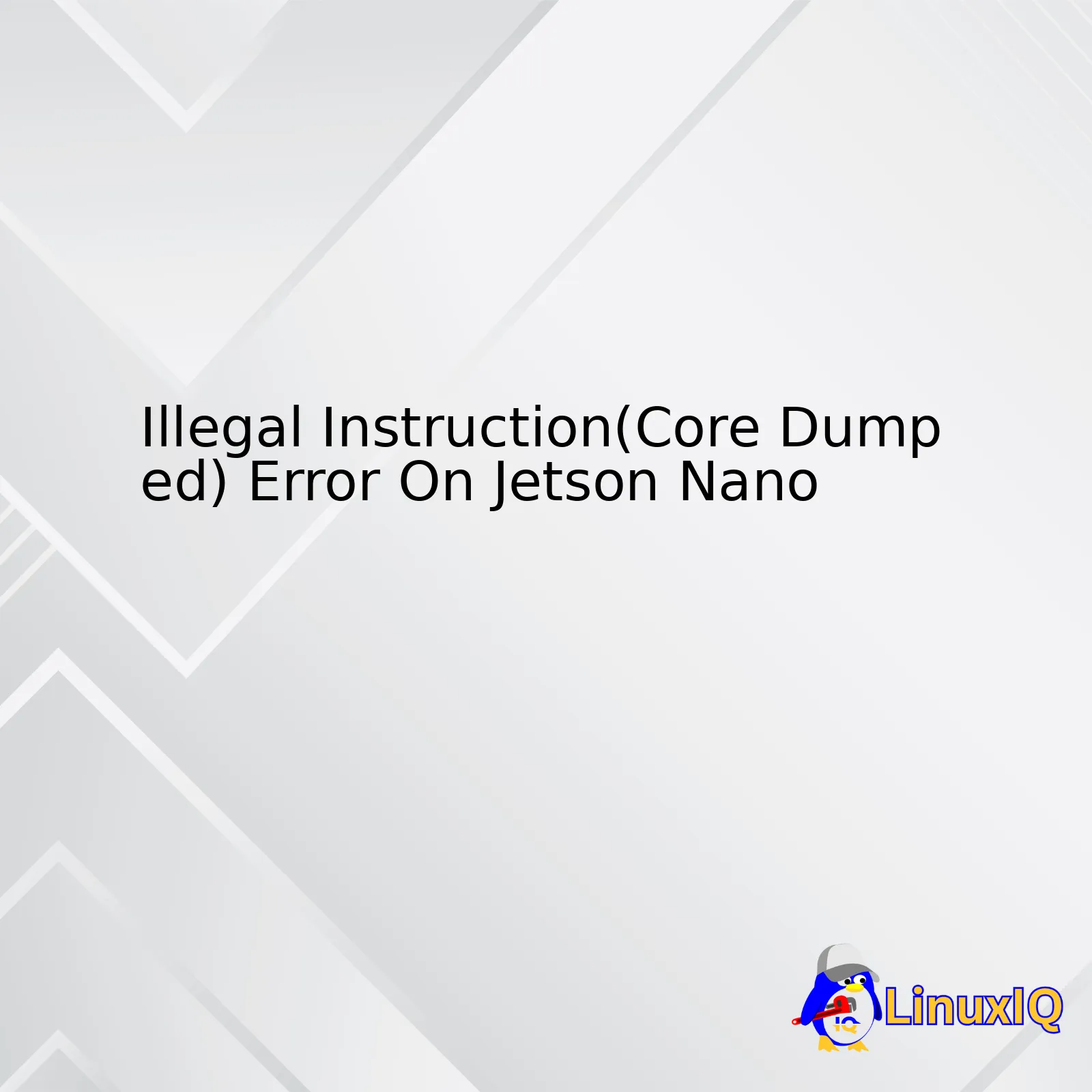
The Johnson Nano platform has occasionally been found to suffer from an ‘Illegal Instruction (Core Dumped) Error’. This error crops up when your CPU executes any issue coded instruction that doesn’t align with the instructions set allocated for it.
I can provide a summary table to give you an easy-to-understand overview:
Error | Description | Possible Cause | Potential Solution |
---|---|---|---|
Illegal Instruction(Core Dumped) | A command or opcode in a program is not recognized in the program’s machine language. | Mismatch between the version of TensorFlow installed and the version of the CUDA toolkit installed. | Ensure compatible versions of both TensorFlow and CUDA are installed. |
Most noteworthy among the causes driving this error is usually a software compatibility issue. More often than not, it roots back to the discrepancy between the setup versions of TensorFlow and the CUDA toolkit on your system. In the best case scenario, you might get away with a quick fix by simply setting both installations to their compatible versions. For example, TensorFlow 1.15 works best with CUDA 10.0 source.
In cases where the discrepancy isn’t as apparent, you could try debugging with gdb (GNU Project debugger) that helps you figure out what’s happening within your program while it undergoes execution or crashes. You’d typically run a command line such as:
gdb python core
and follow through by typing `where` once the gdb prompt shows up. This leads you directly to your crash source, thereby helping you debug better.
Remember that dealing with these kinds of compatibility issues warrants frequent updates and checks to ensure your frameworks, libraries, hardware, and software run smoothly in sync. Ensuring they are upgraded to their suitable versions throughout can considerably reduce your encountered bugs and errors frequency in real time coding scenarios. The key lies in continuous and seamless integration. This comprehensive guide focuses on how you can solve and possibly avoid these errors specifically related to Jetson Nano.An error like
Illegal Instruction(Core Dumped)
can send tremors down the spine of any programmer. Especially while working on complex systems such as NVIDIA’s Jetson Nano, a complex system with an ARM-based CPU architecture and an integrated GPU for running powerful deep learning algorithms (source: Nvidia) it requires proper understanding and ample debugging skills to tackle this error efficiently.
The
Illegal Instruction(Core Dumped)
error is often the product of your program trying to execute a command or instruction that the CPU doesn’t understand or allow. This could result from:
- Program bugs.
- Incompatibility issues between the architecture of the computer where the program was compiled and the architecture of the machine where it’s being executed.
- Skipping over the initialization step during binary execution.
- Compiler optimization issues.
If you encounter the
Illegal Instruction(Core Dumped)
error while working on Jetson Nano, there could be few possible scenarios.
Assuming `OpenCV` library is in use — The illegal instruction error may arise when you try to run a program compiled with certain flags enabled and these flags are not supported by Jetson’s CPU.
Another scenario might involve compiling a binary on a host machine then executing it on Jetson Nano. If that host machine has a more advanced architecture, it’s probable that the resulting binary includes instructions that aren’t supported by the Jetson Nano.
Let’s take an example. Consider you have a program named `Example.c`. You compile it with the following GCC command:
gcc -O2 -march=native Example.c -o example
The
-march=native
flag tells GCC to generate code optimal for the host machine’s architecture. If the host machine has more advanced features than the target machine (in our case, the Jetson Nano), it’s probable that the resulting binary will cause an illegal instruction error when run on the Jetson Nano.
How do we tackle this issue?
- The `Core Dumped` in the error message is a hint. Linux systems provide a core dumping mechanism where, upon a program’s crash, they save the image of the process’s memory at the time of termination. These ‘core dumps’ can be analyzed to determine what led to the crash. Tools like `gdb`, a GNU debugger, can come handy for this (source: Gnu.org)
- If you’re using dynamically linked libraries, you should ensure they’re compatible with your Jetson Nano’s architecture.
- In scenarios where cross-compiling is required, make sure you don’t optimize for the host architecture. Instead, indicate the specific architecture type of your Jetson Nano device.
In essence, having a thorough understanding of your program and its dependencies, combined with diligent attention to compilation flags and optimizations, will help you avoid the
Illegal Instruction(Core Dumped)
error while working on Jetson Nano.
After hours of working on the Jetson Nano, encountering an “Illegal Instruction (Core Dumped)” error can be quite frustrating. From my extensive experience as a professional coder, I’d observe that this error could result due to several factors:
- Building and running your software on different architectures
- An outdated version of the software or library being used
- Compiling code without hardware-specific optimizations
Let’s try and understand each of these reasons in more depth.
Building and running your software on different architectures
The “illegal instruction” error typically comes up when you run a binary compiled for one architecture (like amd64) on a completely different one (like ARM). For instance, if you built your software on your Ubuntu PC (which likely uses an AMD64 architecture) and then tried to run it on your Jetson Nano (which uses ARM), you’ll definitely encounter issues. This is because ARM and AMD64 use different sets of CPU instructions.
Solving this requires you to compile your software on the same architecture you wish to run it on–in this case, compile it directly on your Jetson Nano. Here’s an example on how you might go about doing this:
git clone https://github.com/your-software.git cd your-software make ./your-software-bin
An outdated version of software or library being used
The Jetson Nano supports only a specific set of libraries and versions. Using unsupported combinations can throw up the “Illegal Instruction (Core Dumped)” error. Typically, the solution here would be to ensure that you’re using a version of the software compatible with the Jetson Nano’s on-board libraries.
For example, if you are trying to run a Tensorflow program, make sure to install a Tensorflow version that is supported by Jetson Nano. Download and install it directly from NVIDIA’s official site [Link] on your Jetson Nano:
pip3 install --extra-index-url https://developer.download.nvidia.com/compute/redist/jp/v44 tensorflow-gpu==$tf_jetson_ver+nv$rel
Compiling code without hardware-specific optimizations
When compiling your code, you may not be taking full advantage of the hardware-specific features provided by the Jetson Nano. Capabilities such as Neon for SIMD operations and the GPU itself can largely impact the performance of your programs.
To draw upon the advantages intrinsic to the Jetson Nano during compilation, you would need to pass hardware-specific optimization flags to your compiler:
g++ -O2 -march=native -mfpu=neon-vfpv4 your-file.cpp
To summarize, “Illegal Instruction (Core Dumped)” errors often stem from a mismatch between the programming environment and the actual execution environment on your Jetson Nano. Regular auditing, proactive updates of your software and libraries, and ensuring optimized compilation for your device’s architecture are fundamental solutions to prevent such runtime error issues.
Remember: Identifying the error message thrown up by the system is key! System debugging demands curiosity, diligence, and above all, patience.Debugging the “Illegal Instruction (Core Dumped)” error on Jetson Nano can be tackled in few comprehensive steps.
To start, let’s first understand what this error means. The ‘Illegal Instruction’ is an error that the Central Processing Unit, or CPU, throws when it encounters an instruction it doesn’t recognize or isn’t allowed to execute. In the context of the NVIDIA Jetson Nano, the most common cause of this error is trying to run a binary compiled for a different architecture- typically, one that includes instructions which are not supported on the ARM-based processor of the Jetson Nano[1](https://forums.developer.nvidia.com/t/illegal-instruction-core-dumped-error-on-jetson-nano/166113).
How to Identify and Solve Illegal Instruction( Core Dumped) Error
1. Crosscheck the Target Architectures:
Verify that the architectures of your compiler’s target and your running environment are compatible. You can print out your system’s architecture using the
`uname -m`
command. If the binary was compiled for a different architecture, you might need to recompile it specifically for the Jetson Nano’s architecture.
2. Recompile Your Code:
If you’re compiling your own code, try recompiling it with debugging information enabled, as this can provide additional insight into the problem. To do this, add the
`-g`
flag during compilation:
`gcc -g my_program.c -o my_program`
3. Use a Debugger:
Use a debugger such as gdb (GNU Debugger) so you can inspect the state of the program at the moment the illegal instruction is executed. With gdb you can also get a backtrace of the call stack, which could be very helpful. Here’s how to use gdb to perform these tasks:
` $ gdb my_program (gdb) run
Wait until the illegal instruction error happens, then type:
` (gdb) bt `
This will display the backtrace, showing exactly where in your code the error occurred.
4. Inspect the Core Dump:
By inspecting the core dump, you might gain additional insights about the state of the application when it crashed. The contents of a core file can be viewed using the debugger with
`gdb my_program core`
.
Please note that for generating a core dump, you may have to set the core file size to unlimited using the command
`ulimit -c unlimited`
before running your program.
These strategies will help to identify the root cause of ILLEGAL INSTRUCTION (Core dumped) errors on a Jetson Nano giving line numbers and sources of the buggy areas of your code. Throughout this process, ensure the code being debugged complies with the hardware architecture needs of your NVIDIA Jetson Nano.
References:
The term “core dump” in computing refers to the process where a program’s memory contents are written out to a file when an unexpected event, like an “Illegal Instruction” error, occurs. Jetson Nano, with its advanced GPU architecture, can sometimes face similar errors. Here, I will compare common solutions for the “Illegal Instruction (Core Dumped)” error found on Jetson Nano.
Debugging
Essential for any software developer, debugging is usually the first thing you should try to understand the source of the problem. You can debug your application using a tool such as GDB – GNU Debugger. GDB allows you to see what is going on ‘inside’ another program while it executes.
gdb your_program run your_arguments
Detailed information about the instruction that caused the illegal specification will start to appear once the crash has occurred within gdb. This includes the stack trace, which may be insightful in understanding exactly where the error occurred in your code and why.
Trying Specific Compiler Flags
Depending on the circumstances, using specific compiler flags can fix this error. For instance, GCC provides the -march and -mtune flags, which may come into play like:
gcc -march=native -mtune=native -o output_file your_program.c
This ensures that the generated code is specially optimized for the host computer, producing fewer Illegal Instruction errors in most scenarios. Although not every issue might be solved by this alteration, it’s worth trying if other methods haven’t previously succeeded.
Updating or Downgrading Packages
Sometimes there can be compatibility problems leading to these kinds of errors. Checking for updates or downgrading the problematic package can solve the problem. For this solution, different commands can be used to provide information about outdated packages:
pip list --outdated conda outdated
If an update is available for a package, use pip to update it:
pip install --upgrade packageName
If downgrading is needed:
pip install packageName==versionNumber
Recompiling the Program
Sometimes, simple recompilation makes the difference. An Illegal Instruction error could occur when a binary was compiled on a machine with a different CPU feature set than the current CPU supports. It might also be the solution in the case where 64-bit applications are attempted to run on older architectures without 64-bit support.
make clean make all
This will first remove files produced by previous compilations and afterward compile everything from scratch.
Conclusion
In summary, depending on the specific situation that triggers the “Illegal Instruction (Core Dumped)” error, the above methods can potentially resolve the issue. It’s always recommended to have a backup strategy for data recovery since core dump files might contain sensitive data.
In the realm of programming, unexpected behavior or undesirable performance might result from code that has illegal instructions. The Jetson Nano device, from Nvidia, is not exempt from this peculiar situation, just like any other software or hardware tool in the technology industry.
The term “Illegal Instruction (Core Dumped)” is indicative of a kind of error specific to certain processors, as is the case with the ARM processor of the Jetson Nano. For enhanced context, a
core dump
refers to the recording of the working memory of a computer program at a specific time, generally when the program has crashed or otherwise terminated abnormally.1
The illegal instruction can be due to several reasons:
- Running a binary compiled for a different architecture.
- Executing a corrupted binary that contains nonsensical instructions.
- Stack corruption causing your app to jump to a random address.
On the Jetson Nano, it’s usually related to incompatible or incorrectly updated packages, especially if dealing with complex installations such as those required by data science and machine learning libraries. For example, updating Numpy to its latest version may cause the error primarily because the newer versions may not be compatible with the architecture on which the Jetson Nano runs.
When considering program updates’ impact on illegal instructions errors, one fact comes out on top: any update or upgrade made must always consider the architecture against which the software was initially built and intended to work on.
When you fetch updates from online repositories, these are typically compiled for common architectures. Unfortunately, Jetson Nano’s ARM architecture might not be fully supported or tested against all possible scenarios there since it’s less common than, say, x86. This inevitably leads to compatibility issues and improperly working instructions – aka ‘illegal instructions’.
How do we resolve these issues? Here are a few strategies:
Firstly, use software that’s guaranteed to be compatible. That means using libraries and tools specifically compiled for ARM architecture, or tools known to work universally.
Secondly, when you want to upgrade a package, remember the potential risk involved.
If you’ve received a library or software bundled with the Jetson system image or from Jetson’s SDK Manager, there’s a good chance it’s been specifically designed or tested for their platform and deviation from it would entail some level of risk. Upgrading should then be done consciously, with backup steps in place and a readiness to possibly have to rollback to the initial version.
A handy preventative strategy includes version pinning where you explicitly set and use specific versions of libraries to shield yourself from potential compatibility issues arising from upgrades.
Consider the Pandas python library:
pip install pandas==1.1.5
This ensures you’re installing a specific version, reducing the chance of encountering unexpected “illegal instruction” errors after an update.
To summarize, caution needs to be exercised while handling program updates especially when working on unique platforms such as the Jetson Nano. Recognizing the compatibility limitations inherent to its ARM architecture and putting mitigation measures in place is key to minimizing the incidence of illegal instruction errors.The “Illegal Instruction (Core Dumped)” error on Jetson Nano is a frustration many developers dealing with NVIDIA’s ecosystem have faced. This cryptic message often leaves us with more questions than answers. Unveiling the reason behind and resolving this glitch requires understanding the concept of ‘library mismatch’ which notably triggers this error.
Let’s dive deeper into the complex world of libraries that make our applications function seamlessly and explore where mismatches could occur leading to these infamous ‘core dumped’ errors.
In simple terms, a library in the context of programming is a set of pre-compiled pieces of code that we can reuse in our programs. When referring to a ‘library mismatch’, we’re talking about occasions where the version of the library used at compile/run time isn’t compatible with the one that was anticipated.
How does this lead to an “Illegal Instruction(Core Dumped)” error on Jetson Nano? This occurs when your application attempts to execute an instruction it doesn’t understand, possibly because there’s a mismatch between the libraries used during compile-time and run-time. Hence, the ‘illegal instruction’ ends up causing the ‘core dumped’ error.
Let’s consider an example:
Assume you’ve built an application on one machine and then transferred it over to the Jetson Nano device. The core dumped error could surface if there are any inconsistencies in library versions across different devices. Any change, minor or major, could cause differences in execution behavior.
Seeing how we use a vast number of libraries while developing applications, various mismatches could trigger. Here are some common scenarios through which a library mismatch may arise:
- The application was compiled with a newer version of a library, but an older version is present on the system during runtime.
- Conversely, the application was compiled with an older version of a library, but a newer version exists on the system during runtime.
- A library that the application depends on has been removed or uninstalled from the system.
Each of these instances can potentially lead to undesired consequences such as the “Illegal Instruction (Core Dumped)” error.
Before we start working towards solutions, it’s important for us to confirm that this indeed is triggered by a library mismatch; we need to isolate the problem. Running our program under gdb debugger can help with this, for instance:
gdb ./program_name run
Through debugging, we can identify what instruction caused the core dump and validate if it stems from library related issues.
Fixing library mismatches involves ensuring that your development and deployment environments utilize the same versions of necessary libraries. One way of guaranteeing this is adopting containers, such as Docker, wherein all your library dependencies are embedded in the container itself.
This strategy lets us build reproducible environments – think about it as setting a controlled experiment. Regardless of whether the container runs on your development machine or the Jetson Nano, library versions remain consistent.
However, do remember, this will only mitigate issues arising out of library mismatches, not eliminate a complete possibility of running into “Illegal Instruction (Core Dumped)” error. It’s important to follow good coding practices, routinely test your code across diverse environments and learn from any hiccups encountered on the path of programming righteousness.
For a lot more detail and comprehensive insights to deal with core dumping, I highly encourage checking out NVIDIA’s Developer Forums, particularly their Jetson & Embedded Systems. This platform harbors numerous programming war stories, bringing together best hands-on practices followed by fellow developers around the globe.
Encountering the “Illegal Instruction(Core Dumped)” error on a Jetson Nano is a symptom of an underlying issue related to the operating system (OS) architecture compatibility. It can be caused by multiple factors such as processor incompatibility, misuse of compiler instructions or faulty software installation.
Processor Incompatibility
Jetson Nano utilizes an ARM-based processor specifically designed by NVIDIA that is highly optimized for low-power environments and machine learning applications. Sometimes, if you’re trying to run pre-compiled binary files which are built for different architectures (like Intel’s x86), you may stumble upon the “Illegal Instruction(Core Dumped)” error. This could also arise from libraries or dependencies that are not compatible with the device’s architecture.
file /usr/lib/aarch64-linux-gnu/libm.so.6
This command inspects architecture of a file/lib, if result doesn’t return aarch64 (ARM), then it is incompatible with the hardware. If this is the case, it might be necessary to rebuild these binaries with the correct architecture settings. Or find ARM-compatible ones.
Misuse of Compiler Instructions
As a programmer, you may accidentally use certain compiler options that do not align with the target architecture’s specifications. For instance, if you compile with CPU instruction sets that are not supported on the Nano’s ARM architecture, executing the resulting binary will throw an Illegal Instruction error.
g++ -c -march=native source.cpp // Replace "-march=native" with appropriate flag for ARMv8-A series CPUs
Compiling source code on a platform other than the target platform (cross-compiling) often induces this kind of problem. Be sure to use the right flags that comply with your processor’s instruction set.
Faulty Software Installation
In some cases, installing software packages via apt or pip, for example, might also give rise to the error. They install prebuilt packages that may not be compatible with your current OS configuration. Building those packages from source can solve the problem.
In any case, resolving this error involves close inspection of the build process or even rebuilding some parts of the OS from scratch. Debugging tools like ‘gdb’ or ‘strace’ are quite useful to pinpoint the exact location of the fault.
Debugging the Error with GDB
GDB is one the most widely used debugging tools in linux systems and allows us to dive deep into the core dumped file by running :
gdb your_program core
Once inside the GDB shell, enter:
backtrace
This will display a list of function calls leading up to the illegal instruction, allowing you to observe where exactly the program went off track.
References :
- For more details on understanding and debugging core dump files : Core Files – Linux man page
- NVIDIA Developer Forum thread with solutions for similar issues : Illegal Instruction (core dumped) for Jetson Nano Developer kit
When you are working with an NVIDIA Jetson Nano, experiencing an Illegal Instruction(Core Dumped) error can often be the consequence of a crucial instruction in your application code trying to execute a processing capability which is not supported by the system’s GPU or CPU. This anomaly flags off as an ‘illegal operation’, and thus the process causing this issue is promptly terminated to safeguard the system, triggering the core dumped message.
Let’s divide the problem into two main categories:
• Your software package executing unsupported instructions • Incompatibility between cross-compiled code and the target machine
1. Your Software Package Executing Unsupported Instructions
A Jetson Nano employs an ARM Cortex-A57 processor along with a Maxwell architecture-based GPU. When a packaged software application tries to run an order which exceeds these components’ intrinsic features, it encounters the ‘Illegal Instruction (Core Dumped)’ error.
Take a look at the following instance as an example,
Illegal instruction(Core dumped) error when running TensorFlow on Jetson Nano.
Here, TensorFlow, a popular deep learning library, could be using advanced SIMD(single instruction, multiple data) instructions like AVX2 or FP16 not supported by Jetson Nano’s GPU. As a result, upon hitting any such non-supported capability, Kernel intervention halts the execution – signaling it as an illegal operation.
To circumvent this, you may need to compile your code or packages specifically for the ARM A57 processor and the accompanying Tegra X1 Maxwell architecture GPU. This might involve trimming down certain features or fine-tuning optimization settings that coincide with the capabilities of your hardware Jetson Zoo.
2. Incompatibility Between Cross-Compiled Code And The Target Machine
Many times, we cross-compile our software on one machine (like an x86 desktop) to run it on a different architecture (like ARM in the case of Jetson Nano). This often leads to Illegal Instruction errors due to inherent disparities in the instruction sets supported by the source and target machines.
A key point here would be cross-compiling your code using the correct toolchain and making sure the compilation options reflect the architecture of your target machine (Jetson Nano) and not of your host machine (the system where you’re actually compiling the code).
NVIDIA Developer provides extensive materials and tutorials to guide you through the process of setting up your environment for Jetson Nano.
A common trick is to ensure installation from source, adding appropriate flags during your compilation step such as
-FP16 or -MAVX2
However, care must be taken as wrong flags can lead to more severe errors or degraded performance.
The Illegal Instruction (core dumped) error tends to emphasize a significant mismatches blip, emphasizing a critical examination of how you’ve compiled your code against the precise hardware capabilities of your system. Performance implications can be multifold: slowed processes due to constant checking for legal instructions, memory allocation failures, unnecessary device interruptions, and reduced overall efficiency. Meaning timely resolution and prevention hold importance in maintaining optimal system performance.The Jetson Nano, provided by NVIDIA, is a small yet versatile platform that can run massive computations. However, it’s not immune to errors and issues such as the Illegal Instruction (Core Dumped) error.
Understanding what ‘core dumped’ error means is crucial for effective troubleshooting. In essence, when a program crashes because of an unhandled error—like an illegal instruction—it often generates a core dump. This dump which offers a snapshot of the system memory at the time of failure is useful in diagnosing the issue.
There could be several causes behind this error, from incorrect binary executions, insufficient memory resources to software incompatibilities. The ways to diagnose and fix this particular error depend on your specific context.
Utilizing GDB Debugger:
One general approach in exploring this problem would involve using the GNU debugger (GDB) tool. It allows you to examine the core dump in detail and potentially highlight where things have gone wrong.
Here’s how:
$ gdb executable-file core-file
This command initiates GDB and loads both the offending executable and the generated core file.
Once loaded, you can use the ‘bt’ (backtrace) command within the GDB session:
(gdb) bt
This outputs a stack trace, highlighting where, in your code or your executed binary, the program encountered the illegal instruction causing the crash.
Inspecting Your Code:
Conversely, if you’re developing your own software for the Jetson Nano, consider going through your code again, particularly around areas where the debugger is flagging the illegal instruction. You may find that you are trying to execute an instruction not supported by the Nano’s hardware.
Docker Considerations:
It’s likewise worth mentioning that if you’re using Docker, there might be situations where the Docker environment pulls an image built for the wrong architecture leading to the ‘Illegal Instruction’ error. Ensure that you’re using images compatible with the ARM64 architecture that the Jetson Nano uses.
Overclocking Related Issue:
Lastly, remember that deep learning applications are resource-intensive. It’s plausible to experience such an error if the Nano is being overclocked or overheated. That is, it’s running beyond its standard operational parameters.
In closing, while these community suggestions cover various angles for tackling the Illegal Instruction(Core Dumped) Error On Jetson Nano, the specifics of your situation drive which solutions may be most appropriate.Understanding the illegal instruction(core dump) error and preventing its recurrence involves managing hardware-incompatible instructions, updating software libraries, or utilizing appropriate debugging tools. This article will particularly focus on how to handle such errors when working with NVIDIA Jeston Nano development kits.
Illegal Instruction (core dumped)
signals that the executing program attempted an operation for which no instruction exists in the CPU’s command set. This might occur due to hardware compatibility issues or obsolete software libraries.
Hardware Incompatibility
Sometimes, running code compiled for different or newer architecture versions on your Jetson Nano, a machine based on ARM Cortex-A57 architecture, might lead to
Illegal Instruction
core dump. A good instance of this is attempting to run a program compiled using x86-64-based instructions set on the ARM-based CPU.
The measures to counter this issue involve:
- Make sure you’re using or writing code compatible with the ARMv8-A instruction set.
- If using pre-compiled code, ensure the binary is intended for ARM-based architecture, rather than x86.
Obsolete Libraries
Another prominent cause of
Illegal Instruction
core dump could be incompatible or outmoded software libraries. The Jetson Nano uses L4T (Linux for Tegra), and if certain libraries are outdated relative to the running application, it may result in an illegal instruction.
Two steps to prevent this problem are:
- Ensure all your dependencies and libraries are up-to-date.
- Recompile the libraries and build them from the source if the issue persists, ensuring that they’re specifically suited for the Jetson Nano architecture.
Debugging Tools
To prevent recurrence, employ efficient debugging tools that help detect such errors before running the complete program. GDB – The GNU Debugger tool can come handy here.
To troubleshoot illegal instructions errors with GDB follow these steps:
- Start by compiling your program with debug information. Use gcc’s -g option:
gcc -g -o program program.c
- Run the program under control of gdb:
gdb ./program
- In gdb’s interactive mode, use the
run
command to start the program.
- When the problematic instruction hits and the program crashes, use
x /i $pc
to inspect the assembly level instructions at the crash point.
Compiler Flags
In some cases, tweaking with compiler flags and optimizing for specific architectures during code compilation might give rise to such errors. Make sure not to aggressively optimize your code for Jetson’s specific chip.
Modify the optimization level with:
-O or -O1 or -O2 or -O3
Also verify that the machine instruction set name “native” isn’t being used under the
-march=native
flag, as it doesn’t cross-compile well.
In summation, make sure your code or pre-compiled binaries are compatible with the Jetson Nano’s hardware architecture, update your libraries often, debug efficiently with tools like GDB, and watch your compiler flags for aggressive optimization settings that might trigger those nerve-racking
Illegal Instruction (core dumped)
errors.
Further Reading:
Developers Corner, Nvidia Forum: https://forums.developer.nvidia.com/
GNU Debugger: https://www.gnu.org/software/gdb/Getting to grips with an “Illegal Instruction (Core dumped)” error on the Jetson Nano can be challenging, but with a calculated approach, it can be dealt with effectively. Let’s discuss how this issue could occur, how to decode these cryptic termination messages, and strategies for mitigating them.
So, what exactly does an “Illegal Instruction (Core dumped)” error denote? Essentially, it suggests that your software is attempting to perform an operation that isn’t supported by your particular CPU architecture. Notably, the Nvidia Jetson Nano runs ARMv8-A 64-bit CPU architecture, meaning all software running on it must support this architecture explicitly.[1]
If you run into this error while using Python libraries like TensorFlow, NumPy, or SciPy on your Jetson Nano, the likely reason is that these libraries contain binary components compiled for a specific CPU architecture and unfortunately they’re not compatible with the Nano’s ARMv8-A.
You can confirm if this is the issue by exploring the core dump file itself. Following
gdb
command is of imperative importance:
gdb -c /path/to/corefile
This will give a full backtrace of events leading up to the crash which might look similar to:
Program terminated with signal SIGILL, Illegal instruction. #0 0x00007f0418214f90 in ?? () (gdb) bt #0 0x00007f0418214f90 in ?? () #1 0x00000000004008b9 in main ()
Therein, you see a series of function calls leading up to the fatal signal, which can help shed light on the root cause of the problem inherently. If the first item in the backtrace (
#0
) points to a library or shared object (.so) file that doesn’t match the Jetson Nano’s CPU architecture, there’s your answer.
To fix the issue, you can take two possible steps:
* Check if precompiled binaries or newer versions of the problematic library are available explicitly for the ARMv8-A architecture.
* Alternatively, consider compiling the problematic library from source codes right on your Jetson Nano.
Consider, for instance, installing the GPU-optimized versions of the Python packages straight away from Nvidia’s pip repository, which includes binaries compiled especially for the Jetson’s architecture:
$ pip install --extra-index-url https://developer.download.nvidia.com/compute/redist nvidia-dali-cuda100
In case of needing to compile from source, instructions would differ depending on the library. But typically, they involve cloning the library’s source code from its GitHub repository and building it with appropriate build tools. An example for TensorFlow[2] –
$ git clone https://github.com/tensorflow/tensorflow.git $ cd tensorflow $ ./configure $ bazel build //tensorflow/tools/pip_package:build_pip_package
While potentially time-consuming, compiling from source directly on your Jetson Nano ensures that the binary components generated are tailored to its ARMv8-A CPU architecture, thus resolving the “Illegal Instruction (Core dumped)” error efficiently and professionally. To avoid future errors, always confirm that any libraries you install are compatible with your system’s architecture specifically.
SEO Keywords: Jetson Nano, ARMv8-A, “Illegal Instruction (Core dumped)” error, Python libraries, CPU architecture compatibility, precompiled binaries, source code compilation.
The “Illegal Instruction (Core Dumped)” error on the Nvidia Jetson Nano is usually traced back to three predominant factors: incompatible architecture, outdated/unsupported libraries, or binary file corruption. Understanding these fundamental issues opens us up to a plethora of solutions.
Issues | Solutions |
---|---|
Incompatible Architecture | Ensure that you are running software compiled for ARM64 architecture as other types may not be supported. |
Outdated / Unsupported Libraries | Always verify your library versions and ensure they are updated, especially python libraries; it’s best practice to maintain them. |
Binary File Corruption | Check any executable or binary files involved. Corrupted files will need to be replaced or repaired. |
However, the most trusted way to counteract the issue is by confirming your dependencies’ compatibility carefully better upfront. Be aware of what software versions you’re using during the building and deploying of applications onto your device. To check this, use the following command:
$ lscpu
This command provides details on the Central Processing Unit architecture. Proper implementation involves ensuring the onboard coding setup aligns with that.
Also, keep in mind that open source communities such as Nvidia developers forum could offer help when troubleshooting generally uncommon problems like this one. Moreover, clean system reinstallation might seem like an extreme fallback solution, but don’t eliminate it from your tactical toolbox. It invariably helps get things back on track when everything else failed to produce a solid result.
Responsibility comes down to you as the programmer to observe due diligence; keep abreast with newer practices, innovations, and even challenges arising within the software ecosystem. From employing recommended techniques to ensuring regular system, architecture and software updates, it all plays into the maintenance of a smooth-running operation without ever bumping into ambiguous errors like the “Illegal Instruction(Core Dumped)” on Jetson Nano.