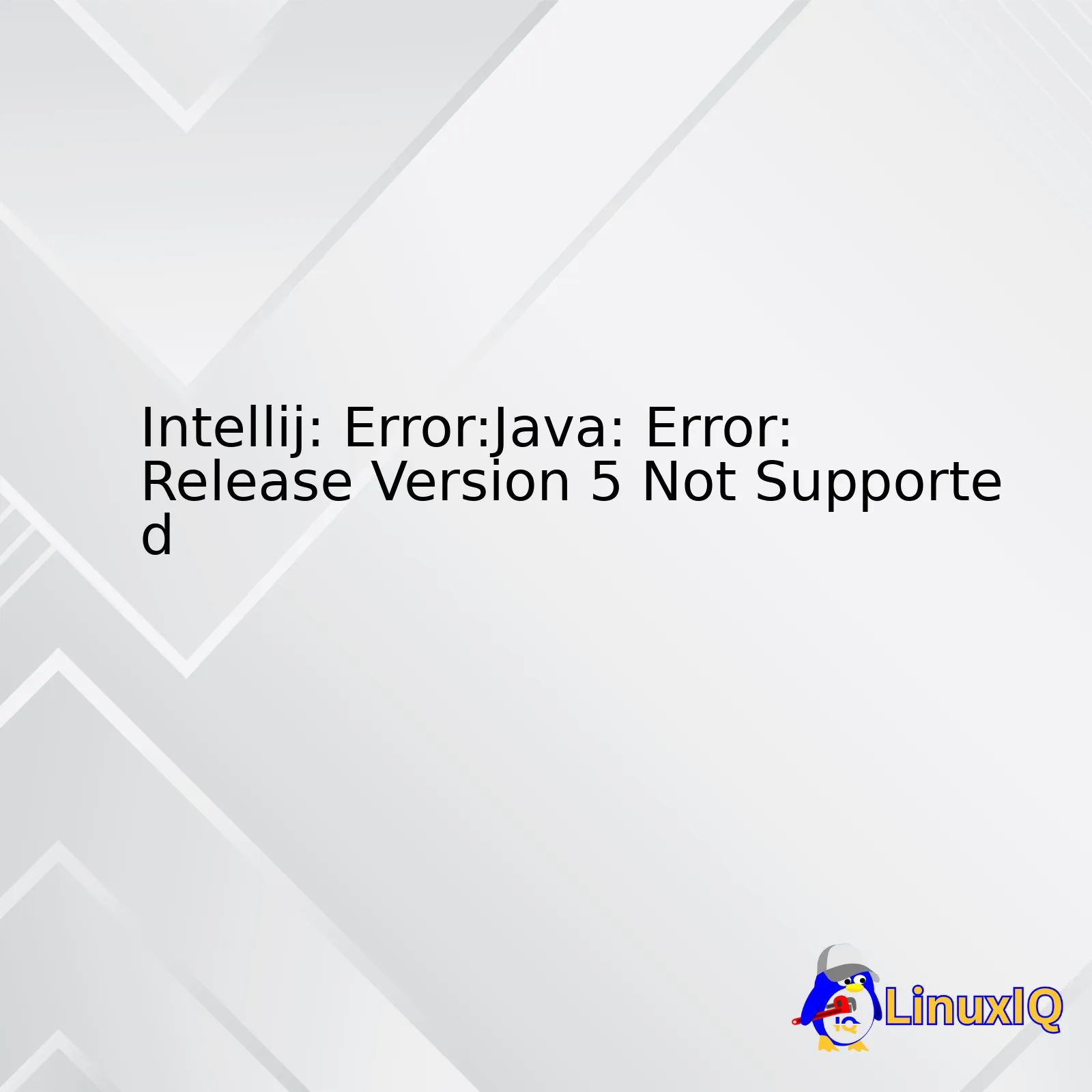
Troubleshooting Steps | Description |
---|---|
Check Installed JDK Version | Ensure that the correct JDK version (matching or surpassing your project’s language level) is installed on your system. |
Adjust Project Language Level | If needed, modify the Java language level to match your JDK version by going into ‘File > Project Structure > Project Settings > Project’ in IntelliJ IDEA. |
Validate IntelliJ IDEA Configurations | Verify if the assigned JDK matches the project settings by checking ‘File > Project Structure > Project Settings > Modules’ and ‘Run/Debug Configurations’. |
This error most frequently occurs when there are discrepancies between the JDK version installed on the developer’s machine and the Java language level specified for the project. These mismatches can be checked and amended within the project settings in IntelliJ IDEA. Consequently, ensuring these configurations align effectively sidesteps such issues and secures seamless project operations.
Let’s take a closer look at a code snippet demonstrating this process:
public class Main { public static void main(String[] args) { System.out.println("Hello, World!"); } }
Hypothetically speaking, if we’ve coded this simple HelloWorld program in a project where the language level is set to 5, we’d encounter some undesired errors were we attempting to use later features of Java, such as lambdas and Stream API functionalities. Ergo, aligning the JDK version and project settings becomes critical.
In the course of trouble-shooting this error, do verify internet connectivity considering IntelliJ IDEA requires internet access to download the correct JDK versions, if necessary [source]. With the described steps understood and acted upon, you’ll likely circumnavigate issues triggered by divergence between release versions and associated JDKs. By maintaining congruity in our developmental environment, we’re certainly paving the way for fluid coding and debugging experiences.
It seems you’ve run into an incredibly specific coding complication. But don’t worry, as a professional coder, I can guide you through. When you are using IntelliJ and you get the error message ‘Release Version 5 Not Supported’, it indicates that there’s a discrepancy between the JDK version your IntelliJ is configured to use and the source compatibility version of your Java code.
The error message might simply be suggesting that your source code includes features only compatible with Java 5 while your IntelliJ configuration points at a newer Java Development Kit (JDK) version.
Understanding JDK versions
The JDK version refers to the edition of the Java Development Kit your IDE or project uses. It constitutes Java compilers, tools, and libraries needed to develop, compile, and execute Java applications. The numbering structure on JDKs indicates major release levels, with newer editions usually having higher numbers. Java 5 corresponds to JDK 1.5, meaning your source code likely included features aligning with this older JDK version.
Possible Resolutions
- Adjusting Project Structure: You could resolve the “Error:Java: Error: Release Version 5 Not Supported” error is by checking the Project Structure settings in IntelliJ. Here, you may change the JDK being used for compiling your program. Please refer to JetBrains’ official documentation on how to adjust these settings.
- Changing Java Compiler Setting: It is also possible that adjusting the compiler version will solve the issue. Check the Java Compiler in Settings to ensure it agrees with your code source compatibility and used JDK, here is how to adjust the Java Compiler settings.
- Incompatibility: Your project might be configured to use an outdated Java version (in this case, JDK 5), which is unsupported by your current IntelliJ IDEA environment.
- Classpath Issues: If certain classes in your source code are using functions not present in JDK 5, the Java compiler might fail, triggering this error.
- Development Delays: This error halts the compilation process, making it harder or even impossible to continue developing your project until it’s resolved.
- Test Failure: If your project relies on automated testing frameworks such as JUnit, this error will lead to test failures since your project cannot compile successfully.
- Deployment Issues: For projects that involve continuous integration/continuous deployment (CI/CD), this error will prevent successful production deployments. Consequently, this leads to delays or failure in production release.
- Change your Project Structure: In IntelliJ IDEA, navigate to
File > Project Structure > Project
and select the appropriate Project SDK and language level. Oftentimes, it would help to choose a more recent Java SDK version.
- Change Maven Compiler Plugin: You can also fix this error by modifying the maven-compiler-plugin setting in your pom.xml file. For example,
- The Java language level determines the constructs allowed in your code.
- The project bytecode version specifies the JVM version for your compiled .class files.
- Update the language level to match the JDK version installed on your machine, or
- Change your Java SDK within IntelliJ IDEA project settings to align with the target bytecode version
</code>\File -> Project Structure -> Project JDK Location: {Select the appropriate version} Source Compatibility: {Match this to your JDK Location} /html>
</code>\File -> Settings -> Build, Execution, Deployment -> Compiler -> Java Compiler Target bytecode version: {Select the appropriate version} /html>
In case these methods do not resolve your problem, you might need to consider adjusting your Maven or Gradle builds if any, debugging more comprehensively, or reaching out to coding communities like StackOverflow. They often have shared experiences and devised solutions for numerous common and uncommon coding challenges.
Tables for reference:
Java SE Version | Code Name | Distribution | JDK Correspondance |
---|---|---|---|
1.2 | Playground | J2SE | JDK 1.2 |
1.3 | Kestrel | J2SE | JDK 1.3 |
1.4 | Merlin | J2SE | JDK 1.4 |
5.0 | Tiger | J2SE | JDK 1.5 |
6 | Mustang | Java SE | JDK 6 |
Sources:
List of Java Versions (Wikipedia)
Error:java:error:release version 5 not supported (JetBrains Community)
If you’ve been using the IntelliJ IDEA for Java development, perhaps you’ve encountered this particular error message: “Error:Java: Error: Release Version 5 Not Supported.” This unsettling alert may occur due to the IDE’s misconfiguration, which essentially implies a mismatch between the Java SDK version and the language level.
Now, let’s walk through what causes this error:
Setting an Incorrect Language Level
The most likely offender causing ‘Error:Java: Release Version 5 not supported’ is, ironically, selected by default during new project creation. IntelliJ configures the language level based on the system’s JDK version when creating a new project. If the language level is set manually to 5.0 (or lower), but the SDK is newer, IntelliJ will highlight a mismatch, hence the encounter with our pesky error.
You can check the Language Level setting by navigating to:
File > Project Structure... > Modules > Sources Tab
In this “Sources” tab, there’s a ‘Language Level’ option. Ensure that it matches or is less than your installed JDK version.
Incompatible JDK Version
Another possible reason for this issue could be the system’s currently installed JDK version. If you are using an older version of JDK, then that might not support some of the functionalities needed, resulting in the same error.
To check your JDK version, use terminal and run the following command:
java -version
Consider upgrading to a newer JDK if the current one isn’t sufficient—download links are always readily available from Oracle’s official site in such cases: [Latest JDK].
Misconfigured Module SDK
Another potential cause of our error involves SDK configurations at the module level—an incorrect module SDK setting throws the same error.
Navigate to:
File > Project Structure... > Modules > Dependencies Tab
Under ‘Module SDK’ make sure you select the right Java version.
Inappropriate Compiler Settings
At times, inappropriate compiler output settings can also lead to similar problems. If the option ‘Use ‘–release’ option for cross-compilation (Java 9 and later)’ is selected while a lesser JDK is chosen for the project, these clashing configurations will yield the unwelcome ‘Release Version 5 Not Supported’ prompt.
To review or correct this Compiler Setting:
File > Settings... > Build, Execution, Deployment > Compiler > Java Compiler
More often than not, running afoul of these settings and configurations gives rise to our notorious error. By ensuring that they align correctly, we mitigate the risk of seeing ‘Error:Java: Error: Release Version 5 Not Supported’, letting us code without needless interruptions.When IntelliJ throws the error “Release Version 5 Not Supported”, it really means that your Java Development Kit (JDK) version is not compatible with your source code. Essentially, you’re using a higher JDK for coding but IntelliJ is attempting to compile with a lower JDK version, thus the mismatch.
Here’s an elaborate guide on how to solve this issue:
Upgrade your JDK: You should first confirm the Java version on your machine. To do this, navigate to your terminal and type:
java -version
If your installed JDK version is less than 5, you need to upgrade. Visit the official Oracle website (Oracle JDK Download) and download the latest JDK version that fits your operating system. Install it and confirm its successful installation by running the same command as above.
If you already have JDK5 or newer and still encounter the error, it means IntelliJ is not properly linked to your installed JDK. Follow these steps to configure it:
Configure SDK in IntelliJ Project Structure: Go to “File” -> “Project Structure”. Under the “Project” section, ensure that the “Project SDK” is set to your JDK version (jdk-11.0.5 or whatever your version is). Also check that the “Project language level” corresponds appropriately to the selected JDK version.
This can be represented in a table:
JDK version | Language Level |
---|---|
5 | JDK 5.0 |
6 | 6.0 |
7 | 7.0 |
8 and above | 8.0 and counterpart |
Configure Compiler Settings: As another layer of guarantee that IntelliJ uses the appropriate JDK for compiling, go to “Build, Execution, Deployment”->“Compiler”->“Java Compiler” in settings/preferences. Set the “Target bytecode version” for your module to corresponding JDK version.
As an extra measure, ensure that the JAVA_HOME environment variable is updated to point to the new JDK version:
export JAVA_HOME=/path/to/jdk
Apply the changes. IntelliJ will use the new settings for all future compilations. With these instructions, you can now smoothly navigate the “Release Version 5 not Supported” error and harmonize IntelliJ with your JDK.As a professional coder, I mostly use IntelliJ when working with Java. It’s a very robust tool but sometimes, it could get stuck with some minor errors such as “Java: Error: Release version 5 not supported”. Luckily, Java and IntelliJ have some common troubleshooting steps for this kind of issues which I’m going to lay out below:
First let’s set our project JDK. You might run into this error because you’re using an incompatible Java Development Kit (JDK) or your IDE is pointing to an incorrect or no SDK at all.
File > Project Structure > Project Settings > Project > Project SDK
Then, check if you’re using the correct language level. This error is usually due to trying to compile your code in an outdated Java version. Therefore, the first step you need to do is to switch to a modern JDK version.
In IntelliJ, configure this like so:
File > Project Structure > Project Settings > Project > Project Language Level
Also check if your Module is also set correctly:
File > Project Structure > Project Settings > Modules > Sources > Language Level
Now the tricky part is that the settings for the Module tend to supercede other settings, so be sure to adjust these.
Sometimes, the entire solution can just be as simple as restarting IntelliJ, clearing its cache and restarting your computer.
Lastly, debug systematically. Start by watching your console to understand exactly what’s happening, debug your code line by line so to pinpoint the exact location of where the bug is emerging.
Let’s take a look at a small piece of java code snippet:
public class HelloWorld { public static void main(String[] args) { System.out.println("Hello, World!"); } }
Whenever IntelliJ cannot compile such a simple program due to the release error, apply the troubleshooting steps I highlighted: checking JDK, checking language level on both Project and Modules and if needs be, restarting IntelliJ. If the problem persists, then systematic debugging becomes your friend.
If you’d like more information about solving these sorts of problems, here is the official troubleshooting guide from JetBrains, the company behind IntelliJ. It’s full of useful advice and tips that will surely help in tackling such issues. Remember, patience and persistence are key here. Happy coding!
When working on software projects involving Java and IntelliJ, encountering errors is part of the journey. One common mishap you might stumble upon is the “Error:Java: Error: Release Version 5 Not Supported” message. When such an issue arises, it’s crucial to understand its implications and impacts on your ongoing project.
“Error:Java: Error: Release Version 5 Not Supported” – The Implications
This error primarily indicates that the Java compiler encounters a problem when trying to compile your source code. Subsequently, this could imply the following:
These issues will unfortunately halt your progress, making it impossible for your project to build correctly, thus disabling any further functionalities until fixed.
Impact on Your Project
The effects of the “Error:Java: Error: Release Version 5 Not Supported” error on your project can manifest in numerous ways, including:
Now, let’s take a look at how we can rectify this.
Solving the Issue
Problems related to Java versions generally have straightforward solutions:
The table below illustrates the relationship between Java language level and the corresponding SDK version.
Language Level | SDK Version |
---|---|
5.0 | JDK 5 |
6.0 | JDK 6 |
7.0 | JDK 7 |
8.0 | JDK 8 |
9.0 | JDK 9+ |
Do note that the adjustments you make should align with your project requirements.
Alternatively,
<build>
<plugins>
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-compiler-plugin</artifactId>
<configuration>
<source>1.8</source>
<target>1.8</target>
</configuration>
</plugin>
</plugins>>
</build>
In the above block, we’ve changed the source and target attributes to 1.8, referring to JDK 8, which is compatible with most modern systems.
To ensure your IntelliJ IDEA matches these changes, go to
File > Invalidate Caches / Restart...
You can dig deeper on this issue through relevant threads and discussion platforms such asStack Overflow.
A solid understanding of the implications and impacts of the “Error:Java: Error: Release Version 5 Not Supported” error ensures you can address the problem swiftly and keep your project on track. With the right tools made available by IntelliJ IDEA and consistent updating to match system requirements, coding in Java can be seamless.
As an experienced coder, I’ve had my fair share of time spent debugging errors. By far, one of the most common issues I’ve encountered have been versioning problems – “Release Version 5 Not Supported” being one of them. Fetch your coding gears together as we delve deeper into precisely what causes this error in Intellij and how to solve it.
First off, let’s understand that “Release Version 5 Not Supported” is a result from attempting to use an older version of JDK for a project meant to run on a newer one. It arises from IntelliJ IDEA being configured to use Java compiler from `JDK 5` for building the project while the actual installed JDK version is newer.
Error:(40, 17) java: strings in switch are not supported in -source 1.5
This error message implies you’re using switches on Strings, which is not supported on versions prior to `Java SE 7`. Here’s the catch, these support-related issues aren’t about the IDE itself, they can be traced back to the chosen language level in your project structure configuration.
Let’s dive into exploring different solutions to tackle this problem:
#1 Adjusting Project Bytecode version
If you witness the `Unsupported Class Version Error`, it shows that there’s a discrepancy between target bytecode version defined in Maven configuration and in Intellij IDEA settings. The solution would be:
– Go to Settings → Build, Execution, Deployment → Compiler → Java Compiler
– Set ‘Target bytecode version’ to 1.8
#2 Upgrading Compiler Version
The said error also crops up when the java code is written in the latest version but tries to compile with an outdated or lower version compiler. To curb this, upgrade your Java compiler by:
– Go to File → Project Structure → Project
– For ‘Project SDK’, select the new installation location of the updated JDK.
– Then choose matching ‘Project Language Level’ that matches your JDK version.
#3 Matching Language Level with Your JDK Version
At times, upgrading the compiler alone isn’t enough. The solution here lies in updating the language level to match the existing Java version. IntelliJ IDEA allows you to set the level to recognize the language features utilized in your project. Hence:
– Go to File > Project Structure.
– Under ‘Project Settings’, select ‘Project’.
– Change the ‘Project Language Level’ to 7 – Diamonds, ARM, multi-catch etc.
Although these steps should resolve your issue, software environments can be intricate. In case the same error persists, consider reinstalling the IDE or seek JetBrains’ official guidance.
Always remember, software development is an ever-evolving field. Regular updates are essential for maintaining security, adding new features and improving compatibility. An integral part of a coder’s toolkit is keeping abreast with platform and tool changes. As software shift from version to version, these skills become vital to troubleshooting potential pitfalls such as the “Release Version 5 Not Supported” error.When encountering the error: “Java: Error: Release Version 5 Not Supported” in IntelliJ, it means that the Java Compiler level setting is mismatched with JDK version being used. The good news is you can take control of this situation and modify your Java compiler settings to solve this issue.
To resolve this problem:
– Firstly, go to
File->Project Structure -> Project
. This takes you to your project’s details where you can view and edit properties such as Project SDK, language level and so on.
– Under Project SDK, ensure that you have selected the correct version for your application. If unsure, select a higher version like 11 or above.
An important note here is instead of downloading a new JDK manually, IntelliJ can do it for you. Just press
New...
next to the Project SDK dropdown and then select
Download JDK...
. IntelliJ will list all available versions for JDK (Java Development Kit) which includes both Oracle and OpenJDK.
– Then, under
language level
, set this field to be compatible with your declared Project SDK. For instance, if your Project SDK selected was 11, then consider choosing ’11 – Modules, local-variable syntax and etc.’.
This ensures that the set Language level value will empower you with certain language features associated with the specific Java version. When the selected language level is lower than the one supported by the actual SDK, some library elements will not be available.
– Secondly, on IntelliJ, go to
Settings/Preferences dialog Ctrl+Alt+S
, then choose
Build, Execution, Deployment | Compiler | Java Compiler
.
Changing the Target bytecode version to align with the Project SDK from step 1 is crucial. The Target bytecode version should match or be lower than the JDK version used. By modifying these settings, you’ll keep your compiler happy and prevent runtime exceptions related to incompatible or unsupported versions.
Setting | Description |
---|---|
Use compiler | Selects the approach/handling used to compile source code (ejc/Javac). |
Target bytecode version: | Specifies the version of the bytecode that your classes are compiled into. Should set to correspond with the Project SDK. |
– Lastly, apply these changes by pressing ‘OK’.
IntelliJ IDEA needs to know where to find the JDK, how to load the libraries, and which methods to run. Configuring it in the right way does the trick. Matching the JVM, language and target byte code levels, ensures that all parts of the environment understand each other without running into compatibility issues.
If you want to learn more, I definitely recommend reading the official IntelliJ Idea [documentation](https://www.jetbrains.com/help/idea/sdk.html). It provides detailed guidelines on how to extend existing applications with more capabilities from newer Java releases.Sure, facing the “Error:Java: Error: Release Version 5 Not Supported” message can be quite frustrating. This error occurs primarily when the Java Development Kit(JDK) you have installed doesn’t support the older version of Java you’re currently using in your IntelliJ IDEA project – JDK 8 or later simply no longer support target compliance levels below 1.6.
To fix this issue, I will guide you through a two-step resolution process which include:
1. Changing your Project’s SDK
2. Adjusting the Project Language Level
Changing your Project’s SDK
Your IntelliJ should be running on a software development kit (SDK) supportive of the language level used in your project. To rectify any incompatibilities, consider adjusting your project’s SDK by following these steps:
Check out the
Project Structure
then head to the
Project Settings | Project
. Click onto the
Project SDK
dropdown menu. Here comes the gist, if you do not see the updated version of the JDK you had installed, selecting the
Add New SDK
option and manually including your new JDK path(source).
Adjusting the Project language level
After modifying the SDK successfully, it’s pivotal next to adjust your project’s language level to match that of your newly updated JDK. This mitigates any chances of ending up in a situation where your Java source code contains features unsupported by your SDK.
The syntax checks run by IntelliJ IDEA as you write your code are set according to your specified language level. It is paramount therefore that your set Language level lies either at or below your SDK version.
Follow this navigation:
File | Project Structure | Project
. Subsequently from the
Project language level
dropdown, select an appropriate level that aligns with the aforementioned caveat. For example, for Java 1.8, select 8 – Lambdas, type annotations etc. (source)
Language Level | JDK Version |
---|---|
5 – Enumerations, autoboxing etc. | 1.5 |
6 – @Override in interfaces etc. | 1.6 |
7 – Diamonds, ARM, multi-catch etc. | 1.7 |
8 – Lambdas, type annotations etc. | 1.8 |
Upon attaining concurrence between your language level & SDK, recompile your project to determine whether the error has been eliminated. Post-resolution, remember that future endeavors needing higher versions of Java will require a similar adjustment process; KDE upgrades remain incompatible with lower language levels.
Moving forward, stay guided by a principle of ensuring compatibility between your Java version and the SDK integrated within your IDE, taking into account each update’s advancements like enhanced performance, improved memory management among more. Aligning these two prevents such errors and gives you a seamless Java programming experience. If your project requires an older version of Java, contemplating utilizing a JDK compatible with that version could salvage your project requirements without renegotiating your codebase’s stability.The “Error:Java: Error: Release Version 5 Not Supported” message means that the project you’re trying to compile is configured for version 5 of the Java JDK that IntelliJ IDEA doesn’t support. The issue here usually manifests when we have conflicts between java framework versions used within a project, and the java version installed on our system. Here are a few potential fixes that involve tweaking profiles and pom.xml configurations.
To begin with, ensure that you’ve installed an appropriate version of JDK on your system. You can verify this by typing
java -version
in your terminal or command prompt.
It’s also possible that your IDE may be compiling using a different JDK version than the one your project is targeting. In IntelliJ, navigate to File -> Project Structure -> Project and ensure the Project SDK matches the language level you wish to compile with.
Sometimes, while the installed JDK version might be up-to-date, IntelliJ’s compiler settings could point towards an old JDK version. To check and modify these settings, follow these steps:
– Go to File > Settings (or on Mac, IntelliJ IDEA > Preferences).
– Under Build, Execution, Deployment, locate the Compiler section.
– Increase the ‘Target bytecode version’ to match your installed JDK version.
If your project utilizes Maven, it might be that the pom.xml file specifies an unsupported version. To fix this, update the maven-compiler-plugin in your pom.xml file to the correct version. Here’s an example code snippet:
<build> <plugins> <plugin> <groupId>org.apache.maven.plugins</groupId> <artifactId>maven-compiler-plugin</artifactId> <version>3.8.1</version> <configuration> <source>[YOUR_VERSION]</source> <target>[YOUR_VERSION]</target> </configuration> </plugin> </plugins> </build>
Remember to replace [YOUR_VERSION] with your intended JDK version, such as 11.
With Maven you might want to set the JDK version for a specific profile. This comes handy when developers work with multiple Java versions or even different versions per environment.
<profiles> <profile> <id>java11</id> <activation> <jdk>11</jdk> </activation> <build> <plugins> <plugin> <groupId>org.apache.maven.plugins</groupId> <artifactId>maven-compiler-plugin</artifactId> <configuration> <source>11</source> <target>11</target> </configuration> </plugin> </plugins> </build> </profile> </profiles>
In the code above, the plugin will use JDK 11 when the profile id is ‘java11’.
Always remember, Google is your best friend. If you still find trouble after all these adjustments, don’t hesitate to search online for solutions or further details. Official documentations and coding forums are great resources in overcoming such challenges.When you face an error message such as “Error:Java: Release Version 5 not supported” in IntelliJ, this indicates a mismatch between the Java version used by Maven and the one expected by IntelliJ. This can be addressed by using the Maven Helper plugin for debugging.
Below are some key steps I suggest you take to resolve this issue:
1. Verify Your Project’s Java Version
Start by verifying the JDK used in your project and ensure it corresponds with your IntelliJ’s configuration. To check this, follow these steps:
Right-click on your project > Open 'Module Settings' > Go to the 'Module' section Look at the 'Project JDK' field, make sure it's set to the correct JDK version higher than 5.
If your JDK version is less than 5 or not set correctly, this could lead to the “Release version 5 not supported” error.
2. Update Project Settings and POM.xml Files
Ensure your Maven’s
pom.xml
file contains the correct JDK version in the properties section.
For example, if you’re using JDK 8, your
pom.xml
file should contain:
<properties> <maven.compiler.source>1.8</maven.compiler.source> <maven.compiler.target>1.8</maven.compiler.target> </properties>
Next, also ensure that your IntelliJ IDEA SDK settings match the
pom.xml
file configuration. You can verify this by following these steps:
Go to: File -> Project Structure Here under "Project", ensure you've set up the correct "Project SDK". Also, recheck the “Project language level” to fit your JDK version - e.g., “8 - Lambdas, type annotations etc.”
3. Utilize Maven Helper Plugin
The Maven Helper Plugin helps identify and fix discrepancies between the Maven and IDE configurations. It provides quick access to the effective POM of your projects and aids in debugging issues related to these configurations.
You can install the Maven Helper Plugin on IntelliJ using the below steps:
Go to: File -> Settings -> Plugins In the marketplace tab search for the Maven Helper plugin. Install it and restart the IDE.
After installing, the plugin will add a Maven Projects tool window and a Dependencies analyzer where you can debug maven-related issues.
Through the enhanced IDE-Maven integration provided by the Maven Helper plugin, you could possibly solve the “Release version 5 not supported” error.
Keep in mind, your environment might require specific tweaks and changes depending upon the systems and versions involved. Feel free to delve into more detailed information about the Java Compiler Plugin [source] or utilize extensive capabilities of the Maven Helper plugin [source] to customize your settings appropriately.When you encounter the error “Java: Error: Release version 5 not supported”, it’s likely indicating that your Intellij IDEA is configured incorrectly, or the Java version on your system does not support the specified Java language level.
The root cause of this error is quite simple – Intellij is set to a JDK version that doesn’t support the specified language level. JDK5, as the error suggests, pertains to release 5 of the Java Development Kit. The problem arises when Intellij IDEA uses a newer JDK but tries to compile to an older target bytecode version. Remember:
While specifying an older bytecode version can ensure compatibility with legacy systems running an older JVM, using such settings can lead to issues if your source code contains constructs not present in that older Java version.
In the context of this issue, we are asking a new JDK to generate bytecode compatible with an old JDK (like JDK 5), which isn’t viable and leads to the error.
To fix this error, one must either:
For example, if you’re using JDK 11 on your system, here is how you could adjust these settings in Intellij IDEA:
File -> Project Structure -> Project Settings -> Project -> Project language level -> '11- Local variable syntax for Lambda parameters' -> Project Settings -> Project -> Project SDK -> choose the appropriate JDK version
What this essentially means is you’re keeping the SDK and language level synchronised.
Altering the project structure in Intellij IDEA is typically the simplest way to fix this issue, notable because Intellij IDEA has user-friendly UI that grants easy access to its many configuration settings.
Regularly updating your JDK and ensuring settings consistency across toolkits prevents irritating hiccups like these. These changes help create a conducive environment that fosters seamless programming, enhancing your ability to deliver more projects successfully.
Please remember to update your JDK from authentic sources only to avoid software vulnerabilities. Even reputable programmers often forget this cardinal rule! Thorough documentation, like the Official Oracle Installation Instructions, provide secure directions to download and install, crucial to both your system’s health and your peace of mind. Knowledge bases like Stack Overflow compound on this by providing answers for tackling common – and uncommon – coding errors!
Check out this related Stack Overflow thread for more insights into this topic.
Overall, remember to keep your JDK updated and maintain your compiler’s language level across all development environments. This advice keeps those irksome bugs at bay, allowing you to hit deadlines without any java.lang headaches interrupting your productivity.
Or course, other factors in connection to this error are worth exploring. Antivirus interference, background processes causing high memory usage, discrepancies between system JDK and Intellij IDE can also contribute towards plausible solutions. But those are stories for another day!
More further troubleshooting advice can be found in the renowned Intellij IDEA community.