C++ Coroutine specification and examples, you may want to visit ISO/IEC JTC 1 N4736 paper and cppcoro github repo.
In C++20, several new features were introduced to help programmers work more effectively. Ranges and views are two of these useful features that can make your coding tasks easier and less prone to error. Below, I’ll be providing an explanation of what these features are and how you can use them in your G++ projects.
Ranges in C++20
Ranges were introduced in C++20 as a library for bringing together algorithms and containers. This combination helps to cut down on boilerplate code and improve semantic elegance when dealing with sequential containers like vectors, lists, and others.
A sample use of ranges looks somewhat like the one shown below:
#include#include #include #include int main() { std::vector v = {0, 1, 2, 3, 4, 5}; auto even_vals = v | std::views::transform([](int n) { return n * 2; }); for (int n : even_vals) std::cout << n << ' '; }
This code doubles each value in vector
v
, using a range-v3 algorithm via the pipe operator
|
. The transformed results are stored into the
even_vals
object which is then used to print the values. This approach allows the manipulation of container elements to be simpler and more expressive.
Views in C++20
Views are lightweight objects, introduced in C++20, which provide alternative views into existing data structures (like arrays, strings, etc.). They are essentially non-owning partial views into the sequence defined by a range. By applying views to ranges, we are able to generate information from ranges without creating new containers or modifying the original data.
Consider the following example:
#include#include #include int main(){ std::vector numbers{1, 2, 3, 4, 5, 6, 7, 8, 9}; auto odd_numbers = numbers | std::views::filter([](int n){ return n % 2 == 1; }); for (int n : odd_numbers) std::cout << n <<‘,’; }
This simple piece of code uses a view to filter out all odd numbers from a vector. Views can thus reduce resource usage and improve performance because they do not replicate data - they simply refer to it.
Using C++20 in G++
If you want to take advantage of these C++20 features through G++, you need to ensure that you're using a G++ compiler version with C++20 support. As of writing, this would be GCC 10 or later. To specify the C++ version, you use the
-std
flag followed by the version specifier.
Here's how you compile a C++20 program with G++:
g++ -std=c++20 program.cpp -o program
After executing the above command, the C++20 code inside
program.cpp
will compile into an executable named
program
.
Remember to always update your compiler to its latest version so that you'll be able to utilize all the aforementioned features of C++20 and stay up-to-date with the standard language revisions.
To know more about G++ compilations, here is an online hyperlink reference for further reading: GCC and the C++ Standard
Using std::format for text formatting in C++20 can be an intuitive way for developers to manage the display of their outputs. It's a part of the new features included in C++20 to make handling strings more convenient and less error-prone. However, using it legitimately will require knowledge of some focal aspects such as how to install G++ on your operating system, because you need the G++ version that supports C++20 to use std::format function at all.
A significant note is that as of this moment, GCC (thus G++) does not yet have complete full support for C++20, which includes the availability of std::format. However, you can follow the progress of their C++20 implementation here.
To check whether the installed version of G++ supports C++20 or not, you can use the command:
g++ --version
This will return the installed version of G++. The support for C++20 started with GCC from version 10. To make sure your g++ compiler uses C++20, use the compiler switch -std=c++2a. Here's an example:
g++ -std=c++2a myfile.cpp
Once you've established that C++20 is supported, you could potentially utilize std::format. This function would typically be adopted like below:
std::string s = std::format("Hello {}", "World"); // Will format string as "Hello World"
std:format mediates convenience and improved performance for developers dealing with string manipulations. Although not currently supported on all compilers, including G++, it emphasizes the direction modern C++ is heading towards—becoming more user-friendly and shielding programmers from common errors.
One day when it becomes available on the G++, remember to incorporate it into your programming arsenal as an efficient tool for text formatting and string manipulation tasks.
Function | Description |
---|---|
std::format() |
Formats text according to the specified format string and returns the result as a string |
Refer online for more details about std::format.
Please recall only to use features your installed version of G++ supports. Always verify the programming tools and languages versions before starting major projects. This will ensure a smooth programming experience and prevent compatibility issues during development.
C++20 introduces a wide array of new features that elevate the productivity and effiency of programming in C++. Among these novel features, the Spaceship Operator, or Three-Way Comparison operator `<=>`, makes a debut. This operator contributes to simplification of code, reduction of boilerplate involved in comparison operations, and provides an intuitive way to express comparisons.
To utilize the exciting features of C++20 in G++, you will need the latest version that supports this standard which, at the time of writing, is g++-10. Install it via a package manager such as apt for Ubuntu:
sudo apt install g++-10
Once installed, you can enable the C++20 standard by adding the `-std=c++20` flag when compiling:
g++-10 -std=c++20 your_file.cpp
Now let's get into the fervent exploration of using the Spaceship Operator in some C++20 code!
The spaceship operator comes handy in comparing two variables. It doesn’t just tell us whether the values are same or not but provides detailed comparison information.
If 'a' and 'b' are two variables:
auto result = a <=> b;
Here’s what we can derive from the result:
* If `result < 0`, then `a < b`
* If `result == 0`, then `a == b`
* If `result > 0`, then `a > b`
Take a look at a practical code sample: